Compiling C++ code transforms your written code into an executable program using a compiler, such as g++, which can be done with a simple command in the terminal.
Here's a basic example of how to compile a C++ file named `example.cpp`:
g++ example.cpp -o example
Understanding Compilation in C++
What is Compilation?
Compilation is a crucial process in programming that transforms your human-readable C++ code into machine-readable instructions. This process is essential because computers can only understand binary code. Compiling C++ code ensures that your program can execute the functionality you’ve programmed.
The C++ Compilation Process
The compilation of C++ code involves several steps, each serving a unique purpose:
-
Preprocessing: During this phase, the preprocessor takes care of directives (like `#include` and `#define`). It prepares the source code before actual compilation starts.
-
Compiling: The compiler translates the preprocessed code into an intermediate object code. Here, syntax checks are performed to catch errors.
-
Assembling: The assembler converts the object code into machine code, creating an object file (`.o` or `.obj`).
-
Linking: Finally, the linker combines multiple object files and resolves external references to produce the final executable program.
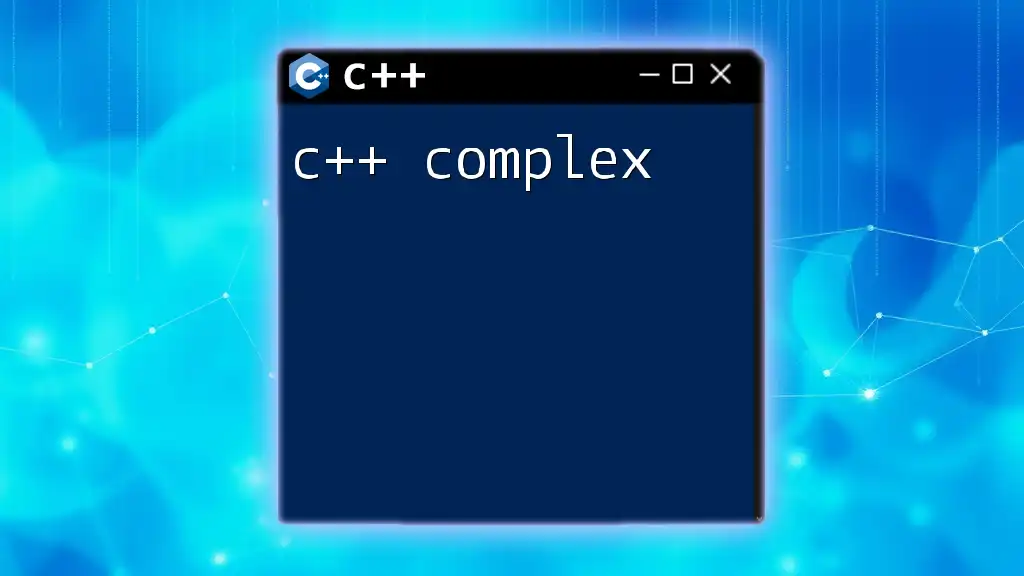
Setting Up Your C++ Development Environment
Choosing a C++ Compiler
Selecting the right C++ compiler is vital for any developer. Here are a few popular options:
- GCC (GNU Compiler Collection): An open-source compiler widely used in Linux environments. It supports multiple programming languages, which makes it versatile.
- Clang: Known for its fast compilation and excellent diagnostics. It's user-friendly and is also open-source.
- MSVC (Microsoft Visual C++): A favored option among Windows developers. It integrates well with Visual Studio, making it robust for Windows application development.
Each compiler has its strengths and weaknesses—GCC is widely recognized for reliability, Clang for speed and error messages, and MSVC for a strong IDE experience. Choose one that best suits your development needs.
Installing a C++ Compiler
Here’s a quick guide to installing GCC, a widely used C++ compiler.
For Windows:
- You can use MinGW. Download the installer and follow the prompts, ensuring you select the C++ compiler during the installation.
For macOS:
- Install Xcode and its command-line tools. Open terminal and execute:
xcode-select --install
For Linux:
- In most Linux distributions, you can install GCC through the package manager. For Debian-based systems, use:
sudo apt install g++
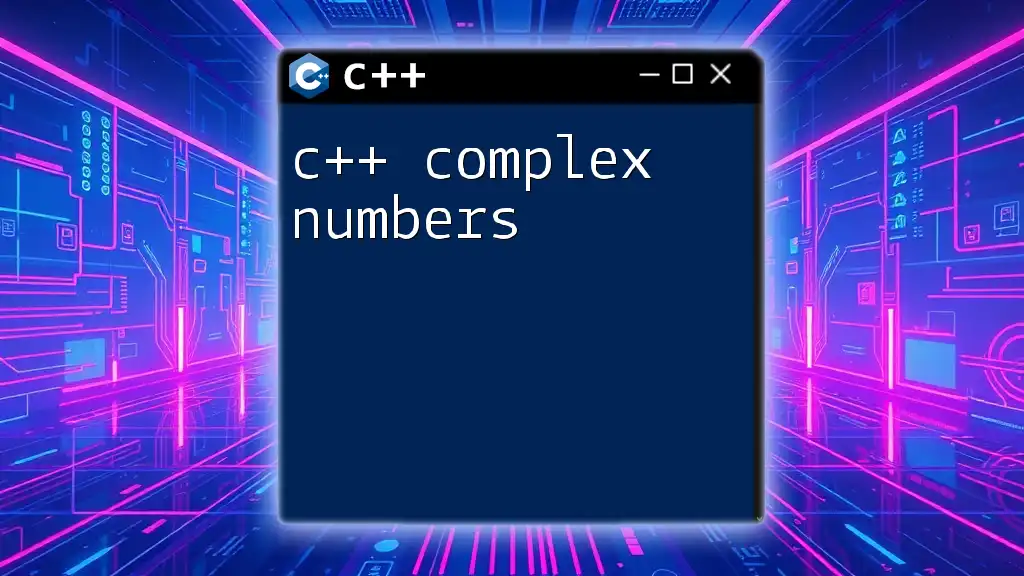
Writing Your First C++ Program
Creating a Simple "Hello, World!" Program
To get started with C++, begin by writing a simple program that prints "Hello, World!" to the console. Here's what it looks like:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- #include <iostream>: This line includes the input-output library, allowing us to use `std::cout`.
- int main(): This defines the main function where program execution starts.
- std::cout << "Hello, World!": This outputs the string to the console.
- return 0;: Signals the program finished successfully.
Saving and Organizing Your Code
When saving your C++ source files, use the `.cpp` extension to clearly identify your files as C++ source code. Additionally, adhere to a consistent naming convention for files (e.g., use lowercase letters and underscores) to enhance organization and avoid confusion.
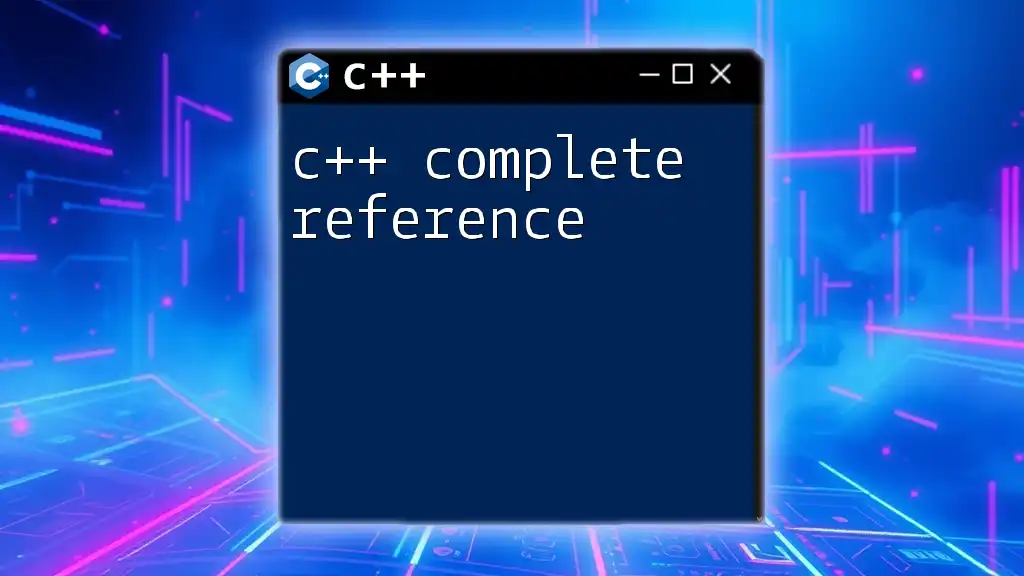
Compiling Your C++ Code
Compiling with Command Line
Now that you have your C++ program ready, it’s time to compile it. Open your terminal or command prompt and navigate to the directory where your `hello.cpp` file is located. Use the following command to compile:
g++ hello.cpp -o hello
This command compiles the `hello.cpp` file and generates an executable named `hello`.
- `g++`: This is the compiler command.
- `hello.cpp`: This is your source file.
- `-o hello`: This specifies the output file name.
Common Compilation Errors
While compiling, you may encounter various errors. Here are a few common ones:
- Syntax Errors: Often caused by typos or incorrect syntax. Read the error message carefully—it usually points to the specific line.
- Undefined References: This happens when a function or variable is declared but not defined. Ensure all declarations have corresponding definitions.
- File Not Found: Ensure that your terminal is pointed to the correct directory where your source files exist.
To troubleshoot these errors, revisit your code and use the messages provided by the compiler as guides.
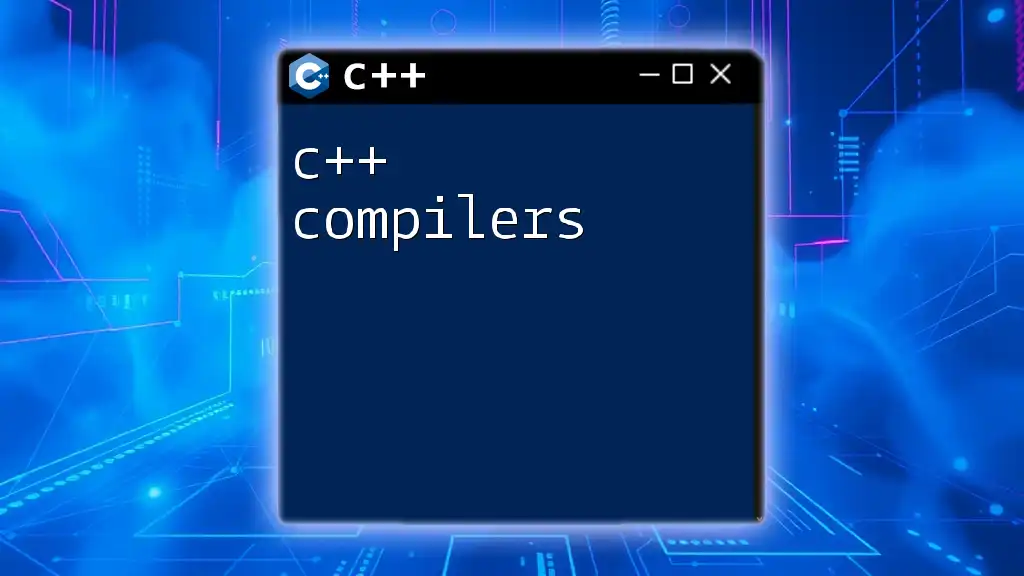
Advanced Compilation Techniques
Using Makefiles
As your project grows, managing compilation manually can become cumbersome. Here, a Makefile comes into play. A Makefile automates the compilation of multiple C++ source files. Here’s a simple example:
all: main
main: main.o utils.o
g++ -o main main.o utils.o
main.o: main.cpp
g++ -c main.cpp
utils.o: utils.cpp
g++ -c utils.cpp
clean:
rm -f *.o main
This Makefile defines how to build the final executable `main` from source files `main.cpp` and `utils.cpp`, while also providing a clean command to remove object files.
Compilation Flags and Options
When compiling C++ code, various flags can enhance functionality:
- -Wall: Enables all warnings. It’s wise to compile your code with this flag to catch potential issues.
- -O2: Activates optimization to produce faster code, although it may increase compile time.
- -g: Generates debugging information, useful for when you need to debug your program.
Using compilation flags can make a significant difference in your development workflow and the performance of your final executable.
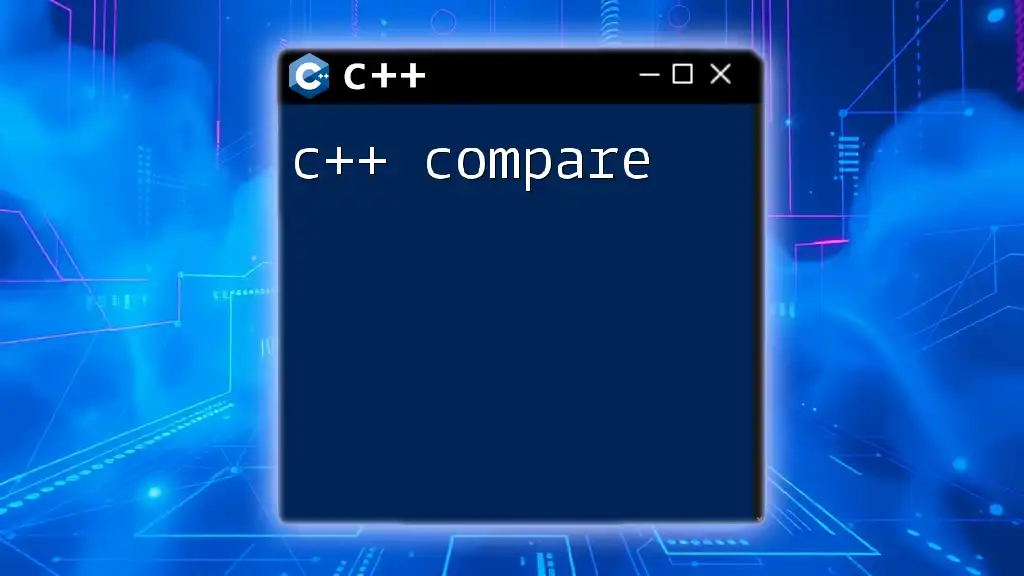
Compiling C++ with IDEs
Using Integrated Development Environments (IDEs)
For many developers, using an IDE to compile C++ code can make the process smoother and more intuitive. IDEs like Visual Studio, Code::Blocks, or CLion provide a user-friendly interface with built-in tools for code editing, compiling, and debugging.
To compile your code in an IDE:
- Create a new project.
- Add your C++ source files within the project.
- Hit the compile button (usually a green play button) to build your project.
Debugging Your Compiled Code
One of the many advantages of using an IDE is its debugging capabilities. You can set breakpoints to pause execution at certain points and inspect variable values. Most IDEs provide step-through functionality, allowing you to execute your code line by line to check for issues. This feature drastically simplifies troubleshooting and enhances code quality.
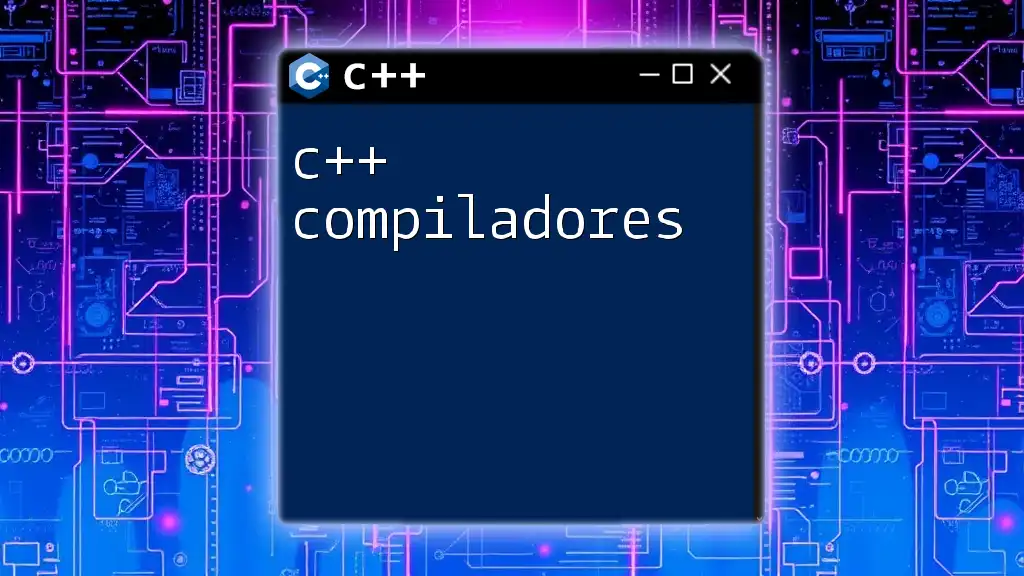
Optimizing Your Compilation Process
Tips for Faster Compilation
To speed up compilation times, consider these techniques:
- Precompiled Headers: Use precompiled headers for frequently used libraries and headers. This resource can save time during the compilation process.
- Incremental Builds: Modify only the files you've changed and let the compiler rebuild only those parts, rather than recompiling the entire project.
Best Practices for C++ Compiling
Adhering to some best practices can help in compiling your C++ code more effectively:
- Organize your project structure logically, grouping related files into folders.
- Use clear and descriptive names for your source files and functions.
- Keep your compilation commands simple, using Makefiles or IDE features to automate repetitive tasks.
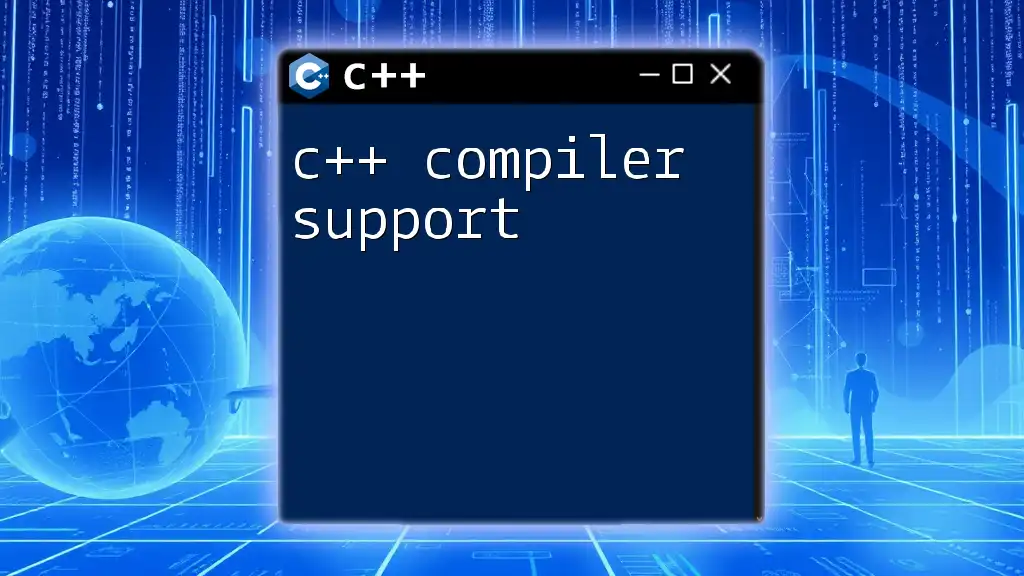
Conclusion
Recap and Next Steps
In this comprehensive guide, we explored the essentials of compiling C++ code—covering everything from installation and writing your first program to advanced techniques like Makefiles and IDE usage.
Further Reading and Resources
For continued learning, consider looking into recommended resources, including books on C++ programming, online tutorials, and community forums where you can seek help and advice. These resources will help you deepen your understanding of C++ and refine your compilation skills as you go along.