The `register` storage class in C++ is used to suggest to the compiler that a variable should be stored in a CPU register for faster access, though the compiler may choose to ignore this suggestion.
Here's a code snippet demonstrating its use:
#include <iostream>
int main() {
register int count = 0; // declare count as a register variable
for (int i = 0; i < 100; ++i) {
count += i;
}
std::cout << "Sum: " << count << std::endl;
return 0;
}
What is the `register` Keyword?
The `register` keyword in C++ is a storage-class specifier primarily intended to suggest that a variable should be stored in a CPU register instead of RAM. Although it provides a hint to the compiler for performance optimization, its actual relevance has diminished with modern compilers’ sophisticated optimization strategies.
Historical Context
Historically, when programmers interacted more directly with hardware, using `register` made sense because it explicitly indicated that a variable was critical for performance in tight loops or frequently accessed data. However, as compilers have become better at optimizing code, their capability to decide the best storage location for variables has surpassed manual hints from programmers.
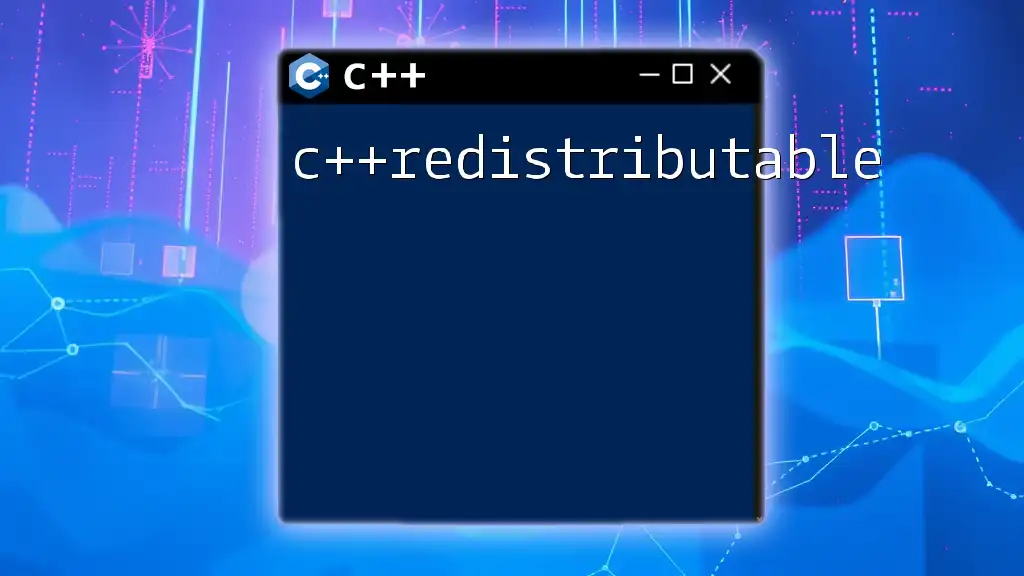
Purpose of Using the `register` Keyword
The primary purpose of the `register` keyword is to optimize the performance of specific variables. When a variable is stored in a register, access time to that variable is reduced, as registers are designed to be the fastest storage locations within a CPU.
Optimization
Variables declared as `register` are intended for fast access. Accessing data stored in a register is significantly quicker than retrieving data stored in main RAM, which is critical for performance-sensitive applications. Although using `register` can improve performance in some situations, it’s worth noting that compilers are very good at optimizing variable storage on their own.
Limitations of `register`
One of the significant misconceptions about `register` is that it guarantees that a variable will be placed in a register. This is not true. Modern compilers often ignore the `register` keyword if they determine that it is not advantageous. Additionally, variables that require a lot of storage cannot be placed in registers.
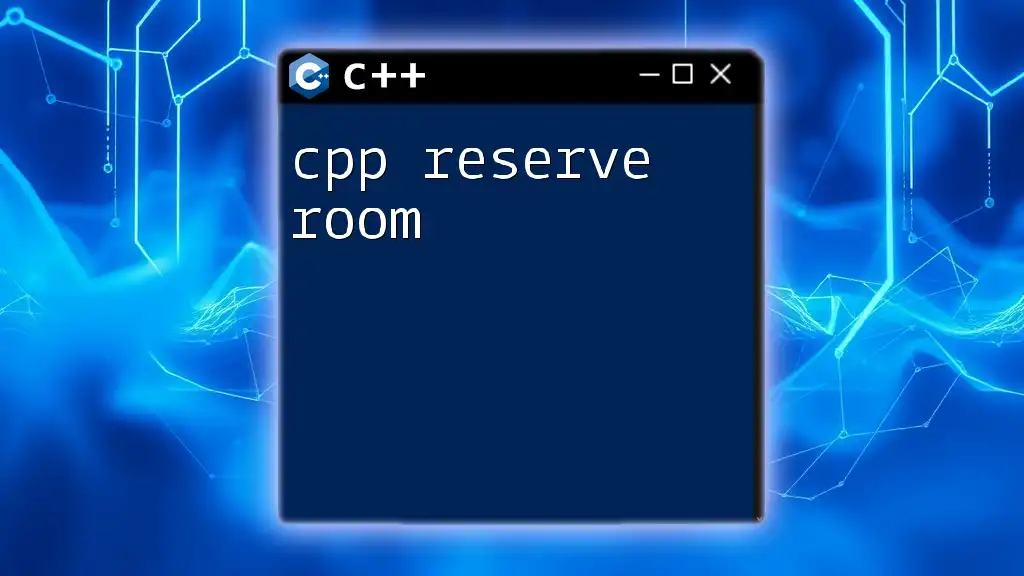
Syntax of `register`
Basic Syntax
The basic way to declare a variable as a register is by using:
register int x;
This line suggests that `x` should ideally be allocated to a register for optimized performance.
Scope and Lifetime
Variables declared with the `register` keyword must have local scope. They cannot be global or static. Also, while the lifetime of a register variable is limited to the block in which it is defined, it cannot be referenced with the address-of operator (`&`) because its address is not meaningful due to its potential register allocation.
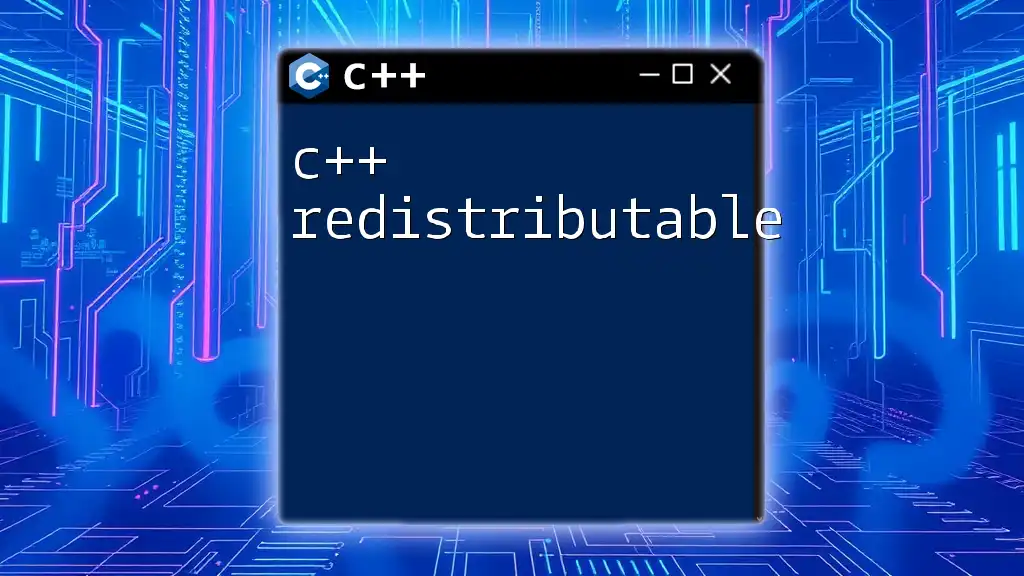
Examples of `register` in C++
Basic Example
Using a `register` variable in a simple function can illustrate its use. Below is an example:
#include <iostream>
using namespace std;
void useRegister() {
register int i; // Suggests the variable 'i' is stored in a register
for (i = 0; i < 10; i++) {
cout << i << " ";
}
}
int main() {
useRegister();
return 0;
}
In this example, variable `i` is a candidate for storage in a register. The loop iterates and outputs numbers from 0 to 9. The compiler may choose to prioritize this variable’s storage for improved performance.
Complex Example
To demonstrate the potential of using multiple `register` variables, consider the following function that sums squares:
#include <iostream>
void complexFunction() {
register int counter;
register float sum = 0.0;
for (counter = 1; counter <= 5; counter++) {
sum += counter * counter; // Calculate sum of squares
}
std::cout << "Sum of squares: " << sum << std::endl;
}
int main() {
complexFunction();
return 0;
}
In this case, while both `counter` and `sum` are suggested as `register`, the performance benefit is often negligible. The compiler is likely to optimize this efficiently without explicit hints.
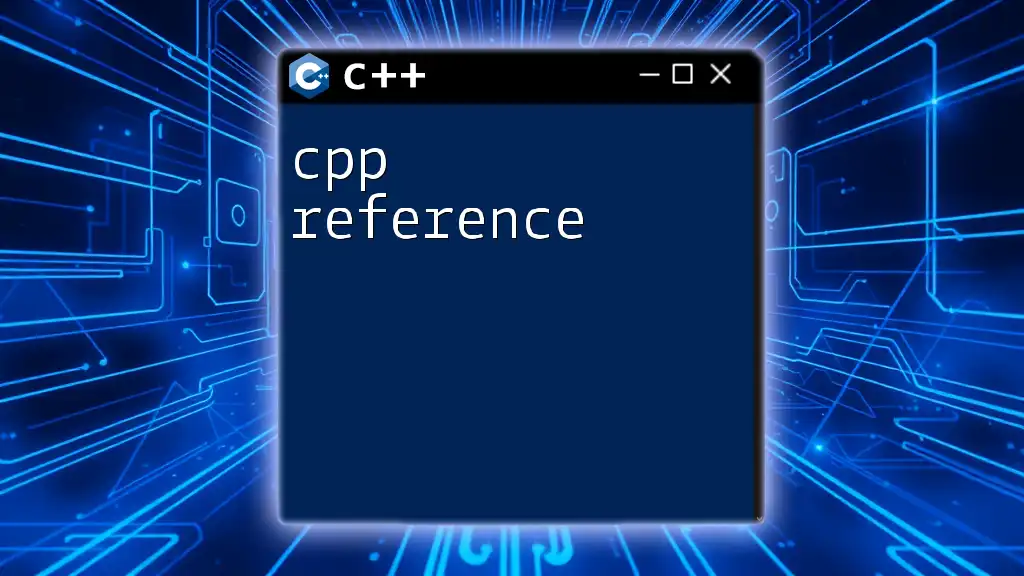
Best Practices for Using `register`
When to Use `register`
Using `register` can be beneficial in situations where performance is crucial, such as within tight loops or when handling frequently accessed variables. However, consider that most modern compilers handle optimization well, and it may be unnecessary to use `register` in many cases.
When to Avoid `register`
Despite its potential benefits, there are certain instances where using `register` may be counterproductive:
- Complex Data Types: Avoid `register` with large structures or classes, as they cannot fit into a CPU register.
- Compiler Optimization: Trust the compiler; modern compilers often outsmart manual hints.
- Debugging Difficulties: Using `register` may complicate debugging because variables can become harder to track and monitor.
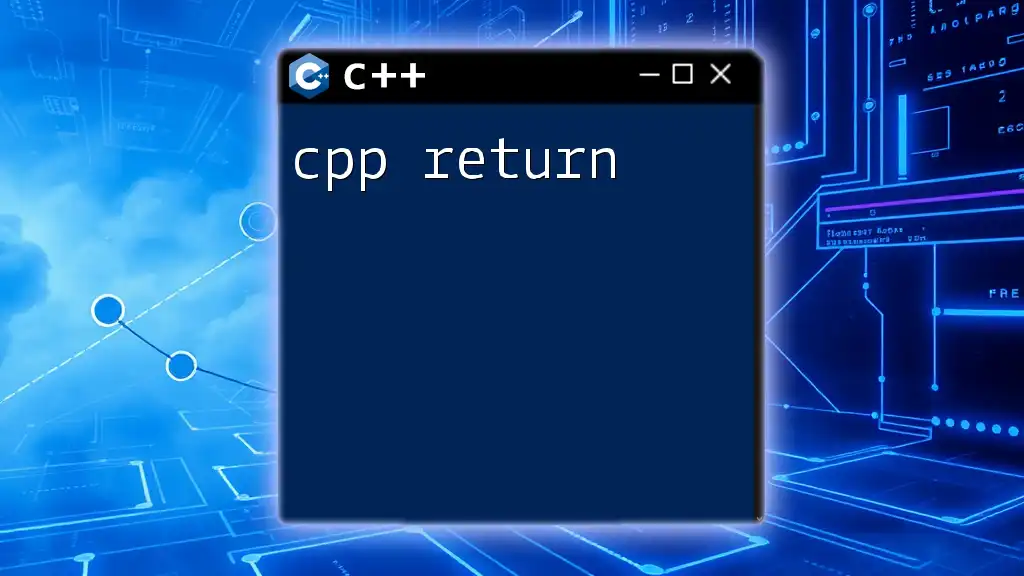
Modern Considerations
Compiler Optimization Technologies
Today's compilers are adept at optimization without the need for manual hints from programmers. Compilers apply various algorithms to optimize the placement of variables in memory, taking into account the specific architecture and workloads. This makes the `register` keyword less critical in contemporary C++ programming.
Profiling and Performance Analysis
To determine bottlenecks in performance, it’s advisable to use profiling tools. These tools can help identify where optimizations are genuinely needed rather than relying on `.register` as a catch-all solution. By using profiling techniques, developers can focus on areas of their codebase that require performance enhancements based on actual data rather than assumed requirements.
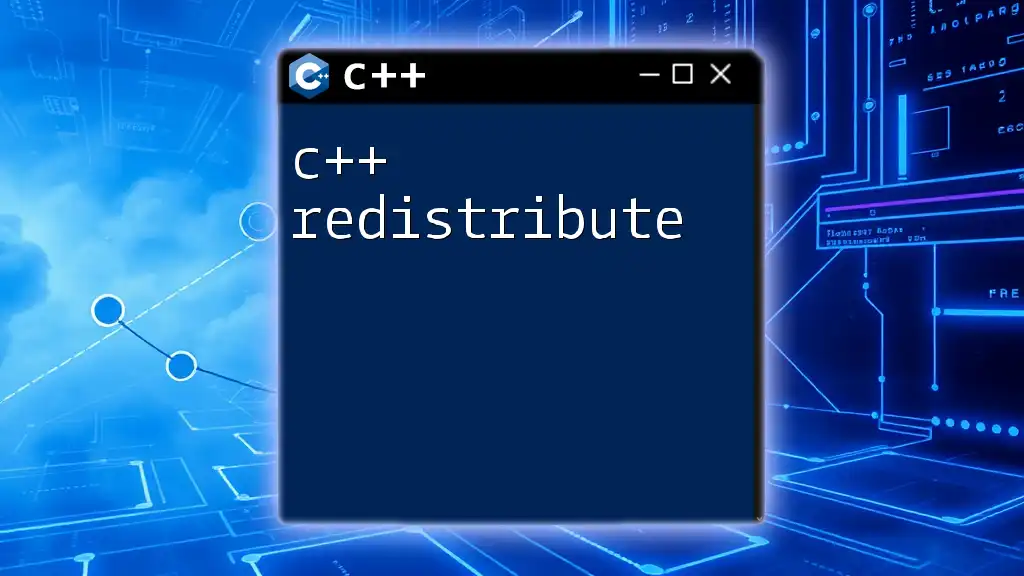
Conclusion
In summary, the `register` keyword in C++ serves as a mechanism for suggesting that a variable be stored in a register to optimize access time. However, with modern compilers' capabilities, manual hints are often unnecessary. As C++ evolves, understanding how to effectively leverage hardware and compiler optimizations will be crucial for performance-oriented programming. Being aware of when to use—or avoid—`register` is part of a developer's toolkit to create efficient, sophisticated applications in C++.
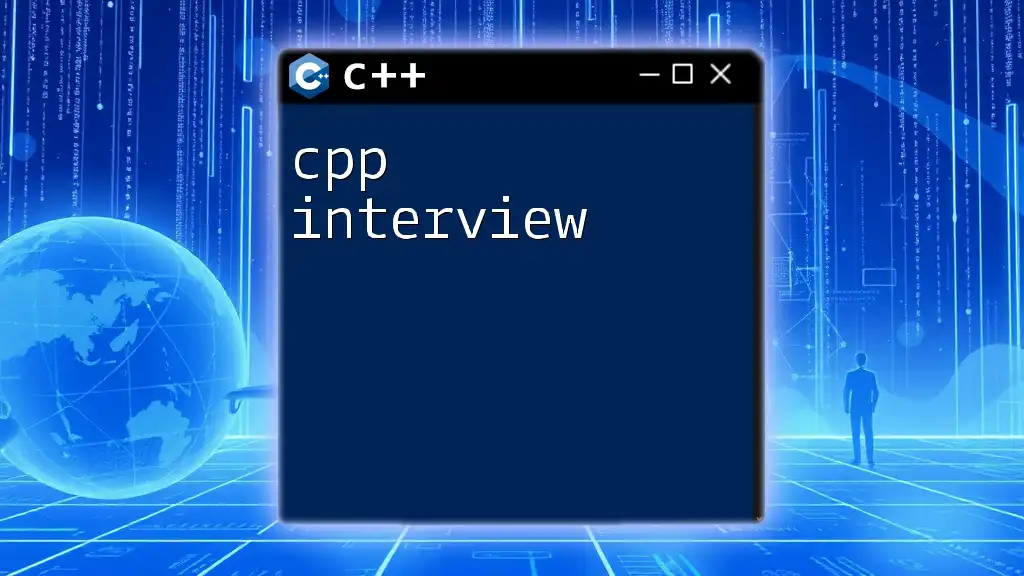
Additional Resources
For those looking to dive deeper into C++ optimization techniques, consider exploring comprehensive books, tutorials, and online articles surrounding compiler optimization. Engaging with communities can also yield valuable insights and personal experiences regarding the use of `register` in various applications.