C++ commands refer to the instructions or statements used to perform various operations in C++ programming, and here's a simple example that demonstrates how to output text to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Basic C++ Commands
Variables and Data Types
In C++, variables are used to store data values. Before using a variable, you must first declare it. This includes specifying its type, which informs the compiler what kind of data the variable will hold.
Declaring Variables
You can declare variables like this:
int number; // integer variable
float price; // floating-point variable
Here, `int` and `float` are data types.
Data Types Overview
C++ supports several built-in data types:
- int: Used for integers (whole numbers).
- char: Represents a single character (e.g., 'a', 'B').
- float: Used for single-precision floating-point numbers.
- double: For double-precision floating-point numbers.
- bool: Represents Boolean values (true or false).
Understanding these data types is fundamental, as they affect how data is stored and manipulated in memory.
Operators
Operators in C++ allow you to perform operations on variables and values.
Arithmetic Operators
These include basic functions such as addition, subtraction, multiplication, and division. For example:
int sum = a + b; // addition
int product = a * b; // multiplication
Relational Operators
These operators are used to compare two values. They include:
- `==` (equal to)
- `!=` (not equal to)
- `>` (greater than)
- `<` (less than)
- `>=` (greater than or equal to)
- `<=` (less than or equal to)
Logical Operators
Logical operators allow you to combine multiple conditions. They include:
- `&&` (AND)
- `||` (OR)
- `!` (NOT)
For an example:
if (a > b && b < c) {
// your code here
}
This checks if 'a' is greater than 'b' and 'b' is less than 'c'.
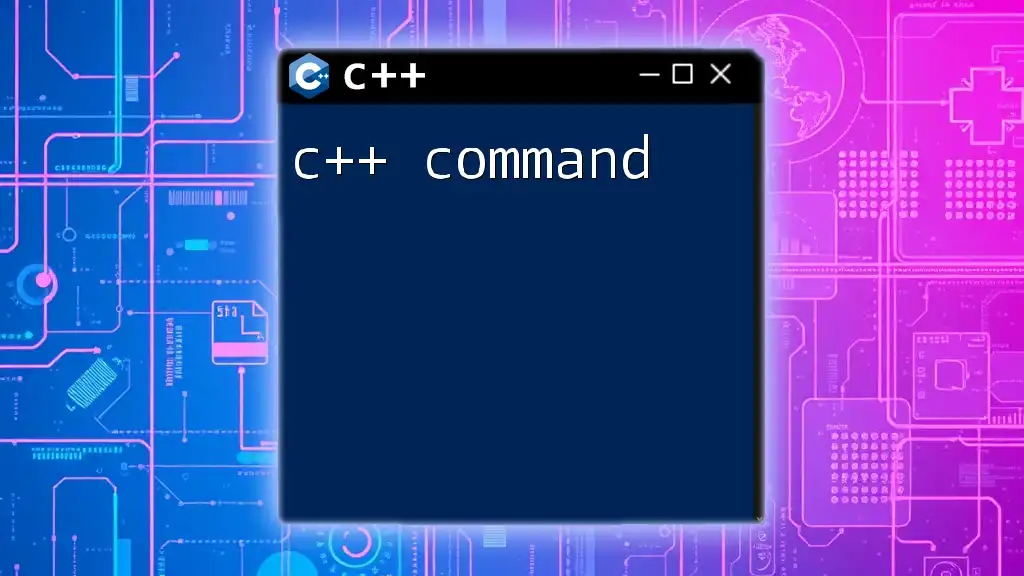
Control Flow Commands
Control flow commands allow your program to execute different sections of code based on certain conditions or iterations.
Conditional Statements
If Statement
One of the most commonly used constructs. It allows for conditional execution of code.
if (condition) {
// code to execute if condition is true
}
You can enhance its functionality with `else` and `else if` for additional conditions.
Switch Statement
Useful for executing different parts of code based on the value of a variable.
switch (variable) {
case 1:
// code for case 1
break;
case 2:
// code for case 2
break;
default:
// code if no cases match
}
Looping Statements
For Loop
A loop that iterates a specific number of times.
for (int i = 0; i < n; i++) {
// code to execute
}
The `for` loop is particularly useful for counting iterations.
While Loop
Continues to execute as long as the condition remains true.
while (condition) {
// code to execute
}
Do-While Loop
Similar to the `while` loop, but it guarantees at least one execution of the loop body.
do {
// code to execute
} while (condition);
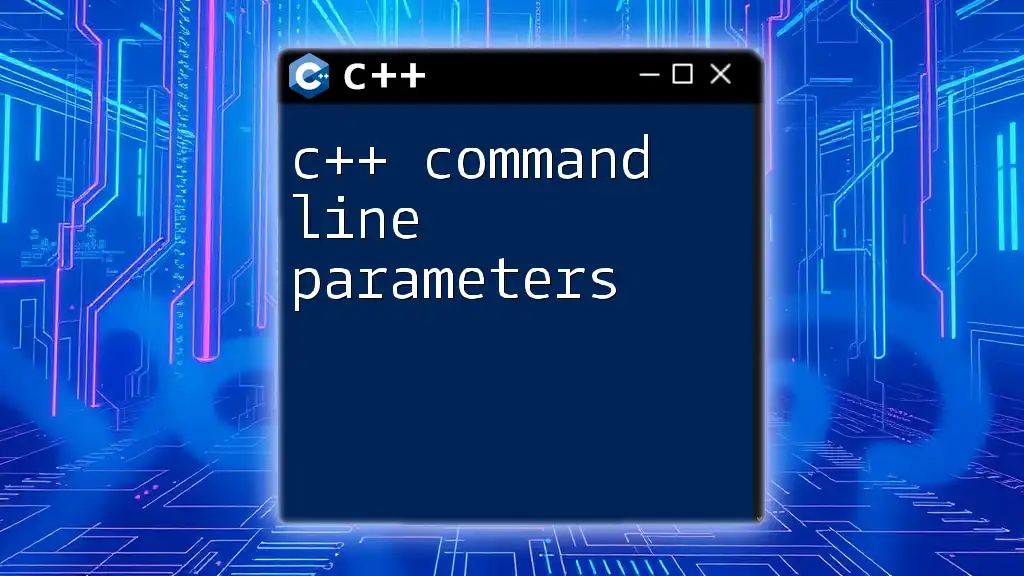
Functions in C++
Functions allow you to group a set of related operations into a single block, making your code cleaner and reusable.
Defining Functions
Syntax and Structure
A function is defined by its return type, name, and parameters. Here’s an example:
returnType functionName(parameters) {
// function body
}
For instance:
int add(int a, int b) {
return a + b;
}
Function Overloading
C++ allows you to define multiple functions with the same name but different parameters. This is known as function overloading.
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Inline Functions
Benefits of Inline Functions
Using `inline` can improve performance by reducing the overhead of function calls. An inline function is defined using the `inline` keyword:
inline int square(int x) {
return x * x;
}
This can be particularly beneficial for small, frequently-used functions.
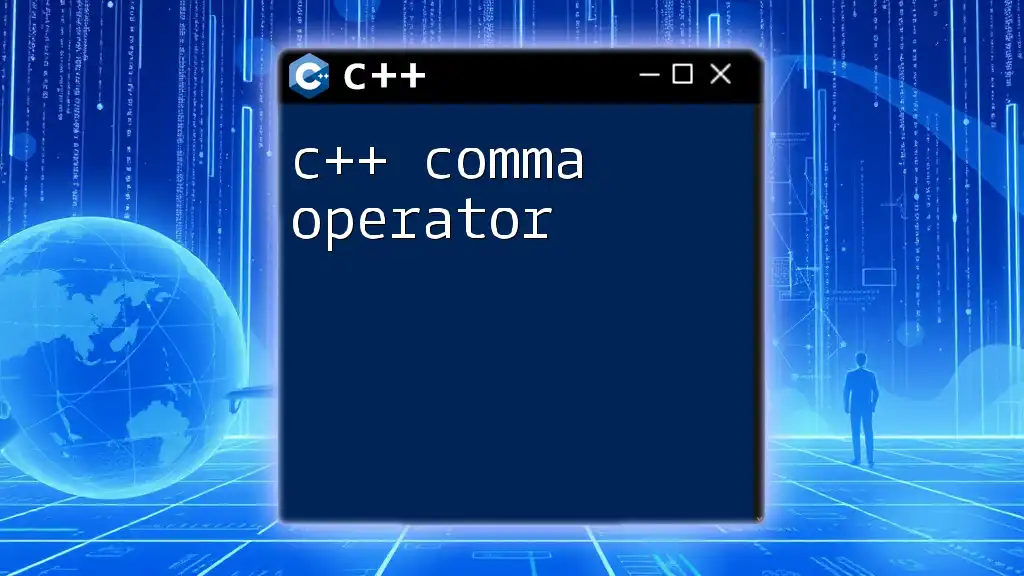
Input and Output Commands
Input and output commands are essential for interacting with users and other systems.
Using Input/Output Streams
`cin` and `cout`
These are part of the iostream library and are used for basic input and output processes. You can prompt for input and display output as follows:
cout << "Enter a number: ";
cin >> number; // capturing user input
You can chain together multiple outputs using `<<`.
File I/O
Reading from Files
Utilizing file streams allows you to interact with file data. Here is a simple example:
#include <fstream>
std::ifstream inFile("input.txt");
std::string line;
while (getline(inFile, line)) {
// process each line
}
Writing to Files
You can also write data to files similarly:
#include <fstream>
std::ofstream outFile("output.txt");
outFile << "Hello, World!";
File operations are crucial for data persistence in applications.
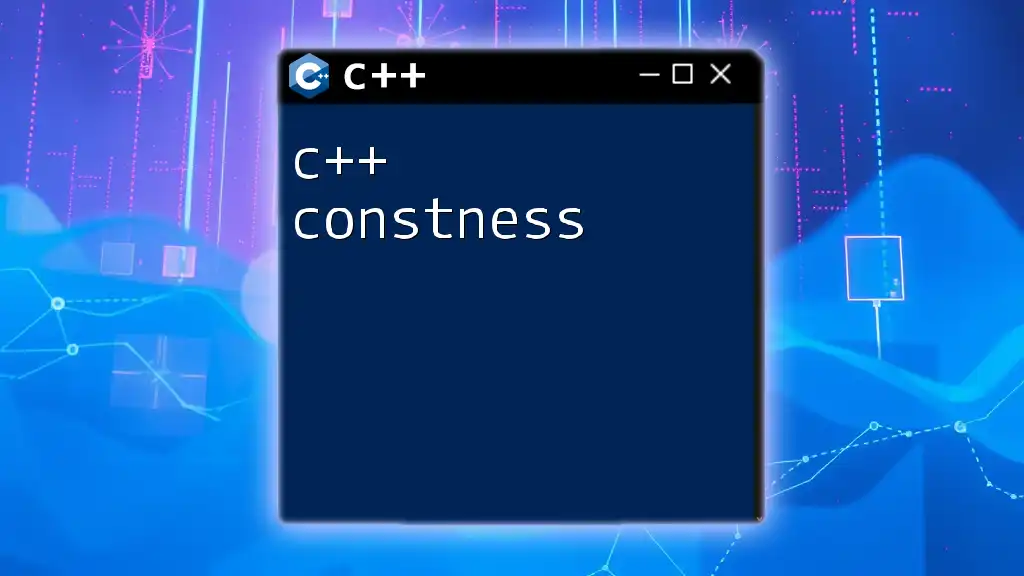
Object-Oriented Commands
C++ supports object-oriented programming, which can greatly enhance data management through encapsulation, inheritance, and polymorphism.
Classes and Objects
Defining a Class
A class acts as a blueprint for creating objects. You define a class as follows:
class Car {
public:
void drive() {
// implementation
}
};
Creating Objects
To create an object (or instance of a class), you can do:
Car myCar; // instantiate a Car object
myCar.drive(); // call the drive method
Inheritance and Polymorphism
Understanding Inheritance
Inheritance allows one class to inherit properties and methods from another. Here’s a simple example:
class Vehicle {
public:
void start() {
// common start method
}
};
class Car : public Vehicle {
// Car inherits from Vehicle
};
Polymorphism in C++
This concept allows methods to do different things based on the object that it is acting upon. It can be achieved through:
- Compile-time polymorphism (function overloading or operator overloading)
- Runtime polymorphism (using virtual functions).
Example of a virtual function:
class Vehicle {
public:
virtual void honk() {
// generic honk
}
};
class Car : public Vehicle {
public:
void honk() override {
// specific car honk
}
};
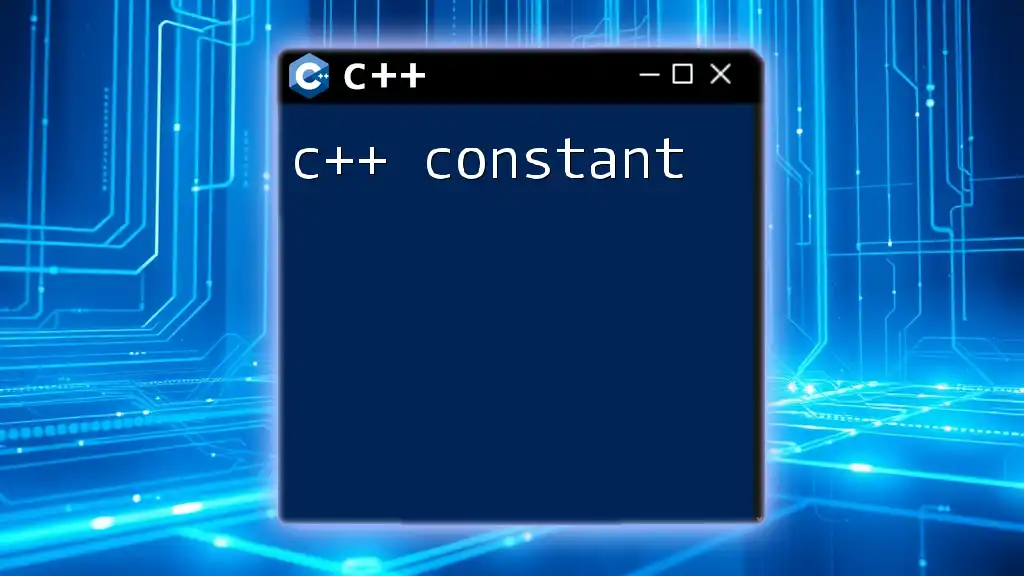
Commonly Used C++ Commands
Using the Standard Template Library (STL)
The STL provides standard data structures, algorithms, and iterators. It simplifies tasks significantly while providing robust implementations.
Introduction to STL
Commonly used components include vectors (dynamic arrays), lists (doubly linked lists), sets, and maps (key-value pairs). For example, a vector can be declared and used as follows:
#include <vector>
std::vector<int> numbers; // declaring a vector
numbers.push_back(10); // adding an element
Memory Management Commands
Dynamic Memory Allocation
Utilizing `new` for dynamic memory allocation allows for flexibility:
int* array = new int[size]; // allocate memory dynamically
Deallocating Memory
Once you are done with the memory, it’s essential to free it:
delete[] array; // deallocate memory
Failure to do so can lead to memory leaks, which are critical to manage in larger applications.
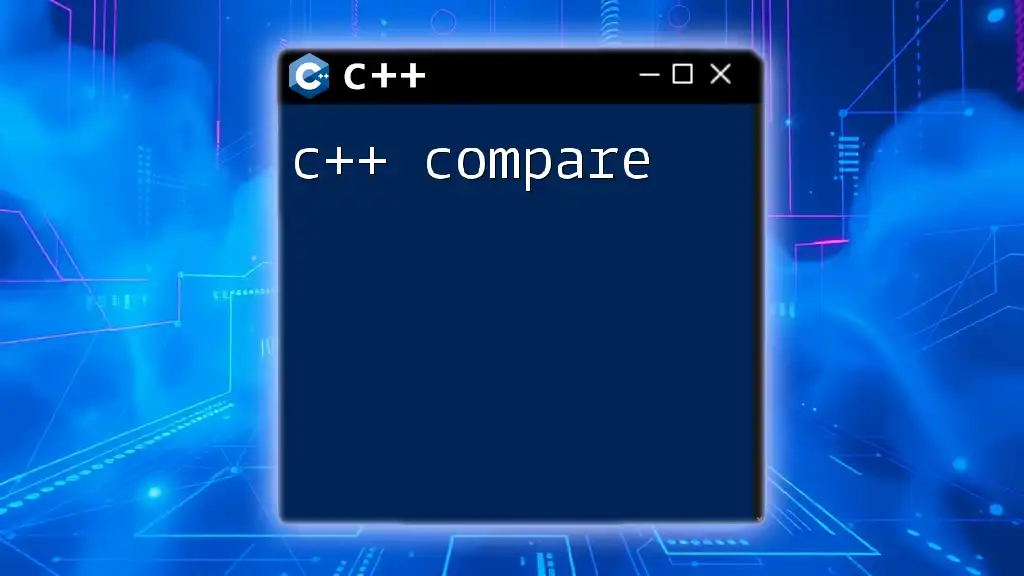
Conclusion
Understanding C++ commands is vital for anyone looking to become proficient in programming. The foundation built on variables, operators, control structures, and object-oriented concepts will empower you to write efficient and effective code.
Further Learning Resources
To enhance your skills, consider exploring various books, online courses, and official documentation. Engaging with communities can also help you deepen your understanding and problem-solving abilities.
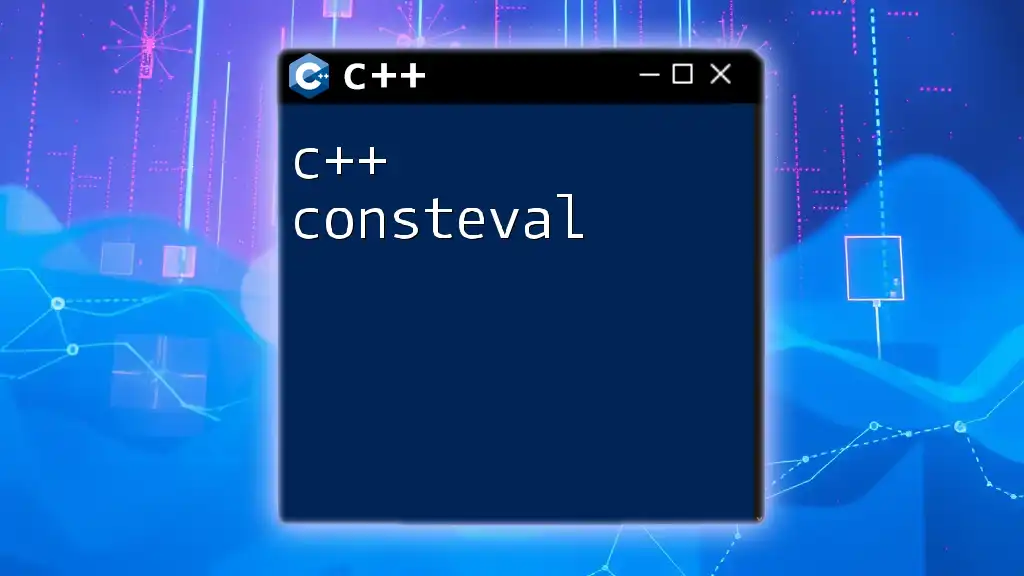
Call to Action
Join our C++ learning community today to immerse yourself in hands-on experience, interactive lessons, and mentorship opportunities. Start your journey into the world of C++ commands now!