C++ code examples illustrate fundamental programming concepts through simple scripts, enabling learners to grasp syntax and usage quickly.
Here’s a basic example of a C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Syntax and Structure
Before diving into specific C++ code examples, it is crucial to grasp the fundamental structure of a C++ program. A typical C++ program begins with preprocessor directives, includes necessary libraries, and has a well-defined `main` function, which serves as the entry point of the application.
Here is a basic example to illustrate this structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` allows us to use input/output functionalities such as `std::cout`. Inside the `main` function, we print "Hello, World!" to the console, and the program returns 0, indicating successful execution.
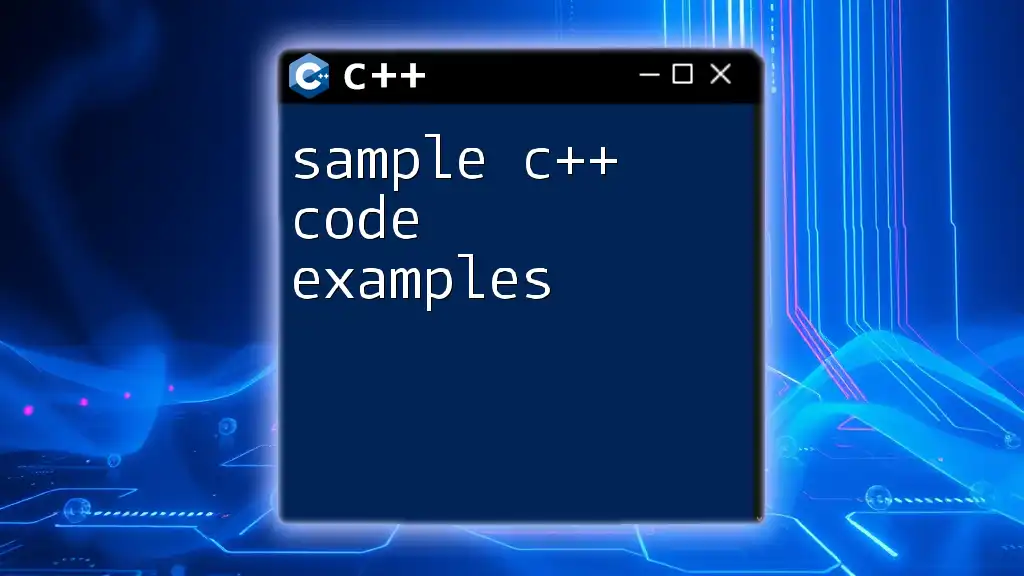
Essential Elements of C++ Code
Variables and Data Types
Variables play a pivotal role in C++ programming as they store data that can be manipulated throughout the code. Each variable must be defined with a specific data type that dictates what kind of data it can hold. Common data types include:
- `int`: for integers
- `double`: for floating-point numbers
- `char`: for characters
Here’s an example demonstrating variable declaration and usage:
int age = 30;
double salary = 50000.50;
char initial = 'A';
These variables can now be used in various operations within the program, showcasing how fundamental they are to C++ code examples.
Control Structures
Control structures enable the flow of the program based on conditions. They are essential for making decisions and repeating actions.
If-Else Statements
Conditional statements allow your program to execute different paths based on boolean expressions. Here is a simple C++ code example using an if-else statement:
if (age > 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
In this example, the program checks whether the variable `age` is greater than 18 and prints the appropriate message based on the condition.
Loops
Loops are used to repeat a block of code multiple times. The primary types of loops in C++ include `for`, `while`, and `do-while`.
Here's a simple loop that prints numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This loop initializes `i` to 0 and keeps incrementing it until it reaches 5, printing each value in the range.
Functions in C++
Functions are blocks of code designed to perform a particular task and can be reused throughout the program to avoid redundancy.
Defining and Calling Functions
Defining functions introduces modularity to your C++ code. Here’s a simple function definition and invocation:
void greet() {
std::cout << "Welcome to C++!";
}
int main() {
greet(); // Call to greet function
return 0;
}
In this code, `greet` is a function that prints a welcoming message. It is called within `main`, demonstrating how functions can encapsulate tasks.
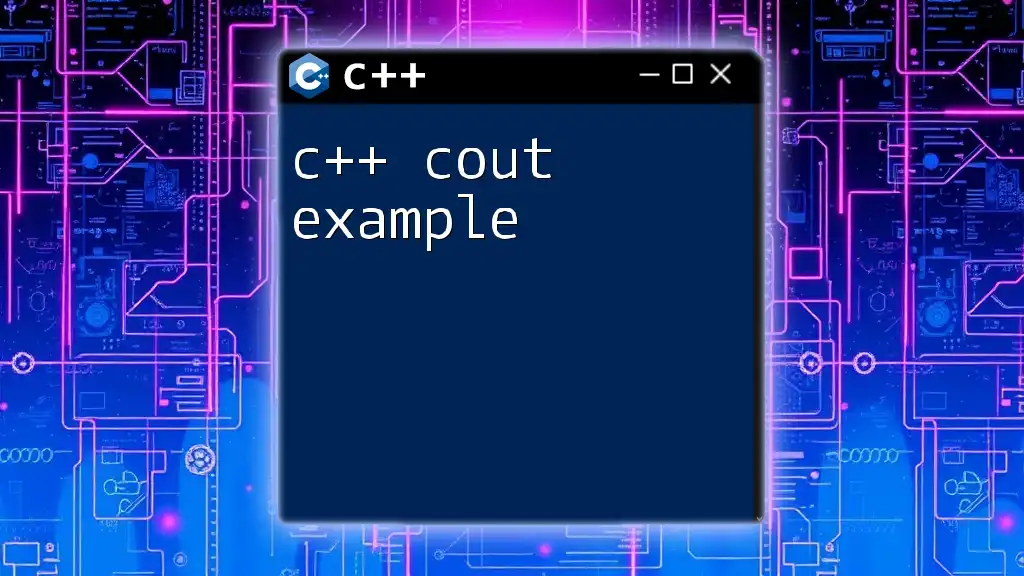
C++ Code Examples for Common Use Cases
File Handling Example
File handling is a crucial aspect of C++ programming, allowing you to store and retrieve data efficiently. We can read from and write to files using the `fstream` library.
Here's an example of writing to a file:
#include <fstream>
#include <iostream>
int main() {
std::ofstream outfile("example.txt");
outfile << "Hello, File!" << std::endl;
outfile.close();
return 0;
}
In this snippet, we create an output file stream, write to "example.txt", and then close the file. This basic file handling is an essential skill for effective data management.
Object-Oriented Programming in C++
C++ is known for its object-oriented programming (OOP) capabilities, allowing us to create classes and objects, encapsulate data, and implement inheritance.
Classes and Objects
Classes are blueprints for creating objects and typically contain member variables and functions. Here's a sample code demonstrating a simple class and its object:
class Car {
public:
void honk() {
std::cout << "Honk! Honk!" << std::endl;
}
};
int main() {
Car myCar;
myCar.honk(); // Call honk function from Car class
return 0;
}
This code creates a `Car` class with a method `honk`. An object of `Car`, named `myCar`, is instantiated and used to call the `honk` method.
Inheritance Example in C++
Inheritance allows one class to inherit the properties of another, promoting code reusability. Here’s an example of single inheritance:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started!" << std::endl;
}
};
class Bike : public Vehicle {
public:
void ringBell() {
std::cout << "Bike bell rings!" << std::endl;
}
};
int main() {
Bike myBike;
myBike.start(); // Inherited from Vehicle class
myBike.ringBell();
return 0;
}
In this example, `Bike` inherits from `Vehicle`, allowing it to call the `start` method in addition to its own `ringBell` method.
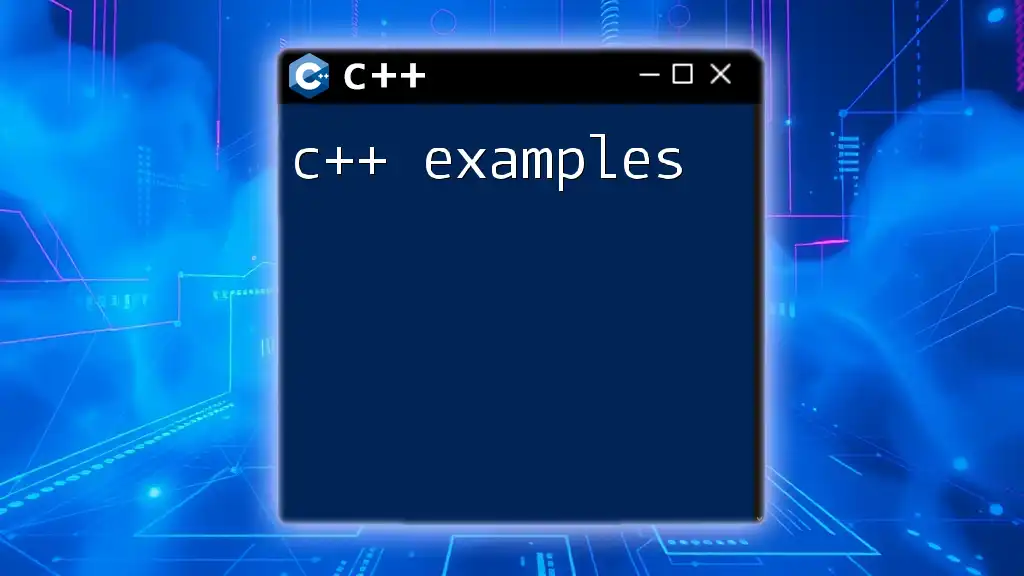
C++ Sample Programs for Beginners
Simple Program in C++: Basic Calculator
Creating a basic calculator is an excellent way to understand fundamental concepts like user input, conditions, and operations. Here’s a complete example:
#include <iostream>
int main() {
double num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter operator (+, -, *, /): ";
std::cin >> operation;
std::cout << "Enter second number: ";
std::cin >> num2;
switch (operation) {
case '+':
std::cout << "Result: " << num1 + num2 << std::endl;
break;
case '-':
std::cout << "Result: " << num1 - num2 << std::endl;
break;
case '*':
std::cout << "Result: " << num1 * num2 << std::endl;
break;
case '/':
if (num2 != 0)
std::cout << "Result: " << num1 / num2 << std::endl;
else
std::cout << "Division by zero is not allowed!" << std::endl;
break;
default:
std::cout << "Invalid operation!" << std::endl;
}
return 0;
}
This program prompts the user for two numbers and an operation. It then performs the requested operation using a switch-case statement, demonstrating control flow and basic arithmetic.
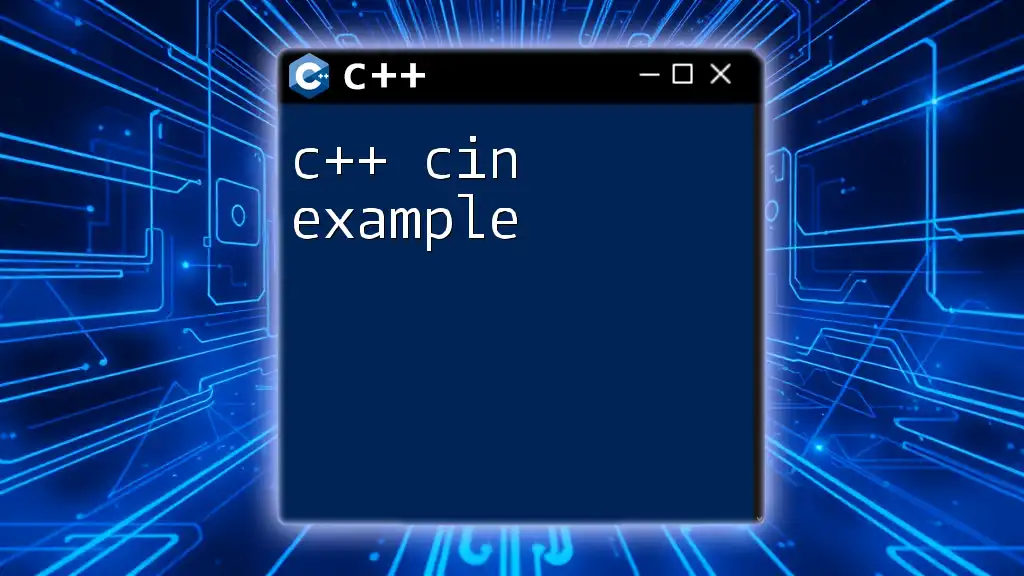
Resources for Further Learning
To deepen your understanding of C++, consider exploring various resources that provide additional C++ source code examples and learning materials. Quality resources include:
- Books dedicated to C++ programming.
- Comprehensive online courses that accommodate different learning styles.
- Official documentation and tutorials.
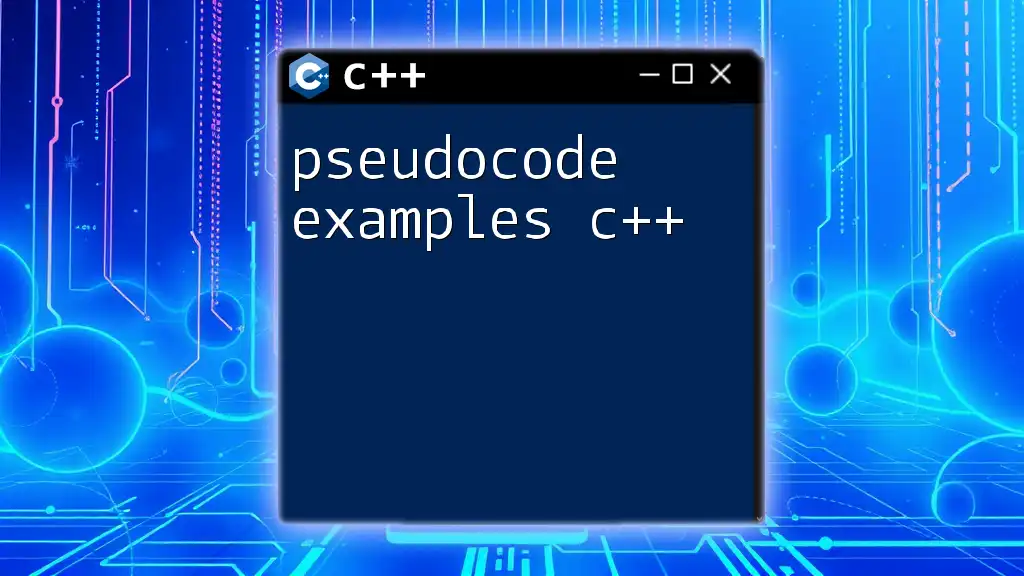
Conclusion
Practicing with C++ code examples helps solidify your understanding and proficiency in the language. The snippets provided in this article offer a starting point for experimenting and building your own programs. Remember, the best way to learn programming is to write code! Embrace the process, explore advanced concepts, and let your projects evolve through practice.
Call to Action
Feel free to subscribe for more in-depth content on C++ programming, and don’t hesitate to share your thoughts or questions in the comments section! Happy coding!