The C++ `list` is a sequence container that allows for efficient insertion and removal of elements from any position within the list.
Here's a simple example of how to use a `list` in C++:
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
myList.push_back(6); // Add 6 at the end
myList.push_front(0); // Add 0 at the beginning
for (int n : myList) {
std::cout << n << ' '; // Output the elements
}
return 0;
}
What is a List in C++?
A list in C++ is a sequence container that encapsulates dynamic size arrays. Unlike arrays, lists in C++ can grow or shrink, which provides a level of flexibility that is both powerful and practical in programming. This versatility allows developers to efficiently handle collections of data that might change size during the execution of a program.
When considering data structures, it's essential to differentiate between lists and arrays. Arrays are fixed in size, meaning that once you declare them, you cannot change their length. Conversely, lists can change dynamically, allowing for easy addition and removal of elements.
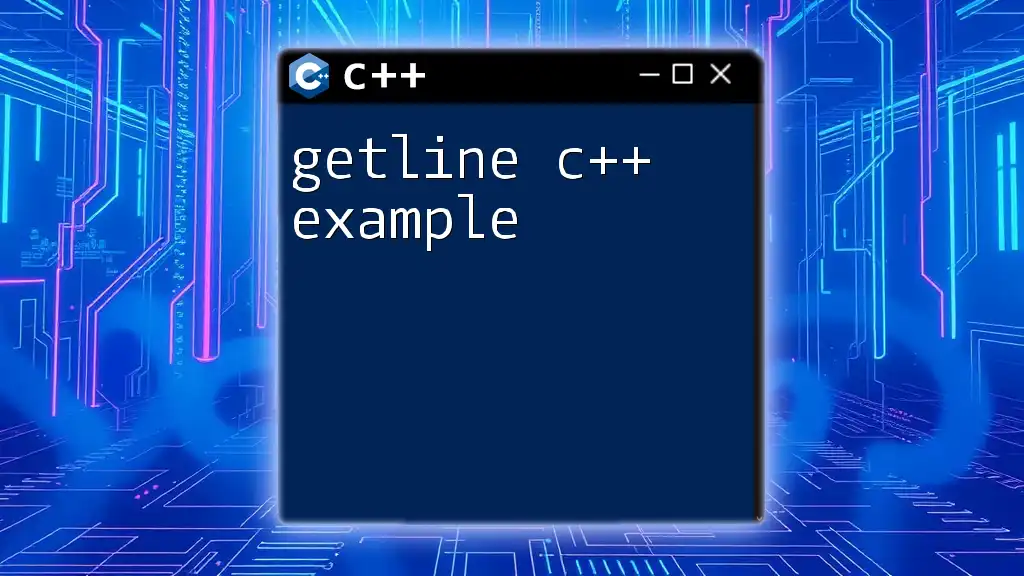
Key Features of C++ Lists
C++ lists provide several unique characteristics:
- Dynamic Size: Lists can adjust their size as elements are added or removed.
- Ease of Insertion and Deletion: Lists allow for efficient insertion and deletion of elements from both the front and the back, which is particularly beneficial when working with large datasets.
- Memory Management: Lists manage memory automatically, helping to prevent memory leaks and fragmentation.
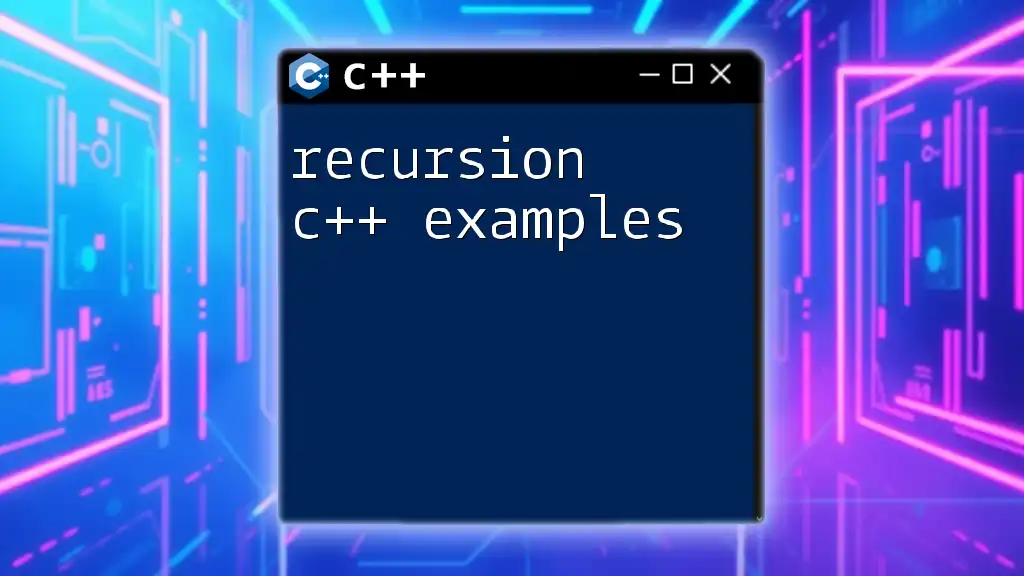
Getting Started with C++ Lists
Including the Necessary Header
To use lists in C++, you need to include the `<list>` header. This header is part of the C++ Standard Library, which contains many useful data structures and algorithms.
#include <list>
Creating a Simple List
Creating a list in C++ is straightforward. You define the type of elements the list will hold and then instantiate it. For example, the code below creates a list of integers:
std::list<int> myList; // creating a list of integers
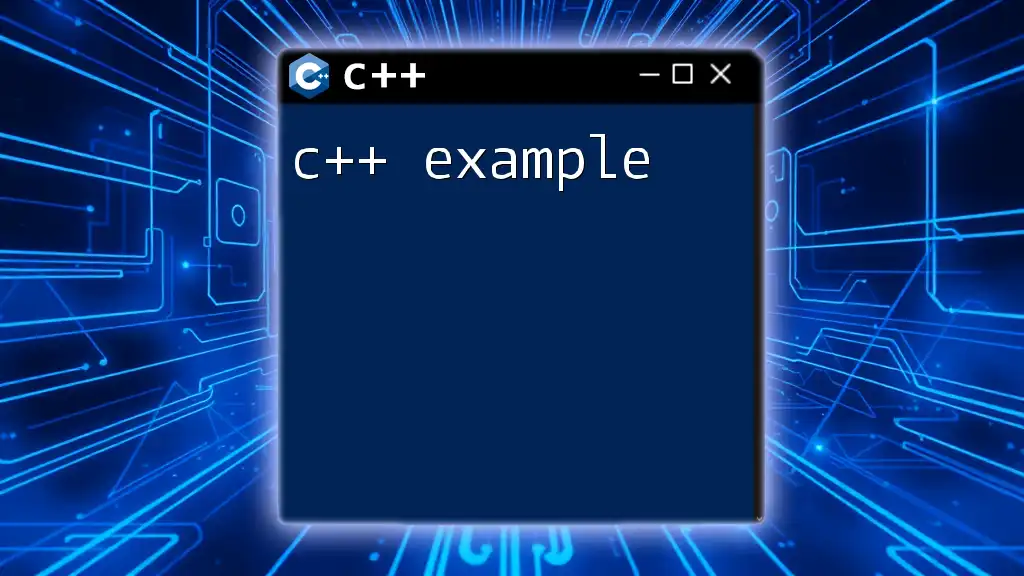
Basic Operations on C++ Lists
Adding Elements to a List
Adding elements to a C++ list can be accomplished using the `push_back` and `push_front` functions. These functions allow for inserting elements at the end or the beginning of the list, respectively.
myList.push_back(1); // Add 1 to the end of the list
myList.push_front(0); // Add 0 to the front of the list
The above operations illustrate how easily elements can be appended to a list, enhancing its utility in dynamic data handling.
Removing Elements from a List
Just as easy as adding elements, you can remove elements from a list using `pop_back` and `pop_front`. The following code demonstrates how to remove the last and the first elements:
myList.pop_back(); // Removes the last element from the list
myList.pop_front(); // Removes the first element from the list
These operations make lists an efficient choice for applications requiring frequent modifications.
Accessing Elements in a List
One of the distinctive features of lists in C++ is their use of iterators for accessing elements. Iterators provide a way to navigate through the list, as shown in the code below:
for (auto it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " "; // Output elements in the list
}
This loop iterates over the list, printing each element, underscoring the list's accessibility and ease of use.
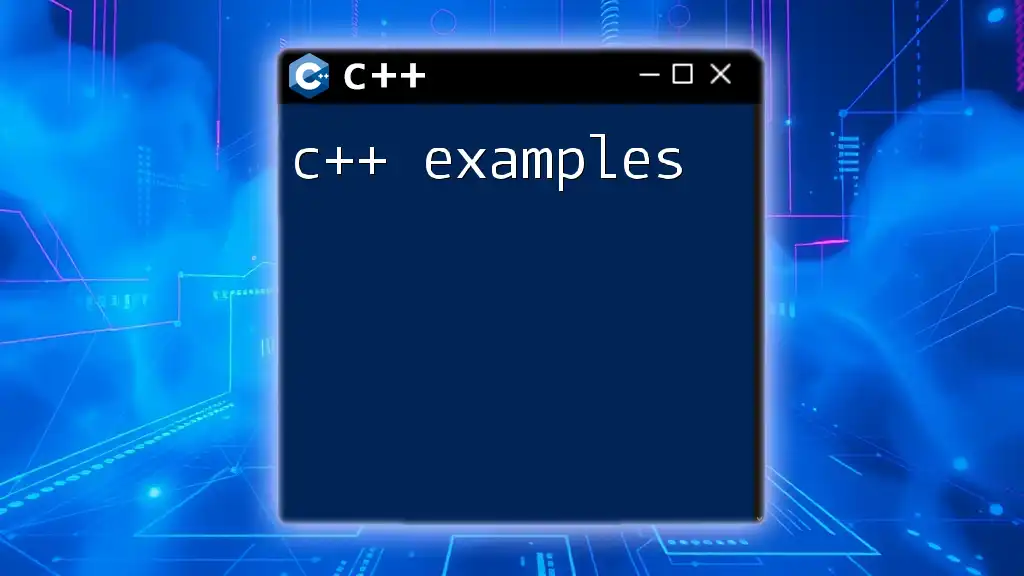
Performing Advanced Operations
Sorting a List
C++ lists come equipped with a built-in functionality to sort the elements. The `sort()` function can be invoked on the list, resulting in a sorted arrangement of the elements:
myList.sort(); // Sorts the elements of the list in ascending order
Sorting operations are common in programming, and lists make it simple to achieve ordered data effortlessly.
Merging Two Lists
Merging two lists is another advantageous feature of C++ lists. The `merge()` function can seamlessly combine two lists into one:
std::list<int> anotherList = {4, 5, 6};
myList.merge(anotherList); // Merges anotherList into myList
This functionality is particularly useful when aggregating data from multiple sources, simplifying the development process.
Reversing a List
Reversing the order of elements in a list can be achieved easily with the `reverse()` function. This operation provides a simple way to invert the order of the elements:
myList.reverse(); // Reverses the order of elements in the list
Reversing can be beneficial in numerous scenarios, whether for displaying items in reverse order or during certain algorithmic implementations.
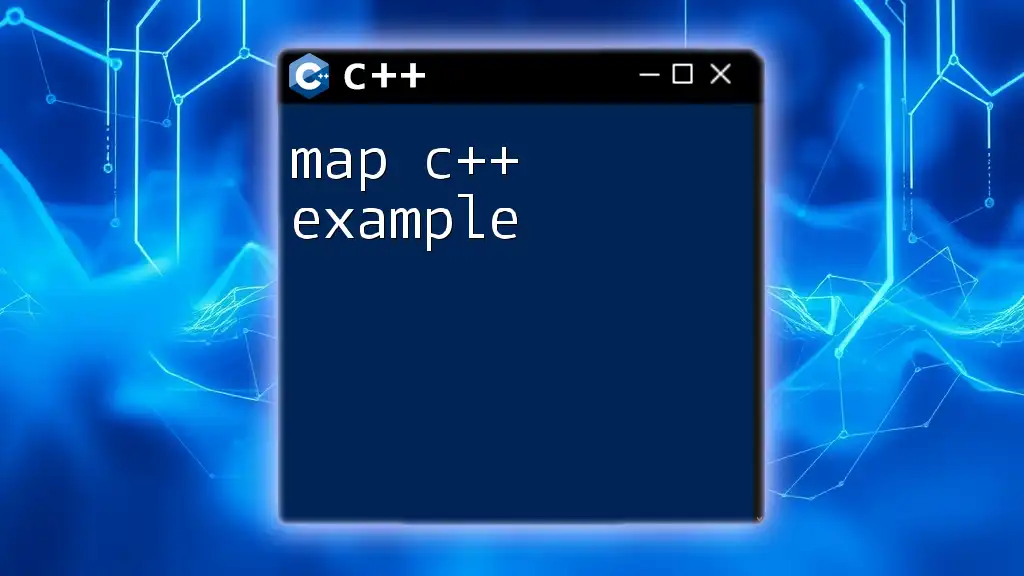
Practical Example: Using C++ Lists
Example Scenario
Let's consider a practical example where lists might be beneficial: managing a to-do list in a simple C++ program. This scenario can demonstrate the utility of lists in real-world applications.
// Program to demonstrate the use of lists to manage a to-do list
#include <iostream>
#include <list>
#include <string>
int main() {
std::list<std::string> todoList;
// Adding tasks to the to-do list
todoList.push_back("Complete C++ assignment");
todoList.push_back("Read a book");
todoList.push_back("Go for a walk");
// Displaying the to-do list
std::cout << "To-Do List:" << std::endl;
for (const auto &task : todoList) {
std::cout << "- " << task << std::endl;
}
return 0;
}
In this example, we define a list of strings to represent tasks. We add several tasks to the list and then iterate through the list to display the items. This simple program encapsulates how lists can effectively manage dynamically changing datasets such as a to-do list.
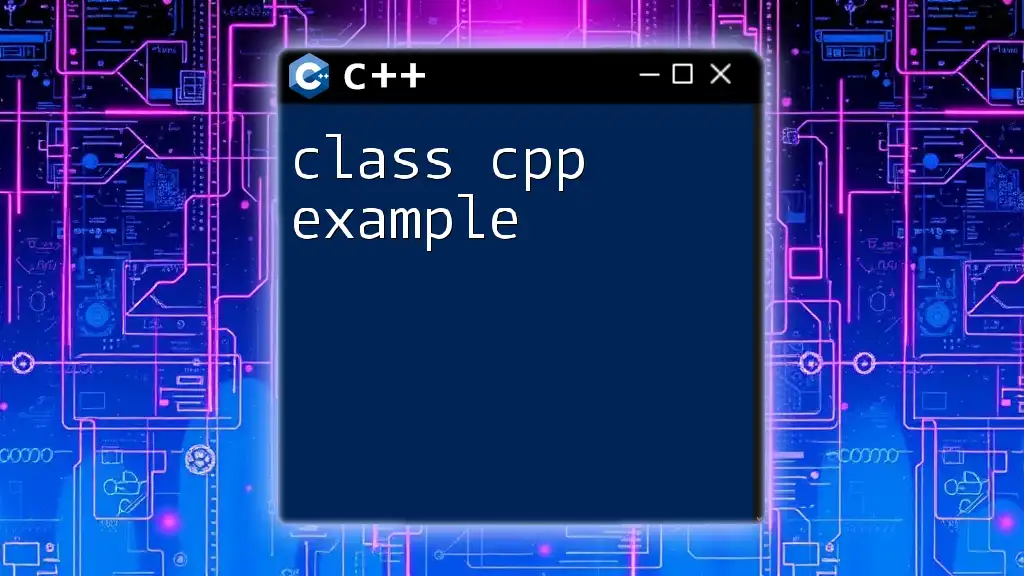
Conclusion
C++ lists are a powerful and versatile tool that can enhance your programming capabilities significantly. By enabling dynamic sizing, efficient insertions and deletions, and advanced operations like sorting and merging, lists become an indispensable component in the C++ programmer's toolkit.
To fully master C++, exploring and practicing with lists will prove vital. Take the time to experiment with lists—create them, manipulate them, and incorporate them into your projects!
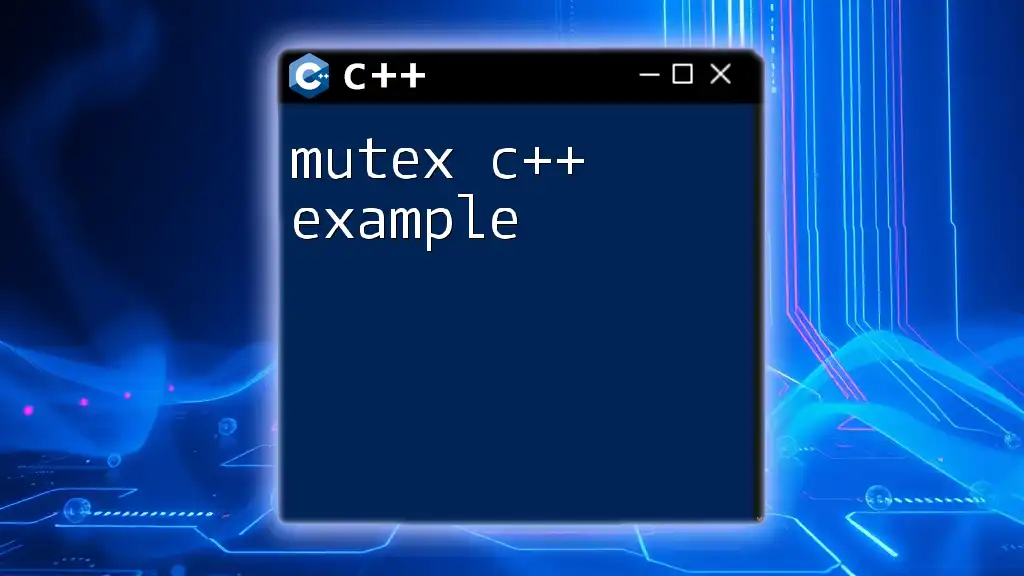
Frequently Asked Questions (FAQs)
What are the advantages of using lists in C++?
The advantages of using lists in C++ include dynamic sizing, efficient insertion and deletion operations, and automatic memory management. These features make it incredibly useful for applications where the volume of data can change frequently.
Can I store different data types in a C++ list?
In C++, a list can only store elements of a single data type. However, you can define a list of a user-defined type or a base class, and store subclasses or various types if you use pointers.
When should I choose a list over a vector?
Choose a list when you need frequent insertions or deletions in the middle of the collection, as lists operate with higher efficiency in such cases compared to vectors. Conversely, if you require random access to elements or performance in accessing and traversing elements, vectors may be a better fit.
How can I convert a list to a vector?
To convert a list to a vector, you can create a vector and then use the `assign` method or a constructor that takes iterators. Here’s an example of using the constructor:
std::list<int> myList = {1, 2, 3, 4, 5};
std::vector<int> myVector(myList.begin(), myList.end());
This integration facilitates flexibility in data structure utilization depending on the program requirements.