In C++, using the `delete` operator on a null pointer is safe and has no effect, as it simply does nothing when the pointer is null.
Here’s a code snippet that demonstrates this:
#include <iostream>
int main() {
int* ptr = nullptr; // Initialize a null pointer
delete ptr; // Safe to delete, does nothing
std::cout << "Pointer deleted safely." << std::endl;
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. Pointers are a powerful feature of C++, allowing for dynamic memory allocation and manipulation of data structures. To declare a pointer, you use the asterisk (`*`) symbol.
Example:
int value = 5;
int* ptr = &value; // ptr now holds the address of value
In this example, `ptr` is a pointer to an integer, and it is initialized to the address of `value`.
The Concept of Null Pointers
A null pointer is a pointer that does not point to any object or valid memory address. It is important to differentiate between null pointers and uninitialized pointers, which may point to random memory locations.
You can create a null pointer in C++ by simply assigning it the value `nullptr` or `NULL`.
Example:
int* nullPtr = nullptr; // nullPtr is now a null pointer
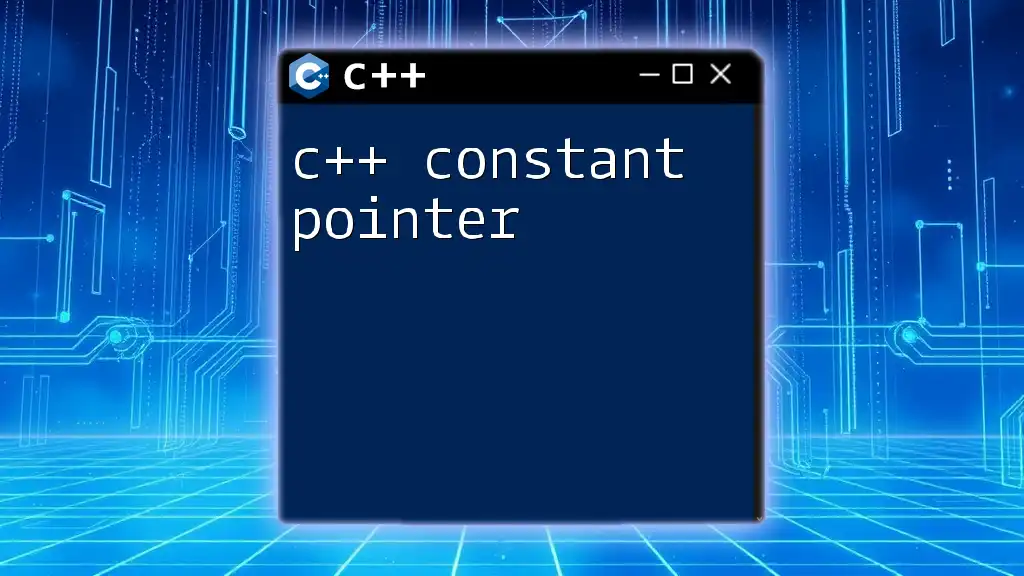
The `delete` Operator in C++
What Does `delete` Do?
The `delete` operator is used in C++ to deallocate memory that was previously allocated with `new`. This is essential for managing memory efficiently and preventing leaks. When you delete a pointer, the memory it points to is freed, and the pointer becomes invalid.
Syntax of `delete`
The general syntax for using `delete` is straightforward:
delete pointerVariable; // Deallocate the memory
Code Snippet:
int* num = new int; // Dynamically allocate memory
*num = 10;
// When done, delete the memory
delete num;
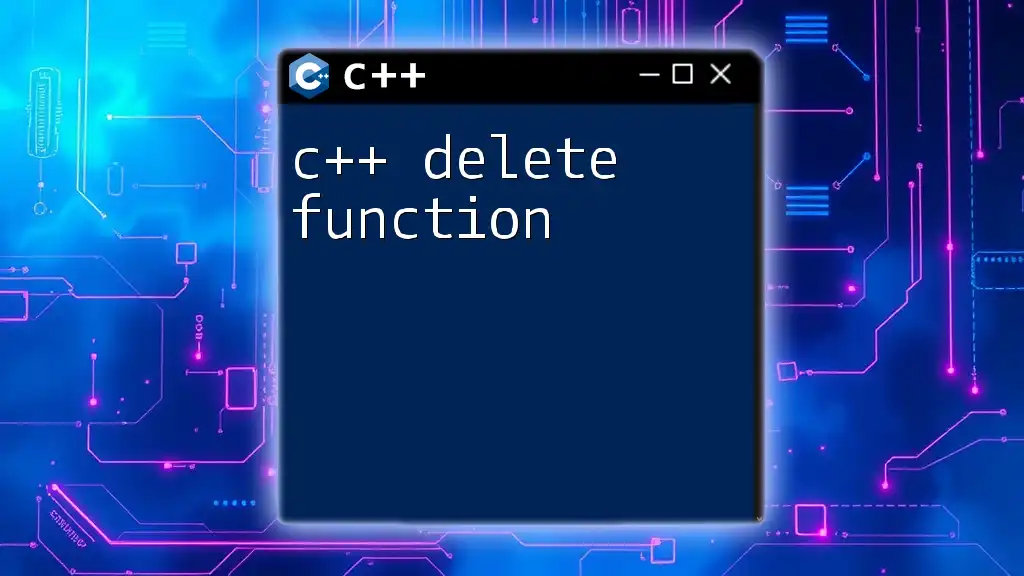
C++ Delete Null Pointer
Can You Delete a Null Pointer?
In C++, if you attempt to delete a pointer that is null (i.e., it holds `nullptr`), it does nothing. This means there are no adverse effects, and it is safe to delete null pointers.
Code Snippet:
int* nullPtr = nullptr;
delete nullPtr; // Safe: does nothing
This behavior eliminates the need for explicit checks before using `delete` on a null pointer, as it guarantees no errors will occur.
Practical Implications of Deleting Null Pointers
The safety of deleting null pointers makes it a common practice in C++ programming. Developers can write cleaner and more concise code without the burden of checking if a pointer is null before calling `delete`.
Example:
int* ptr = nullptr;
// Code logic that may or may not allocate ptr
if ( /* some condition */ ) {
ptr = new int(42);
}
// Safely delete the pointer
delete ptr; // Safe even if ptr is null
C++ Delete nullptr
In C++11 and later, `nullptr` is introduced as a keyword that represents a null pointer type. It is type-safe and eliminates ambiguity.
You can use `nullptr` with `delete` similarly to other null pointers.
Example:
int* ptr = nullptr; // or use int* ptr = nullptr;
delete ptr; // Safe usage
This usage of `nullptr` instead of `NULL` provides better type checking and is recommended for modern C++ development.
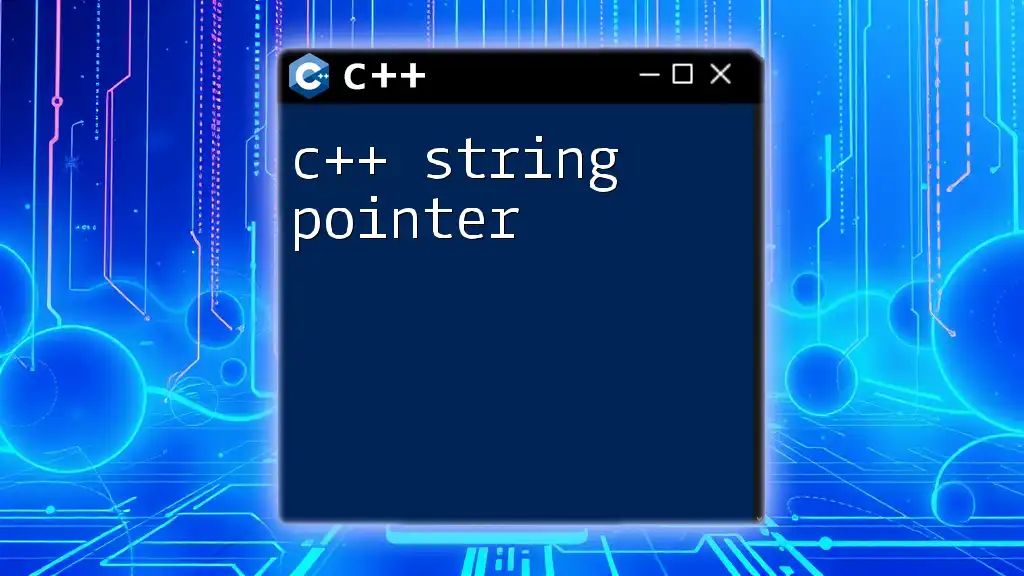
Common Mistakes with Deleting Null Pointers
Attempting to Delete Uninitialized Pointers
One of the most common mistakes among new C++ programmers is attempting to delete uninitialized pointers. This can lead to undefined behavior, crash the program, or corrupt memory.
Example:
int* uninitializedPtr; // Not initialized
delete uninitializedPtr; // Undefined behavior
Misuse of `delete` and Memory Leaks
Failing to delete dynamically allocated memory or attempting to use `delete` multiple times on the same pointer results in memory leaks or program crashes. It’s crucial to only delete memory that has been allocated with `new`.
Code Snippet:
int* data = new int[10]; // Allocating array
delete[] data; // Array must be deleted with delete[]
Using the wrong form of `delete` can lead to memory-related issues in your application.
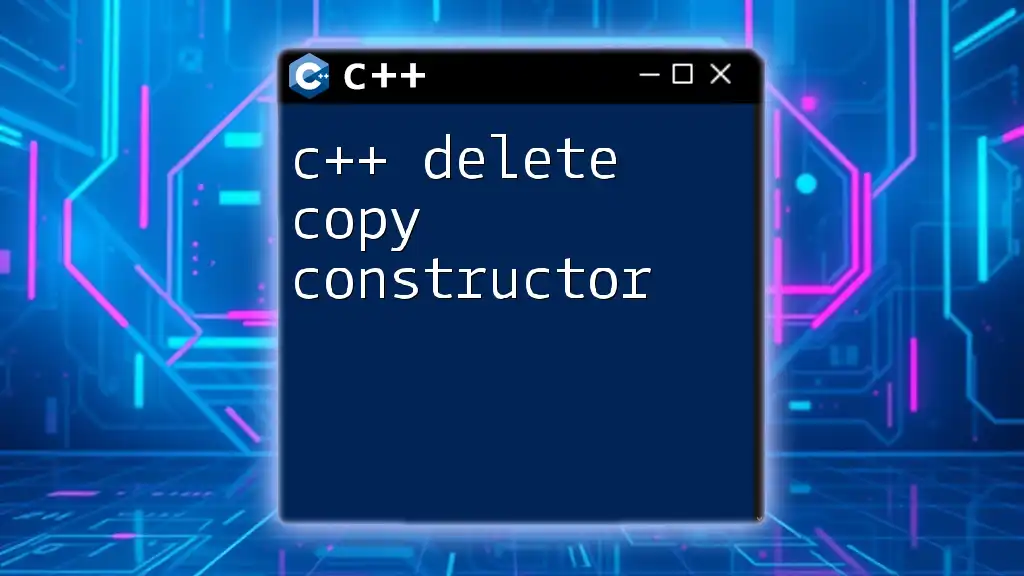
Best Practices for Using `delete` with Null Pointers
Safety Measures
To promote robust memory management, always initialize pointers to `nullptr` when declaring them. This helps prevent errors when attempting to use `delete`.
Utilizing smart pointers such as `std::unique_ptr` and `std::shared_ptr` is a recommended practice in modern C++. Smart pointers automatically manage memory and prevent common memory leaks.
Example:
#include <memory>
std::unique_ptr<int> smartPtr = std::make_unique<int>(10);
// No need to delete; automatically managed
Debugging Memory Management in C++
Debugging memory issues can be complex, but several tools, like Valgrind or AddressSanitizer, can help identify memory leaks and invalid memory access. Understanding the importance of memory management, especially in larger applications, aids in building error-free software.
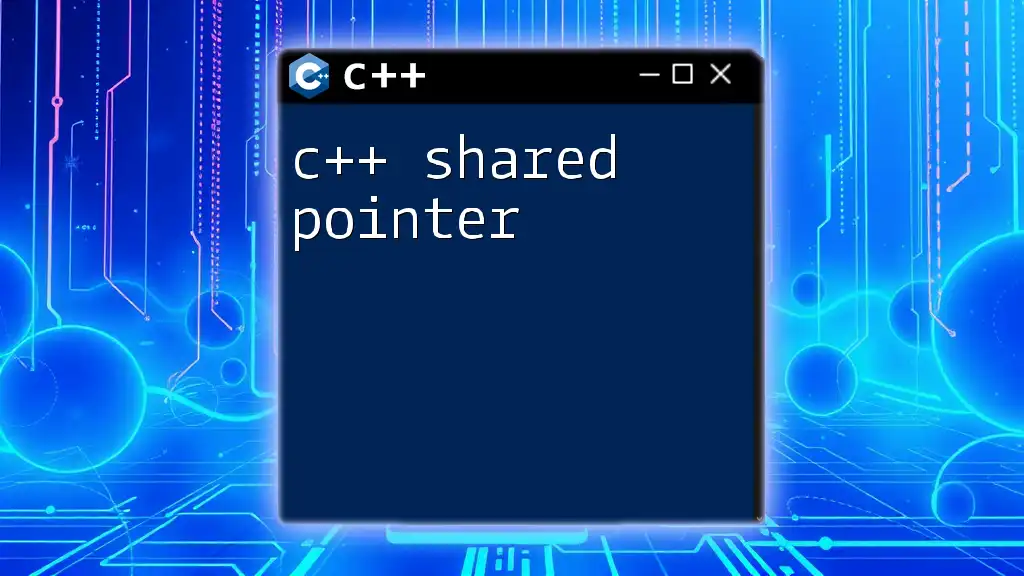
Conclusion
In conclusion, mastering the concept of `c++ delete null pointer` is vital for efficient memory management in C++. Deleting null pointers is safe and encourages cleaner code practices. Emphasizing good memory management practices, such as using smart pointers, enhances program stability and performance. As you continue your journey in C++, remember to explore further resources and refine your skills in handling pointers and dynamic memory allocation.