C++ Codecademy is an online learning platform that offers interactive lessons to help beginners master C++ programming through practical examples and hands-on exercises.
Here’s a simple code snippet demonstrating the use of a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Codecademy?
Codecademy is an online learning platform that focuses on teaching various programming languages and technologies through interactive courses. It provides a hands-on approach to learning, enabling users to write and execute code directly in their web browsers. The platform is designed to cater to beginners and experienced learners alike, with a range of features, including interactive coding exercises, real-world projects, and strong community support.
Choosing Codecademy for C++ offers several advantages. Its user-friendly interface allows learners to progress at their own pace, ensuring a stress-free learning experience. Additionally, interactive lessons reinforce concepts as users engage with practical coding tasks, making the learning process both enjoyable and effective.
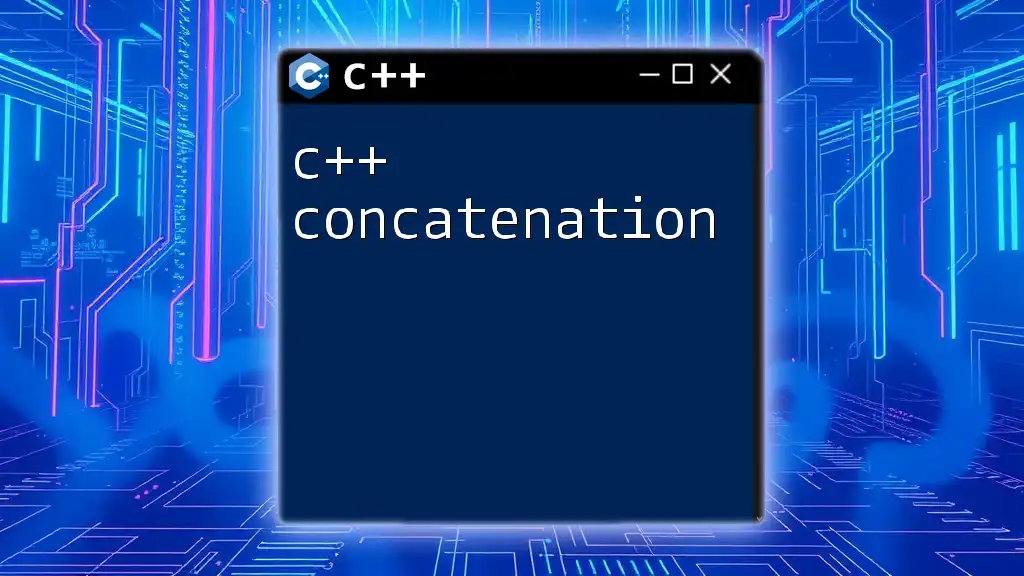
C++ on Codecademy: Overview of the Course Structure
The C++ course on Codecademy is thoughtfully structured into modules, each focusing on specific programming concepts essential for becoming a proficient C++ programmer. Key modules include:
- Introduction to C++: Provides foundational knowledge and syntax.
- Variables and Data Types: Covers how to store and manipulate data.
- Control Structures: Introduces decision-making and looping constructs.
- Functions: Explores how to encapsulate code for reusability.
- Object-Oriented Programming: Offers insights into designing programs using classes and objects.
Throughout the course, learners are guided through clear learning objectives, such as mastering basic syntax, creating efficient programs, and implementing OOP concepts.
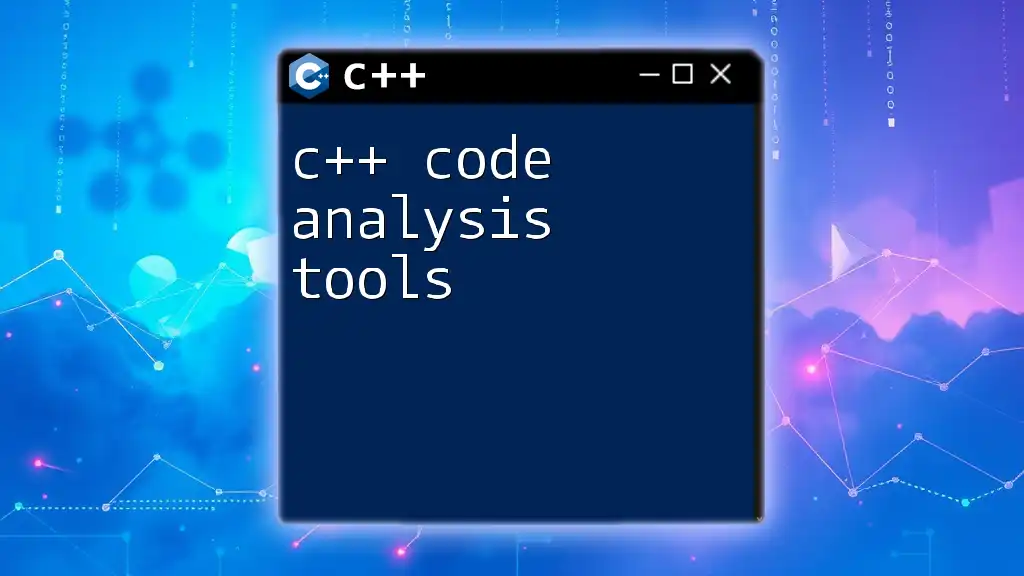
Getting Started with C++ on Codecademy
To kickstart your journey with C++ Codecademy, the first step is to create an account on the platform. This process is straightforward: simply visit the Codecademy website, and follow the prompts to sign up. During registration, you will have the option to choose between a free and a Pro membership. While the free version offers access to fundamental lessons, the Pro membership unlocks advanced projects, quizzes, and real-time feedback from mentors, all of which are particularly beneficial for comprehensive learning in C++.
Setting up your development environment is also crucial. Codecademy provides an online IDE, allowing you to code without needing to install anything. However, if you prefer to work offline, popular IDEs such as Visual Studio, Code::Blocks, or CLion can create a suitable environment for C++ development.
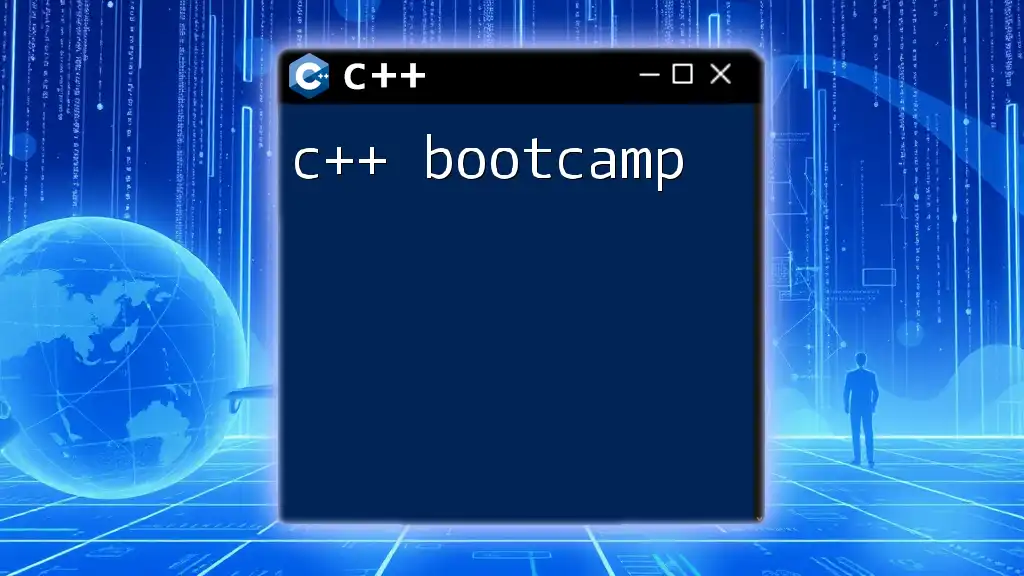
Key Concepts in C++ on Codecademy
Introduction to Syntax and Structure
Understanding the syntax and structure of C++ is vital for beginners. The fundamental syntax enables learners to write code correctly and effectively. For example, a basic "Hello World" program in C++ is structured like this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This snippet demonstrates how to include libraries, define the main function, output text to the console, and return an exit status.
Variables and Data Types
In C++, variables store data, and understanding data types is essential for efficient programming. The most common data types include integers, floats, and characters. Here’s a quick example:
int age = 25;
float height = 5.9;
char initial = 'A';
In this example, we declare an integer variable `age`, a float variable `height`, and a character variable `initial`, demonstrating how to associate values with identifiers.
Control Structures
Control structures guide the flow of programs based on conditional statements and loops. For instance, if-else statements allow the program to make decisions based on conditions. An example code snippet is:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
In this situation, the program checks whether `age` is 18 or older and prints a corresponding message.
Functions in C++
Functions in C++ promote reusability and maintainability. By defining a function, developers can execute a block of code whenever needed with given arguments. Consider this simple function to add two integers:
int add(int a, int b) {
return a + b;
}
In this example, the `add` function takes two integer parameters `a` and `b`, returning their sum. Functions like this streamline the code and help avoid repetition.
Object-Oriented Programming in C++
Object-Oriented Programming (OOP) is a critical paradigm in C++. It focuses on encapsulating data and behaviors in objects created from classes. Here's a fundamental implementation of a class:
class Car {
public:
void honk() {
std::cout << "Beep beep!" << std::endl;
}
};
In this example, the `Car` class contains a public method `honk`, which outputs a sound when invoked. Classes provide a powerful way to structure code and manage complexity through encapsulation and inheritance.
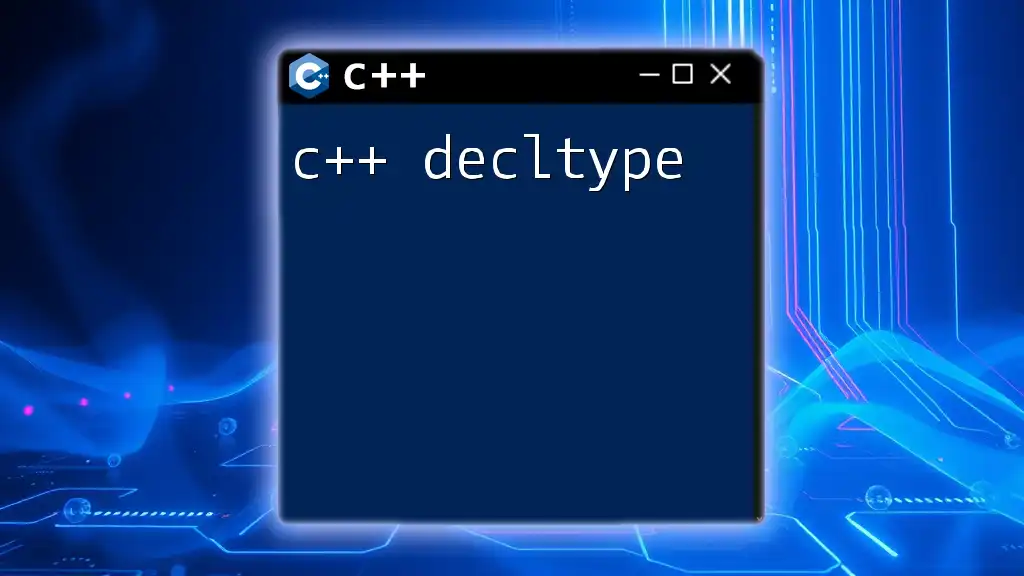
Practice and Assessment
Codecademy emphasizes the importance of practice, incorporating interactive quizzes and challenges throughout the C++ course. These assessments help reinforce learned concepts and provide immediate feedback. Additionally, learners can work on real-world projects that simulate actual software development tasks, enhancing their skills and confidence.
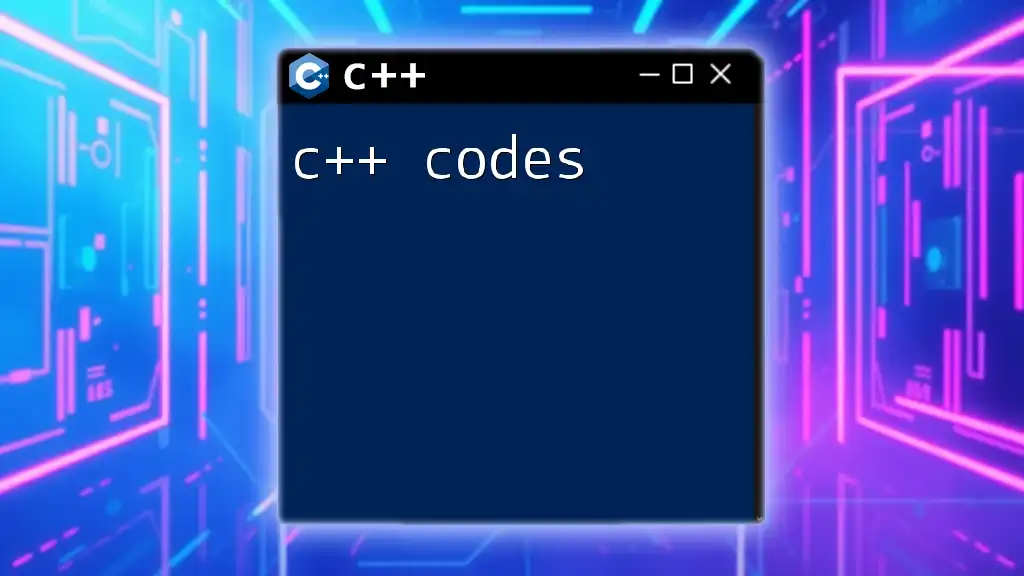
Community and Support on Codecademy
The Codecademy community is a valuable resource for learners. Through forums and discussion groups, users can ask questions, share knowledge, and seek assistance from peers and mentors. Engaging with other learners fosters collaboration and creates a supportive learning environment.
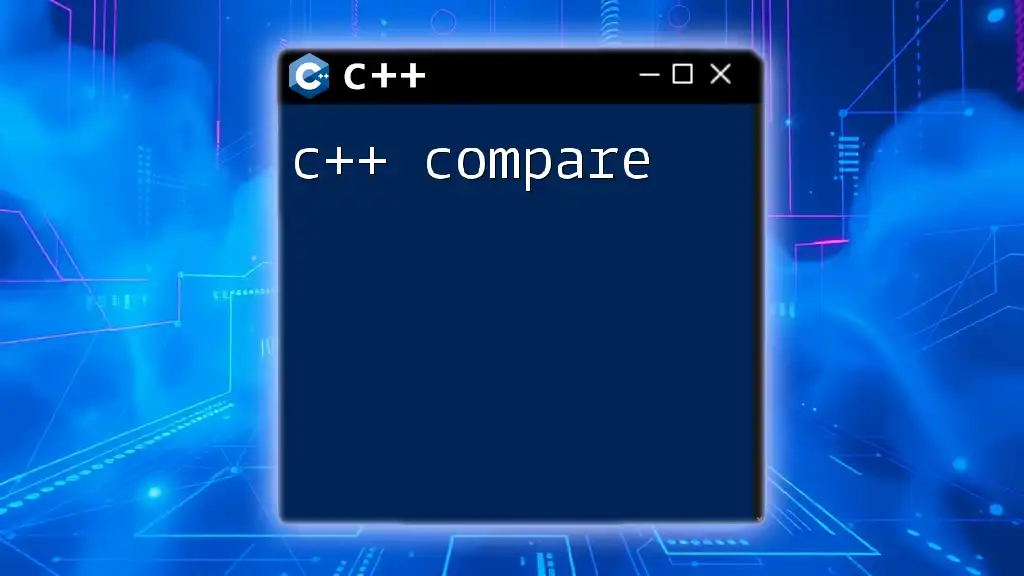
Advanced C++ Topics Available on Codecademy
For those looking to deepen their C++ knowledge, Codecademy offers advanced topics such as Templates and the Standard Template Library (STL), Exception Handling, and Multithreading. These subjects build on the foundational skills acquired and prepare learners for real-world programming challenges.
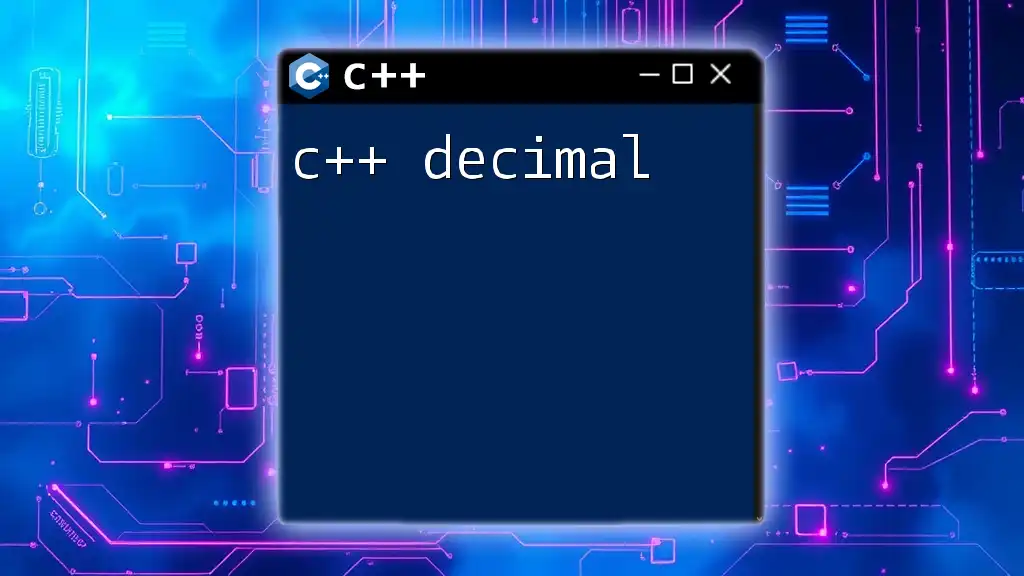
Conclusion
The C++ Codecademy course provides a well-rounded pathway for individuals eager to learn this influential programming language. With modules covering essential concepts, interactive exercises, and a supportive community, learners can confidently navigate their way through the complexities of C++. By continuing to practice and explore resources beyond Codecademy, aspiring C++ programmers will find numerous opportunities to advance their skills and careers.
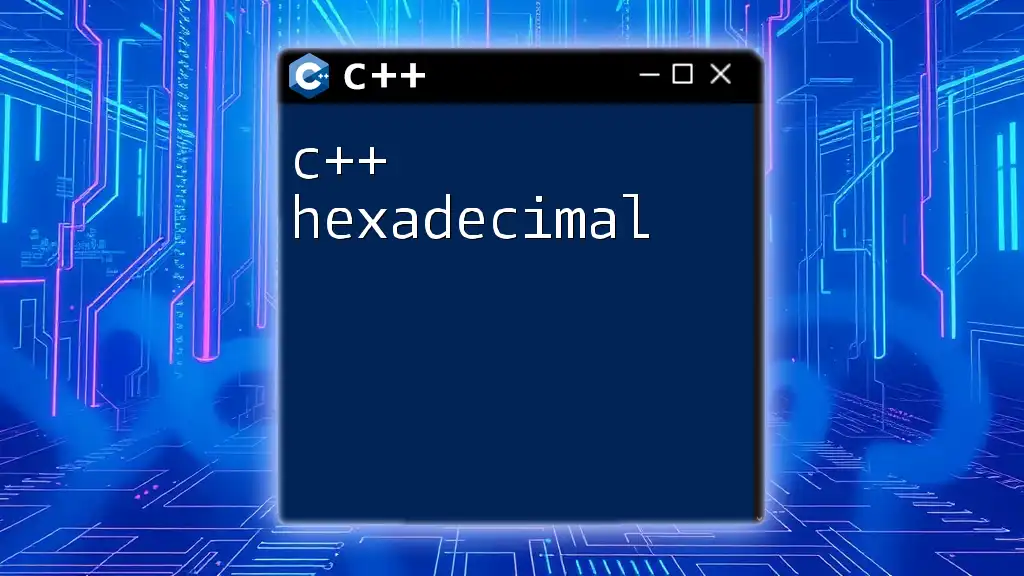
Call to Action
Now is the perfect time to join Codecademy and embark on your journey to mastering C++. Whether you're a complete beginner or looking to refine your skills, Codecademy offers resources that cater to your learning needs. Explore additional resources available on C++ and reach out for personalized guidance as you grow in your programming journey!
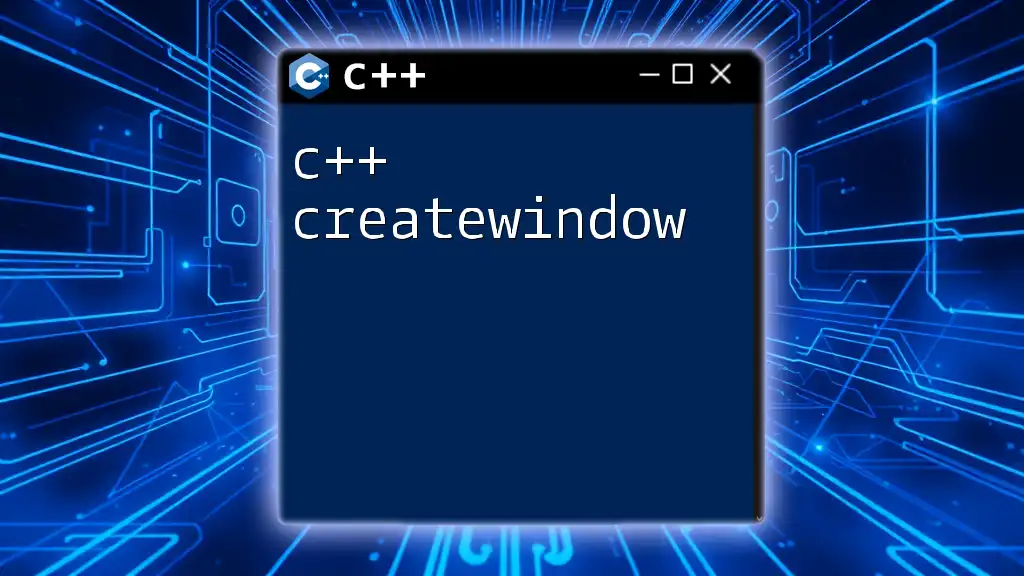
Additional Resources
For those wanting to delve deeper into C++, consider checking out official C++ documentation, recommended books, and discount offers for Codecademy Pro membership to further enhance your coding experience.