C++ early objects refer to the use of objects and classes in the initial stages of learning C++, emphasizing practical application through examples.
Here's a simple code snippet demonstrating the definition and usage of a class in C++:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Understanding Early Objects
Definition of Early Objects
In the context of C++ programming, early objects refer to the first exposure a programmer has to the concept of object-oriented programming (OOP) within the language. This foundation lays the groundwork for understanding how classes and objects operate, making it essential for beginners. Early objects contrast with late objects in that they provide immediate utility and accessibility in programming, allowing users to quickly grasp fundamental concepts.
Why Use Early Objects?
Utilizing early objects in your C++ journey comes with numerous benefits:
-
Improved Code Readability: Early exposure to OOP principles promotes a structured way of writing code. By learning to create classes and objects from the beginning, the overall quality and readability of your code improves, making it easier for others (and yourself) to understand later on.
-
Enhanced Maintainability: Object-oriented programming encourages code reuse and modular design, which significantly decreases maintenance costs over time. When a system is designed with clear boundaries between objects, modifications to one object do not necessarily impact others.
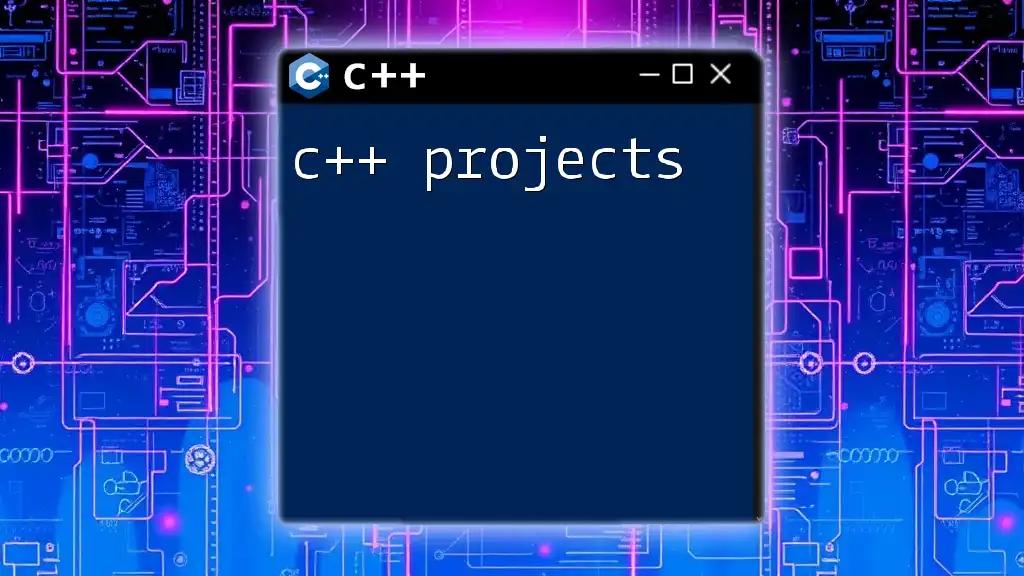
Setting Up Your C++ Environment
Choosing an IDE and Compiler
Before diving into the world of C++ early objects, it’s crucial to set up a comfortable development environment. Popular Integrated Development Environments (IDEs) such as Visual Studio, Code::Blocks, and CLion offer robust features that aid in learning. Each IDE comes with specific installation instructions, but commonly you'll download the executable from the official website, run the installer, and configure it to recognize your desired C++ compiler (e.g., GCC or MSVC).
Compiling Your First C++ Program
To get acquainted with compiling and running a C++ program, it’s beneficial to start with a simple example: the classic "Hello, World!" program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This snippet showcases the structure of a minimal C++ program. Here, `#include <iostream>` includes the input-output stream library, allowing you to use the `std::cout` function to display messages. Compiling this code will give you a solid starting point in understanding the process of transforming source code into executable programs.
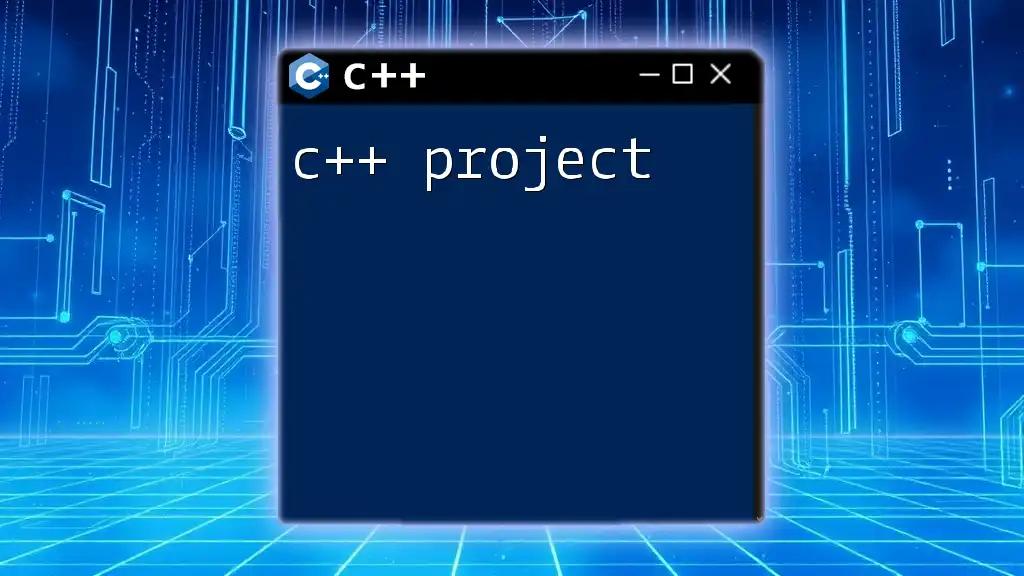
Core Concepts of Early Objects
Classes and Objects
What are Classes?
Classes in C++ serve as blueprints for creating objects, encapsulating both data (attributes) and functions (methods) that operate on that data. The encapsulation provided by classes is a cornerstone of object-oriented programming, promoting better organization and management of code.
Defining a Class
Let's explore how to define a class:
class Car {
public:
std::string brand;
int year;
void displayInfo() {
std::cout << brand << " - " << year << std::endl;
}
};
In this example, we create a `Car` class featuring two public attributes: `brand` and `year`. The class also includes a method called `displayInfo()`, which prints out the car's brand and year. The use of public access modifiers ensures that these attributes and methods are accessible from outside the class.
Creating Objects
Creating an instance of a class, known as an object, is straightforward:
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2020;
myCar.displayInfo();
In this snippet, we instantiate the `Car` object `myCar`, assigning it attributes and invoking `displayInfo()` to display its properties.
Constructors and Destructors
What Are Constructors?
Constructors are special member functions that execute when an object of a class is created. Their primary role is to initialize the attributes of that object. Consider the following example:
class Car {
public:
std::string brand;
int year;
Car(std::string b, int y) {
brand = b;
year = y;
}
};
In this code, the `Car` class features a constructor that takes parameters to initialize the brand and year of the car when an object is instantiated.
Overview of Destructors
Destructors serve the opposite function; they are invoked when an object goes out of scope, allowing for cleanup of resources. Here’s an example:
class Car {
public:
~Car() {
std::cout << "Car object destroyed" << std::endl;
}
};
This destructor simply prints a message when a `Car` object is destroyed, illustrating how destructors can manage resource deallocation.
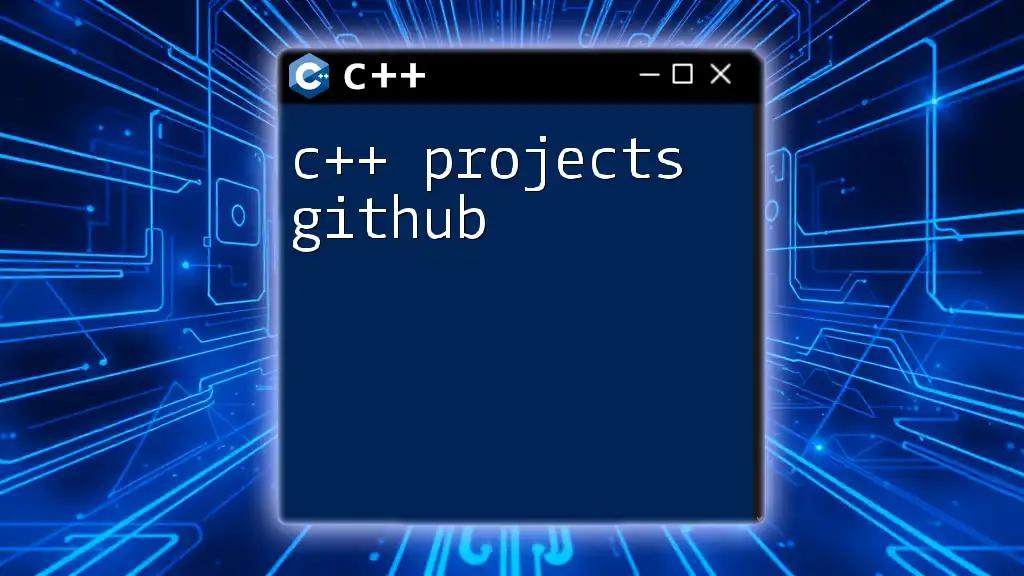
Object Orientation Features in C++
Inheritance
What is Inheritance?
Inheritance provides a mechanism to create a new class from an existing class, allowing the new class (derived class) to inherit attributes and methods from the base class. This promotes code reuse and establishes a hierarchical relationship between classes.
Understanding Base and Derived Classes
Consider the following example that illustrates inheritance:
class Vehicle {
public:
void honk() {
std::cout << "Honk!" << std::endl;
}
};
class Car : public Vehicle {
public:
void display() {
std::cout << "This is a car." << std::endl;
}
};
In this scenario, `Car` inherits from `Vehicle`, gaining access to its `honk()` method. This allows `Car` objects to utilize code from the `Vehicle` class, significantly reducing repetition.
Polymorphism
Concept of Polymorphism
Polymorphism enables objects to be treated as instances of their parent class, with different behaviors based on their runtime types. Polymorphism can manifest in two ways: compile-time (method overloading) and run-time (method overriding).
Example Demonstrating Function Overloading
Function overloading lets you define multiple functions with the same name but different parameters:
void display(int i) {
std::cout << "Integer: " << i << std::endl;
}
void display(double d) {
std::cout << "Double: " << d << std::endl;
}
In this example, the `display()` function can accept either an integer or a double type, showcasing compile-time polymorphism.
Abstraction
What is Abstraction?
Abstraction focuses on exposing only the essential features of an object while hiding unnecessary details. This principle simplifies the interaction with complex systems. Abstract classes, which cannot be instantiated, play a crucial role in achieving abstraction:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
Here, `Shape` serves as an abstract class, enforcing that any derived class must implement the `draw()` method, encapsulating the concept of shape drawing while keeping the implementation details hidden.
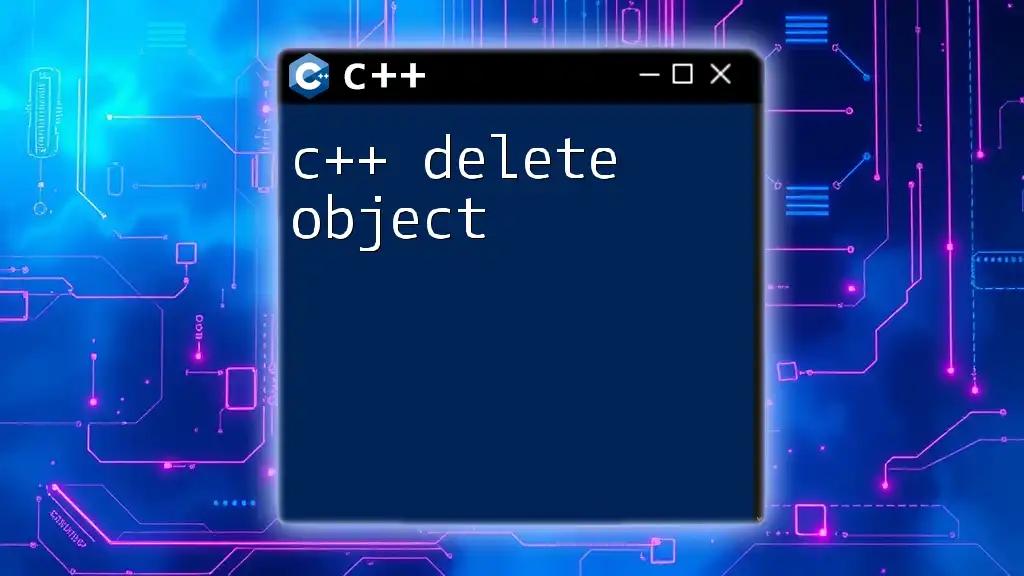
Best Practices in Early Objects
Efficient Class Design
When designing classes, it's essential to follow best practices for effective results. Adhering to the single responsibility principle ensures that a class has only one reason to change, promoting easier maintenance and testing.
Code Readability
Maintaining clear and concise code is critical for collaboration and long-term success. By implementing meaningful naming conventions and adhering to commenting guidelines, you foster a supportive environment for both present and future developers working with your code.
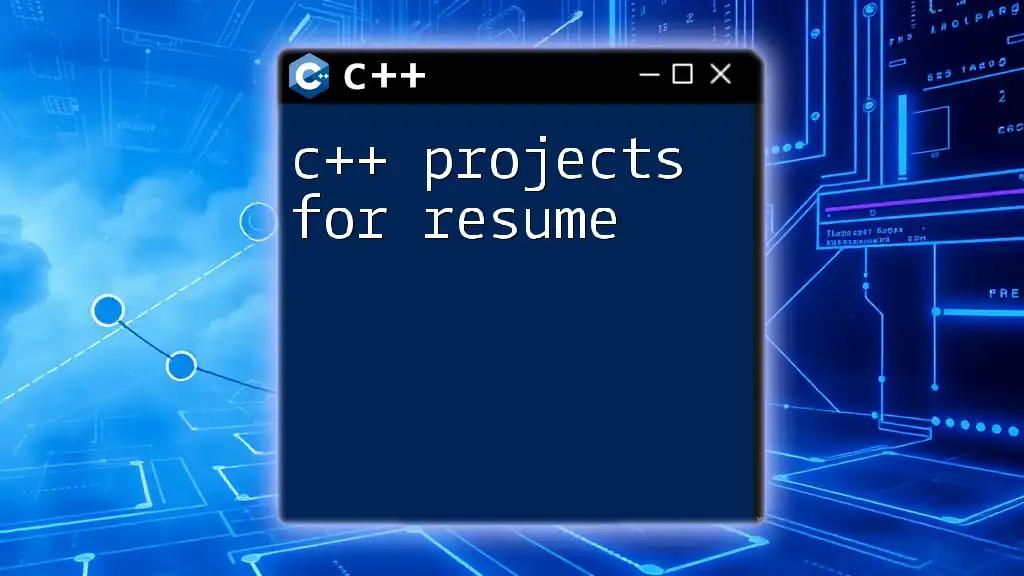
Conclusion
Understanding C++ early objects is fundamental to mastering object-oriented programming in C++. By grasping essential concepts such as classes, constructors, inheritance, and polymorphism, you lay a solid foundation that can be built upon as your knowledge deepens. Embracing these principles will significantly enhance your coding skills, allowing you to approach complex programming tasks with confidence.
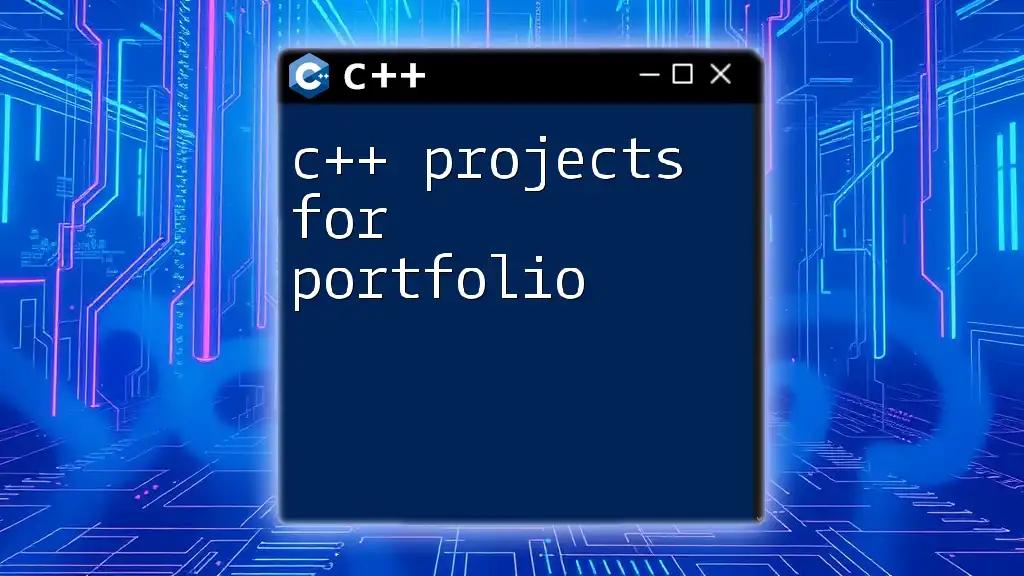
Additional Resources
To further enrich your understanding of C++ early objects, consider exploring recommended books and online courses dedicated to C++ programming. Engaging with communities and forums will also provide invaluable insights and support as you advance your skills.