In C++, the `std::cout` object is used to print output to the console, often employing the insertion operator `<<` to display messages or results.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is `println` in C++?
Understanding Output Functions
In C++, output operations are vital for communicating with users and for debugging purposes. The primary way to output text or data to the console is through the use of output streams. The `std::cout` object, part of the standard input-output library (`<iostream>`), serves as the main conduit for console output.
The Idea Behind `println`
Unlike some programming languages such as Java which incorporate a built-in `println` function, C++ does not have a dedicated `println` method. Instead, output in C++ is achieved through a combination of the `std::cout` stream and manipulators that dictate specific behaviors, such as adding a new line.
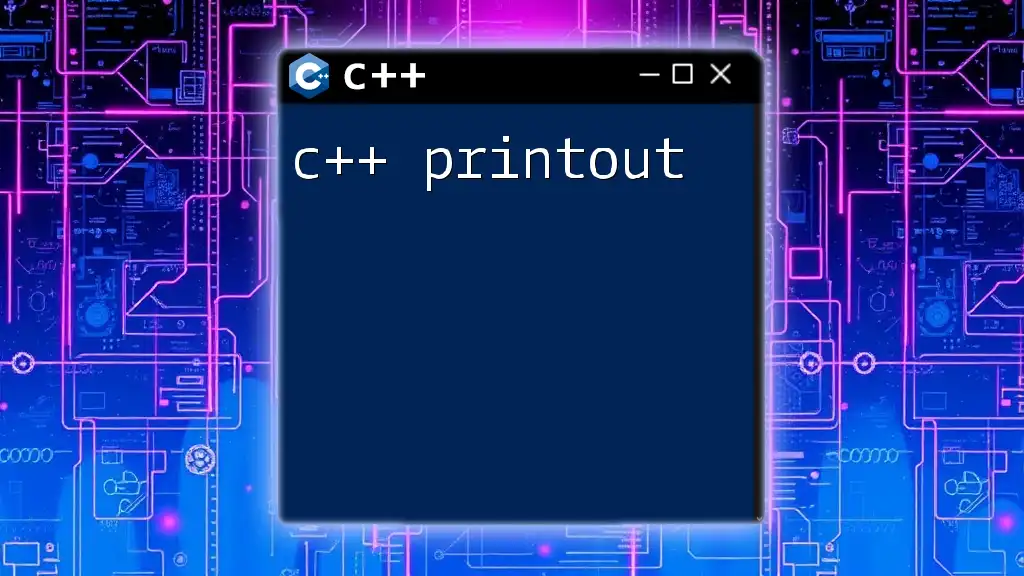
How to Achieve `println` Functionality in C++
Using `std::cout` with Line Breaks
To print text followed by a line break, you can accomplish this by using `std::cout` in conjunction with the `std::endl` manipulator. This sends the output followed by a newline character and flushes the output buffer, ensuring that the output is displayed immediately. Here is a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code, “Hello, World!” is printed to the console, followed by a new line. The main function serves as the entry point of the program, where the actions take place.
Employing `std::endl` and `\n`
Differences Between `std::endl` and `\n`
- `std::endl` not only inserts a newline but also flushes the stream, which can enhance output responsiveness but may also incur a performance cost.
- `\n`, on the other hand, simply adds a newline character without flushing, making it a more efficient choice in scenarios where immediate output isn't necessary.
When to Use Which
Understanding when to use `std::endl` versus `\n` is crucial. If you're printing a large volume of output and performance is a concern, utilizing `\n` can be advantageous. However, if you need to ensure that the user sees the output immediately, especially in debugging scenarios, prefer `std::endl`.
Custom `println` Function
Creating a Function
To mimic the convenience of a `println` method, you can create a custom function. This encapsulation enhances code reusability and can lead to more organized programs. Here’s an example:
#include <iostream>
void println(const std::string &msg) {
std::cout << msg << std::endl;
}
int main() {
println("Hello, World!");
return 0;
}
This `println` function takes a string as an argument and outputs it with a newline. It simplifies printing tasks throughout your code, providing a more consistent experience.
Overloading the `println` Function
To enhance usability further, you can overload the `println` function to accept various data types, like integers and floating-point numbers. Here’s how you can do it:
#include <iostream>
void println(const std::string &msg) {
std::cout << msg << std::endl;
}
void println(int value) {
std::cout << value << std::endl;
}
void println(float value) {
std::cout << value << std::endl;
}
int main() {
println("The value is:");
println(42);
println(3.14f);
return 0;
}
This allows you to call `println` with different types seamlessly, making your code cleaner and more expressive.
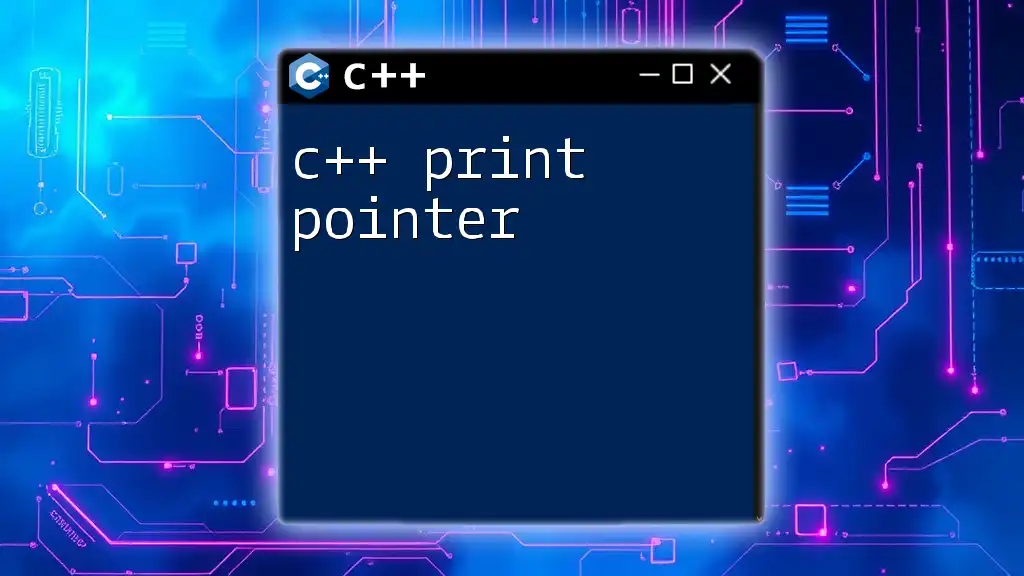
Best Practices for Using `println` in C++
Consistency in Output Formatting
Consistency in how you present output enhances readability and user experience. Consider utilizing output manipulators like `std::fixed`, `std::setprecision`, etc., to format numerical outputs consistently. For instance, if you are dealing with floating-point numbers, you can control the number of decimal places shown:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::fixed << std::setprecision(2);
std::cout << "Pi is approximately: " << 3.14159 << std::endl;
return 0;
}
This code snippet formats the output to show two decimal places, improving clarity.
Efficient Debugging with Output
Proper output statements can significantly aid debugging. By strategically placing `println` calls in your code, you can inspect variable states at runtime. Here's another example:
int main() {
int x = 10;
int y = 20;
std::cout << "Debug: Variable x = " << x << ", Variable y = " << y << std::endl;
return 0;
}
Here, the debug statement presents the values of `x` and `y`, making it easier to trace and verify program flow.
Performance Considerations
While it’s easy to become enamored with output functions, be mindful of performance. Frequent use of `std::endl` can lead to performance issues, especially in loops where output operations are extensive. In such cases, prefer using `\n`.
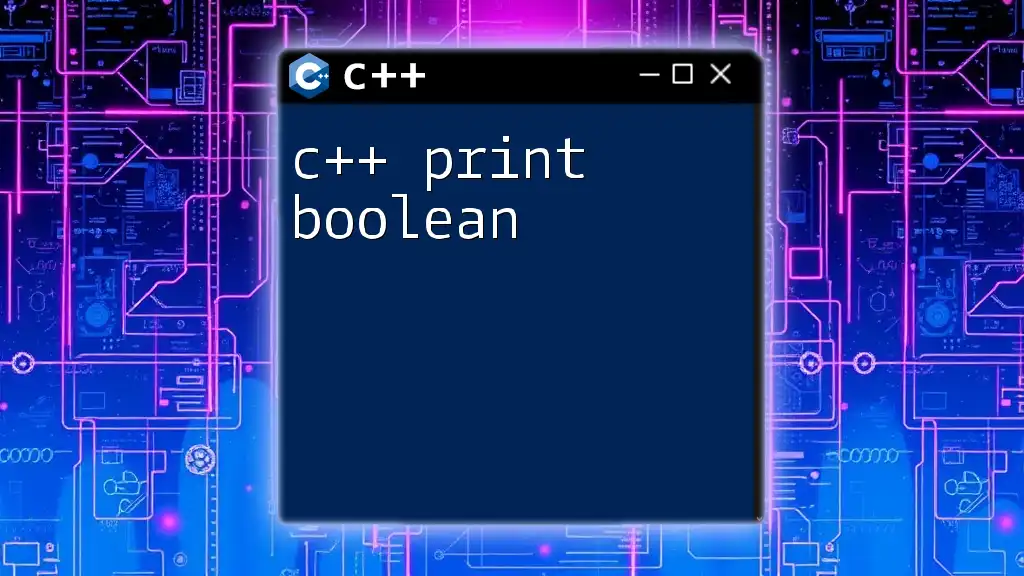
Common Mistakes to Avoid
Misunderstanding of Output Buffers
One common pitfall in console output is misunderstanding how C++ buffers output. If you neglect to flush the stream (intentionally or through mistaken use of `\n`), output may not appear when you expect it. Always be aware of how your output functions interact with the I/O buffer.
Forgetting to Include Headers
Always ensure that you include the appropriate header files. Failing to include `<iostream>` when attempting to use `std::cout` will lead to compilation errors. Keep your code organized and well-commented to prevent such omissions.
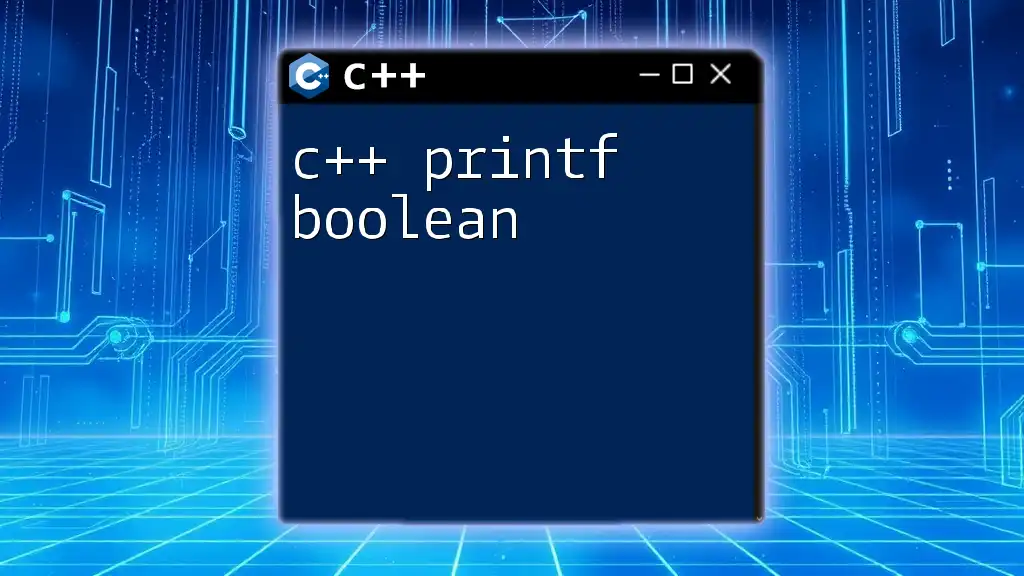
Conclusion
Understanding how to handle output effectively in C++ is foundational for both beginners and seasoned developers. While C++ lacks a native `println` function, through the use of `std::cout` and manipulators, coupled with custom functions, you can easily replicate its functionality. Efficient output handling not only enhances user experience but also aids in debugging and code maintenance. As you expand your programming skills, practice implementing your own output methods and employ these best practices to elevate your C++ proficiency.