A C++ deque (double-ended queue) is a versatile container that allows for the efficient insertion and deletion of elements at both ends.
#include <iostream>
#include <deque>
int main() {
std::deque<int> d;
d.push_back(1); // Add to the back
d.push_front(2); // Add to the front
std::cout << d.front() << " " << d.back(); // Outputs: 2 1
return 0;
}
What is a Deque?
A deque (double-ended queue) is a versatile container in C++ that allows insertion and deletion of elements from both ends. Unlike a vector or a list, a deque enables fast additions and removals at both the front and back, making it a powerful choice for certain algorithms and data structures.
Characteristics of Deque
- Dynamic Size: Similar to vectors, deques can grow and shrink in size dynamically.
- Bidirectional Insertions and Deletions: Unlike vectors, which only allow additions or deletions from the end, deques support operations at both the head and tail.
- Random Access: Deques allow access to elements using an index, similar to arrays and vectors.
Comparison with Other STL Containers
While vectors are excellent for dynamic arrays and lists provide efficient insertions/deletions at any position, a deque combines both functionalities, making it particularly efficient for scenarios where you need to manipulate both ends of the collection frequently.
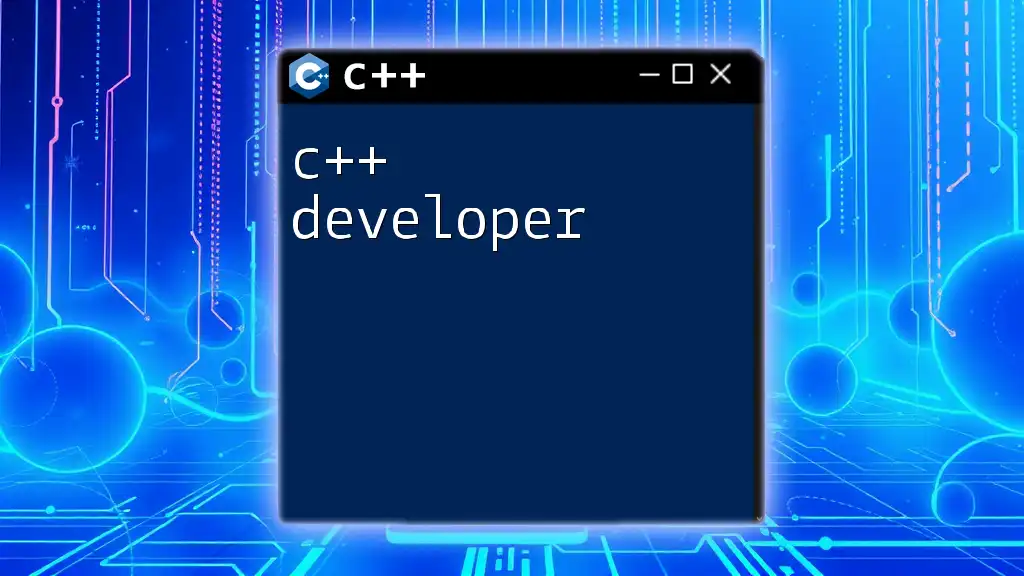
Use Cases for Deque
The deque is useful in various programming scenarios:
- When implementing algorithms that require dynamic, bidirectional access to elements, such as breadth-first search.
- In situations where you need to maintain a collection of items that can be added or removed frequently from both ends, like in task scheduling.
- In multi-threading when you require a buffer that allows both producers and consumers to operate efficiently.
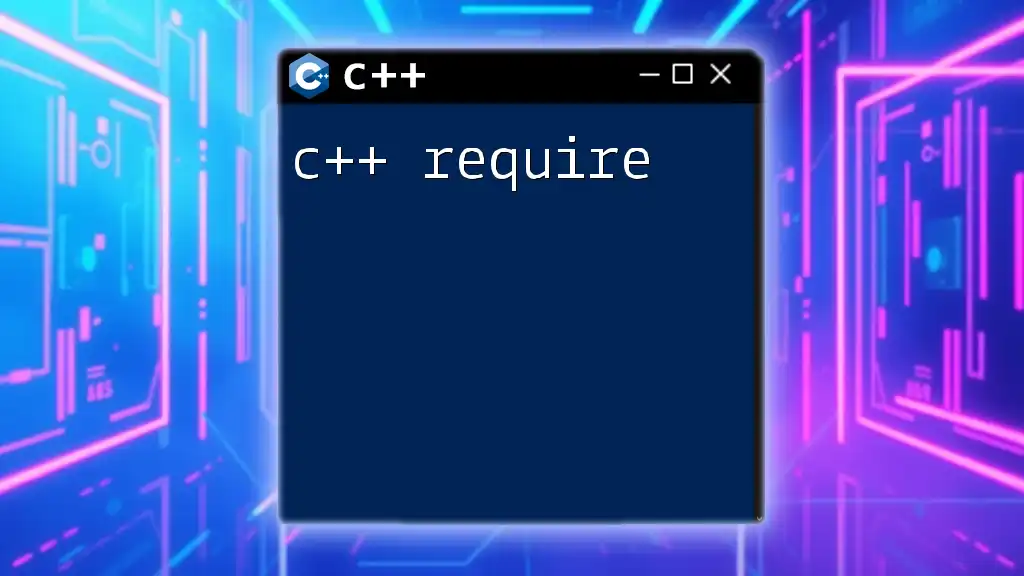
Key Properties of Deque
Dynamic Size
A deque automatically manages its capacity to hold items, ensuring that it can grow as needed. This property is particularly beneficial in applications where the number of elements fluctuates.
Supports Both Ends Insertions and Deletions
With functions like `push_front`, `push_back`, `pop_front`, and `pop_back`, you can quickly modify the deque from either end.
Random Access to Elements
You can access elements in a deque via indexing, allowing for quick retrieval and modification.
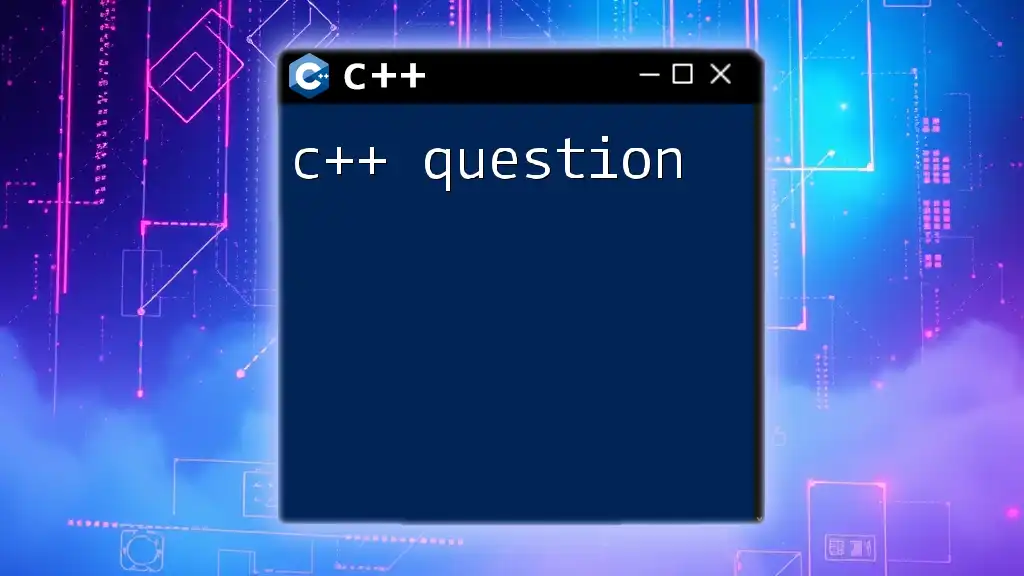
Memory Management in Deque
Deques utilize regions of memory that can be allocated and deallocated on demand. The internal structure comprises multiple nodes, which allows a deque to maintain efficient memory usage even as it grows. The complexity here lies in managing a set of contiguous memory cells rather than being entirely contiguous like a vector.
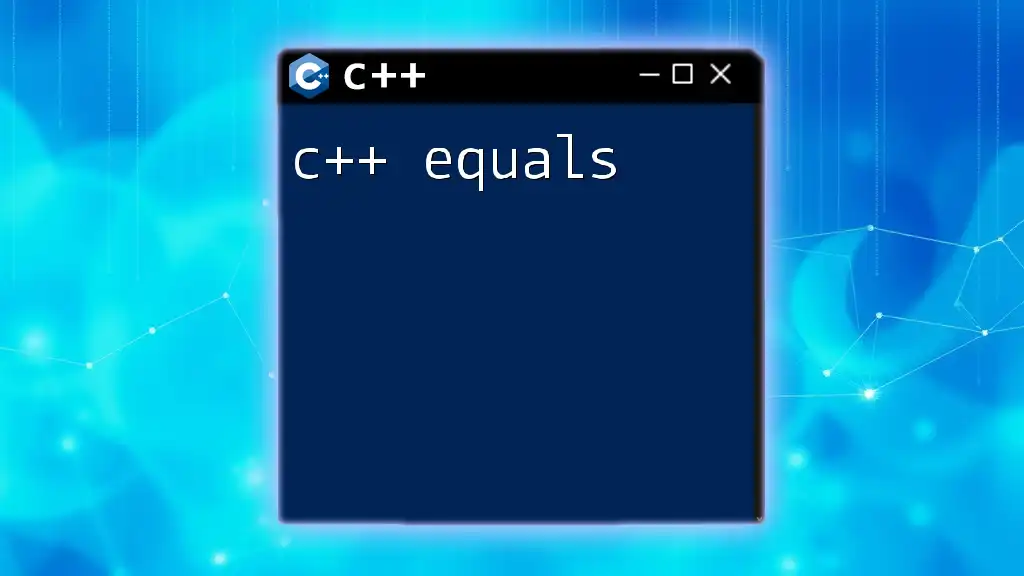
Creating a Deque
Before you can begin working with a deque, you need to include the necessary header in your project:
#include <deque>
You can then create a deque of integers like so:
std::deque<int> myDeque;
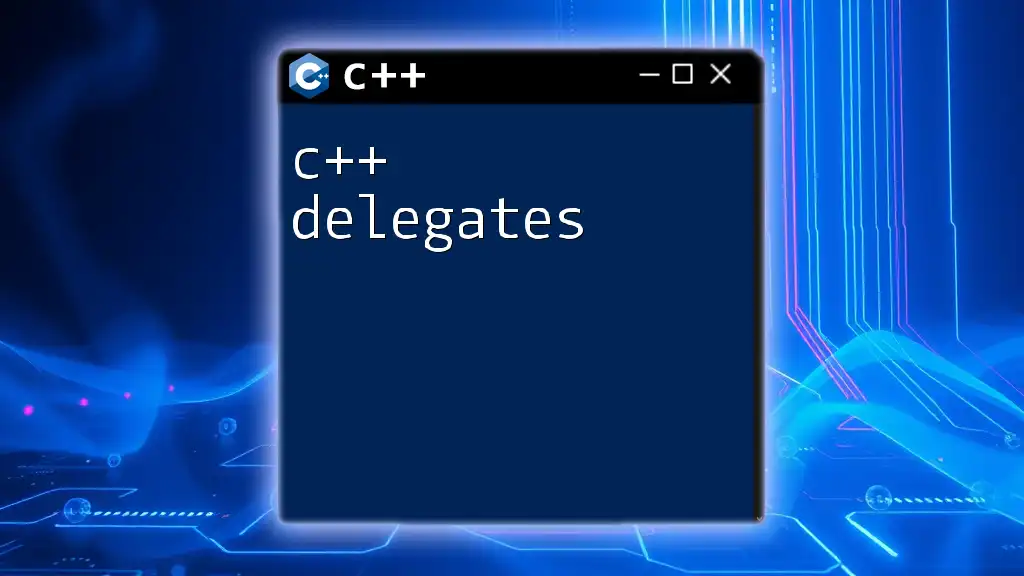
Inserting Elements
Push Back
To add an item at the end of the deque, use `push_back`. This operationing works efficiently as it is optimized for the end of the deque.
myDeque.push_back(10);
myDeque.push_back(20);
Push Front
To insert an item at the front, use `push_front`. This allows you to quickly add elements to the start, enhancing flexibility.
myDeque.push_front(5);
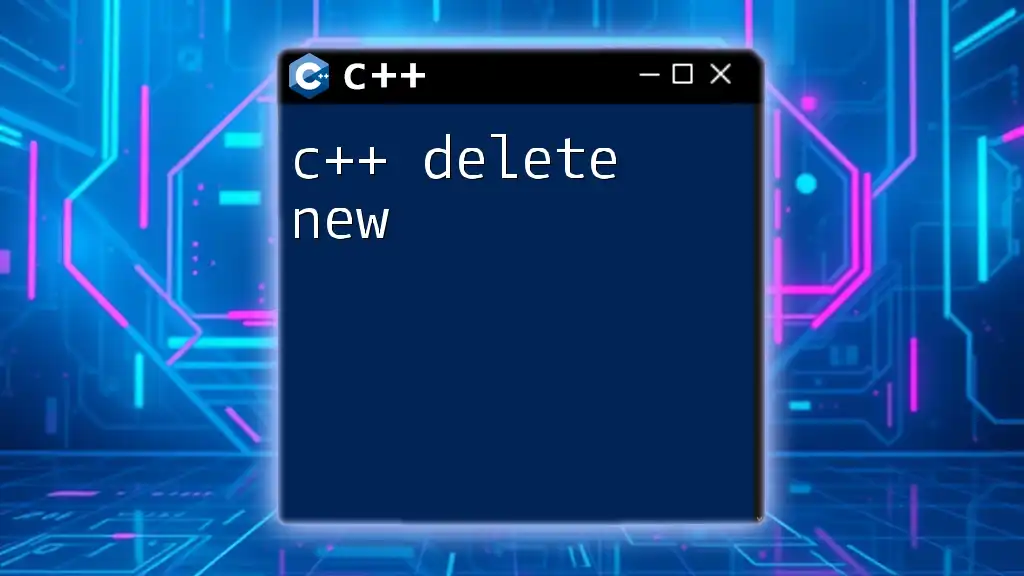
Accessing Elements
Random Access
Access elements in a deque using an index, allowing for fast retrieval.
int firstElement = myDeque[0]; // Access the first element
Front and Back
The `front` and `back` methods enable easy access to the first and last elements.
int frontElement = myDeque.front(); // Access the front item
int backElement = myDeque.back(); // Access the last item
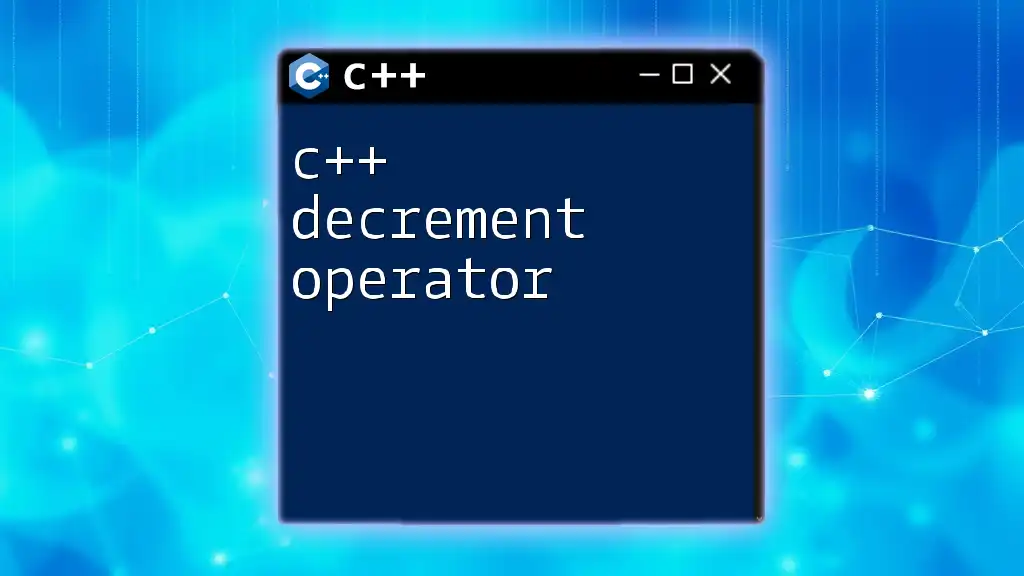
Removing Elements
Pop Back
To remove the last element, call `pop_back`. This operation is similar to `push_back` but removes rather than adds.
myDeque.pop_back();
Pop Front
Use `pop_front` to remove the first element efficiently.
myDeque.pop_front();
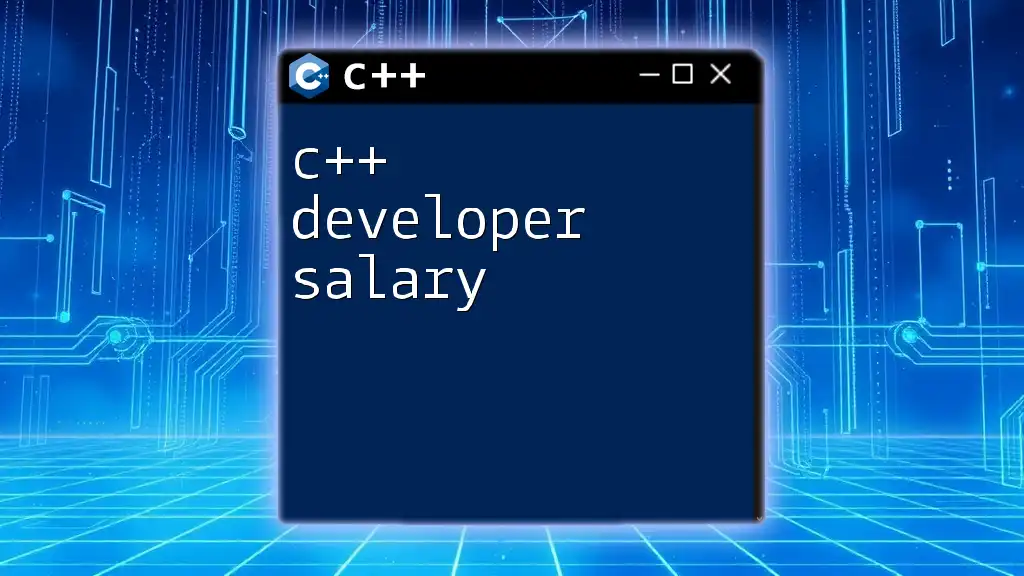
Iterators in Deque
C++ deques support iterators, making it easy to traverse the container. You can access elements using iterators in a loop:
for (auto it = myDeque.begin(); it != myDeque.end(); ++it) {
std::cout << *it << " ";
}
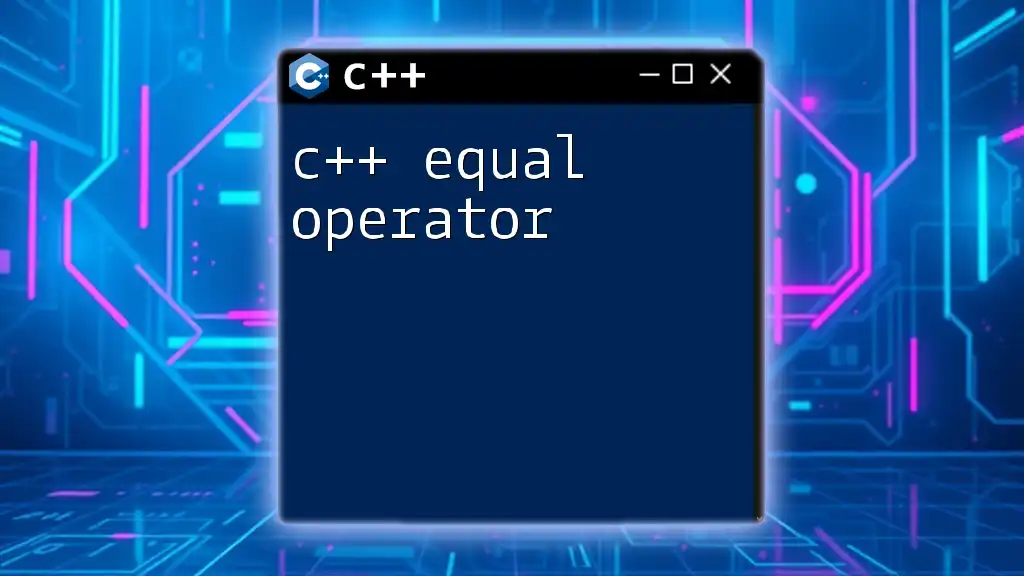
Resizing a Deque
You may need to resize a deque at times. The `resize` function changes the number of elements in a deque:
myDeque.resize(5); // Resize the deque to contain 5 elements
You can also specify new values for the added elements, if necessary.
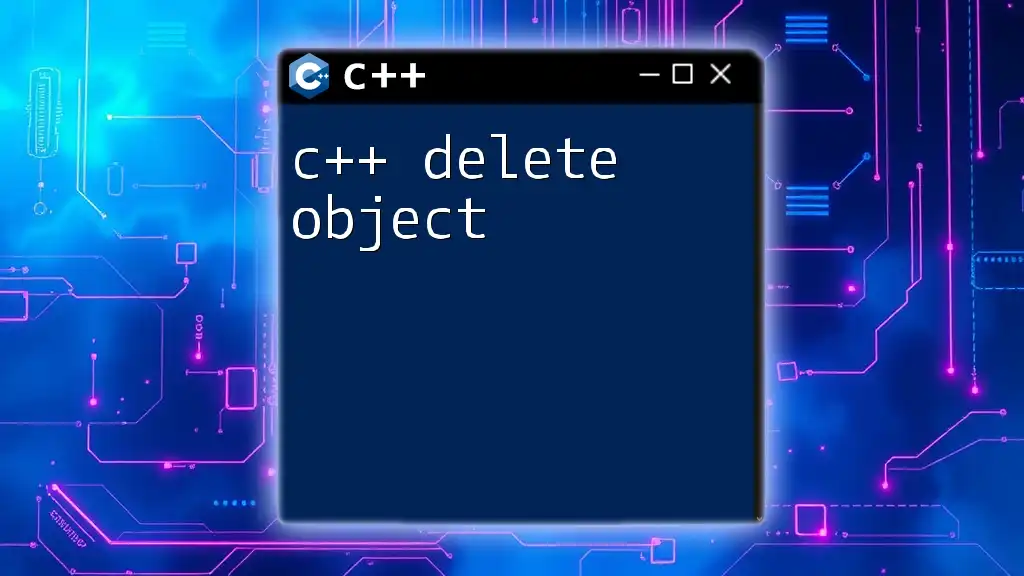
Checking Size and Capacity
To determine how many items your deque currently holds, use the `size` method:
std::cout << "Size: " << myDeque.size() << std::endl;
The method `max_size` provides the maximum number of elements the deque can hold.
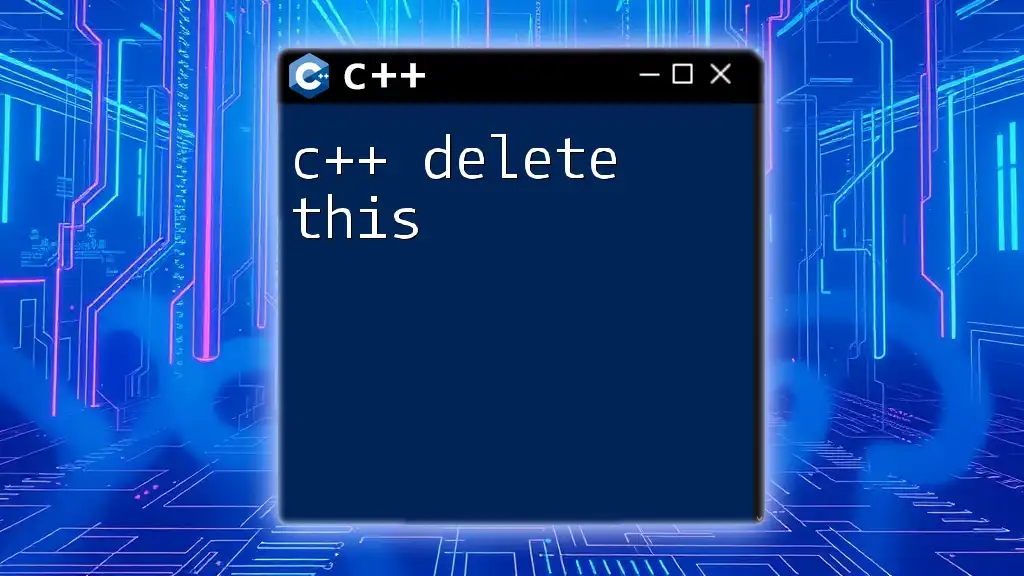
Performance Considerations
Time Complexity of Deque Operations
Understanding the time complexities of various operations helps you optimize your use of deques. Most fundamental operations like `push_front`, `push_back`, `pop_front`, and `pop_back` operate in O(1) time, while accessing an element via indexing is typically O(^n).
Best Practices for Using Deque
When determining whether to use a deque, consider:
- It is more efficient than vectors for frequent insertions and deletions at both ends.
- Avoid using deques for strictly indexed access if performance is a primary concern.
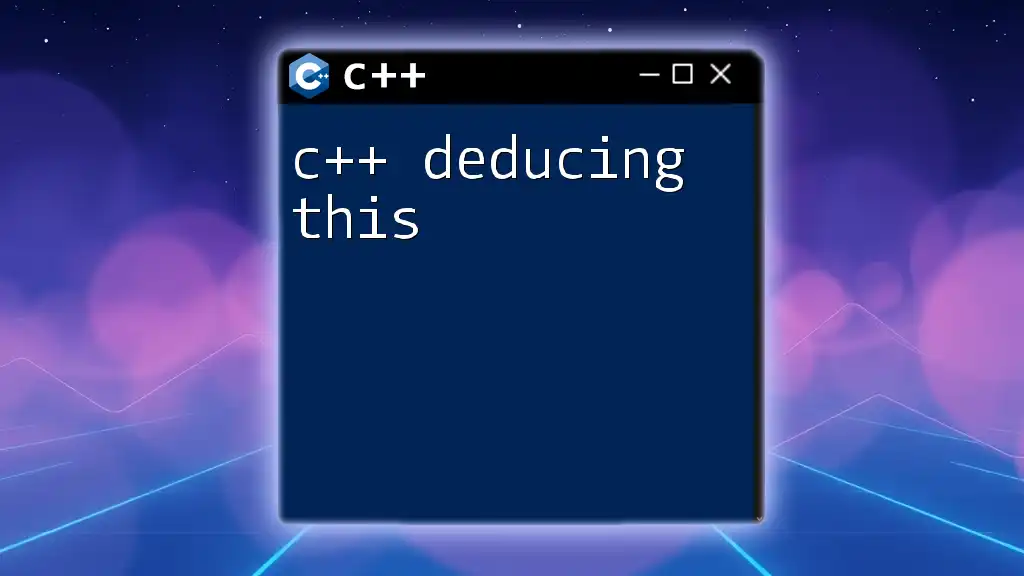
Recap of Key Points
In summary, the C++ deque is a dynamic and flexible container with powerful features that allow for fast insertions and deletions from both ends. By mastering deques, you can significantly enhance the efficiency of your C++ applications.
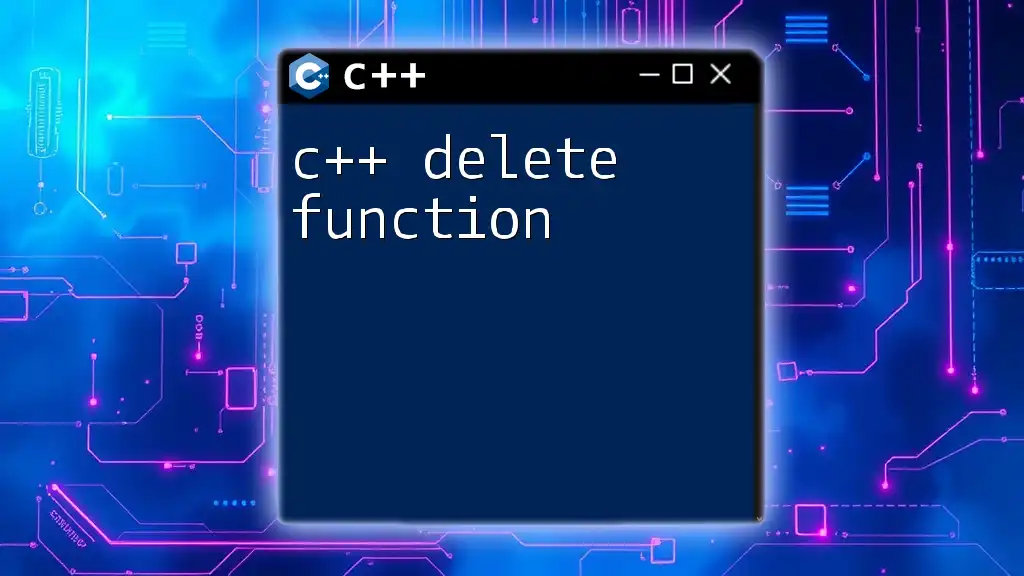
Additional Resources
Consider diving deeper into C++ through various online courses and books, which often include practical examples and exercises involving containers like deques. Checking the official C++ documentation can also provide insights into advanced deque functionalities.
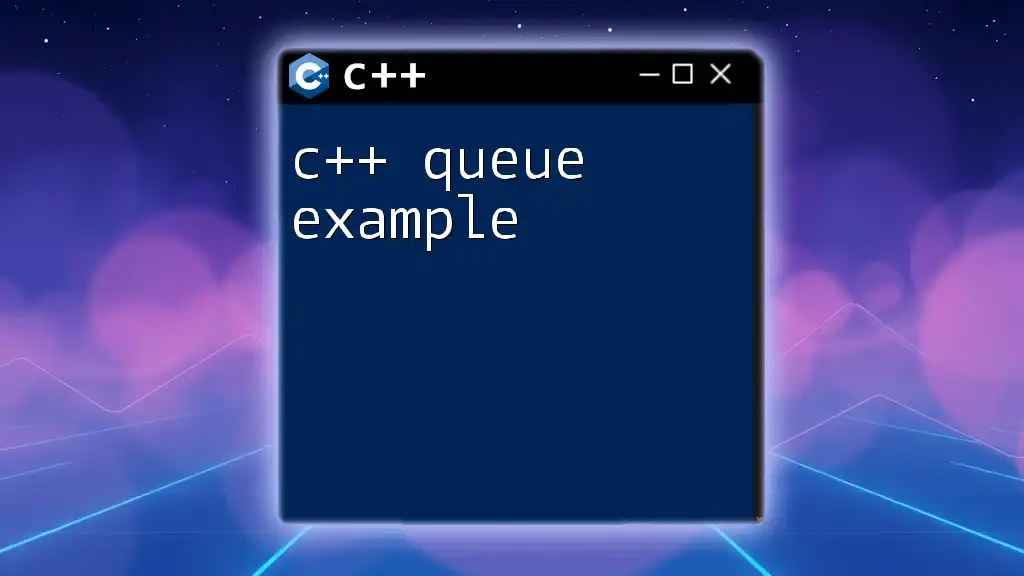
FAQs about C++ Deque
What is the difference between a deque and a vector? A deque allows for insertion and removal at both ends efficiently, while a vector only allows for modifications at one end.
Can a deque store different data types? No, a deque must contain elements of the same data type, similar to most other STL containers.
How does memory allocation work in a deque? Unlike vectors, which allocate a contiguous block of memory, deques manage multiple contiguous memory blocks, allowing for efficient memory use even as the size changes.
By understanding these core aspects of the C++ deque, you are better equipped to implement and leverage this powerful data structure in your programs.