In C++, a function can return a pointer to a variable, allowing you to access the variable's memory address directly, which can be particularly useful for dynamic memory management.
Here's a brief example:
#include <iostream>
int* returnPointer() {
int* ptr = new int(42); // dynamically allocate an integer and assign it a value
return ptr; // return the pointer to the allocated memory
}
int main() {
int* myPtr = returnPointer();
std::cout << "Value: " << *myPtr << std::endl; // dereference the pointer to get the value
delete myPtr; // free the allocated memory
return 0;
}
What is a Pointer?
In C++, a pointer is a variable that stores the memory address of another variable. Pointers are critical in C++ for various reasons, primarily due to their ability to allow for dynamic memory management and efficient data manipulation. Working with pointers helps developers achieve greater control over memory allocation and deallocation, leading to optimized resource management in applications.
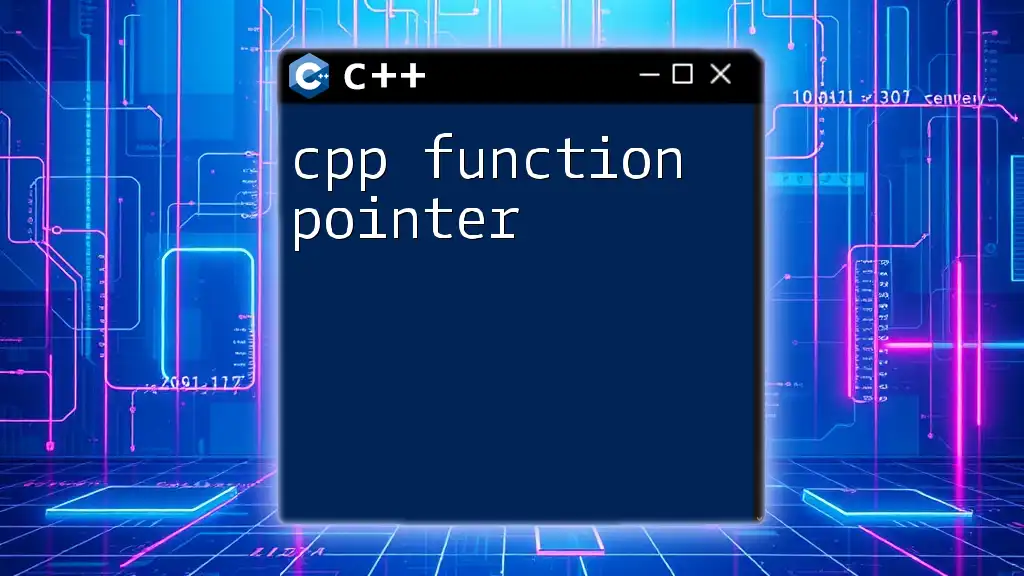
Why Return Pointers?
Returning pointers from functions opens a range of possibilities in programming:
- Dynamic Memory Allocation: When you allocate memory dynamically, it exists until it’s explicitly deallocated. This is crucial in scenarios where you need variable-sized data structures.
- Efficiency: Instead of passing large objects to functions, you can pass their addresses, minimizing memory copying and enhancing performance.
- Shared Access: It allows multiple parts of a program to interact with the same data without making redundant copies.
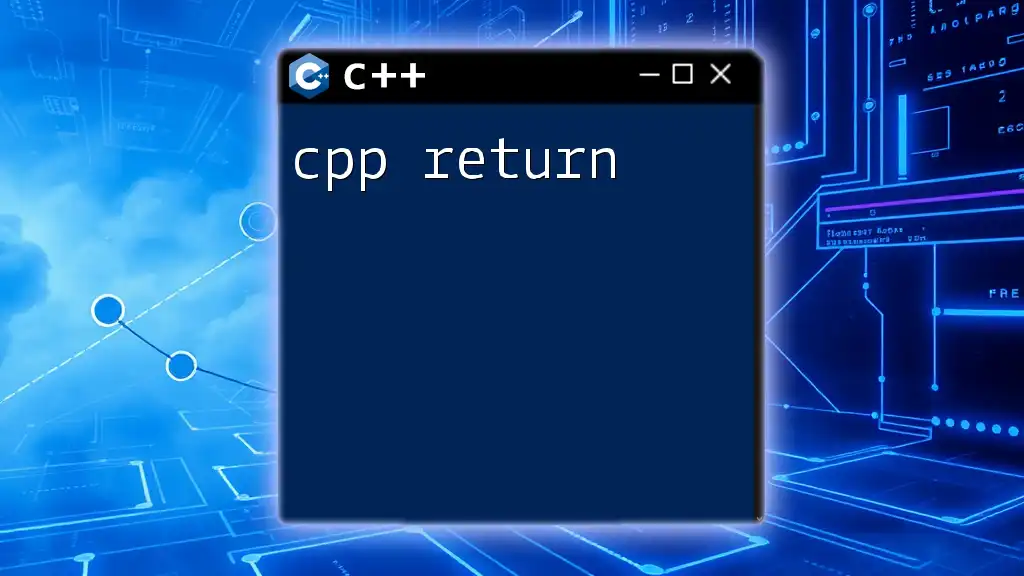
Understanding Pointers in C++
Basic Pointer Syntax
To work with pointers, you must first understand how to declare and initialize them:
-
Declaring Pointers: You declare a pointer by specifying the data type followed by an asterisk:
int* ptr;
-
Initializing Pointers: You initialize a pointer by assigning it the address of a variable:
int variable = 5; ptr = &variable;
Pointer Operations
Pointers come with operations that make them versatile:
-
Dereferencing a Pointer: To access the value at the address pointed to by a pointer, you use the dereference operator (`*`):
int value = *ptr; // Retrieves the value of 'variable'
-
Pointer Arithmetic: You can perform arithmetic operations on pointers, such as incrementing or decrementing the pointer address:
ptr++; // Moves the pointer to the next integer address ptr--; // Moves the pointer to the previous integer address
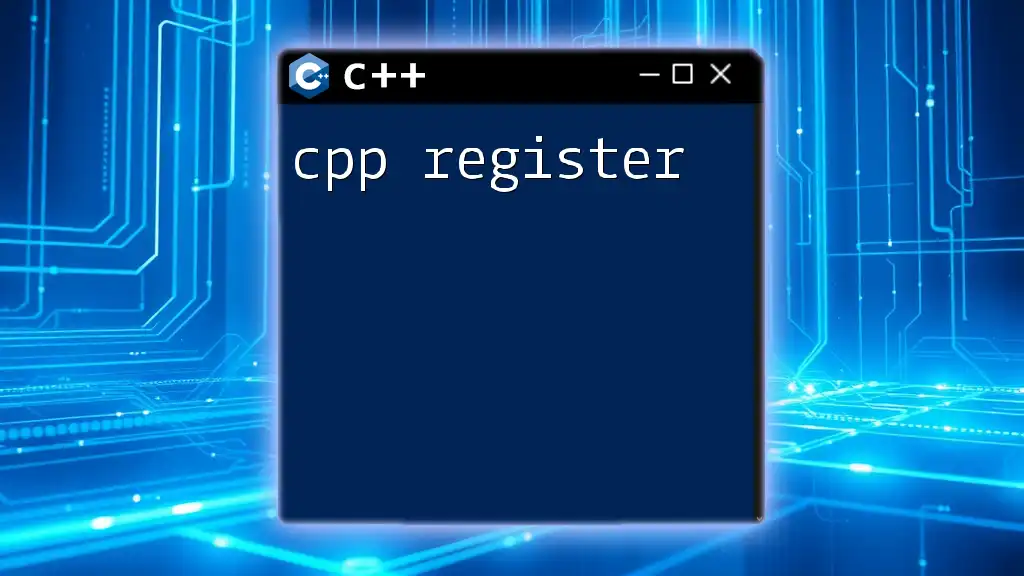
Functions That Return Pointers
Defining Functions to Return Pointers
When creating a function that returns a pointer, the syntax involves specifying the pointer type in the return type:
return_type* function_name(parameters);
Example: Function Returning a Pointer to an Integer
Here’s a simple example of a function that returns a pointer to an integer:
int* createInteger(int value) {
int* ptr = new int(value);
return ptr;
}
In this code detail, the `createInteger` function takes an integer value, dynamically allocates memory for an integer, and then returns a pointer to that allocated memory.
Handling Memory Management
Memory management is crucial when dealing with pointers. Always ensure you deallocate any dynamically allocated memory using the `delete` operator to avoid memory leaks. Here’s a proper cleanup example:
int* intPtr = createInteger(42); // Create an integer with a value of 42
// Use the integer...
delete intPtr; // Proper memory cleanup to avoid memory leak
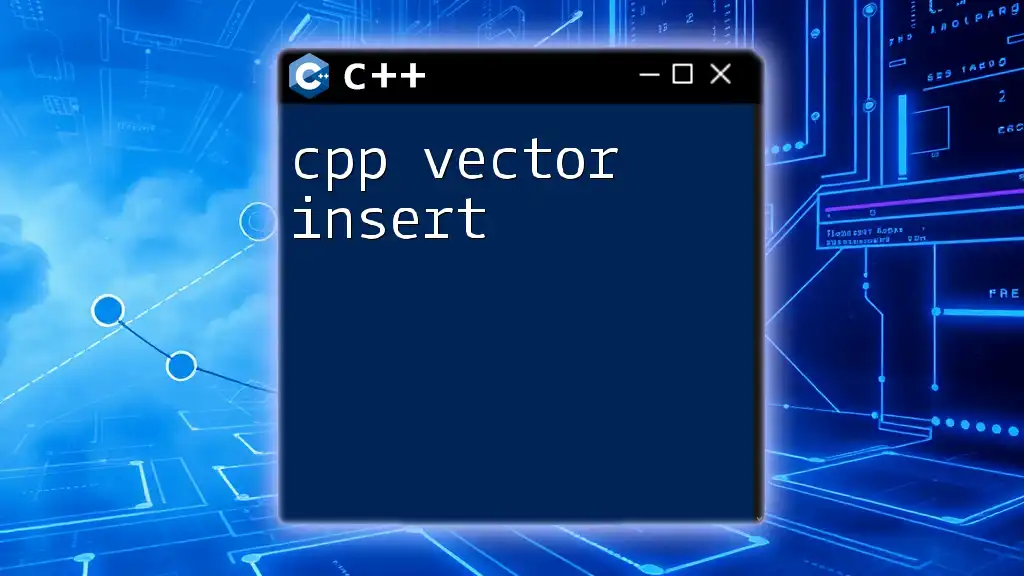
Returning Pointers to Arrays
Function to Return a Pointer to an Array
Returning pointers to arrays follows similar principles. Here’s an example of returning a pointer to a dynamically allocated array:
int* createArray(int size) {
int* arr = new int[size];
return arr;
}
Using the Array Pointer
After receiving the pointer to the array, you can easily work with it, just like you would with any other array:
int* myArray = createArray(5); // Create an array of size 5
for (int i = 0; i < 5; i++) {
myArray[i] = i * 10; // Assign values to the array
}
Remember to deallocate memory after use:
delete[] myArray; // Clean up the array memory
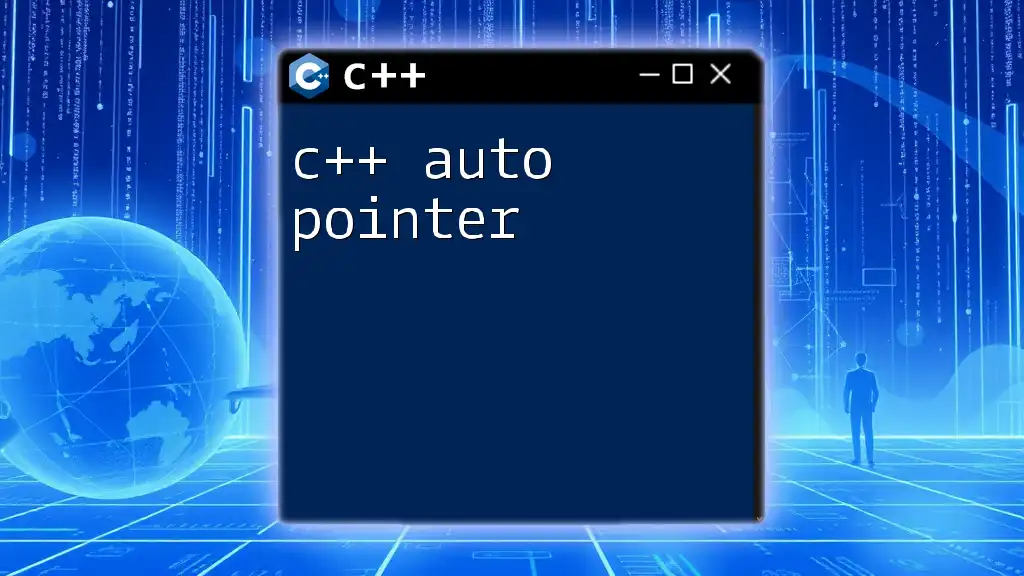
Returning Pointers to Structs
Introduction to Structs
Structs in C++ are user-defined data types that group related variables of different types. Here’s how to create a simple struct:
struct Person {
std::string name;
int age;
};
Function to Return a Pointer to a Struct
You can also create functions that return pointers to structs. Below is an example:
Person* createPerson(const std::string& name, int age) {
Person* p = new Person{name, age}; // Dynamically allocate a struct
return p;
}
Accessing Struct Members
After creating a struct, you can access its members using the `->` operator:
Person* person = createPerson("Alice", 30);
std::cout << person->name << " is " << person->age << " years old."; // Outputs: Alice is 30 years old.
delete person; // Clean up the struct memory
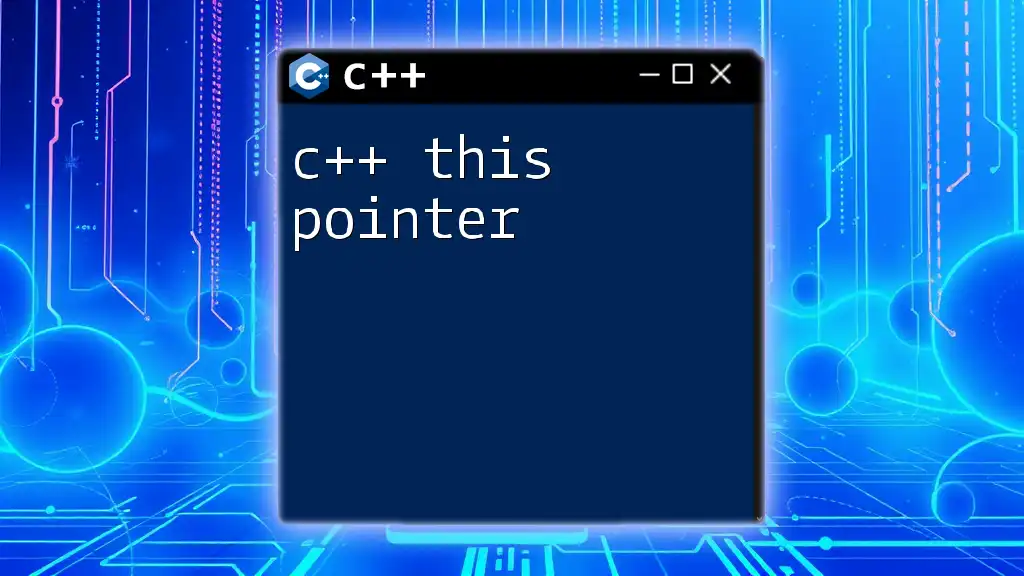
Best Practices When Returning Pointers
Avoiding Dangling Pointers
A dangling pointer occurs when a pointer points to memory that has been deallocated. This can lead to undefined behavior in programs. Avoid dangling pointers by ensuring that pointers don't access freed memory. Always set pointers to `nullptr` after deletion:
delete person;
person = nullptr; // Avoid dangling pointer
Utilizing Smart Pointers
To improve safety and convenience in memory management, consider using smart pointers such as `std::unique_ptr` and `std::shared_ptr`. Smart pointers automatically deallocate the memory they own. Here’s an example using `std::unique_ptr`:
#include <memory>
std::unique_ptr<int> createUniqueInteger(int value) {
return std::make_unique<int>(value); // Automatically handles memory management
}
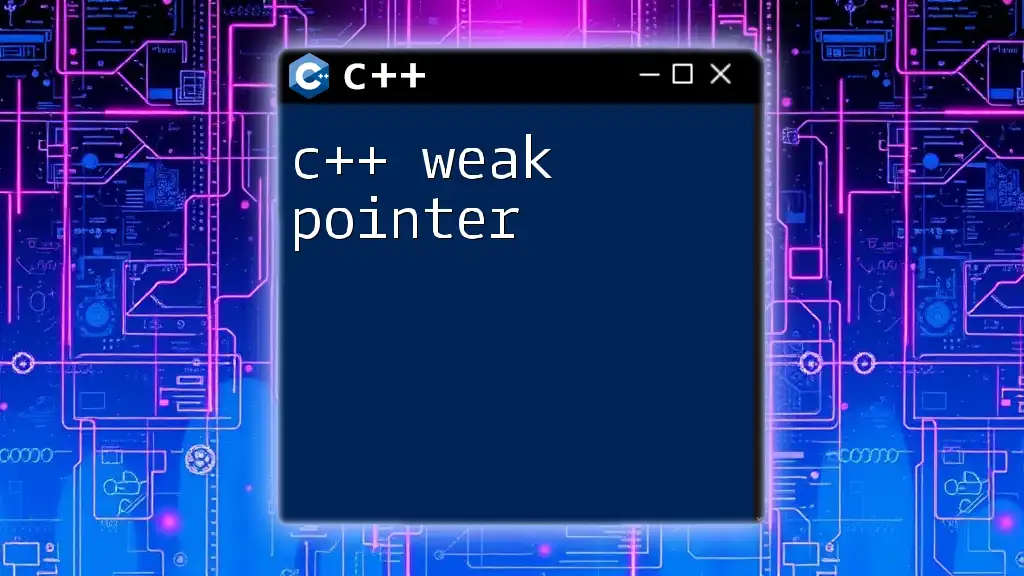
Conclusion
This guide on `cpp return pointer` has covered fundamental concepts of pointers, how to define functions that return pointers, and best practices in managing memory. Understanding pointers enhances your ability to manage resources in your applications effectively.
To deepen your knowledge, practice working with headers, structs, and dynamic memory, as these skills will immensely benefit your programming journey in C++. Happy coding!