The C++ `clamp` function restricts a value to be within a specified range by returning the lower bound if the value is less than it, the upper bound if it's greater, or the value itself if it's within the range.
Here's a code snippet demonstrating the usage of `clamp`:
#include <algorithm> // for std::clamp
#include <iostream>
int main() {
int value = 15;
int lower_bound = 10;
int upper_bound = 20;
int clamped_value = std::clamp(value, lower_bound, upper_bound);
std::cout << "Clamped Value: " << clamped_value << std::endl; // Output: Clamped Value: 15
return 0;
}
Introduction to `std::clamp`
What is `std::clamp`?
`std::clamp` is a powerful utility function introduced in C++17 that allows you to restrict a value within a specified range. By taking three parameters—the value to clamp, the lower bound, and the upper bound—`std::clamp` returns the value if it lies between the two bounds or the nearest bound if it's outside that range. This aspect of C++ clamp is invaluable for maintaining predictable and stable behavior in your code.
Importance of Clamping Values
In many programming scenarios, you might need to ensure that a variable does not exceed certain limits. This is especially true in graphics programming, game development, or any calculation that involves user input. Clamping helps avoid undesirable behavior such as values going out of range, which could lead to crashes or unexpected results.
For example, in a graphics application, clamping color values between 0 and 255 prevents rendering issues when a user sets colors through sliders.
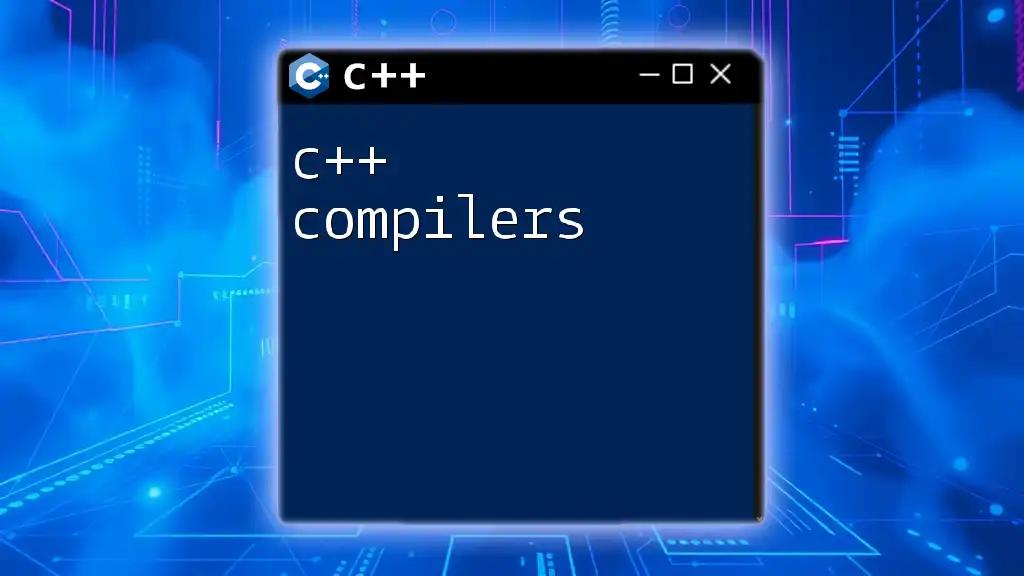
Understanding the Syntax
Basic Syntax of `std::clamp`
The syntax of `std::clamp` is straightforward, allowing easy comprehension:
template< class T >
const T& clamp(const T& v, const T& lo, const T& hi);
- Parameters:
- `v`: The value to be clamped.
- `lo`: The lower bound.
- `hi`: The upper bound.
This function will return:
- `lo` if `v < lo`
- `hi` if `v > hi`
- `v` if `lo <= v <= hi`
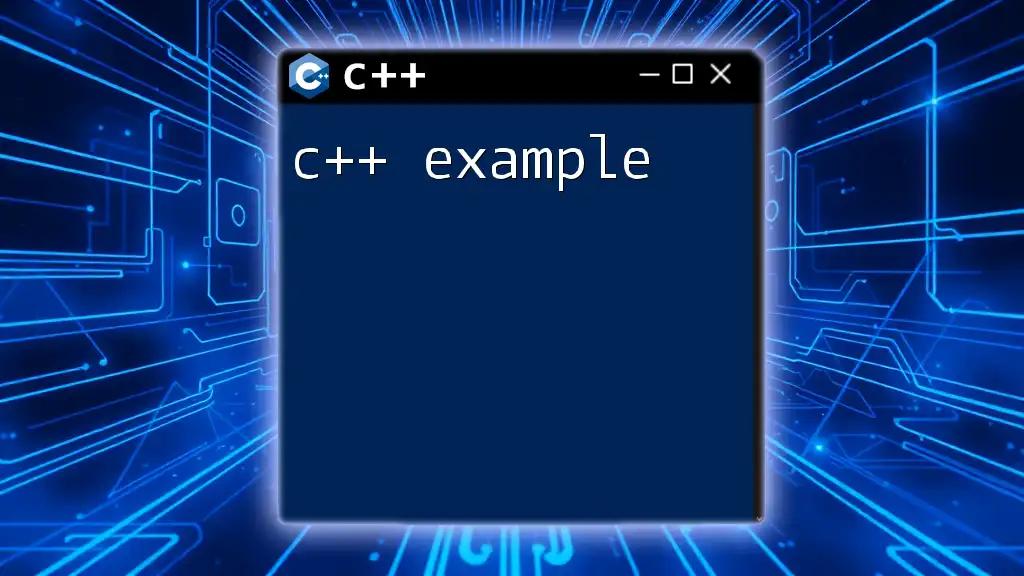
How to Use `std::clamp`
Basic Use Case
Here is a simple example that illustrates the basic usage of C++ clamp with an integer value:
#include <iostream>
#include <algorithm>
int main() {
int value = 15;
int clampedValue = std::clamp(value, 10, 20);
std::cout << "Clamped Value: " << clampedValue << std::endl; // Outputs 15
return 0;
}
In this example, since `15` falls between `10` and `20`, the output will simply be `15`. It's clean, concise, and eliminates the need for multiple conditional statements.
Using `std::clamp` with Different Data Types
`std::clamp` is versatile and can work with various data types, including float values.
float valueFloat = 3.14f;
float clampedFloat = std::clamp(valueFloat, 2.0f, 5.0f);
std::cout << "Clamped Float Value: " << clampedFloat << std::endl; // Outputs 3.14
This example illustrates clamping a floating-point number. By using `std::clamp`, you can ensure that values are kept within specified limits regardless of the data type being used.
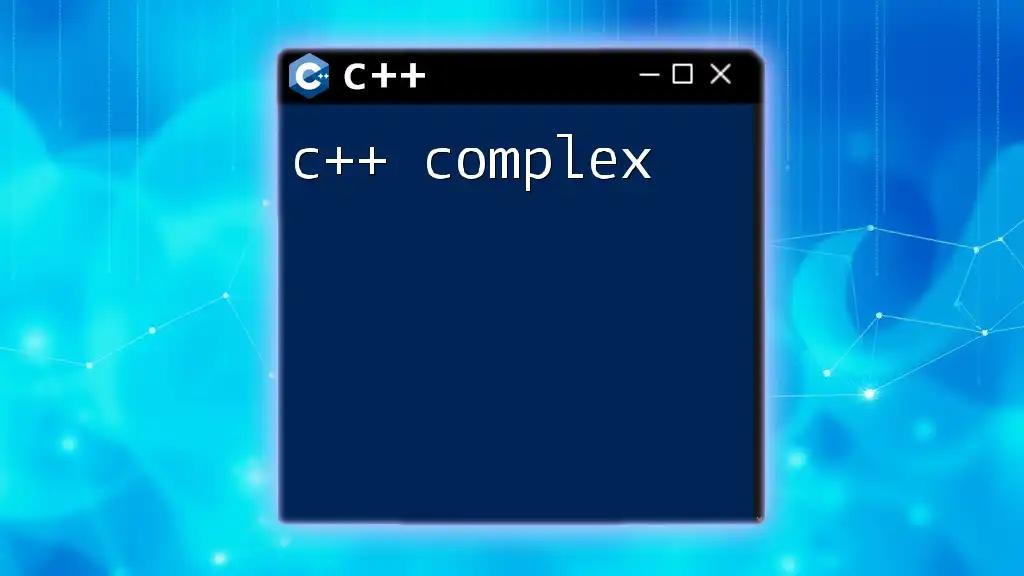
Advanced Usage
Clamping Objects and Structures
You can also use `std::clamp` with user-defined types. However, you'll need to ensure that the type supports comparison operators. Here’s an example with a simple structure:
struct Point {
int x, y;
};
bool operator<(const Point& a, const Point& b) {
return a.x < b.x || (a.x == b.x && a.y < b.y);
}
bool operator>(const Point& a, const Point& b) {
return a.x > b.x || (a.x == b.x && a.y > b.y);
}
Here, the comparison operators allow the `Point` structure to be clamped, as seen in this subsequent application:
Point p = {5, 10};
Point low = {0, 0};
Point high = {10, 10};
Point clampedPoint = std::clamp(p, low, high);
In this case, the `clampedPoint` would represent a point that stays within a defined rectangular area.
Combining with Other Functions
You can combine `std::clamp` with other STL algorithms for more complex tasks. For instance, when manipulating vectors or collections, clamping can help keep values within limits during transformations.
#include <vector>
#include <algorithm>
std::vector<int> numbers = {1, 5, 20, 40, 60};
std::transform(numbers.begin(), numbers.end(), numbers.begin(),
[](int n) { return std::clamp(n, 10, 30); });
This snippet shows how to use `std::clamp` in conjunction with `std::transform` to iterate through a vector of numbers and clamp each value to remain between `10` and `30`. Such combinations showcase the flexibility and utility of C++ clamp in various situations.
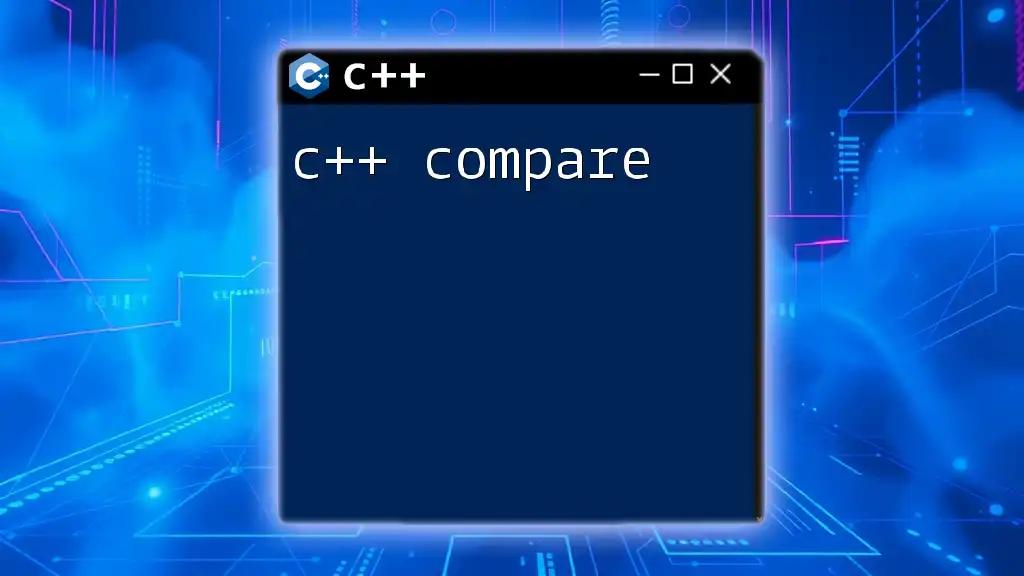
Performance Considerations
Efficiency of `std::clamp`
Using `std::clamp` is not only straightforward but also often more efficient than manual clamping via conditional statements. The function is designed with performance in mind; thus, it involves less boilerplate code and generally leads to better optimization by the compiler.
Consider the manual clamping approach here:
if (value < low) return low;
if (value > high) return high;
return value;
This manual method can increase cognitive load and is more prone to human error. In contrast, using `std::clamp`, the code becomes cleaner and easier to read, which is a significant advantage in larger codebases.
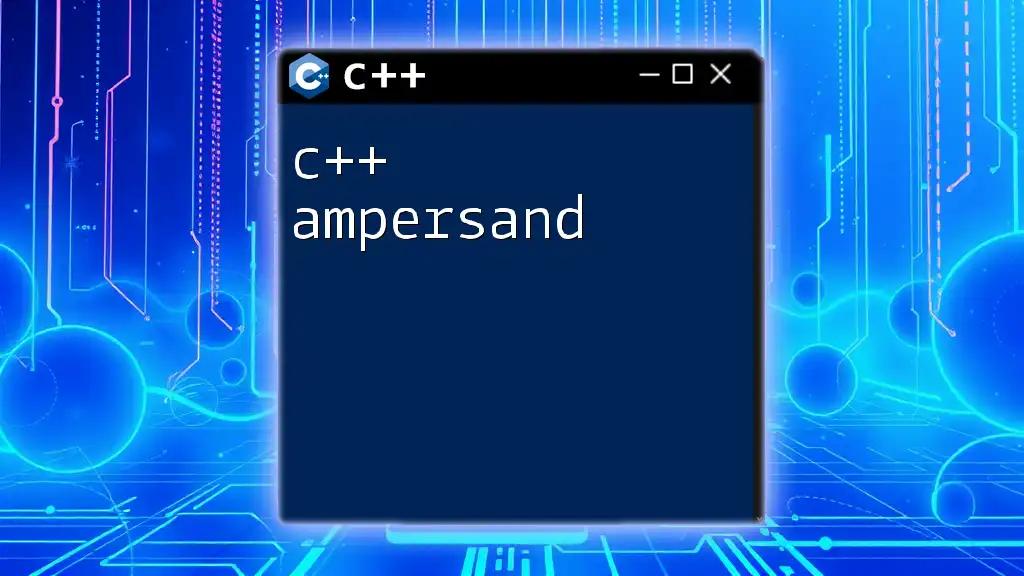
Common Pitfalls
Data Type Issues
One common pitfall when using `std::clamp` is related to mismatched types. Ensure that the value and bounds are of the same type or compatible types; otherwise, you may encounter compilation errors or unexpected results.
Incorrect Boundaries
Another common mistake is incorrectly setting the lower and upper bounds. If the `lo` parameter is greater than `hi`, the results will be incomprehensible. Always validate that your bounds are set correctly before calling `std::clamp`.
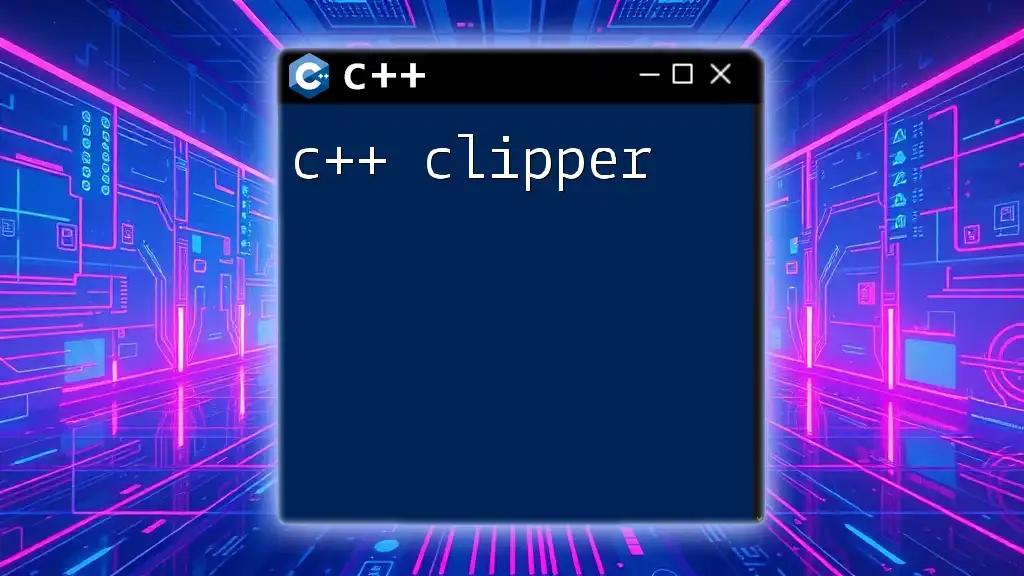
Conclusion
In summary, the utility of `std::clamp` in C++ cannot be overstated. By offering an elegant way to confine values within specified limits, it enhances code readability and maintainability. Make it a habit to utilize this feature in your programming toolkit, and you'll find yourself writing safer and cleaner code.
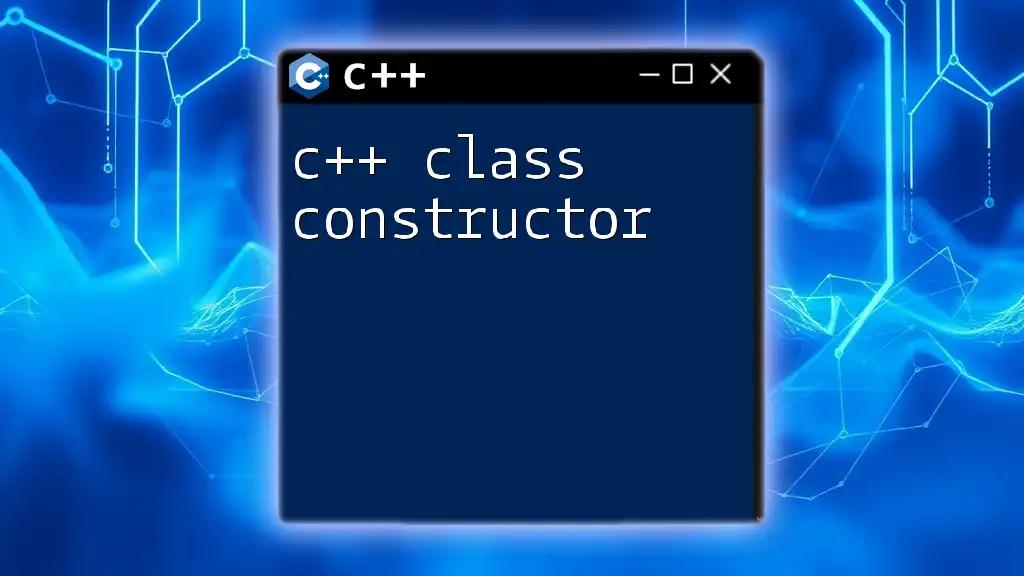
Additional Resources
To deepen your understanding of the `std::clamp`, consider referring to further readings such as official C++ documentation and programming forums where you can engage with fellow developers and share knowledge on C++ best practices.