In C++, the `next_permutation` algorithm from the Standard Library generates the next lexicographical permutation of a sequence, effectively rearranging the elements in a specific order.
Here’s a code snippet demonstrating how to use it:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3};
std::sort(v.begin(), v.end()); // Ensure the sequence is sorted
do {
for (int i : v) std::cout << i << " ";
std::cout << std::endl;
} while (std::next_permutation(v.begin(), v.end()));
return 0;
}
What is a permutation?
A permutation refers to the arrangement of a set of objects in a specific order. In mathematics, it involves calculating the various ways in which a group of items can be arranged. Understanding permutations is crucial in fields such as combinatorial mathematics, probability theory, and algorithm design, where different arrangements lead to different outcomes.
Why use C++ for permutations?
C++ is particularly suited for tasks involving permutations due to its speed and efficiency. Its powerful features, including low-level memory manipulation, optimization capabilities, and the Standard Template Library (STL), make it a robust choice for algorithm implementation. When it comes to working with permutations, C++ allows developers to swiftly generate, manipulate, and analyze permutations without significant overhead.
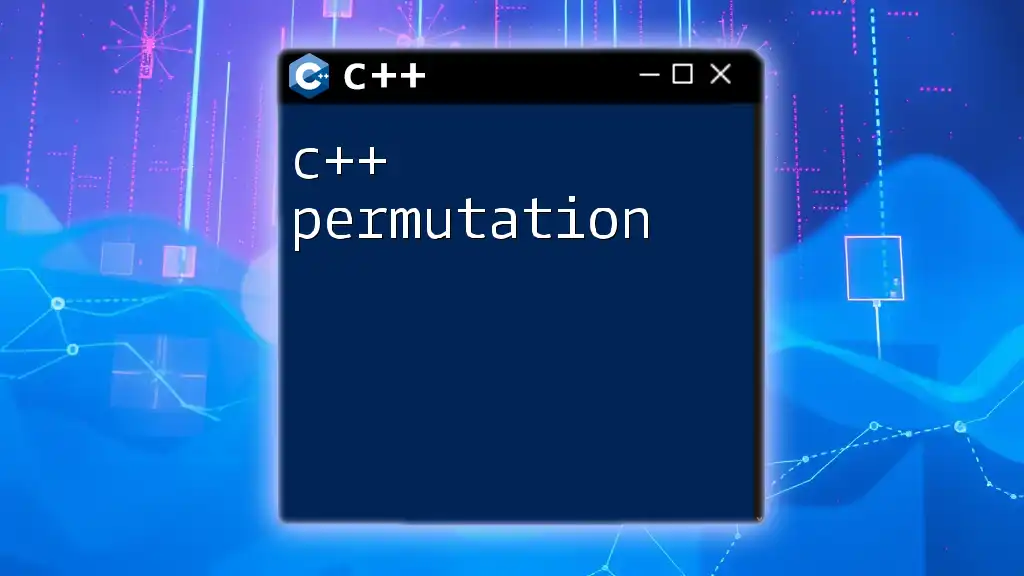
Understanding Permutation
Mathematical Concept of Permutation
In mathematical terms, a permutation of a set is any possible arrangement of its members. For example, the permutations of the set {1, 2, 3} include (1,2,3), (1,3,2), (2,1,3), and so on. The formula for calculating the number of permutations of n distinct objects is given by n! (n factorial), which is the product of all positive integers up to n.
Types of Permutations
- Simple permutations: Arrangements of distinct items without restrictions.
- Permutations with restrictions: Situations where certain items must or must not be placed in specific positions.
- Circular permutations: Arrangements where the order matters but the starting point is arbitrary, such as seating people around a table.
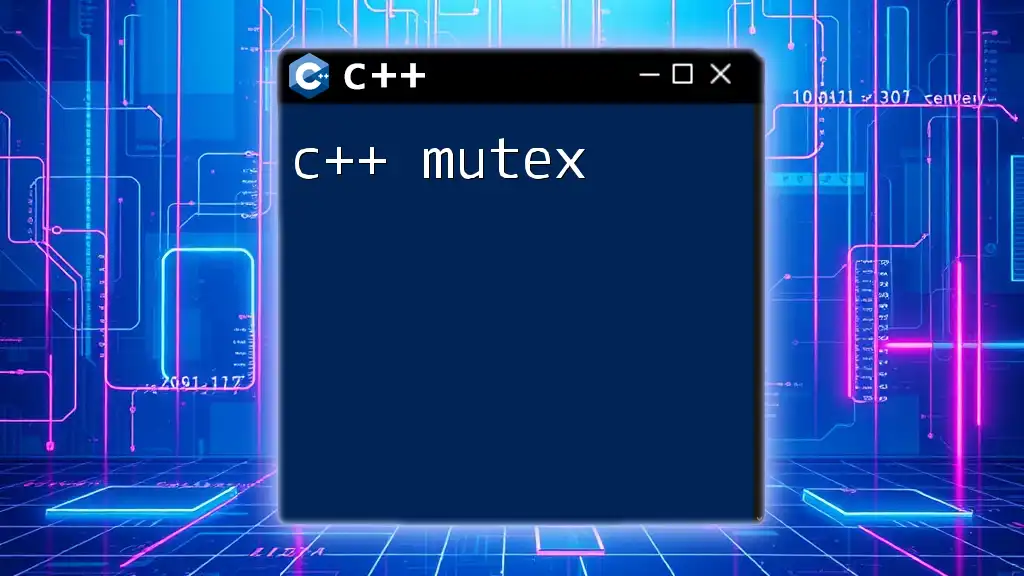
Using C++ to Generate Permutations
Generating Permutations Using STL
The C++ Standard Template Library (STL) provides a built-in function called `std::next_permutation`, which simplifies the process of generating permutations. This function rearranges the elements in a container (e.g., vector, array) into the next lexicographically greater permutation.
To use `std::next_permutation`, you should include the header:
#include <algorithm>
Code Snippet: Basic Usage of `std::next_permutation`
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main() {
vector<int> nums = {1, 2, 3};
// Generate permutations
sort(nums.begin(), nums.end()); // Ensures the initial sequence is in increasing order
do {
for (int num : nums) {
cout << num << " ";
}
cout << endl;
} while (next_permutation(nums.begin(), nums.end()));
return 0;
}
In this example, we first sort the initial vector to ensure the sequence starts in its lexicographically smallest order. The `do-while` loop continues to generate the next permutation until all permutations have been exhausted. Each permutation is printed to the console, showcasing C++'s ability to efficiently handle permutations with minimal code.
Breaking Down the Code
- Sorting: Calling `sort(nums.begin(), nums.end())` ensures that we begin with the smallest permutation, which is crucial for the `std::next_permutation` function to generate all subsequent permutations in increasing order.
- Looping through permutations: The `do` loop prints the current configuration of numbers, and the `while` condition checks for the next permutation until it surpasses the last possible arrangement.
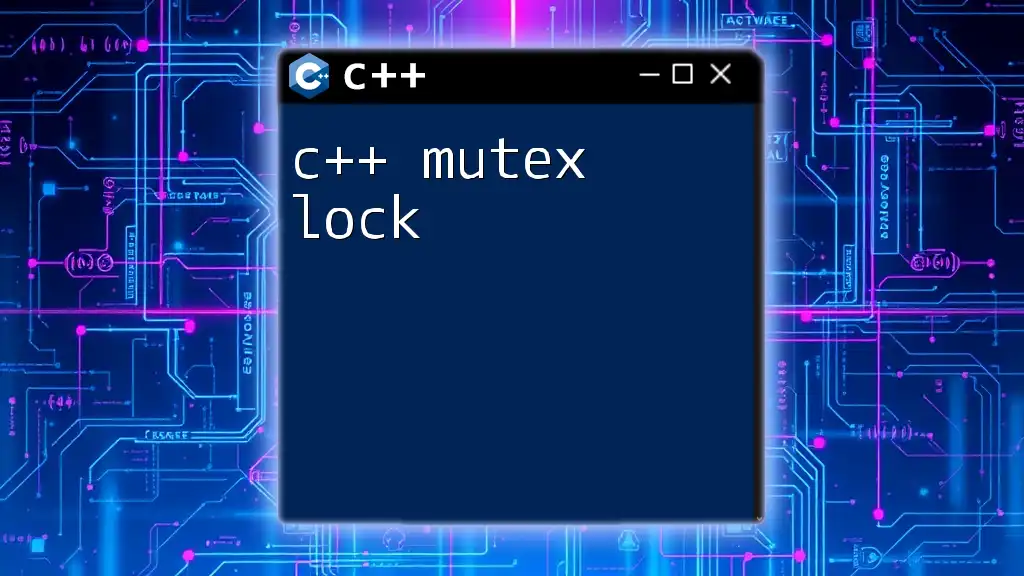
Custom Implementations of Permutation
Recursive Approach to Generate Permutations
The recursive approach to generating permutations is another effective method leveraging the concept of backtracking. By swapping elements and recursively generating all possible arrangements, developers can explore all permutations systematically.
Code Snippet: Recursive Function to Generate Permutations
#include <iostream>
#include <vector>
using namespace std;
void swap(char &a, char &b) {
char temp = a;
a = b;
b = temp;
}
void permute(vector<char> &array, int start, int end) {
if (start == end) {
for (char c : array) {
cout << c;
}
cout << endl;
} else {
for (int i = start; i <= end; i++) {
swap(array[start], array[i]);
permute(array, start + 1, end);
swap(array[start], array[i]); // backtrack
}
}
}
int main() {
vector<char> chars = {'A', 'B', 'C'};
permute(chars, 0, chars.size() - 1);
return 0;
}
This code snippet defines a `permute` function that recursively generates all permutations of a given character array.
Explaining the Recursive Logic
- Base Case: When the `start` index equals the `end` index, it means a complete permutation has been generated, which can then be printed.
- Recursive Case: The function iterates through the elements from the `start` index to the `end` index, swapping each character into the current position and recursively calling itself to generate all arrangements for the rest of the characters.
Backtracking is essential in this algorithm, as it undoes swaps to explore other permutation paths, ensuring all unique orders are generated.
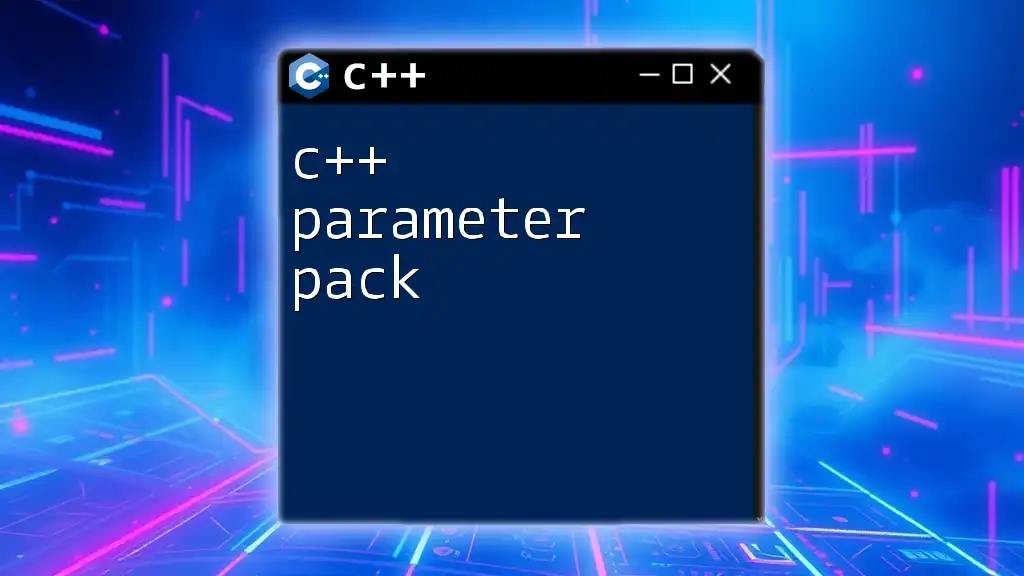
Performance Considerations
Complexity Analysis of Permutations
The time complexity for generating all permutations of a set of `n` elements is O(n!) due to the factorial growth of permutations as the size of the input increases. The space complexity depends on the method employed, typically falling into O(n) for the recursive calls due to the call stack, while the iterative approach is often more memory-efficient.
Optimizing Permutations
To enhance efficiency in generating permutations, consider:
- Heap Memory and Stack Space: Utilize efficient memory management to reduce overhead.
- Handling Duplicates: Implement checks to avoid redundant calculations, particularly when the input contains repeated elements. Sorting the elements at the beginning can simplify this process.
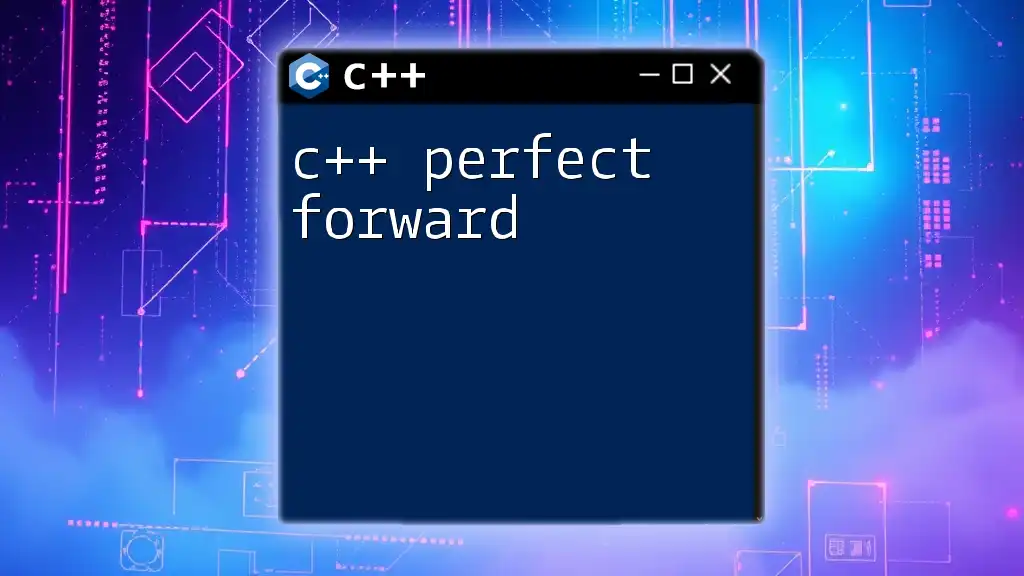
Practical Applications of Permutations
Permutations find widespread applications across various domains, including:
- Solving algorithmic puzzles: Many problems, such as the N-Queens problem, can be tackled using permutation techniques.
- Testing and validation: Generating test cases for software applications by permuting input data to ensure robustness against various combinations of inputs.
- Combinatorial analysis: Analyzing problems that involve arrangements and combinations, such as graph theory and statistical modeling.
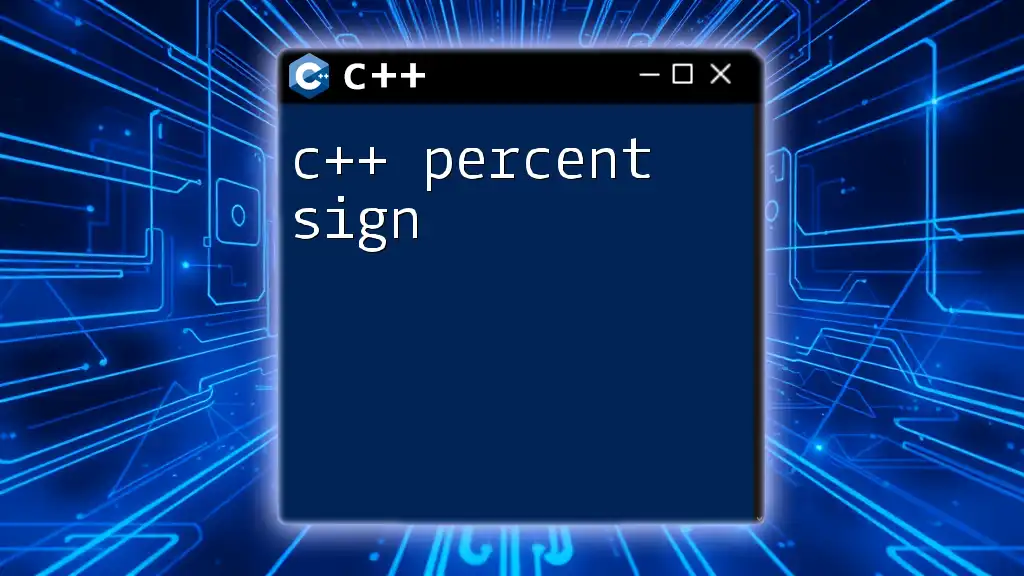
Recap of Key Points
In this guide, we delved into the essence of permutations and explored how C++ can be utilized to generate and manipulate them effectively. By understanding both STL functions and custom recursive implementations, developers can leverage C++’s strengths to handle complex arrangement problems efficiently.
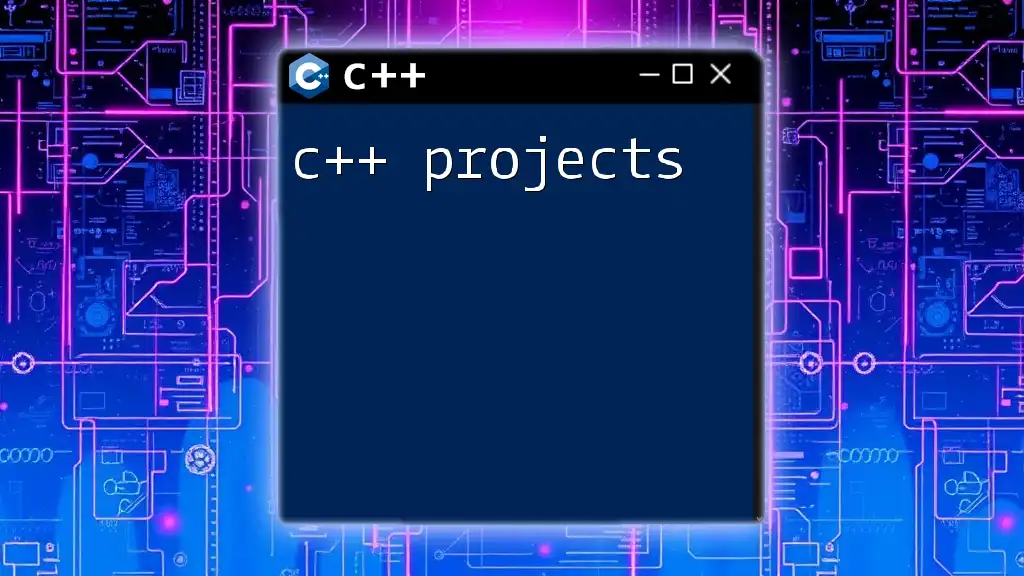
Encouragement to Practice
Mastering the concept of permutations is vital for any aspiring programmer or developer. Hands-on implementation will deepen your understanding and enhance your problem-solving skills.
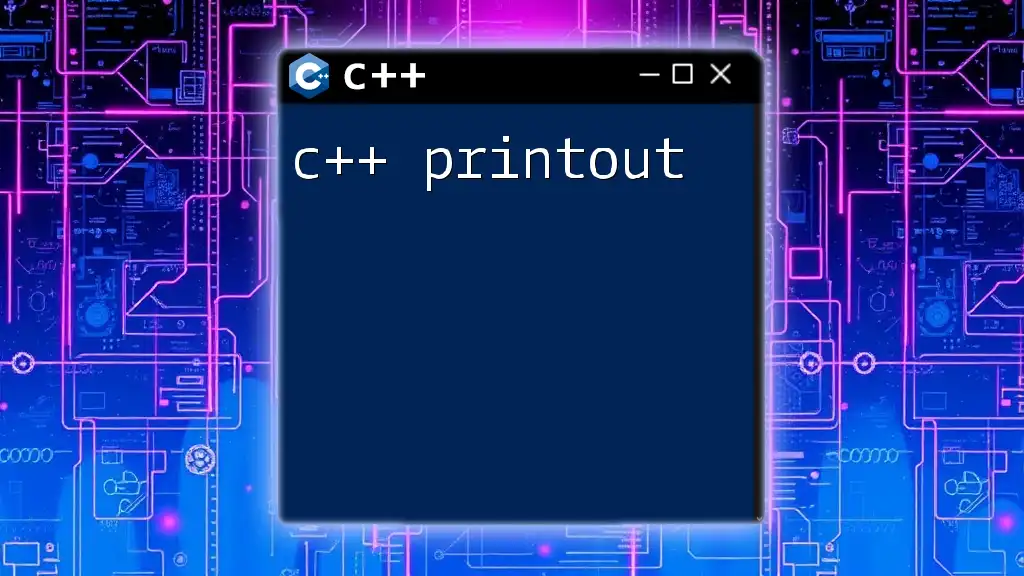
Further Reading and Resources
To further enrich your knowledge on C++ and permutations, explore these resources:
- Comprehensive C++ programming books.
- Online courses focusing on algorithms and problem-solving techniques.
- C++ documentation for STL functions and libraries.
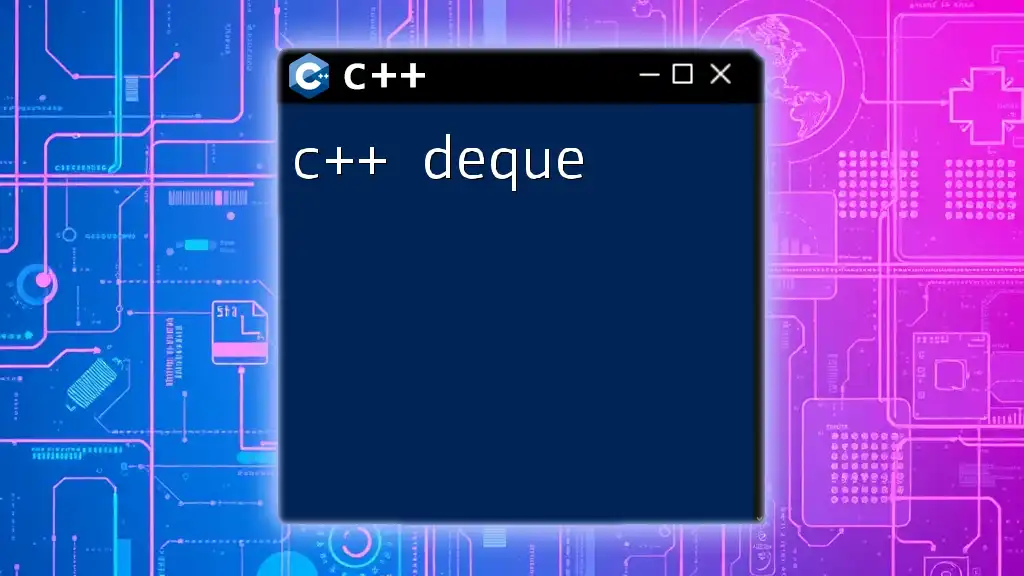
Join Our Community
As you embark on your journey through C++, consider joining our platform for expert guidance, shared resources, and community support. Together, we can explore the exciting world of programming.
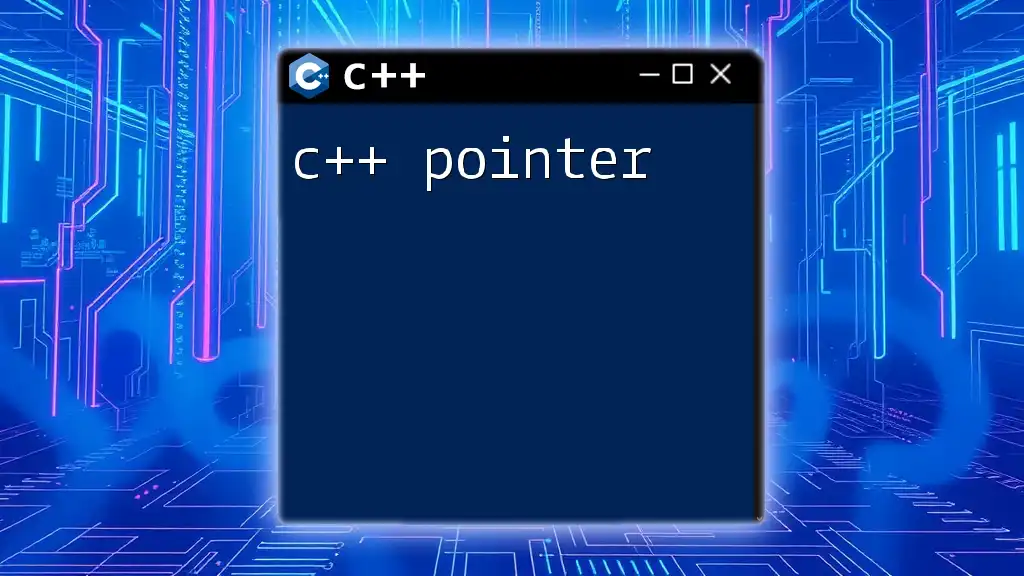
Subscribe for More Tutorials
Don't forget to subscribe for future articles that offer deeper insights into advanced C++ concepts, practical programming techniques, and invaluable resources for continuous learning in software development.