In C++, interviews often involve evaluating candidates' understanding of core concepts and practical skills through coding questions that may require efficient solutions using C++ commands.
Here's a simple example of a C++ program that demonstrates how to reverse a string:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
std::reverse(str.begin(), str.end());
std::cout << str << std::endl; // Output: !dlroW ,olleH
return 0;
}
Understanding C and C++ Concepts
Key Differences Between C and C++
When preparing for a C++ interview, it’s crucial to understand the fundamental distinctions between C and C++. Here are some pivotal differences:
-
Syntax and Features: C is procedural, while C++ supports both procedural and object-oriented programming. This enables C++ to use classes, encapsulation, and polymorphism, offering greater flexibility in software development.
-
Memory Management: While C relies heavily on manual memory management using `malloc` and `free`, C++ introduces constructors and destructors to manage resource allocation and deallocation intelligently, reducing memory leaks and fragmentation.
-
Object-Oriented Programming: C++ allows the creation of objects that can hold both data and functions, a significant leap that improves code organization and reusability.
Essential C and C++ Knowledge
Before diving into the interview questions related to C++, ensure you have a firm grasp of the following essential concepts:
- Data Types and Operators: Familiarity with basic data types such as `int`, `float`, and `char` is necessary. Understanding operators—arithmetic, logical, and relational—is also critical for manipulation and decision-making within your code.
int a = 5, b = 10;
if (a < b) {
// Code executed if a is less than b
}
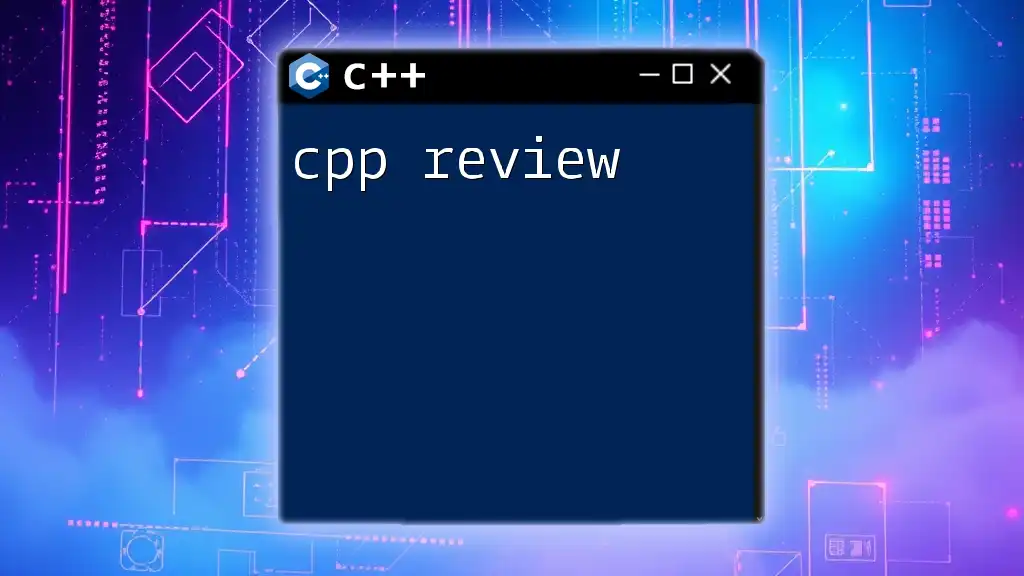
Common C++ Interview Topics
OOP Principles in C++
Understanding the four core principles of Object-Oriented Programming (OOP)—encapsulation, inheritance, polymorphism, and abstraction—is essential.
- Encapsulation: This principle restricts access to certain components of an object, allowing only authorized methods to modify the internal state.
class EncapsulatedClass {
private:
int secret;
public:
void setSecret(int s) { secret = s; }
int getSecret() { return secret; }
};
- Inheritance: It allows a new class (derived) to inherit the properties and methods of an existing class (base), promoting code reusability.
class Base {
public:
void display() { std::cout << "Base class" << std::endl; }
};
class Derived : public Base {
public:
void show() { std::cout << "Derived class" << std::endl; }
};
- Polymorphism: This enables objects to be treated as instances of their parent class. The most common forms are method overriding and overloading.
Memory Management in C++
Effective memory management is a critical aspect of C++ programming. Understanding dynamic versus static memory allocation is vital:
- Static Memory Allocation: This involves declaring variables beforehand. Memory is allocated at compile-time.
int array[10]; // Static allocation
- Dynamic Memory Allocation: Here, memory is allocated at runtime using `new` and released with `delete`.
int* array = new int[10]; // Dynamic allocation
delete[] array; // Dynamic deallocation
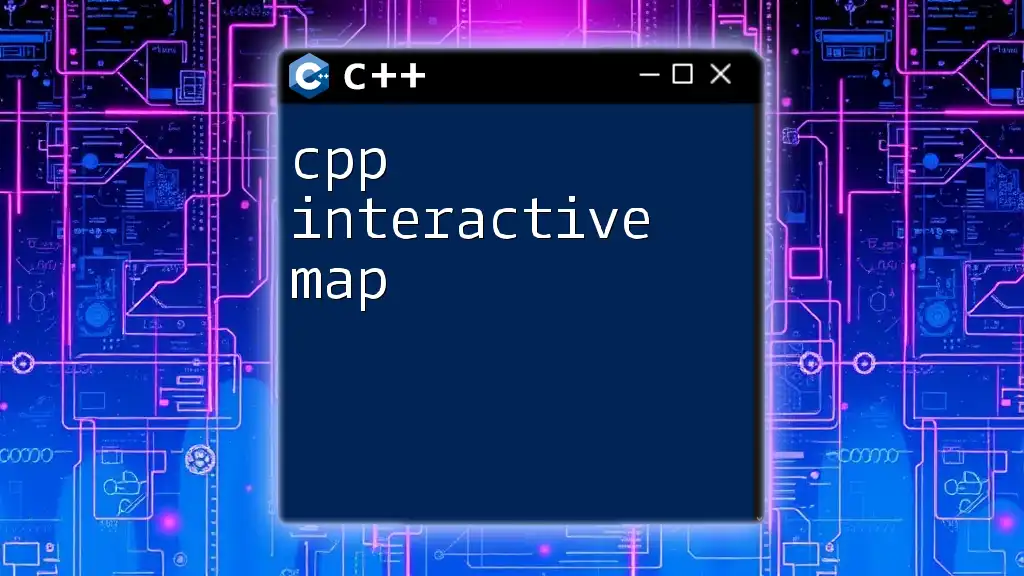
C++ Technical Interview Questions
Basic C++ Interview Questions
Your C++ interview may start with basic questions that assess foundational skills.
- What is a Constructor/Destructor? A constructor is a special member function that initializes objects, while a destructor cleans up before the object is destroyed.
class MyClass {
public:
MyClass() { std::cout << "Constructor called" << std::endl; }
~MyClass() { std::cout << "Destructor called" << std::endl; }
};
- What are Smart Pointers? Smart pointers such as `unique_ptr`, `shared_ptr`, and `weak_ptr` are used to manage the lifetime of dynamically allocated objects more efficiently, preventing memory leaks.
#include <memory>
std::unique_ptr<MyClass> ptr = std::make_unique<MyClass>();
Advanced C++ Interview Questions
As interviews progress, expect more challenging queries:
- Explain the Rule of Five. This rule asserts that if a class requires a user-defined destructor, copy constructor, or copy assignment operator, it likely needs all five special functions: destructor, copy constructor, copy assignment operator, move constructor, and move assignment operator.
class RuleOfFive {
public:
RuleOfFive() { /* Constructor */ }
~RuleOfFive() { /* Destructor */ }
RuleOfFive(const RuleOfFive& other) { /* Copy Constructor */ }
RuleOfFive& operator=(const RuleOfFive& other) { /* Copy Assignment */ }
RuleOfFive(RuleOfFive&& other) noexcept { /* Move Constructor */ }
RuleOfFive& operator=(RuleOfFive&& other) noexcept { /* Move Assignment */ }
};
- What are the benefits of using STL? The Standard Template Library (STL) provides powerful data structures and algorithms that allow for efficient coding and code reusability. Key components include vectors, lists, and maps.
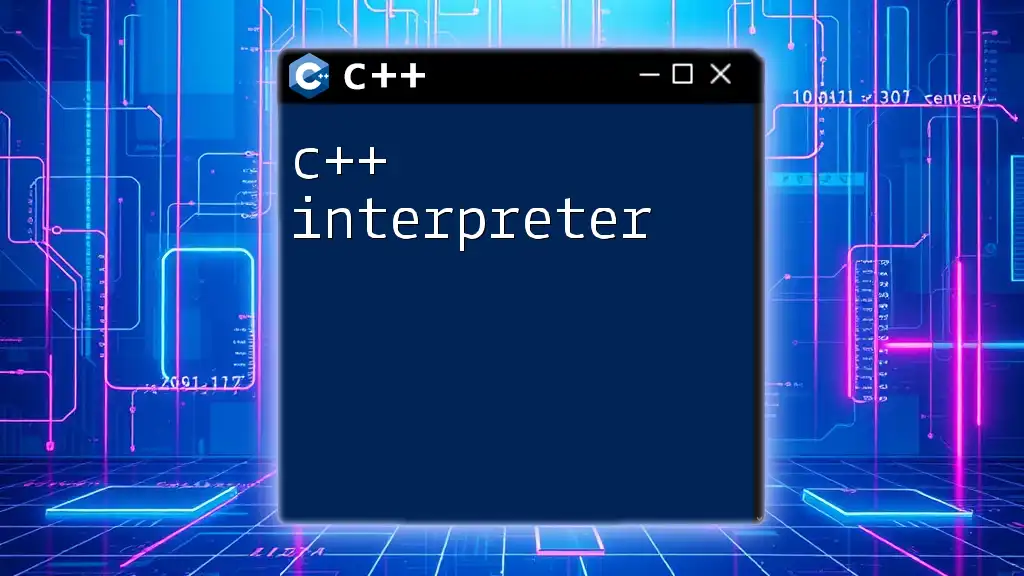
C and C++ Interview Questions
Language-Specific Questions
Building your knowledge base, especially around language-specific nuances, is vital:
- Differences in Pointers and References: A pointer can be reassigned and points to an address, while a reference acts as an alias for another variable and cannot be changed.
int x = 10;
int* ptr = &x; // Pointer
int& ref = x; // Reference
- When to use `const` and `volatile`? Use `const` when a variable should not change after initialization, providing compile-time safety. `volatile` is used for variables that can be changed unexpectedly, such as in multi-threaded environments.
Function Overloading and Templates
Understanding concepts of function overloading and templates enhances versatility in coding:
- What is Function Overloading? This allows you to define multiple functions with the same name but different parameters.
void display(int a) { std::cout << "Integer: " << a << std::endl; }
void display(double a) { std::cout << "Double: " << a << std::endl; }
- Working with Templates: Templates enable you to create functions and classes that can work with any data type.
template <typename T>
T add(T a, T b) {
return a + b;
}
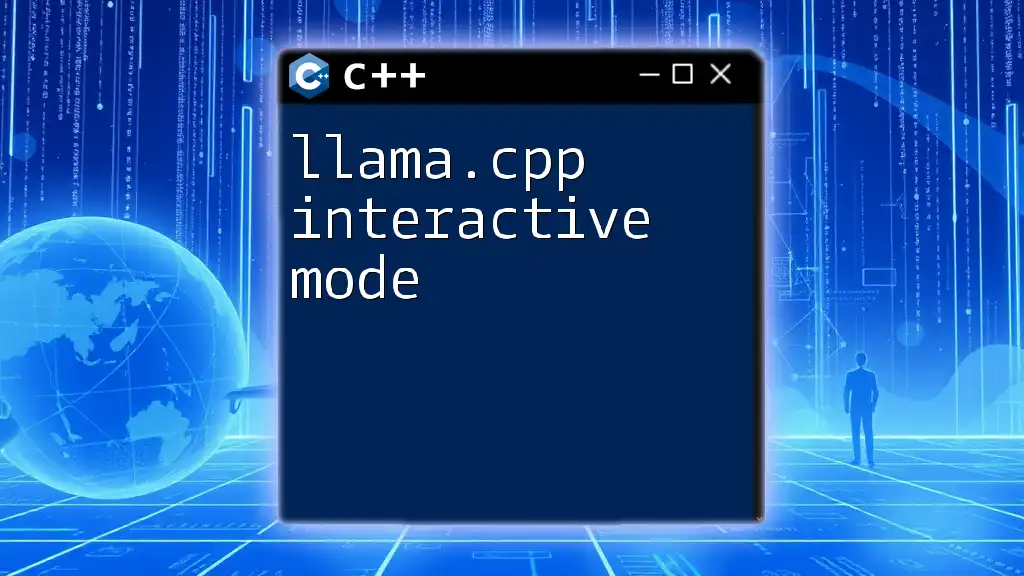
C++ Interview Questions and Answers
Sample Questions with Detailed Answers
- What is an abstract class and interfaces? An abstract class is a class that cannot be instantiated and often contains pure virtual functions. Interfaces provide a contract for classes without implementing any functionality.
class AbstractBase {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
};
- What are Lambdas in C++? Lambda functions provide a concise way to define anonymous functions directly in your code, enhancing functional programming capabilities in C++.
auto add = [](int a, int b) { return a + b; };
std::cout << add(5, 3); // Outputs 8
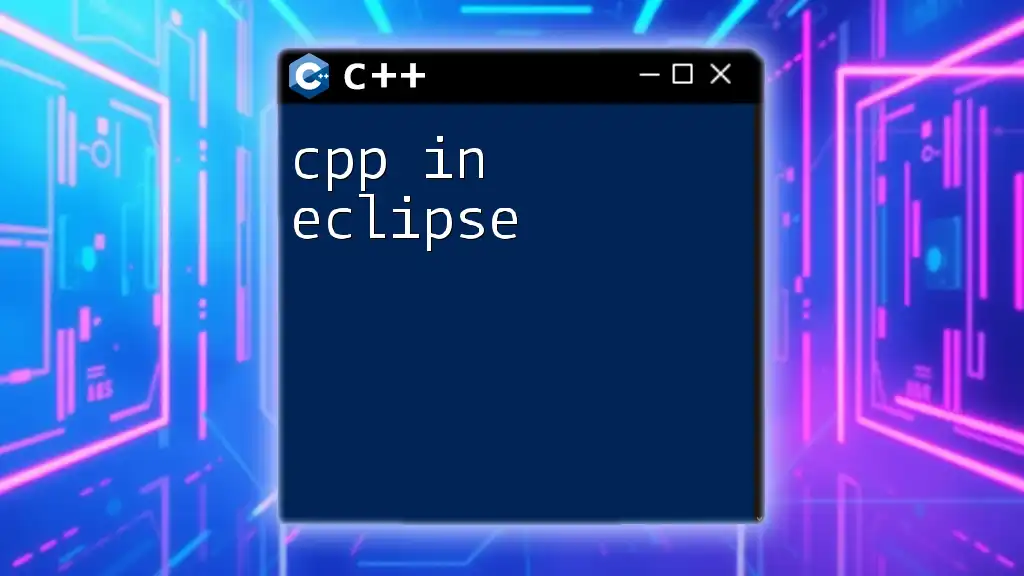
Preparing for C++ Interviews
Resources for C++ Preparation
Preparation requires a curated list of resources to succeed:
- Books, Courses, and Online Communities: Recommended books include "Effective C++" by Scott Meyers and "The C++ Programming Language" by Bjarne Stroustrup. Online platforms like Coursera, edX, and competitive programming sites offer practical exercises.
Mock Interviews and Practice
Consider conducting mock interviews focusing on both theoretical knowledge and coding skills. This practice helps identify areas for improvement and enhances your confidence.
Common Mistakes to Avoid
Be aware of typical pitfalls like:
- Overcomplicating solutions or failing to optimize code.
- Misunderstanding fundamental concepts such as pointers vs. references.
- Lack of proper syntax and use of C++ features.
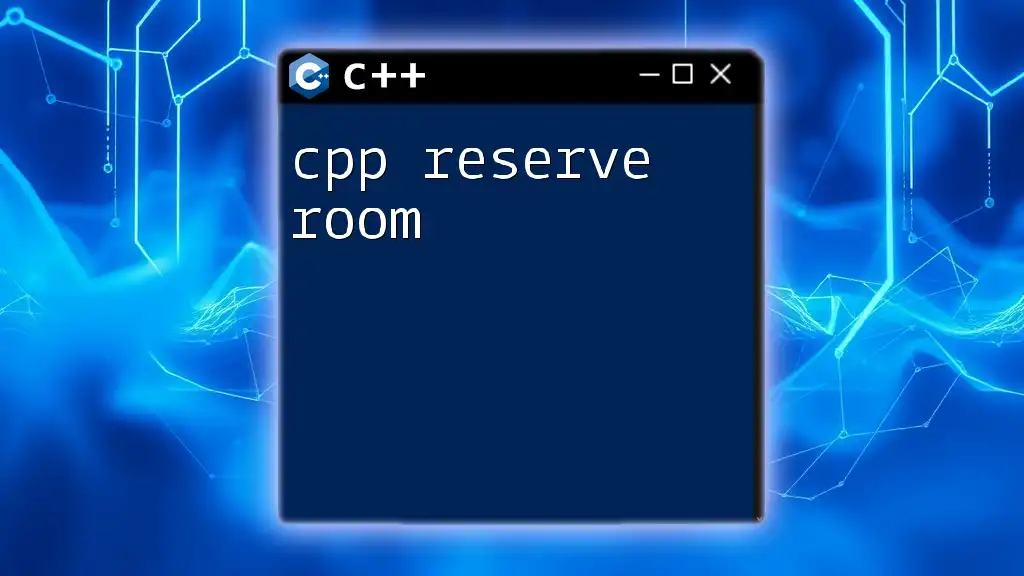
Conclusion
As you prepare for a C++ interview, revisit and master these concepts, principles, and questions. Regular practice and continuous learning are pivotal to becoming proficient in C++ programming. Don’t hesitate to seek feedback and engage with programming communities to further sharpen your skills. Embrace challenges, and you will undoubtedly excel in your C++ interviews!