C++ command line parameters are used to pass information to a program at the time of execution, enabling the program to process input values directly from the command line.
Here’s a simple example demonstrating how to access command line parameters in C++:
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of parameters: " << argc << std::endl;
for (int i = 0; i < argc; i++) {
std::cout << "Parameter " << i << ": " << argv[i] << std::endl;
}
return 0;
}
Understanding Command Line Arguments in C++
Command line arguments are a powerful feature in C++ programming, allowing users to pass inputs to a program at the time of execution. They play a crucial role in making applications dynamic and customizable.
What are Command Line Arguments?
While often used interchangeably, it's important to differentiate between command line parameters and command line arguments. Command line parameters refer to the inputs a program can accept, while command line arguments are the actual values provided by the user.
How Command Line Arguments Work in C++
In C++, command line arguments are handled in the `main()` function, typically defined as:
int main(int argc, char* argv[]) {
// Your code here
}
Here, `argc` (argument count) represents the number of command line arguments passed, including the program name, while `argv` (argument vector) is an array of C-style strings that holds the arguments.
For instance, if a program is run as follows:
./my_program Hello World
`argc` would be 3, and `argv` would contain:
- `argv[0]` = "./my_program"
- `argv[1]` = "Hello"
- `argv[2]` = "World"
This foundational understanding sets the stage for exploring how to efficiently utilize command line parameters in C++.
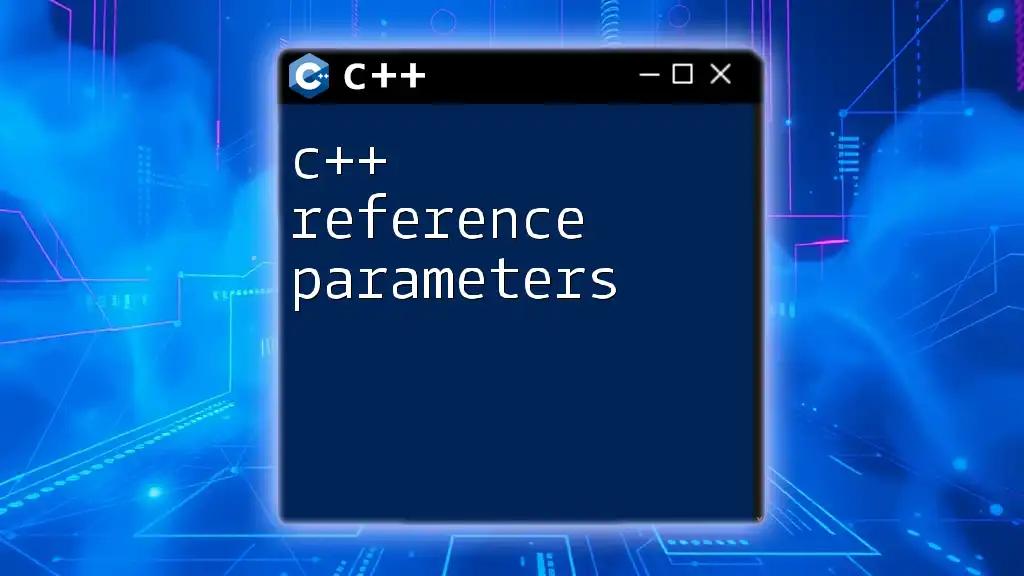
Accessing Command Line Parameters in C++
Basic Access Methods
Accessing command line parameters is straightforward with `argv`. Here's a simple example that displays all parameters passed to a program:
#include <iostream>
int main(int argc, char* argv[]) {
for (int i = 0; i < argc; i++) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
In this example, the loop iterates through all arguments, printing them to the console. This output provides a clear view of the inputs the program received.
Parsing Command Line Arguments
In more complex applications, you often need to parse those arguments intelligently. For instance, if you want to accept a user's name from command line inputs, you might write:
#include <iostream>
#include <cstring>
int main(int argc, char* argv[]) {
if (argc > 2 && strcmp(argv[1], "--name") == 0) {
std::cout << "Hello, " << argv[2] << "!" << std::endl;
} else {
std::cout << "Usage: " << argv[0] << " --name <YourName>" << std::endl;
}
return 0;
}
Here, the program checks if the first argument matches `--name` and prints a welcome message.
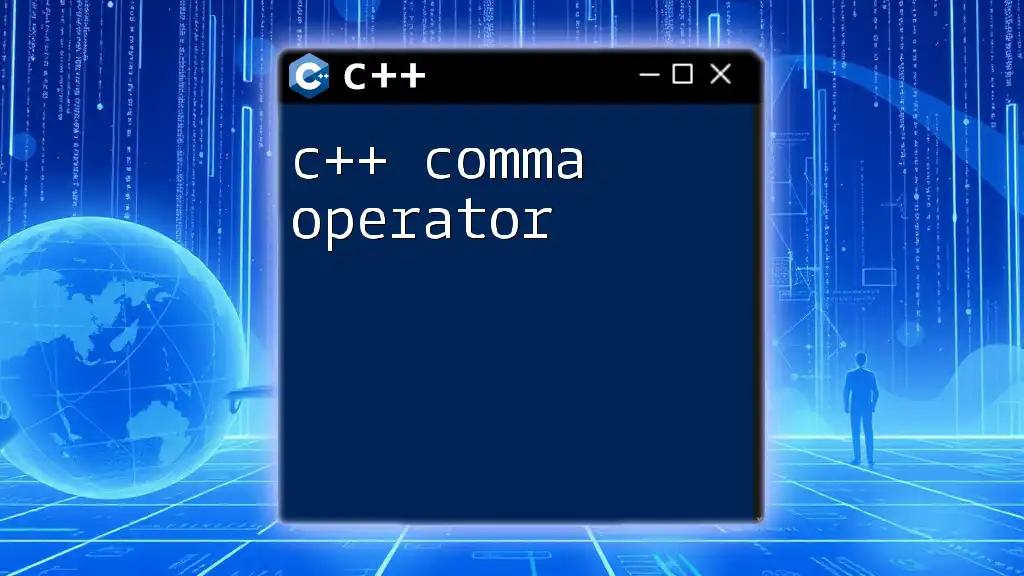
Advanced Usage of Command Line Parameters in C++
Handling Options and Flags
When designing a command line interface, using options and flags can enhance functionality. Consider a scenario where you accept a flag for displaying help information:
#include <iostream>
int main(int argc, char* argv[]) {
if (argc > 1) {
if (strcmp(argv[1], "--help") == 0) {
std::cout << "Help: Use --name <YourName> to greet." << std::endl;
}
}
return 0;
}
This code demonstrates how you can implement conditional logic based on user input, allowing for better control over application behavior.
Using Libraries for Command Line Parsing
For applications with more complex command line requirements, libraries like Boost.Program_options can simplify argument parsing significantly. First, you’ll need to install the Boost library, if not already done.
Here's how you can set up `Boost.Program_options`:
#include <boost/program_options.hpp>
#include <iostream>
#include <string>
namespace po = boost::program_options;
int main(int argc, char* argv[]) {
po::options_description desc("Allowed options");
desc.add_options()
("help,h", "produce help message")
("name,n", po::value<std::string>(), "Name to display");
po::variables_map vm;
po::store(po::parse_command_line(argc, argv, desc), vm);
po::notify(vm);
if (vm.count("help")) {
std::cout << desc << std::endl;
}
if (vm.count("name")) {
std::cout << "Hello, " << vm["name"].as<std::string>() << "!" << std::endl;
}
return 0;
}
This code introduces a dedicated namespace that handles options neatly, allowing your program to be easily extensible for adding new parameters in the future.

Error Handling and Validation
Checking for Proper Input
Validation is essential to ensure your application runs smoothly. Incorrect inputs can lead to unexpected behavior. For example:
if (argc < 2) {
std::cerr << "Usage: " << argv[0] << " <param>" << std::endl;
return 1;
}
This snippet checks if the required arguments are given and prompts the user accordingly.
Handling Errors Gracefully
You should also handle unexpected values to prevent crashes and inform the user. Here’s a simple validation check:
if (!is_valid(argv[1])) {
std::cerr << "Invalid parameter provided." << std::endl;
return 1;
}
In this context, defining a function like `is_valid()` could include checks for allowed characters or specific formats.
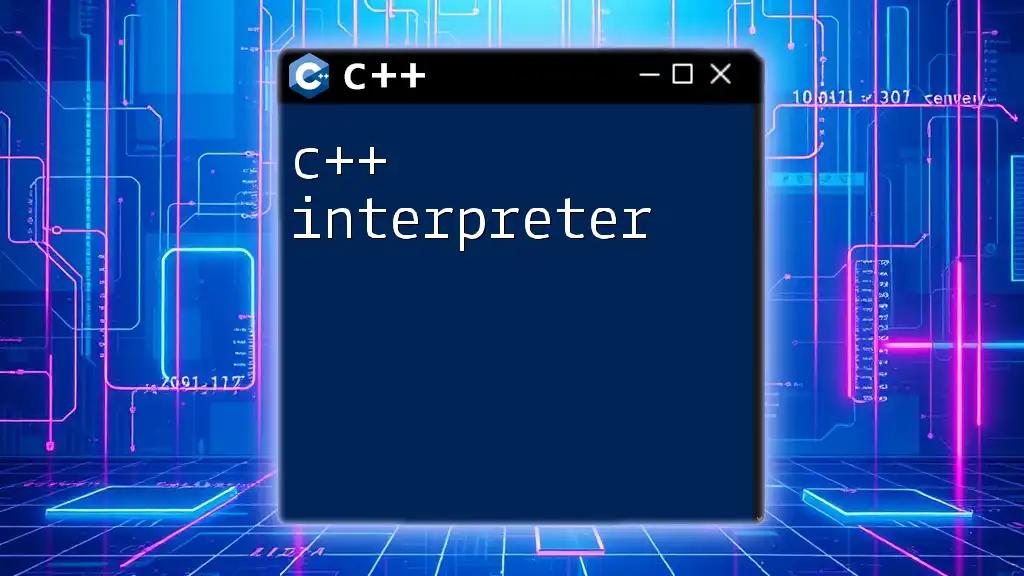
Practical Examples and Use Cases
Building a Simple Command Line Application
Creating a calculator that receives numbers as command line parameters can be an excellent exercise in using command line arguments:
#include <iostream>
#include <cstdlib>
int main(int argc, char* argv[]) {
float sum = 0;
for (int i = 1; i < argc; i++) {
sum += atof(argv[i]);
}
std::cout << "Total: " << sum << std::endl;
return 0;
}
In this example, every number entered as an argument is converted to a float and summed up, demonstrating how command line parameters can drive program logic.
Real-world Use Case Demonstrations
Classically, command line parameters find utility in many applications, like automation scripts or data processing tools. For instance, a file processing program could accept a filename argument to specify which file to process, enhancing usability and flexibility.

Best Practices for Using C++ Command Line Parameters
Keep It User-Friendly
Designing intuitive command line interfaces is crucial. Ensure that the usage of commands is clear and that the program provides helpful feedback when mistakes occur.
Documenting Command Line Features
Clear documentation of how to utilize command line parameters will significantly improve user experience. This transparency fosters confidence in users while interacting with your application.
Testing Command Line Applications
Thoroughly test command line interfaces in various scenarios to ensure they handle input robustly. This practice helps catch edge cases that could lead to unexpected behavior in your application.
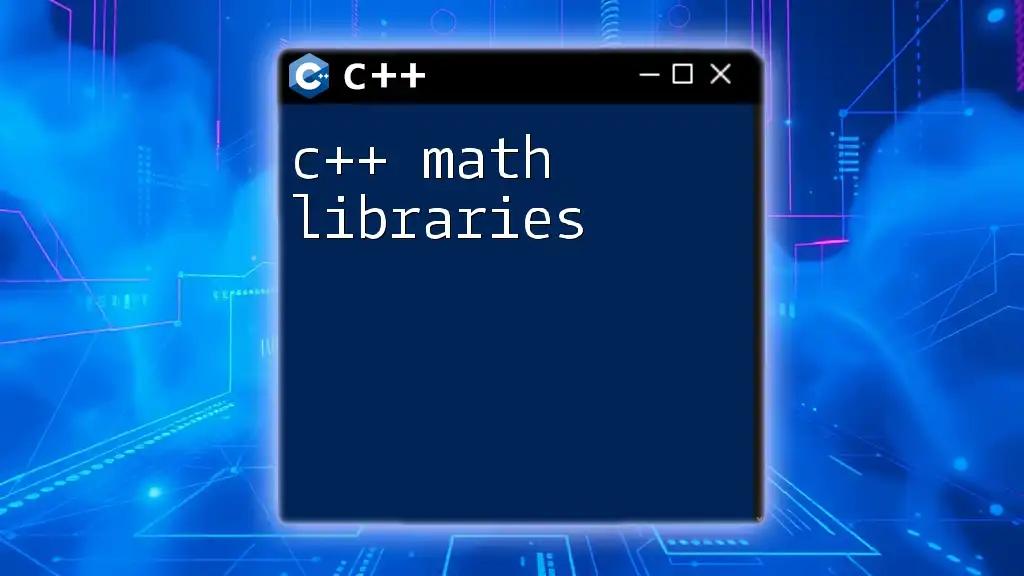
Conclusion
C++ command line parameters empower users to control program behavior dynamically, enhancing interactivity and adaptability. With a solid understanding of how to implement and manage these parameters, you can create efficient and user-friendly applications. Experimenting with your command line capabilities not only boosts your programming skills but also opens up new possibilities in software design.
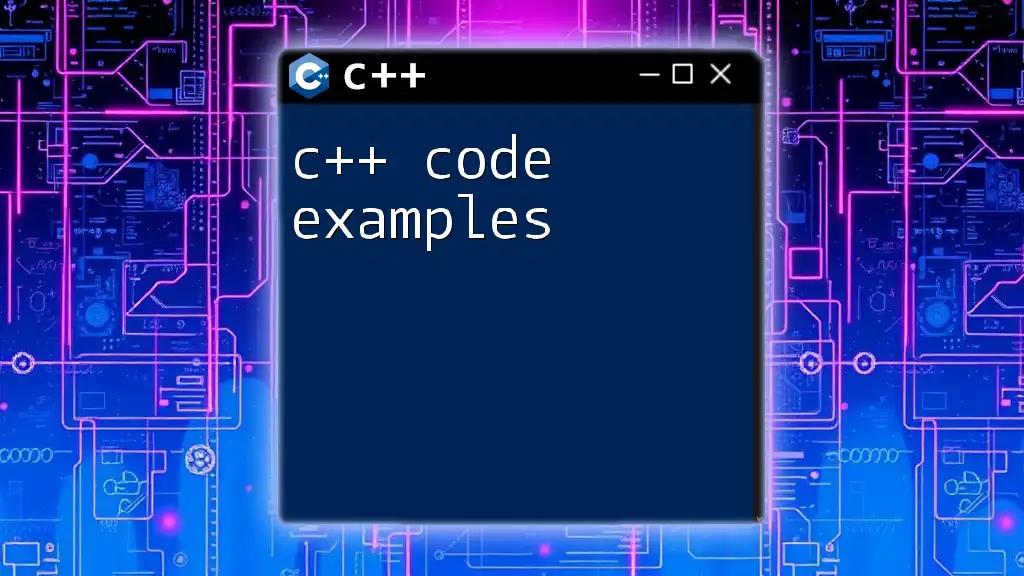
Additional Resources
To delve deeper into C++ command line parameters and enhance your programming knowledge, consider resources such as books on C++ programming and comprehensive guides on relevant libraries like Boost. Exploring these materials will provide you with valuable insights, further enriching your skill set in utilizing command line features effectively.