The C++ print command, commonly realized using the `std::cout` object from the iostream library, allows you to output text or variables to the console. Here's a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the C++ Print Command
What is the C++ Print Command?
The C++ print command is primarily executed through the `std::cout` statement, which belongs to the C++ Standard Library. This command is crucial for outputting information to the console, allowing developers to display text, variables, and other data values. In essence, it serves as the primary means of communication between the program and the user.
Common Cases for Using Print Command
Printing is essential in various scenarios:
- Displaying Variable Values: To show current values stored in variables.
- Debugging Applications: To trace the flow of the program or understand the values of variables at different states.
- Outputting User Prompts and Information: To guide users in inputting data or understanding the program's output.
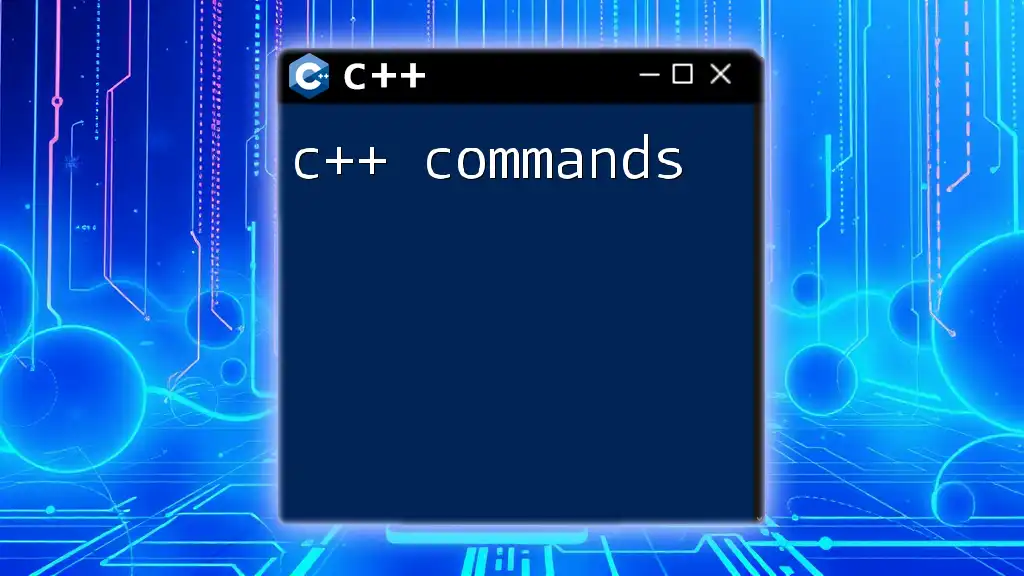
The C++ Print Statement: Syntax and Structure
Basic Syntax of the C++ Print Statement
The syntax for the C++ print statement typically follows this structure:
std::cout << "Your message" << std::endl;
This format involves the use of the `std::cout` stream, along with the stream insertion operator (`<<`) to send data to the output console.
Breakdown of the Syntax
- `std::cout`: This specifies that we are using the standard output stream provided by the C++ Standard Library.
- `<<` Operator: This operator is used to insert the data into the output stream.
- `"Hello, World!"`: This string is an example of the type of information you can print. In C++, strings are enclosed in double quotes.
- `std::endl`: This is a manipulator that sends a newline character to the output, effectively moving the cursor to the next line, and flushes the output buffer.
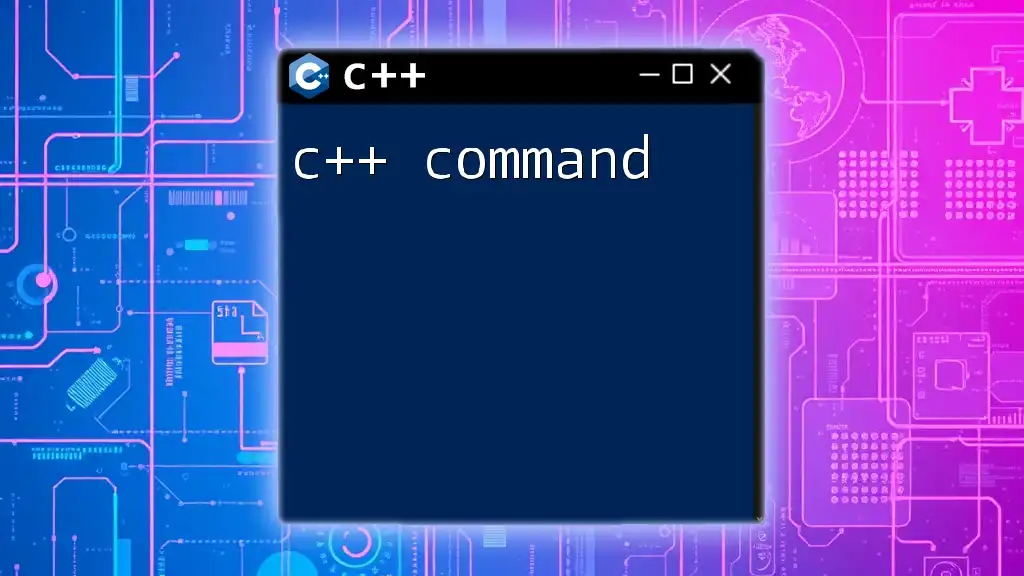
Advanced Print Techniques
Printing Multiple Values
You can use the print statement to output more than one variable or string by chaining the `<<` operator. This is useful for displaying related information together.
#include <iostream>
int main() {
int age = 25;
double height = 5.9;
std::cout << "Age: " << age << ", Height: " << height << " feet" << std::endl;
return 0;
}
In this example, multiple variables are displayed in a single line, enhancing readability and coherence.
Formatting Output
C++ offers various ways to format output, making it presentable. This is particularly useful for controlling the number of decimal places, aligning text, and more.
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << "Value of Pi: " << pi << std::endl;
return 0;
}
In the above code, `std::fixed` and `std::setprecision(2)` are manipulators that adjust the output format of floating-point numbers, ensuring that the value of Pi is displayed with two decimal places.
Special Characters in C++ Print Statement
Special characters, also known as escape sequences, enhance the output of print statements. Here are some common ones:
- `\n`: Newline character that moves the cursor to a new line.
- `\t`: Horizontal tab that adds spacing in the output.
Example showcasing these escape sequences:
std::cout << "First Line\nSecond Line" << std::endl; // \n for new line
std::cout << "Tab\tIndented" << std::endl; // \t for horizontal tab
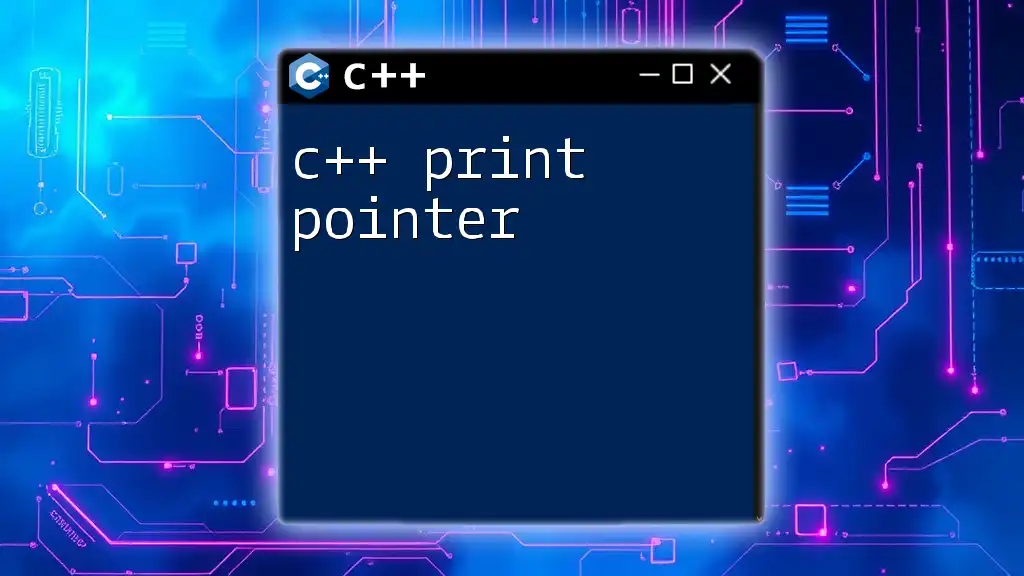
Handling User Input with Print Statements
Prompting User Input
You can effectively guide users to provide necessary input by utilizing print statements to prompt for input. This enhances user interaction with the application.
#include <iostream>
int main() {
int num;
std::cout << "Enter a number: ";
std::cin >> num;
std::cout << "You entered: " << num << std::endl;
return 0;
}
In this example, the program asks users to enter a number, showcasing how print statements can be intertwined with user input to create dynamic applications.
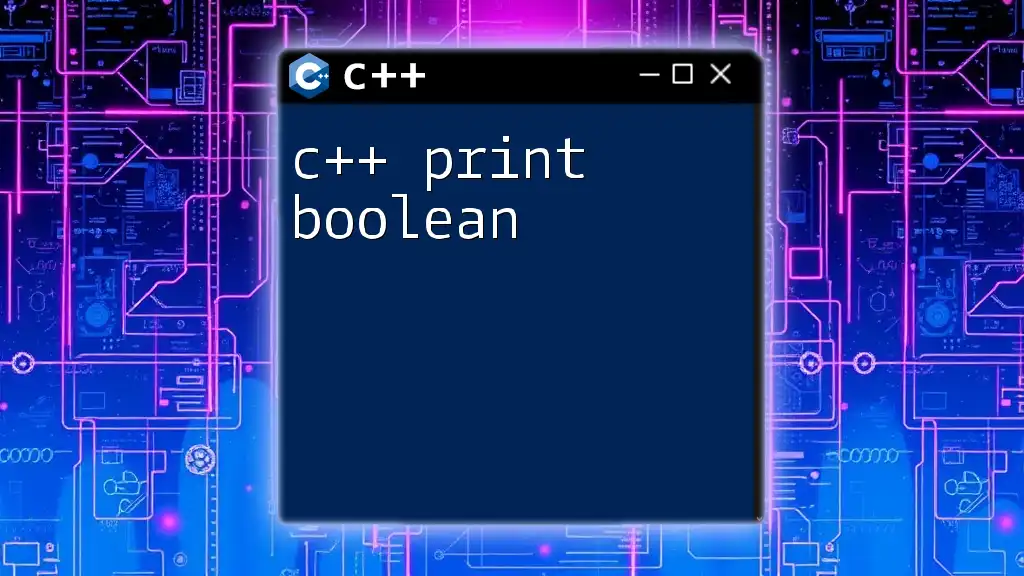
Common Mistakes with C++ Print Command
Not Using the Proper Namespace
One common mistake is forgetting to qualify `cout` with the `std::` namespace. This can lead to compilation errors. To avoid such issues, always ensure that you either use `std::cout` or include `using namespace std;` at the start of your program.
Forgetting to Add std::endl
Another mistake is neglecting to include `std::endl` after output statements, which can lead to unexpected behavior. Without it, the next printed output may not appear on a new line, making the console output hard to read.
// Without std::endl
std::cout << "No new line";
std::cout << "This continues on the same line." << std::endl;
Output:
No new lineThis continues on the same line.
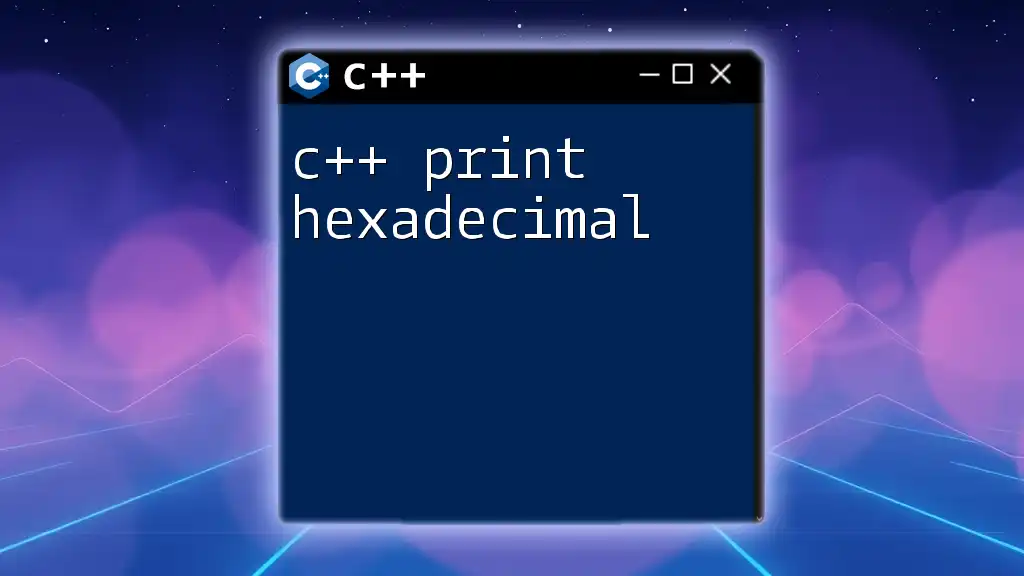
Best Practices for Using Print Statements in C++
Keep It Concise
When using the C++ print command, it's important to deliver messages clearly and efficiently. Avoid cluttering the output with unnecessary information. A concise output is easier for users to read and comprehend.
Be Mindful of Formatting
The formatting of printed output is essential for readability. Consistency in format styles, spacing, and use of uppercase/lowercase letters can significantly enhance the user's interaction with the program.
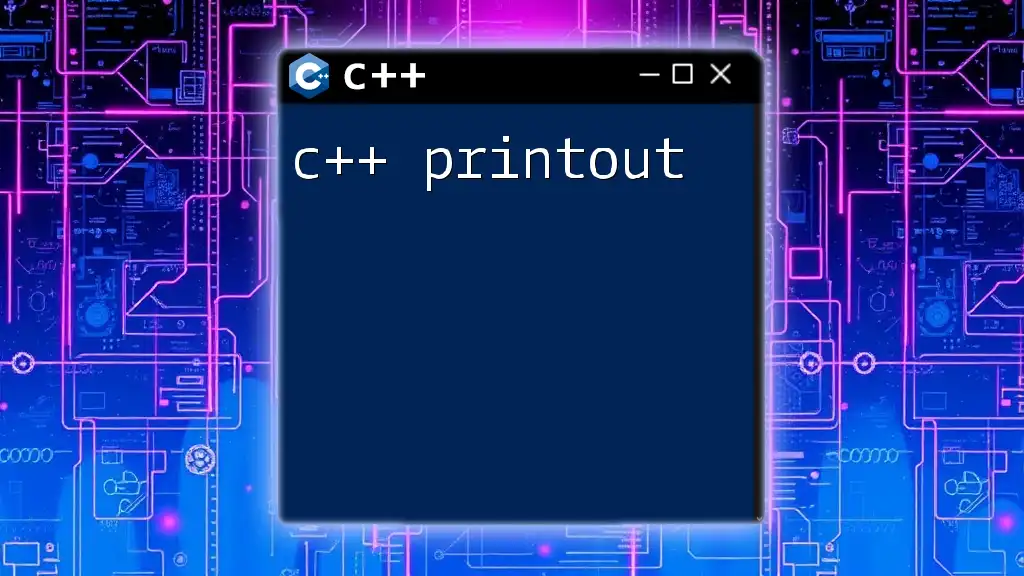
Conclusion
Throughout this article, we explored the various aspects of the C++ print command, including its syntax, advanced techniques, and best practices. Mastering these concepts is vital for effective programming in C++. As you continue to learn C++, utilizing the print command effectively will enhance your ability to communicate with users and debug your applications.