A C++ Bootcamp is an intensive training program designed to quickly equip individuals with essential C++ programming skills through hands-on coding exercises and practical projects.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What to Expect in a C++ Bootcamp
Program Structure
A C++ Bootcamp typically involves a structured curriculum that guides learners through the essential concepts of C++. These programs can vary in duration and format. Many bootcamps offer an intensive schedule lasting anywhere from a few weeks to a few months, accommodating different learning styles with options for online, in-person, or hybrid formats.
In a typical bootcamp, participants can expect to cover a diverse curriculum that includes fundamental programming skills, project-based learning, and advanced topics like object-oriented programming.
Learning Outcomes
Upon completion of a C++ bootcamp, students are equipped with valuable skills and a solid grasp of core programming principles. Graduates commonly emerge with a portfolio showcasing real-world projects, which they can leverage when applying for jobs. They will have practical experience in C++ syntax, data structures, algorithms, and the ability to debug and optimize code effectively.
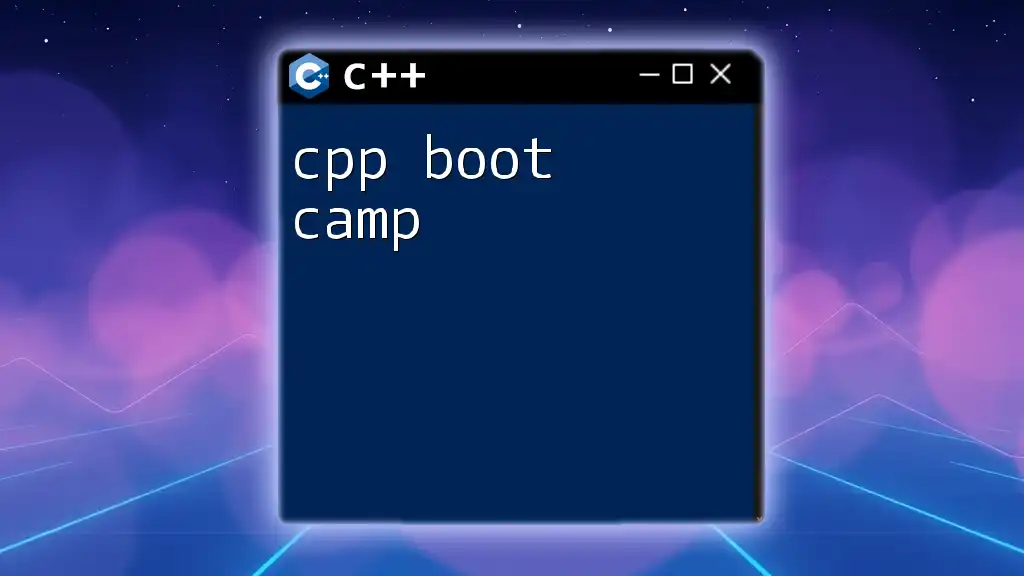
Getting Started with C++
Installation of Development Tools
To dive into C++, the first step is to set up a proper development environment. Choosing the right compiler and Integrated Development Environment (IDE) is crucial for a seamless coding experience.
- Compilers: Recommended compilers include GCC, Clang, and MSVC. For beginners, GCC is often preferred due to its robustness and support for multiple platforms.
- IDEs: Popular IDEs such as Code::Blocks, Visual Studio, or even lightweight editors like Visual Studio Code can streamline your coding process.
Example: Installing GCC on Windows
To install GCC on Windows, follow these steps:
- Download MinGW, a minimalist GNU for Windows.
- During installation, select the C++ compiler and ensure it updates your system's PATH variable.
First Program: Hello World
Every programming journey starts with the iconic "Hello, World!" program. This simple code illustrates the fundamental structure of a C++ program.
Here’s a simple code snippet to get you started:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `#include <iostream>` includes the header file necessary for input-output operations. The `main()` function serves as the entry point of the program, with `std::cout` used to display output on the screen. The `return 0;` statement indicates successful execution.
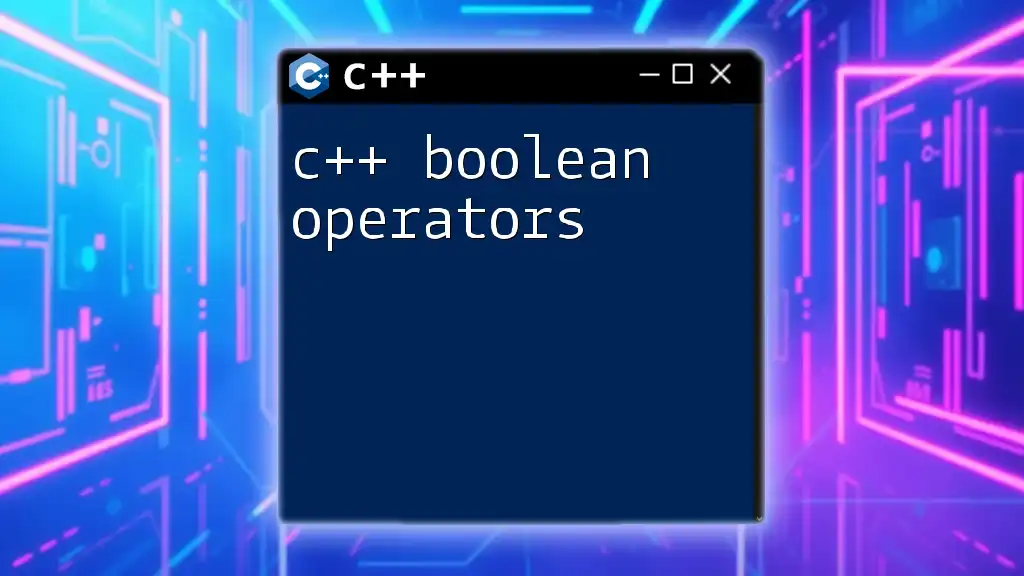
Core Concepts in C++
Data Types and Variables
Understanding data types is critical in C++. C++ supports various fundamental types, including `int`, `float`, `char`, and `double`.
For instance:
int number = 10; // Integer type
float pi = 3.14; // Floating-point type
char letter = 'A'; // Character type
Different data types allow developers to manage memory efficiently and choose the most suitable type for their applications, paving the way for more efficient resource management.
Control Structures
Control structures, such as conditional statements and loops, control the flow of a program's execution.
If Statements and Loops
For example:
for (int i = 1; i <= 5; ++i) {
std::cout << i << std::endl;
}
This loop prints numbers 1 through 5. Understanding flow control is essential for implementing logic in your programs, allowing for dynamic decision-making based on user input or other factors.
Functions and Scope
Functions are essential for organizing code, promoting reusability, and separating logic. Here's how you can define a function in C++:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
This example illustrates function overloading, where multiple functions can share the same name but differ in parameter types.
Scope refers to the visibility and lifetime of variables, which helps prevent naming conflicts and manages memory more effectively.
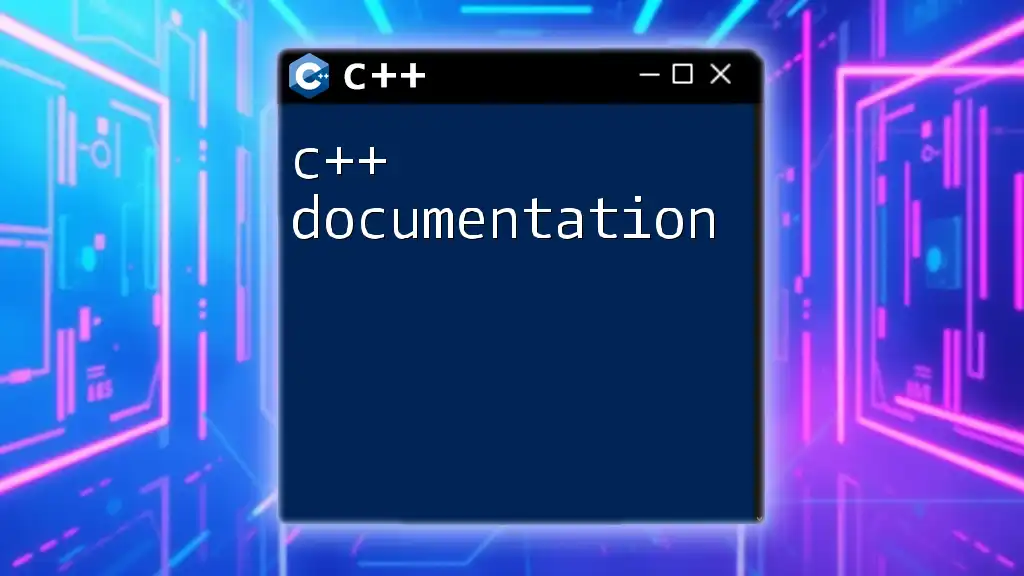
Object-Oriented Programming in C++
Class and Object Fundamentals
C++ is renowned for its support of Object-Oriented Programming (OOP) principles. OOP promotes code organization and modularity by using classes and objects.
Here’s a simple class definition:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this code snippet, the `Dog` class has a public method `bark()`, which outputs "Woof!" to the console. This encapsulation of data and methods is a fundamental tenet of OOP, enabling more complex system modeling.
Inheritance and Polymorphism
Inheritance allows classes to derive properties and behaviors from other classes, promoting code reuse. Consider the following example:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Cat : public Animal {
public:
void meow() {
std::cout << "Meow!" << std::endl;
}
};
Here, `Cat` inherits from `Animal`, gaining access to its `eat()` method while also defining its specific behavior.
Polymorphism, another cornerstone of OOP, enables one interface to control access to a general class of actions, allowing methods to be used interchangeably.
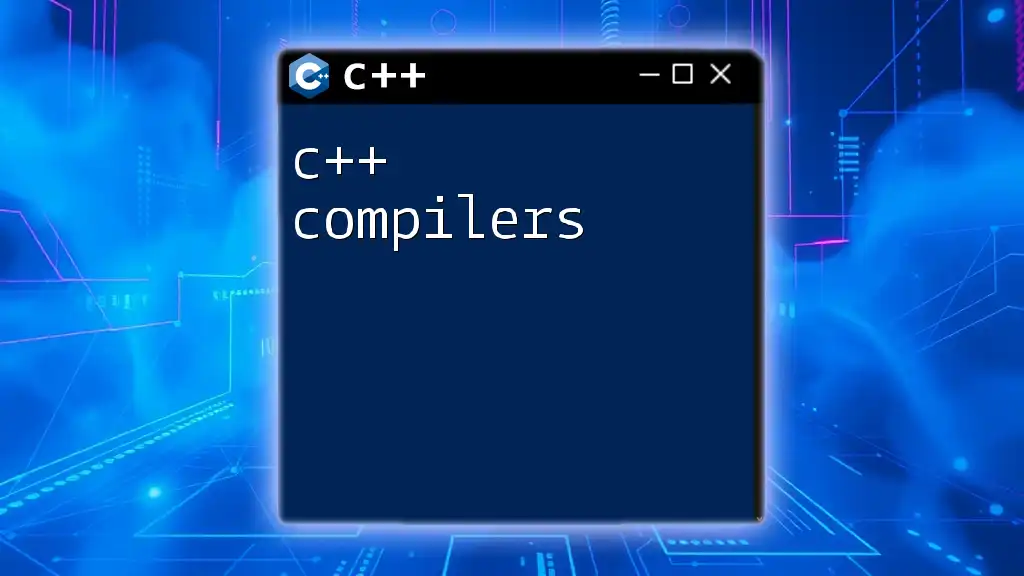
Advanced C++ Features
Templates and Generic Programming
Templates are powerful tools that provide a way to write generic and reusable code. By using templates, programmers can create functions that work with any data type. Consider the following template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can add numbers of any data type, such as `int`, `float`, or even user-defined types, enhancing flexibility and code reuse.
Standard Template Library (STL)
The STL is a vital component of C++ that provides a collection of ready-to-use classes and functions for data structures (like vectors, lists, and maps) and algorithms (like sorting and searching).
For instance, using a vector to store a sequence of integers is straightforward:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4};
for (int num : numbers) {
std::cout << num << std::endl;
}
Utilizing STL not only simplifies coding but also optimizes functionalities, saving time and effort.
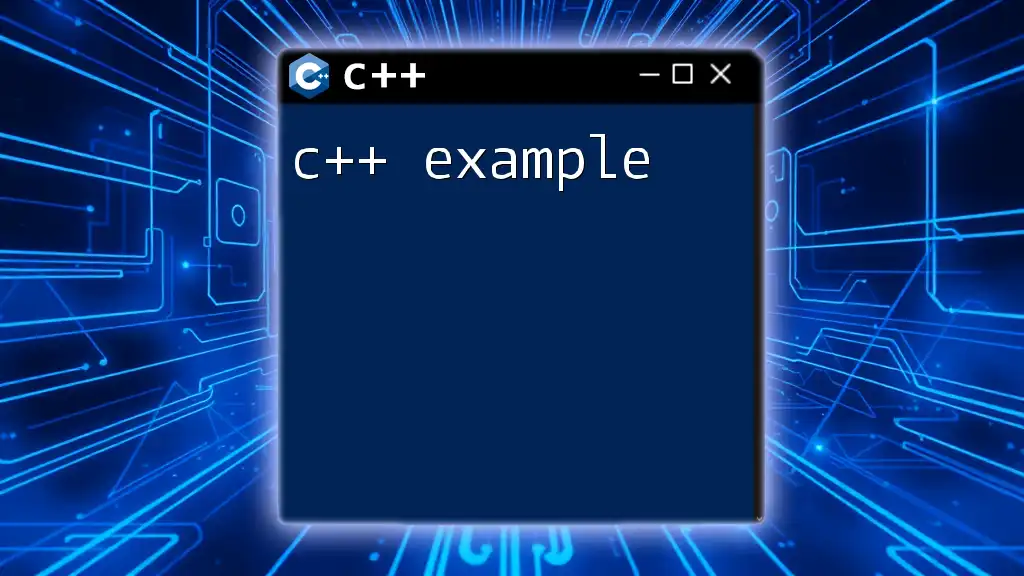
Practical Applications of C++
Building Real-World Applications
Graduating from a C++ Bootcamp typically involves hands-on projects where participants can build real-world applications. These projects can range from simple command-line tools to more complex applications, showcasing problem-solving skills.
Collaborative Projects and Code Reviews
Collaborative coding environments enhance the learning experience through peer interaction and feedback. Utilizing version control systems like Git and platforms like GitHub encourages teamwork, allowing for code reviews and continuous integration practices.
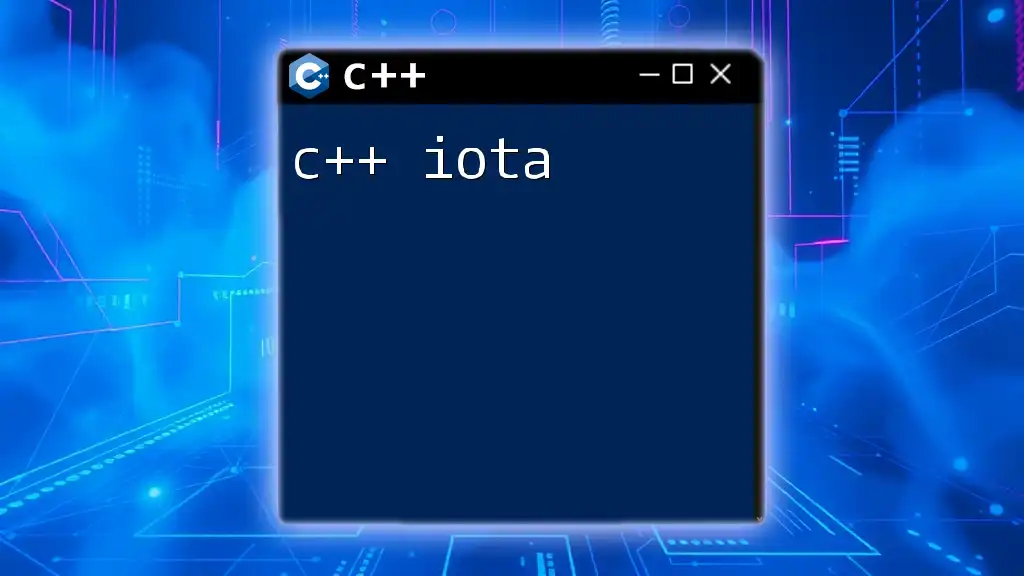
How to Choose the Right C++ Bootcamp
Factors to Consider
When selecting a C++ Bootcamp, several factors should guide the decision-making process:
Curriculum Depth and Breadth: Evaluate whether the bootcamp covers essential programming concepts comprehensively, along with advanced topics. Instructor Qualifications: Look for instructors with industry experience and a strong understanding of C++ applications.
Reviews and Testimonials
Student feedback is invaluable. Investigate reviews to gain insights into others’ experiences and the effectiveness of the program. This information can help determine if the bootcamp aligns with your learning goals.
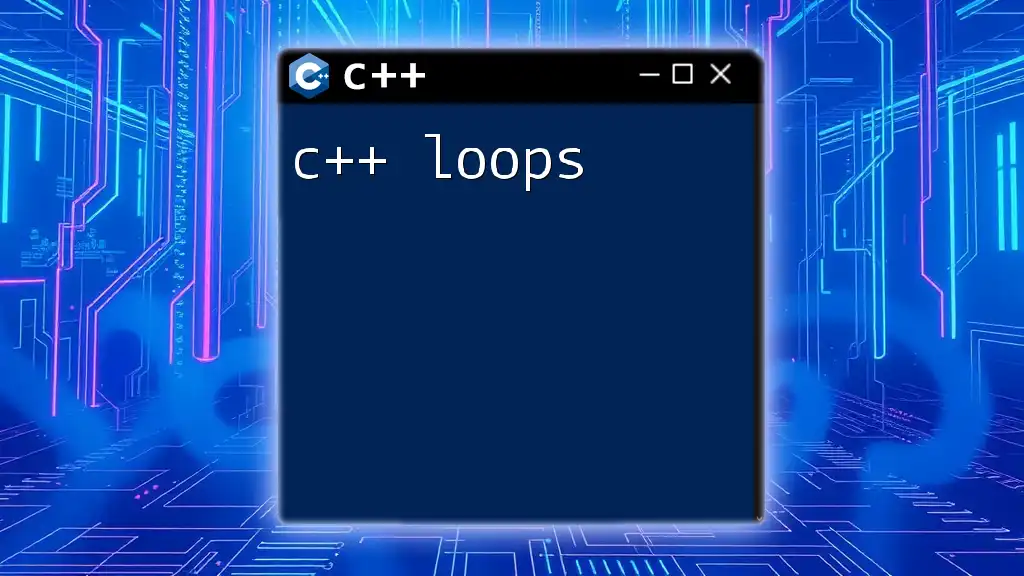
Conclusion
A C++ Bootcamp offers an intensive and focused learning experience, enabling participants to acquire essential programming skills effectively. By engaging actively in hands-on projects, collaborating with peers, and building a strong foundation in C++, graduates are well-positioned to excel in their programming careers. Continue practicing and expanding your knowledge, and consider signing up for a bootcamp if you're eager to elevate your C++ skills!
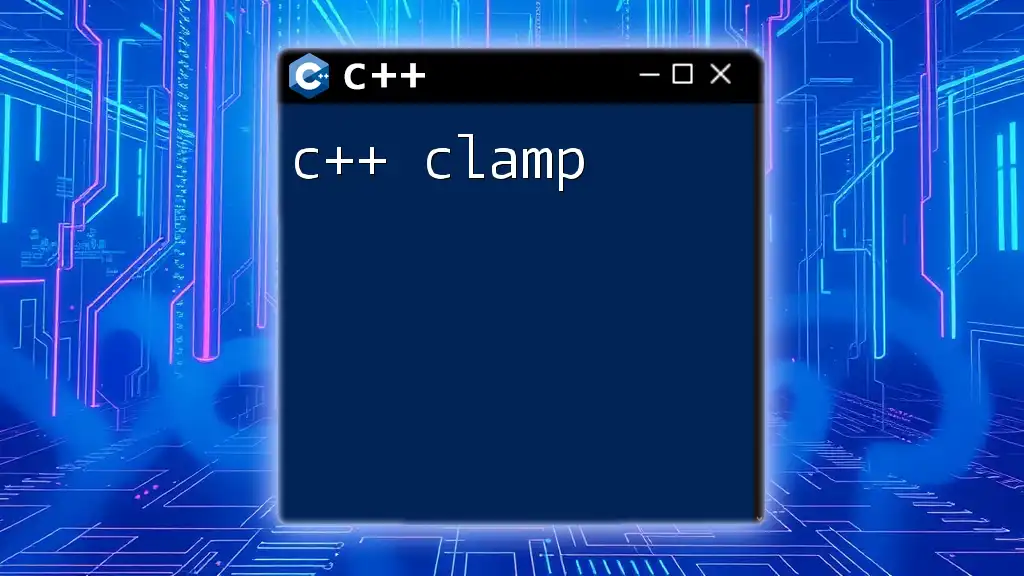
Additional Resources
Further Learning
To continue your C++ journey after a bootcamp, consider exploring recommended books, online courses, and tutorials specific to your learning needs.
Online Communities
Joining online communities can provide ongoing support and motivation. Platforms like Stack Overflow and dedicated Reddit groups can offer invaluable help as you explore advanced C++ concepts and tackle new challenges.