A C++ parameter pack allows you to handle a variable number of template parameters in a function or class template, enabling more flexible and generic programming.
#include <iostream>
#include <tuple>
template<typename... Args>
void printArgs(const Args&... args) {
(std::cout << ... << args) << '\n'; // Fold expression
}
int main() {
printArgs(1, 2.5, "Hello", 'A'); // Outputs: 12.5HelloA
return 0;
}
Understanding Parameter Packs
What is a Parameter Pack?
A parameter pack in C++ is a powerful feature that allows functions and classes to accept a variable number of template arguments. This capability is particularly useful when you want to write flexible and reusable code. There are two types of parameter packs: template parameter packs, which are used in templates, and function parameter packs, which can be found in variadic functions.
The Syntax of Parameter Packs
The basic syntax involves declaring a function or a template with `typename... Args`, where `Args` signifies that it can accept any number of parameters. Here’s how it looks:
template<typename... Args>
void func(Args... args) {
// Implementation
}
In this example, `func` can take any number of arguments of varying types, including none at all. This flexibility allows programmers to create functions that can handle a wide variety of input without needing to overburden the codebase with numerous overloads.

Using Parameter Packs in Functions
Variadic Templates
Variadic templates are an extension of standard templates that permit them to accept any number of template arguments. This is often used in scenarios where you need functions to perform operations on several data types.
A common use case is implementing a function that prints different types of arguments. Here’s an example:
template<typename... Args>
void print(Args... args) {
(std::cout << ... << args) << '\n'; // Fold expression
}
In this example, the `print` function utilizes a fold expression to output all the provided arguments in a single line. This approach simplifies the implementation considerably while providing a versatile function.
Forwarding Parameter Packs
Perfect forwarding aims to retain the value category (i.e., lvalue or rvalue) of function arguments when passing them to another function. This is achieved using `std::forward` in conjunction with parameter packs.
Here’s an example to illustrate this:
template<typename... Args>
void forwardFunc(Args&&... args) {
someOtherFunction(std::forward<Args>(args)...);
}
In this code snippet, `forwardFunc` can take any arguments and perfectly forward them to `someOtherFunction`, ensuring their value type remains intact.
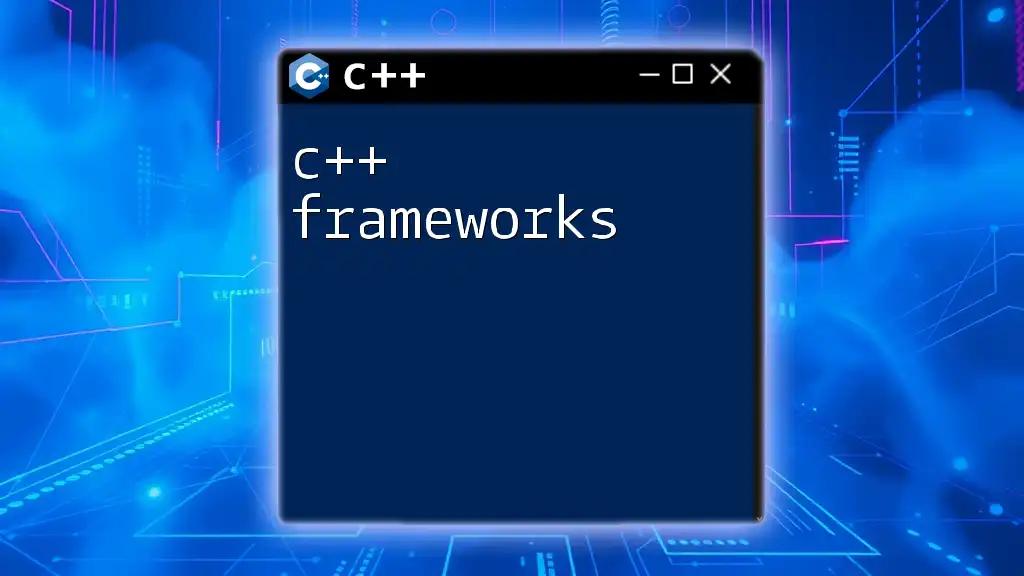
Advanced Techniques with Parameter Packs
Expanding Parameter Packs
Expanding a parameter pack is a crucial technique that allows you to operate on all arguments in a parameter pack uniformly. This is often achieved through fold expressions, a feature introduced in C++17, which makes operations on parameter packs simple and elegant.
Consider the following example that calculates the sum of all arguments passed:
template<typename... Args>
auto sum(Args... args) {
return (args + ...); // Fold expression for addition
}
In the `sum` function, the fold expression `(args + ...)` computes the sum of all arguments efficiently, showcasing the power and simplicity of this feature.
Combining Parameter Packs
You can also combine multiple parameter packs seamlessly, allowing functions to merge different sets of parameters into one cohesive operation.
Here’s how combining can be implemented:
template<typename... Args1, typename... Args2>
void combine(Args1... args1, Args2... args2) {
print(args1..., args2...); // Using print function defined earlier
}
In this example, the `combine` function takes two parameter packs and combines their arguments, printing them all in a single call.

Practical Applications of Parameter Packs
Creating Flexible APIs
One of the most significant advantages of parameter packs is the ability to create flexible APIs that can handle varying degrees of inputs without strict type restrictions.
For instance, a generalized logging function can be designed as follows:
template<typename... Args>
void log(Args... args) {
std::cout << "Log: ";
print(args...); // Reusing print function
}
In this scenario, the `log` function can accept an array of different argument types, providing versatility and convenience for developers.
Class Templates with Parameter Packs
Parameter packs are not limited to functions; they can also be utilized in class templates to create data structures that can hold diverse object types. Here’s an example:
template<typename... Types>
class MultiTypeContainer {
std::tuple<Types...> data;
};
In this `MultiTypeContainer` class, a `std::tuple` is used to store an arbitrary number of types, allowing for a flexible and dynamic collection. This design is particularly useful in situations requiring handling of heterogeneous collections.

Common Pitfalls and How to Avoid Them
Overusing Parameter Packs
While parameter packs are incredibly powerful, overusing them can lead to code that is difficult to read and maintain. It’s essential to strike a balance and use parameter packs in scenarios where their benefits genuinely add value.
Template Instantiation Errors
Understanding templates and their instantiation errors can often be a challenge. When working with parameter packs, it’s crucial to carefully read error messages, as they often indicate issues with the number or types of arguments being passed. Taking the time to troubleshoot these problems can save you significant time in the long run.

Conclusion
C++ parameter packs are a feature that enhances the flexibility of template programming, enabling developers to write functions and classes that can handle a variable number of parameters seamlessly. From variadic templates to class templates, mastering parameter packs opens up a plethora of opportunities for creating scalable and maintainable code.
By practicing with the provided examples and exploring different use cases, you can manifest a deeper understanding of this powerful tool in your C++ arsenal. If you have any questions or wish to share your experiences related to parameter packs, don’t hesitate to reach out and engage with the community!

Further Reading and Resources
To deepen your understanding of C++ parameter packs and C++ templates in general, consider exploring the official C++ documentation, recommended books on Advanced C++ Programming, and online courses focused on C++ template programming.