A C++ queue is a First-In-First-Out (FIFO) data structure that allows for efficient insertion and removal of elements, as demonstrated in the following example:
#include <iostream>
#include <queue>
int main() {
std::queue<int> q;
q.push(10); // Add 10 to the queue
q.push(20); // Add 20 to the queue
q.push(30); // Add 30 to the queue
std::cout << "Front element: " << q.front() << std::endl; // Access front element
q.pop(); // Remove front element
std::cout << "New front element after pop: " << q.front() << std::endl; // Access new front element
return 0;
}
Understanding Queues in C++
A queue is a fundamental data structure that operates on the principle of FIFO (First In, First Out). This characteristic means that the first element added to the queue will be the first one to be removed, similar to a line of customers waiting for service. Queues are prevalent in various real-world scenarios, such as scheduling tasks, managing requests in web servers, and handling asynchronous data.
When compared to other data structures like stacks (LIFO - Last In, First Out), queues provide a distinct methodology for data management. Stacks are useful when you need to access the most recent element first, while queues excel in situations where the order of processing needs to be preserved.
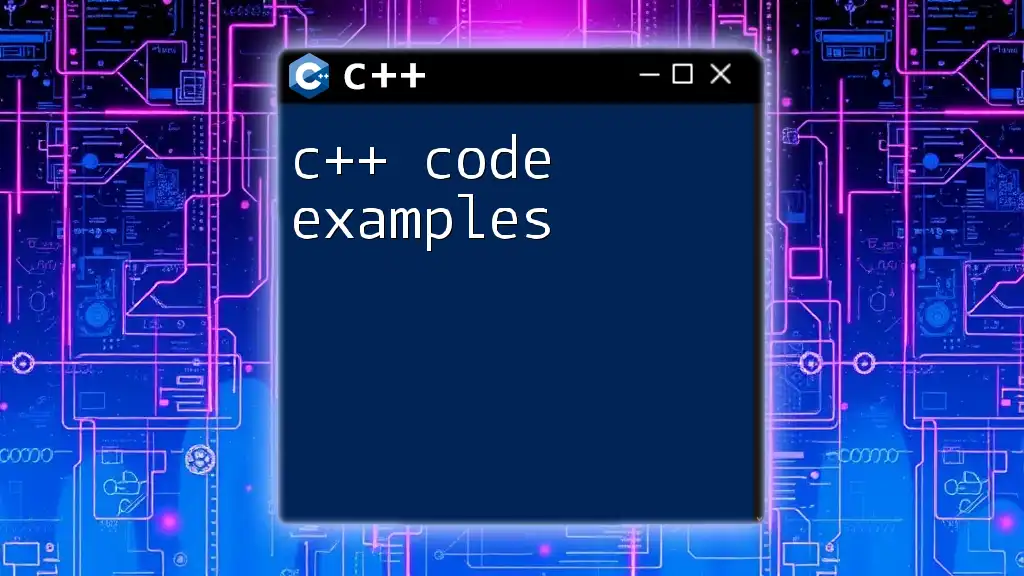
The C++ Standard Library's Queue
C++ offers a powerful set of tools through its Standard Template Library (STL), which includes the `queue` container. This allows developers to easily implement queue data structures without having to manage the underlying complexity themselves.
The STL `queue` is a template that can hold multiple types, making it versatile and easy to use in various programming scenarios. It provides a neat and efficient way to manage a queue, abstracting many of the complexities involved in queue operations.
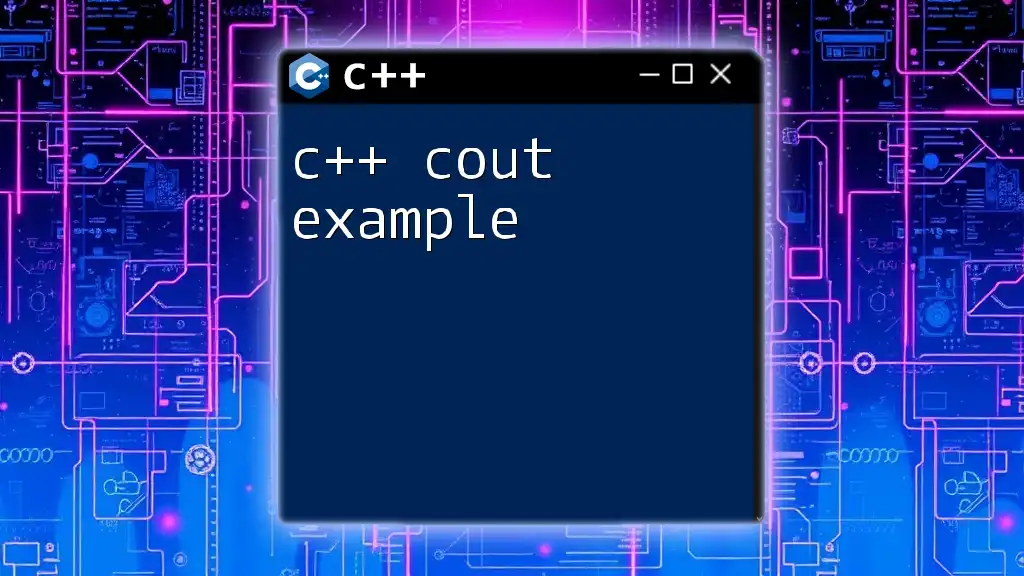
Setting Up the Environment
Before diving into the practical usage of queues in C++, ensure that you have a suitable development environment configured. You'll need to include the queue library in your program to leverage the functionality it offers.
Simply add the following lines to the top of your code:
#include <iostream>
#include <queue>
With this setup, you are ready to begin utilizing queues!
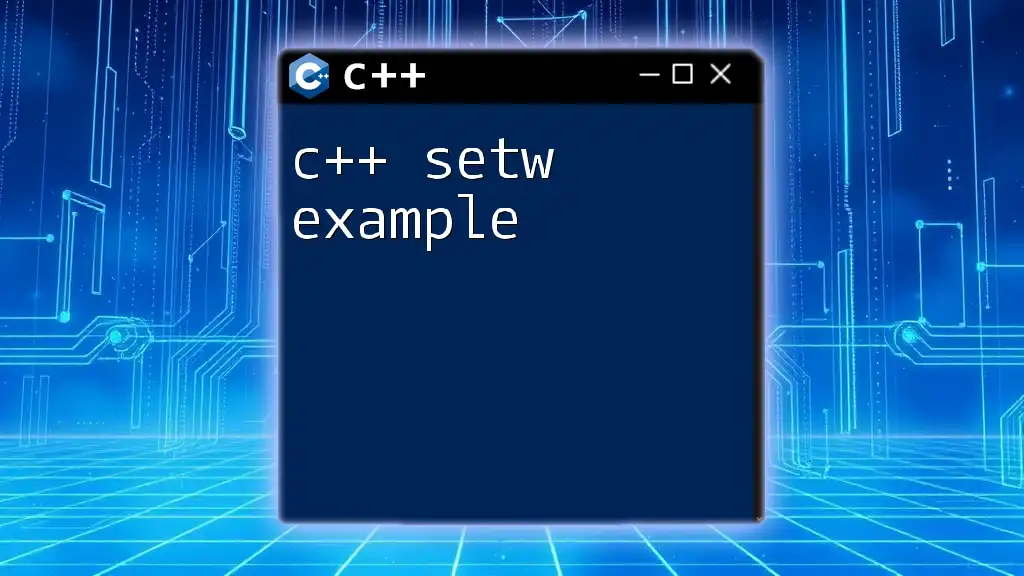
Creating a Queue in C++
To create a queue in C++, you can use the following syntax to declare it:
std::queue<int> myQueue;
In this example, we have created a queue that will hold integer values. You can replace `int` with any other data type depending on your application's needs.
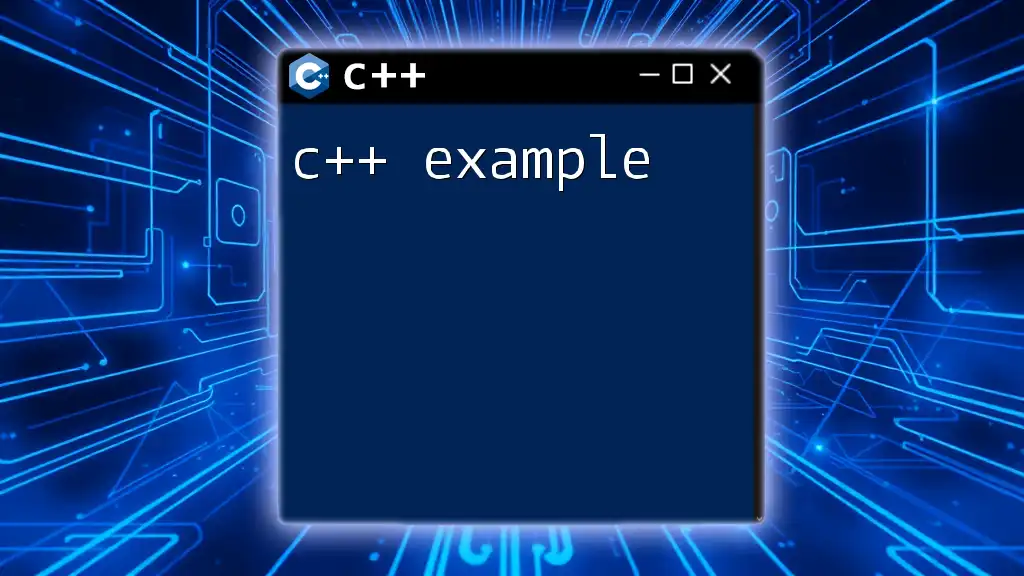
Basic Operations on Queues
Enqueue Operation
The enqueue operation consists of adding items to the back of the queue. In C++, this is performed using the `push()` method. For instance:
myQueue.push(10);
myQueue.push(20);
myQueue.push(30);
After the executions of these lines, the state of the queue will be as follows:
- Front: 10
- Middle: 20
- Back: 30
Thus, 10 will be dequeued first when we proceed to remove elements.
Dequeue Operation
Conversely, the dequeue operation allows for the removal of elements from the front of the queue. This can be done with the `pop()` method:
myQueue.pop();
By calling `pop()`, the element 10 will be removed from the queue, and the new state will be:
- Front: 20
- Back: 30
Accessing Front and Back Elements
To access the elements at the front and back of the queue without modifying it, you can utilize the `front()` and `back()` methods:
int frontElement = myQueue.front();
int backElement = myQueue.back();
Using these methods allows you to peek at the elements, giving you the capability to know what will be dequeued next without making any changes to the queue structure.
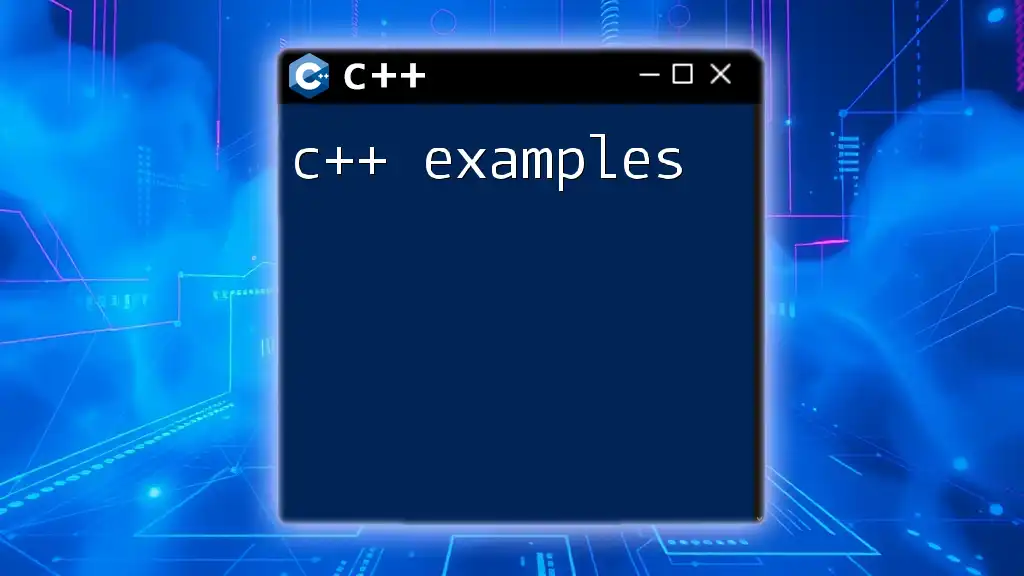
Queue Size and Emptiness Check
Checking Size
The `size()` method is useful for determining how many elements are currently in the queue:
int queueSize = myQueue.size();
This value can guide decision-making processes in your program, such as whether to enqueue more elements or manage resources accordingly.
Checking if the Queue is Empty
To check if the queue contains any elements, you can use the `empty()` method. Here's how to do it:
if(myQueue.empty()){
std::cout << "Queue is empty" << std::endl;
}
This conditional statement allows you to perform actions based on the queue's state, ensuring that you don't attempt to dequeue or access elements from an empty queue.
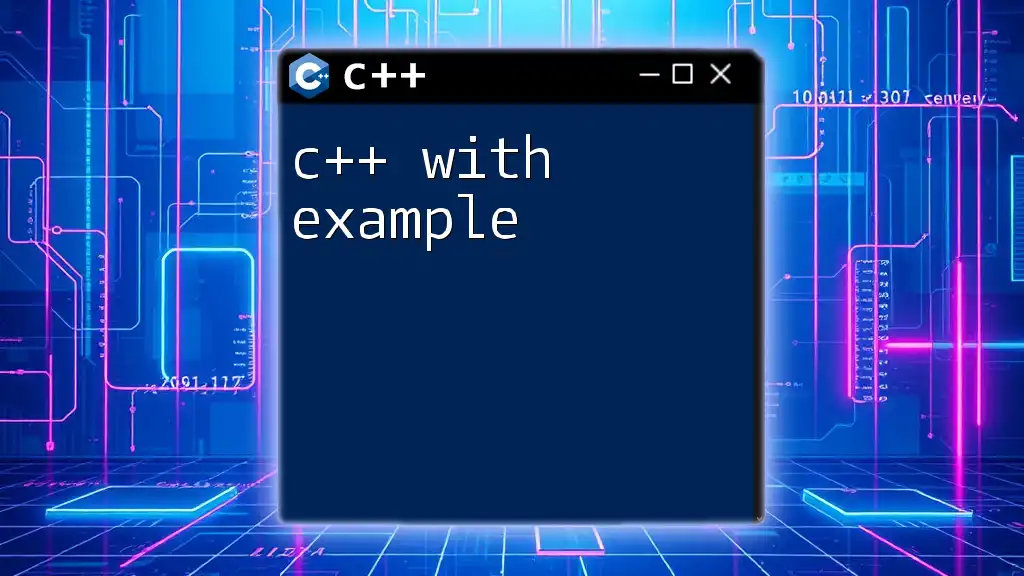
Example Application of C++ Queue
Let's put the concepts of the C++ queue example into a real-world context. We can model a simple ticketing system for customer service that employs a queue for managing customer requests effectively:
#include <iostream>
#include <queue>
int main() {
std::queue<std::string> tickets;
tickets.push("Customer 1");
tickets.push("Customer 2");
tickets.push("Customer 3");
while (!tickets.empty()) {
std::cout << tickets.front() << " is being served." << std::endl;
tickets.pop();
}
return 0;
}
In this example, three customers are added to the queue. The `while` loop processes each customer by serving them in the order they arrived, ensuring that the FIFO principle is upheld. As a result, the system provides a clear representation of how queues function in practice.
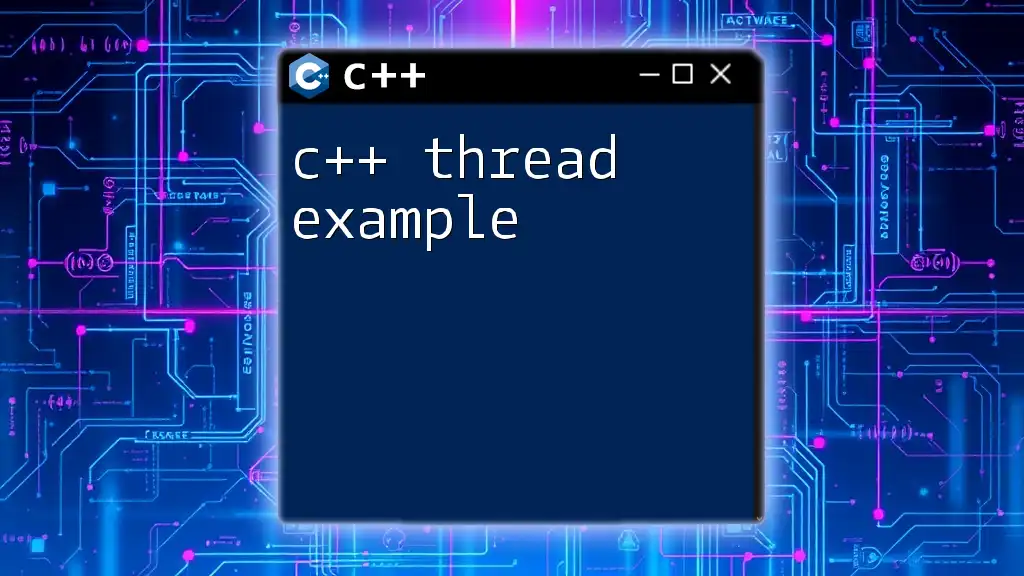
Advanced Queue Operations
Custom Queues
For those who require more complex operations, creating a custom queue class can be beneficial. This approach allows you to tailor the queue to meet specific needs or optimize performance.
Here’s a basic structure for a custom queue:
template<typename T>
class CustomQueue {
public:
void enqueue(T value);
void dequeue();
T front();
bool isEmpty();
int size();
private:
std::vector<T> elements; // Or another suitable container
};
Priority Queue
In addition to standard queues, C++ also offers the concept of priority queues, which allow for prioritizing elements based on certain criteria. In a priority queue, elements with higher priority are served before those with lower priority, regardless of their order in the queue.
To create a priority queue in C++, you can use the standard library as follows:
#include <queue>
#include <vector>
std::priority_queue<int> pq;
This declaration allows you to have a queue that organizes elements based on their value by default, serving higher values first.
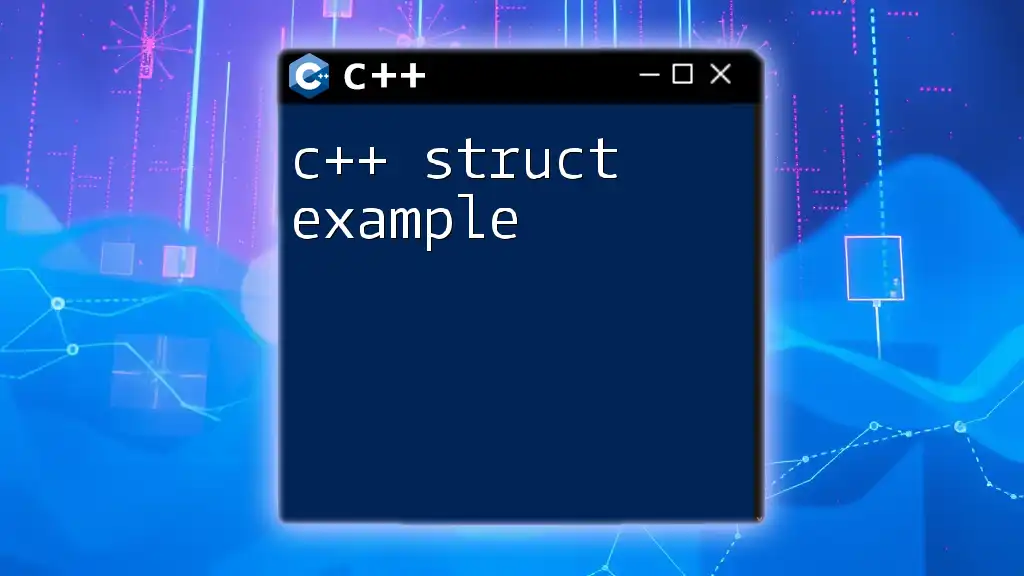
Conclusion
In this article, we explored the C++ queue example in depth, covering its essential operations and applications. By understanding how queues function and the operations available within the C++ STL, you can effectively manage data in a controlled manner that reflects real-world processes. With practice and implementation in various scenarios, you will gain a practical understanding of queues and their benefits in programming.
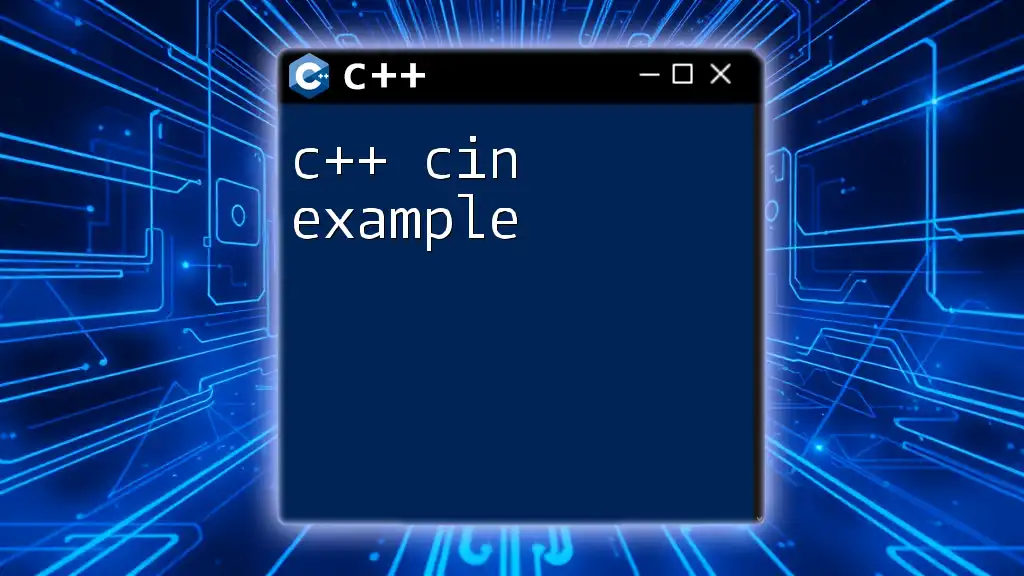
Additional Resources
To further enhance your knowledge of C++ queues, consider exploring the official C++ STL documentation or engaging with books and online courses dedicated to C++ programming. These resources will provide a deeper insight into the intricacies and capabilities of advanced data structures and algorithms, enriching your programming expertise.