Explore practical C++ sample projects that help you grasp essential commands and concepts through hands-on coding experiences.
Here’s a simple C++ program that demonstrates a basic "Hello, World!" output:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Why Create Sample Projects?
Creating C++ sample projects is essential for anyone looking to enhance their programming skills. Engaging in hands-on learning supports theoretical understanding and helps in building practical skills that are crucial in coding. By applying concepts learned in tutorials or textbooks, developers can solidify their knowledge and cultivate their problem-solving abilities.
Selecting the Right Projects
When embarking on C++ projects, it's crucial to select tasks that align with your current skill level. Beginner projects should be straightforward, focusing on core concepts, while advanced projects can delve deeper into algorithms and system design. Consider starting with simple goals and gradually increasing complexity to maintain motivation and growth.
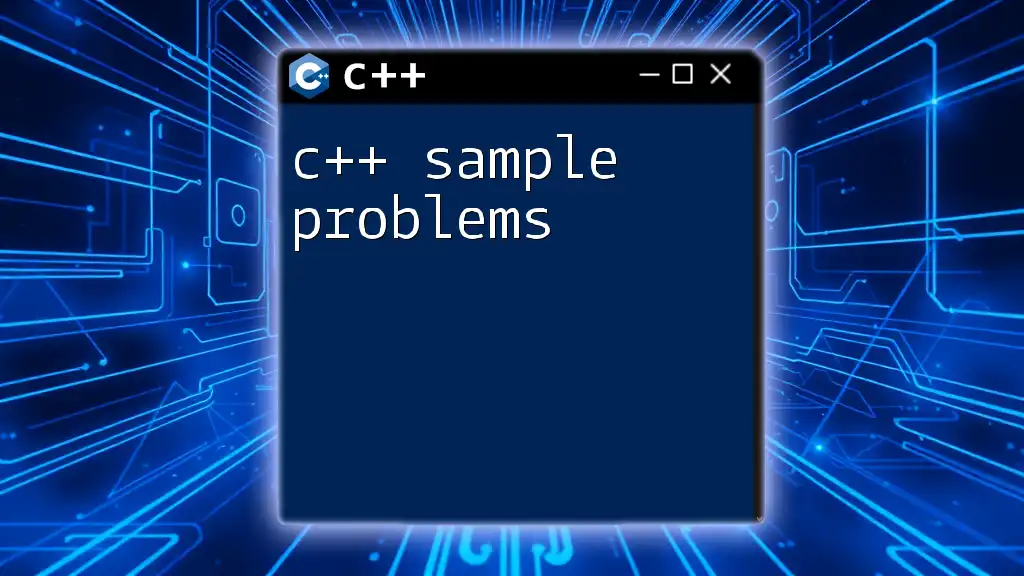
Project Ideas for Beginners
Hello World Program
Every C++ developer's journey begins with the quintessential "Hello, World!" program. This simple script serves as an entry point into the language and showcases fundamental output syntax.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, the program includes the `iostream` library for input and output operations. The `main` function is the starting point, and the `std::cout` statement outputs text to the console. Familiarizing yourself with basic syntax and structure is the first step toward mastering C++.
Simple Calculator
Moving beyond "Hello, World!", a simple calculator project offers a hands-on approach to input and control structures. This project allows learners to grasp the manipulation of variables and basic functions.
#include <iostream>
using namespace std;
int main() {
double num1, num2;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter operator (+, -, *, /): ";
cin >> operation;
cout << "Enter second number: ";
cin >> num2;
switch (operation) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
if (num2 != 0)
cout << num1 / num2;
else
cout << "Division by zero is not allowed.";
break;
default:
cout << "Invalid operator!";
}
return 0;
}
In this code, users input two numbers and choose an operator. The program employs a `switch` statement to perform the requested arithmetic operation. Understanding conditional statements and input handling through this project equips learners with essential C++ tools.
Tic Tac Toe Game
Creating a Tic Tac Toe game further enhances programming skills by introducing data structures and game logic. This project covers arrays, loops, and the use of functions.
#include <iostream>
using namespace std;
char board[3][3] = { {' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '} };
void displayBoard() {
for (int i = 0; i < 3; i++) {
cout << " " << board[i][0] << " | " << board[i][1] << " | " << board[i][2] << endl;
if (i < 2) cout << "---|---|---" << endl;
}
}
// Function to check for a win can be added here
int main() {
displayBoard();
// Additional game logic goes here
return 0;
}
The `board` array represents the game state, while the `displayBoard()` function prints the current state to the console. Expanding this project to include input handling, turn switching, and win-checking logic is a rewarding challenge.
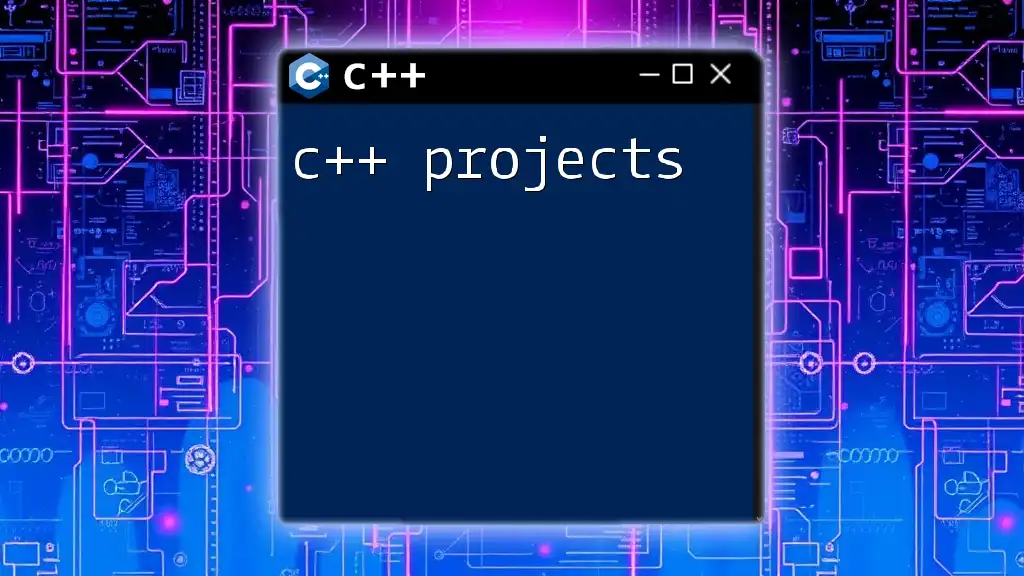
Intermediate Project Ideas
To-Do List Application
This project introduces data structures and file I/O concepts. A To-Do List application facilitates task management and enhances organizational skills. Using structs to define tasks, developers learn to manage collections of data effectively. The final application can save tasks in a file, ensuring they persist across sessions.
Weather Application
Connecting to an API for weather data is a significant step toward understanding network operations in C++. This project demonstrates making HTTP requests and parsing JSON data, which are vital skills for modern application development. Incorporating error handling and data validation will deepen understanding and proficiency.
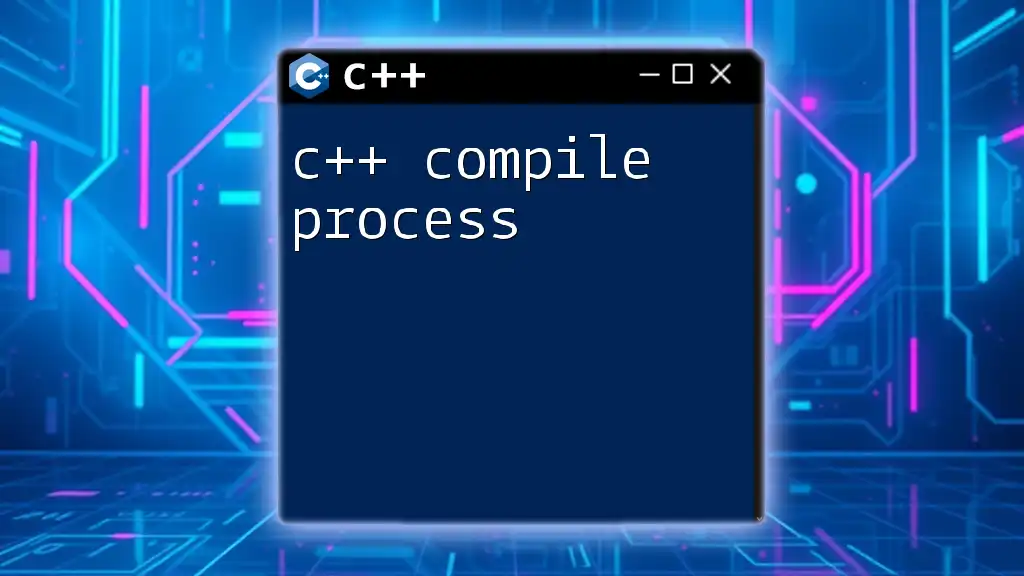
Advanced Project Ideas
Chess Game
Crafting a chess game embodies the art of advanced programming. This project utilizes object-oriented programming (OOP) principles. The use of classes for defining pieces, moves, and board states provides a framework for understanding design patterns. Implementing an AI opponent introduces even deeper complexities, challenging the developer to think critically about algorithms and state management.
Personal Finance Tracker
A personal finance tracker can operate as a robust application that includes database integration and data visualization. By employing SQLite, developers can store and retrieve financial records, allowing users to track their income and expenses efficiently. Introducing graphical representations of data enhances user experience and comprehension, making this project both functional and aesthetically pleasing.
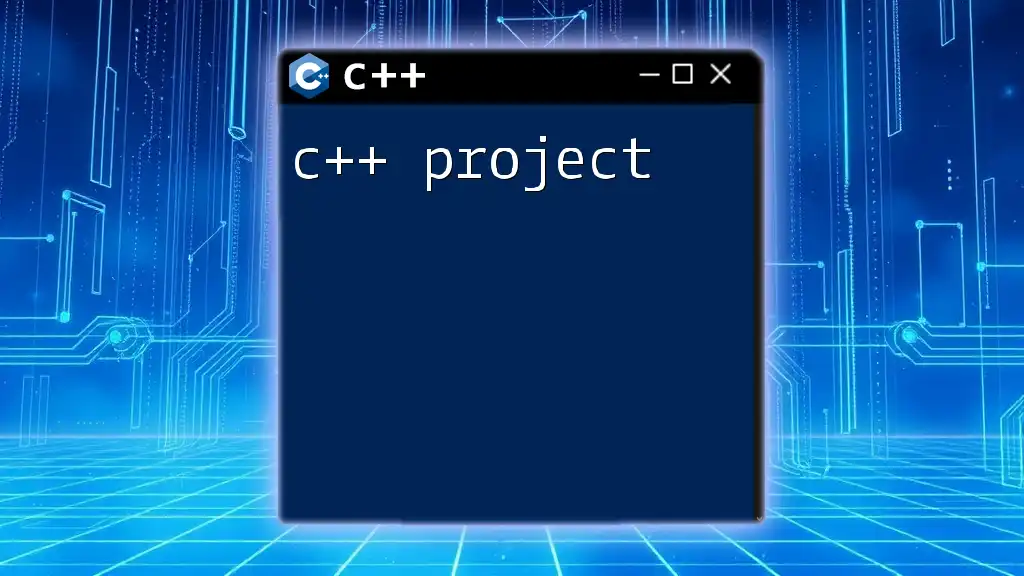
Tips for Success with C++ Projects
Best Practices in Code Writing
Writing clear and maintainable code is paramount for any project. Remember to:
- Comment your code thoroughly to explain why specific decisions were made.
- Use meaningful variable names that convey purpose, enhancing readability.
Frequently Encountered Challenges
As you progress through C++ sample projects, be prepared to face common challenges such as debugging compilation errors, managing memory, and understanding pointers. Seek help from online communities, documentation, and resources to overcome these hurdles.
Resources for Further Learning
To continue your journey in learning C++, explore these resources:
- Online courses and platforms like Codecademy and Coursera
- C++ documentation and forums such as Stack Overflow
- Recommended books like "C++ Primer" by Lippman and "Effective C++" by Scott Meyers
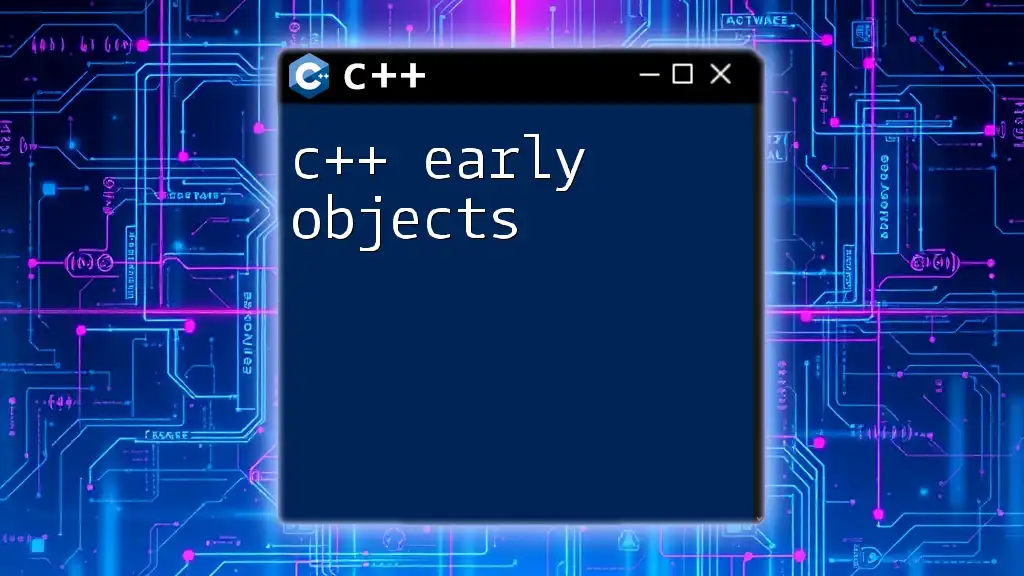
Conclusion
Engaging in C++ sample projects is a transformative experience, allowing developers to bridge the gap between theory and practice. With each project, you'll become more adept at solving real-world problems and refining your skills. Don’t hesitate to start building today; the best way to learn is by doing. Share your projects and experiences as you grow in your journey, and consider what topics you'd like to explore in future discussions. Happy coding!