In C++, the `delete` operator is used to deallocate memory that was previously allocated for an object created with `new`, thereby preventing memory leaks.
Here is a code snippet demonstrating the use of `delete`:
int* ptr = new int(5); // dynamically allocate an integer
delete ptr; // deallocate the memory
ptr = nullptr; // optional: set pointer to nullptr to avoid dangling pointer
Understanding Object Deletion in C++
What is an Object in C++?
In C++, an object is an instance of a class that encapsulates both data and behavior. When you create an object, memory is allocated for it, and it holds its state (attributes) as well as the functions (methods) that operate on that state. The dynamic nature of object creation means one must carefully manage these memory allocations.
Why Do We Need to Delete Objects?
The necessity of object deletion stems from dynamic memory allocation. When an object is created using the `new` operator, it occupies a portion of memory that must be explicitly released back to the system using the `delete` operator. Failing to do so can lead to memory leaks, where memory remains allocated but becomes inaccessible. This not only wastes resources but can eventually lead to performance degradation in long-running applications.
The `delete` Operator in C++
Syntax of `delete`
The `delete` operator is employed to release memory. Its basic use is demonstrated as follows:
- To delete a single object: Use `delete object_pointer;`
- To delete an array of objects: Use `delete[] object_array_pointer;`
Deleting Single Objects
When you allocate an object using `new`, you must ensure to delete it to free up memory. Here is a straightforward illustration of this principle:
class MyClass {
public:
MyClass() { /* constructor code */ }
~MyClass() { /* destructor code */ }
};
int main() {
MyClass* obj = new MyClass(); // dynamic allocation
delete obj; // deleting the object
return 0;
}
In this code snippet, a `MyClass` object is created dynamically. When we call `delete obj;`, the destructor of `MyClass` is invoked, followed by deallocation of the memory occupied by the object. This ensures there is no memory leak.
Deleting Arrays of Objects
Sometimes, you might allocate an array of objects. In such cases, using the correct deletion method is critical. Below is an example:
class MyClass {
public:
MyClass() { /* constructor code */ }
~MyClass() { /* destructor code */ }
};
int main() {
MyClass* objArray = new MyClass[10]; // array allocation
delete[] objArray; // deleting the array of objects
return 0;
}
In this example, `new MyClass[10]` allocates an array of ten `MyClass` objects. To correctly deallocate this memory, `delete[] objArray;` is used. This distinction is vital since using `delete` instead of `delete[]` can lead to undefined behavior, as it may not invoke the destructors of all objects in the array.
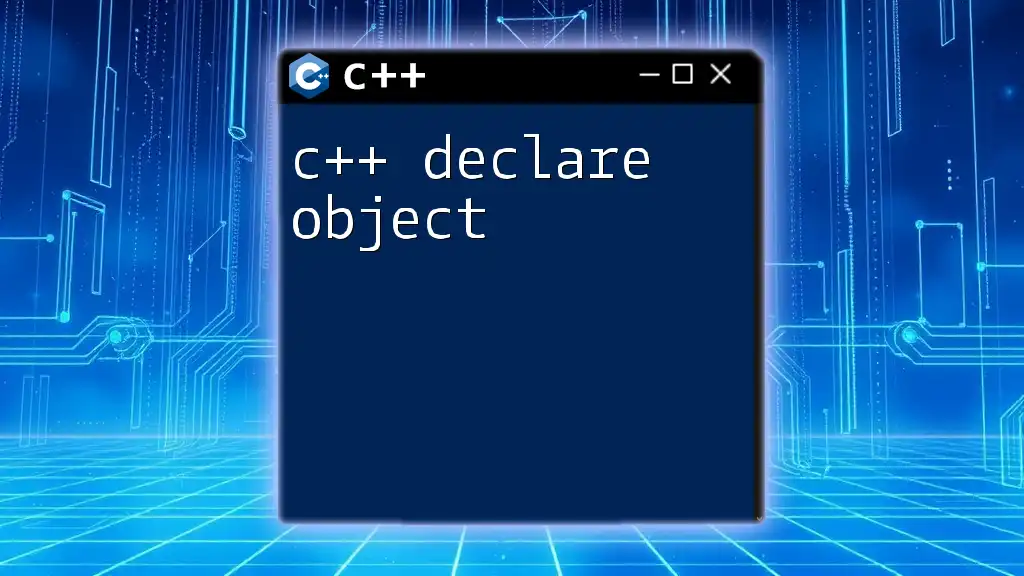
Common Mistakes When Using `delete`
Double Deletion
A common pitfall when managing dynamically allocated objects is double deletion. This occurs when you attempt to delete the same memory address more than once, leading to undefined behavior. For example:
MyClass* obj = new MyClass();
delete obj;
// Attempting to delete again
delete obj; // This will cause issues
To prevent this error, set the pointer to `nullptr` after deletion:
delete obj;
obj = nullptr; // Safeguard against double deletion
Forgetting to Delete
Neglecting to delete dynamically allocated objects can lead to memory leaks. The memory remains occupied for the lifetime of the application, consuming precious resources. This can be addressed effectively by employing smart pointers in modern C++.
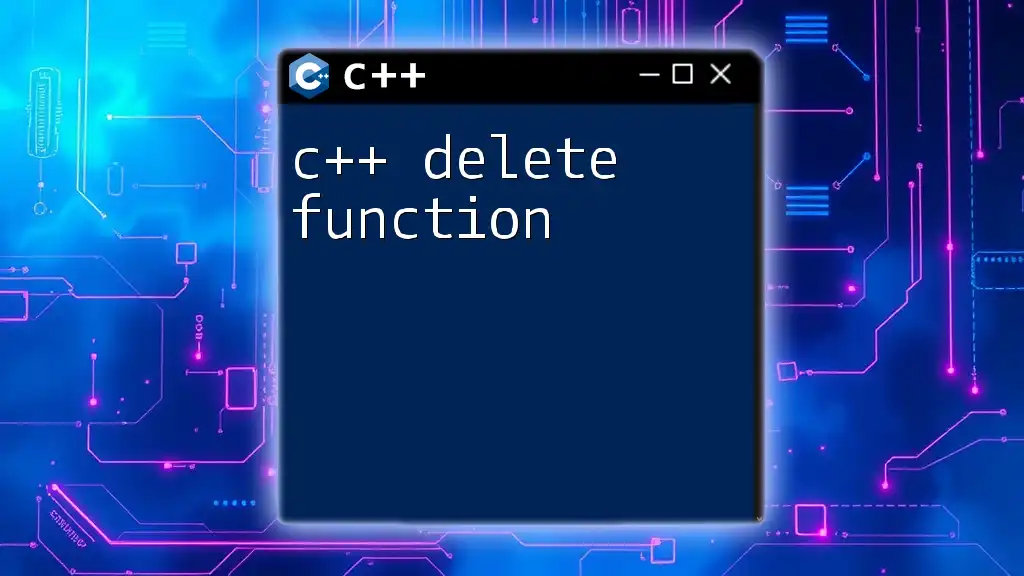
Best Practices for Object Deletion in C++
Using Smart Pointers
Smart pointers are part of the C++11 standard and provide a convenient way to manage memory automatically. They ensure that objects are deleted correctly when they’re no longer needed, drastically reducing the chances of memory leaks.
For instance, using `std::unique_ptr`:
#include <memory>
class MyClass {
public:
MyClass() { /* constructor code */ }
~MyClass() { /* destructor code */ }
};
int main() {
std::unique_ptr<MyClass> obj = std::make_unique<MyClass>(); // automatic memory management
// No need to manually delete
return 0;
}
Here, `std::unique_ptr` automatically deletes the `MyClass` object when it goes out of scope, which alleviates the programmer’s burden of manual memory management.
Resource Management Concepts
The concept of RAII (Resource Acquisition Is Initialization) is central in C++. By tying resource management (like memory allocation) to object lifetime, C++ provides robust mechanisms for ensuring that resources are freed appropriately. This principle underlies the behavior of smart pointers and is foundational for effective memory management in C++.
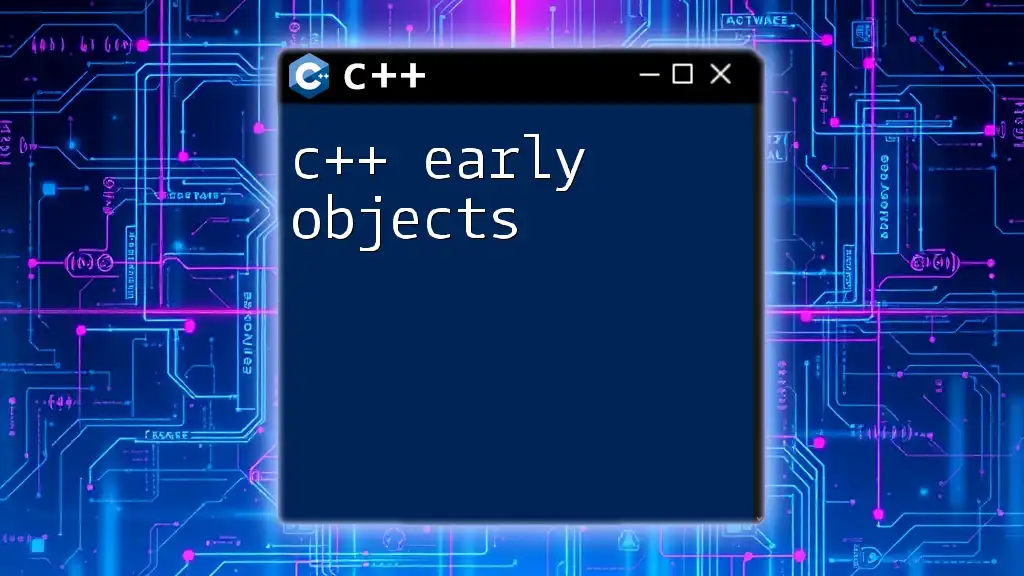
Conclusion
Understanding how to effectively use `c++ delete object` is crucial for any C++ programmer. Properly managing object deletion ensures efficient use of memory and prevents leaks that can lead to performance issues. By adopting modern practices such as smart pointers and embracing RAII, you can streamline memory management in your applications, making your code safer and easier to maintain. Embracing these principles will not only enhance your programming skills but also contribute to writing more efficient software.