Here’s a concise explanation along with a code snippet showcasing the use of `cin` in C++:
In C++, `cin` is used to take input from the standard input stream, allowing users to provide data to the program.
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
What is C++ cin?
Understanding the cin Object
The `cin` object in C++ plays a crucial role in handling user input. It is a standard input stream, a part of the `<iostream>` library, which enables the program to read data from the keyboard (or another input source). Essentially, `cin` allows users to provide input to the program, making interaction with applications possible.
The Syntax of cin
The syntax for using `cin` is fairly straightforward. The extraction operator `>>` is essential for reading data into a variable. Here's an example of how you can use `cin` in a simple C++ program:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this example, the user is prompted to enter their age, which is then stored in the variable `age` and displayed back to them.
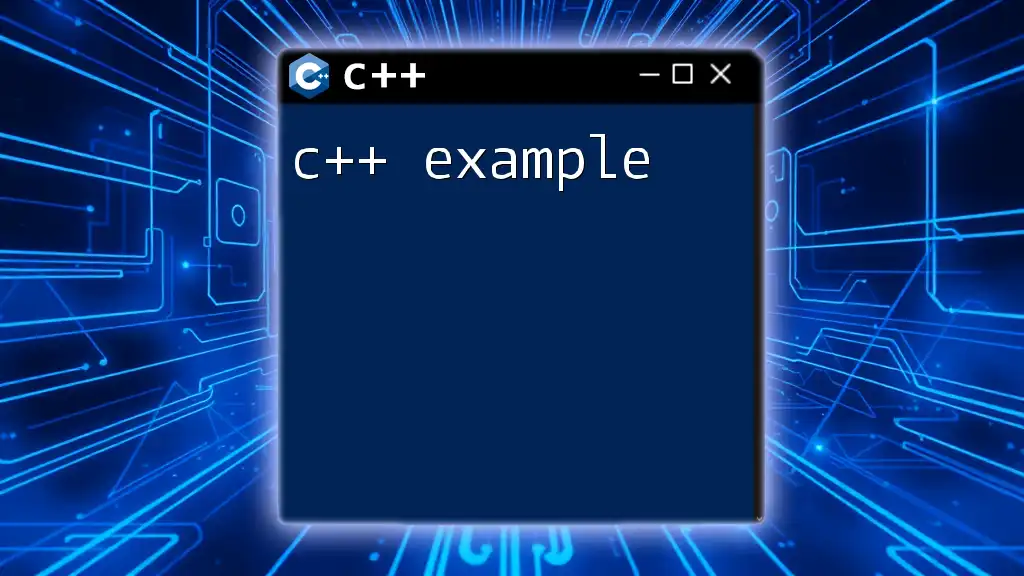
How to Use cin in C++
Basic Input Operations
C++ allows you to read various data types using `cin`, including integers, floating-point numbers, and strings. Here’s how you can do it:
- Reading an integer: You can read an integer by directly assigning `cin` to an integer variable.
int number;
cout << "Enter an integer: ";
cin >> number;
- Reading a floating-point number: To read a float, declare a float variable and use `cin` similarly.
float price;
cout << "Enter a floating point number: ";
cin >> price;
- Reading a string: String inputs can also be accepted using `cin`, but remember that it stops reading at the first whitespace.
string name;
cout << "Enter your name: ";
cin >> name;
Putting it all together, here’s a program that demonstrates how to read multiple data types:
#include <iostream>
#include <string>
using namespace std;
int main() {
int number;;
float price;
string name;
cout << "Enter an integer: ";
cin >> number;
cout << "Enter a floating point number: ";
cin >> price;
cout << "Enter your name: ";
cin >> name;
cout << "Integer: " << number << ", Price: " << price << ", Name: " << name << endl;
return 0;
}
Common Pitfalls When Using Cin
While using `cin`, programmers might encounter various challenges, particularly when handling input errors. One common issue occurs when users input an incorrect data type. If, for example, a user tries to enter a letter when the program expects a number, it will raise an error.
You can check for this by verifying whether the input operation succeeded with `cin.fail()`. Here's an example of handling such an error:
#include <iostream>
using namespace std;
int main() {
int value;
cout << "Enter a number: ";
cin >> value;
if (cin.fail()) {
cout << "Invalid input! Please enter numeric values only." << endl;
cin.clear(); // Clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Ignore the invalid input
}
return 0;
}
In this snippet, if the user enters an invalid value, we catch the error and reset the error state of `cin`, allowing for new input attempts.
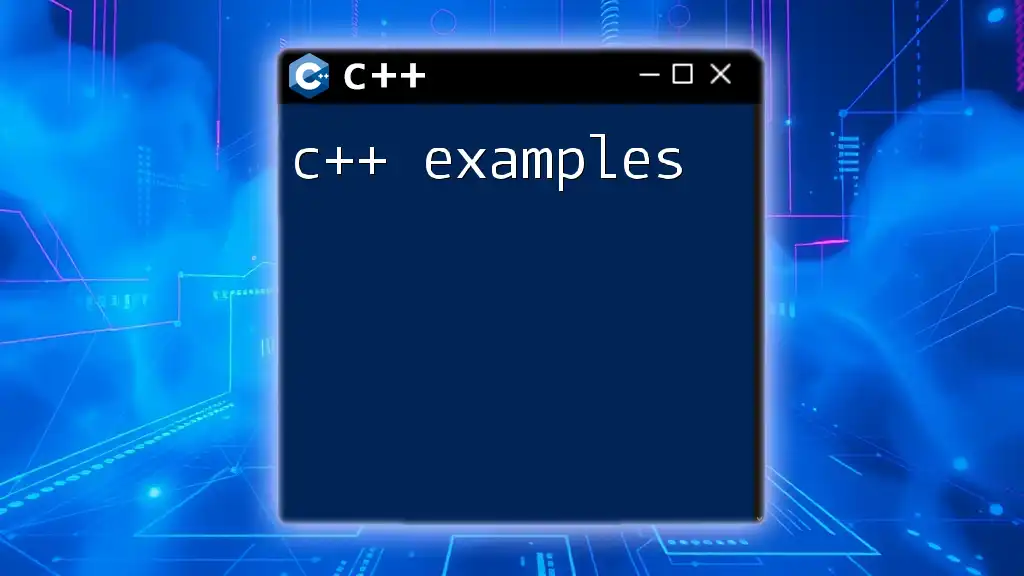
Advanced Usage of cin
Using cin with Custom Data Types
In addition to basic data types, `cin` can also be used to read inputs into user-defined classes or structures. This can be particularly useful when collecting complex data from users.
Here's an example of a C++ class for a book, allowing user input for the title and author:
#include <iostream>
using namespace std;
class Book {
public:
string title;
string author;
void display() {
cout << "Title: " << title << ", Author: " << author << endl;
}
};
int main() {
Book book;
cout << "Enter book title: ";
cin >> book.title;
cout << "Enter book author: ";
cin >> book.author;
book.display();
return 0;
}
In this example, the user is prompted to provide a book’s title and author, both of which are stored as properties of the `Book` class.
cin and Input Validation
Input validation is essential to ensure that the data received from users meets specific criteria. In C++, you can implement input validation by using loops to enforce constraints on user input.
For instance, if you want to ensure that a user enters an option between 1 and 5, you can utilize a `do-while` loop like this:
#include <iostream>
using namespace std;
int main() {
int choice;
do {
cout << "Enter a choice (1-5): ";
cin >> choice;
} while (choice < 1 || choice > 5);
cout << "You chose option " << choice << endl;
return 0;
}
In this example, the program continues to prompt the user until a valid input is provided, ensuring that the choice meets the required criteria.
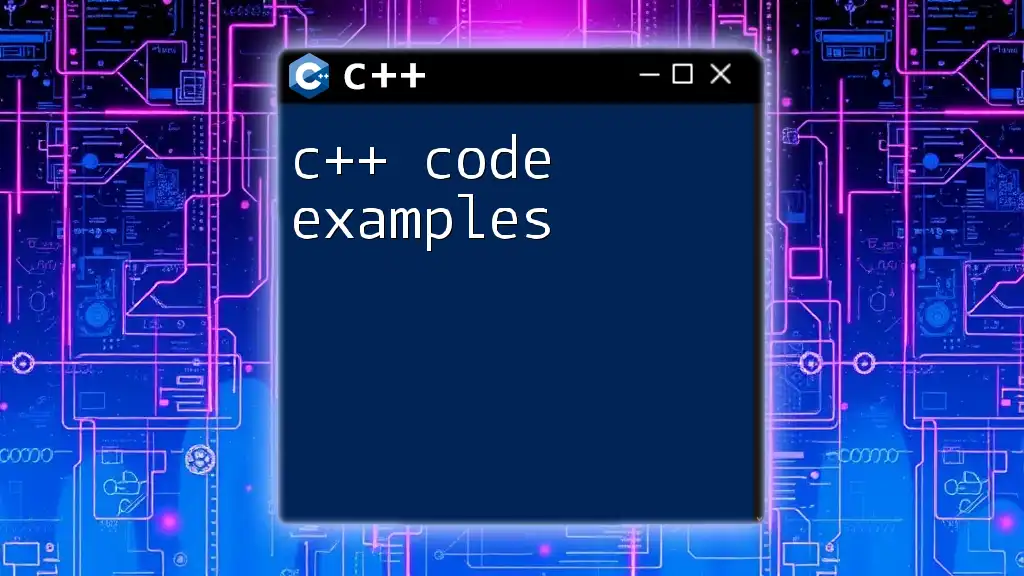
Conclusion
In summary, the `cin` object is an integral part of user interaction in C++ programming. By understanding its syntax and potential pitfalls, as well as mastering various input operations and validation methods, you can create robust applications that handle user inputs gracefully. Don’t hesitate to experiment with `cin` in your C++ projects, as mastering it allows for engaging and dynamic user experiences.
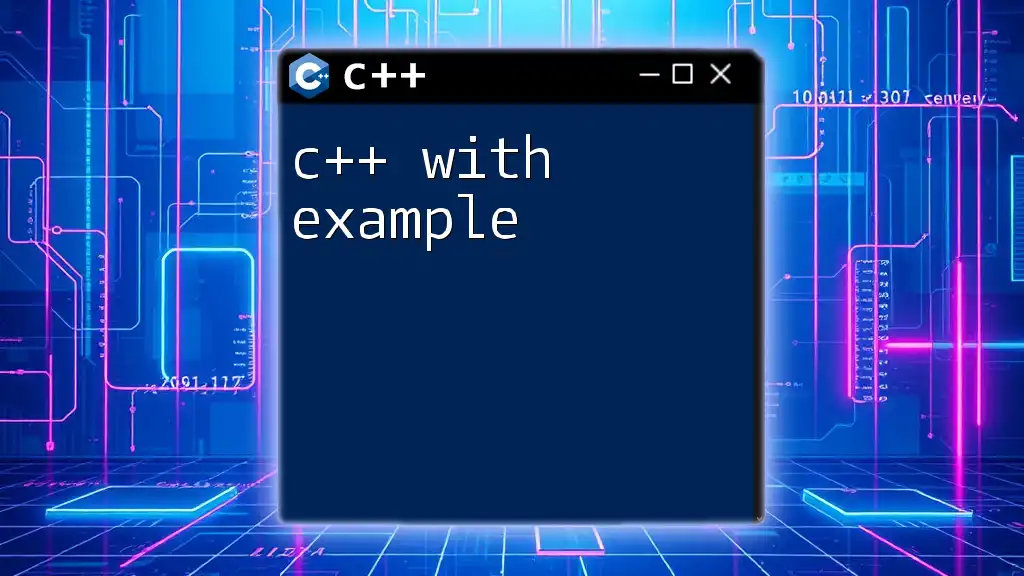
Additional Resources
For further learning, consider checking out the official C++ documentation or various online resources. Engaging with communities and forums can also provide additional support, insights, and practical experiences as you delve deeper into C++ programming.