Eclipse is a powerful Integrated Development Environment (IDE) that allows developers to efficiently write, compile, and debug C++ programs using its built-in tools and features.
#include <iostream>
int main() {
std::cout << "Hello, C++ in Eclipse!" << std::endl;
return 0;
}
Setting Up Eclipse for C++
Downloading and Installing Eclipse
Choosing the Right Version:
When it comes to developing C++ applications, selecting the correct version of Eclipse is crucial. You should opt for the Eclipse IDE for C/C++ Developers version, which comes pre-packaged with the necessary tools and features tailored for C++ programming.
Installation Steps:
To install Eclipse, follow these steps:
- Navigate to the [Eclipse download page](https://www.eclipse.org/downloads/).
- Select the C/C++ version specifically designed for developers.
- Download the appropriate installer for your operating system.
- Execute the installer and follow the instructions provided, ensuring that you select the correct workspace location.
Configuring the Eclipse IDE
Setting Up Workspaces:
A workspace is a directory where all your projects and resources will reside. It’s important to define this location upon your first launch of Eclipse. You can create multiple workspaces to organize projects by type or function. To create or change a workspace, go to File > Switch Workspace > Other... and select or create a new one.
Installing C++ Development Tools (CDT):
The C/C++ Development Tooling (CDT) is essential for C++ development in Eclipse. If it is not included in your installation, follow these steps to install it:
- Open Eclipse and navigate to Help > Eclipse Marketplace...
- Search for "C/C++ Development Tools" and click Go.
- Follow the prompts to install CDT and restart Eclipse.
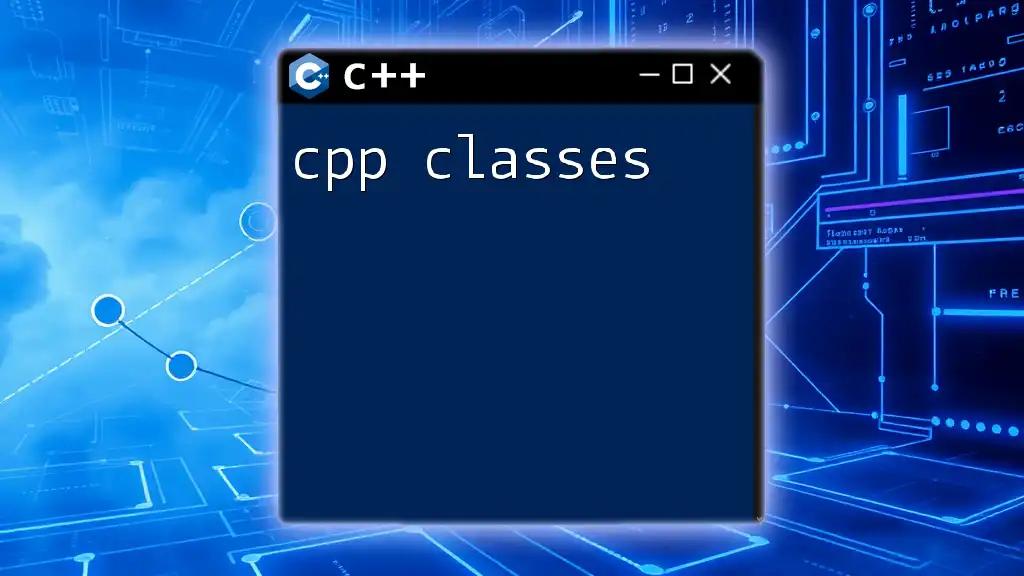
Creating Your First C++ Project in Eclipse
Starting a New C++ Project
Project Types in Eclipse:
Eclipse supports various project types for C++. The two primary types are:
- C++ Project: A more controlled environment where you manage the build process yourself.
- C++ Managed Make Project: Automatically handles the build configurations for you.
Step-by-Step Project Creation:
To create a new C++ project, follow this procedure:
- Click on File > New > C++ Project.
- Choose the desired project type (e.g., C++ Project).
- Enter your project name and select a location for it.
- Click Finish to create the project.
Writing Your First C++ Program
Now that your project is set up, it's time to write your first code.
Code Example: Hello World Program
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explaining the Code Snippet:
- `#include <iostream>`: This directive includes the input/output stream library, which allows you to use `std::cout`.
- `int main() {...}`: This declares the main function, which serves as the entry point for any C++ program.
- `std::cout << "Hello, World!" << std::endl;`: This line prints "Hello, World!" to the console, followed by a newline.
- `return 0;`: This signifies successful execution of the program.
To compile and run the program, click on the green play button in the Eclipse toolbar.
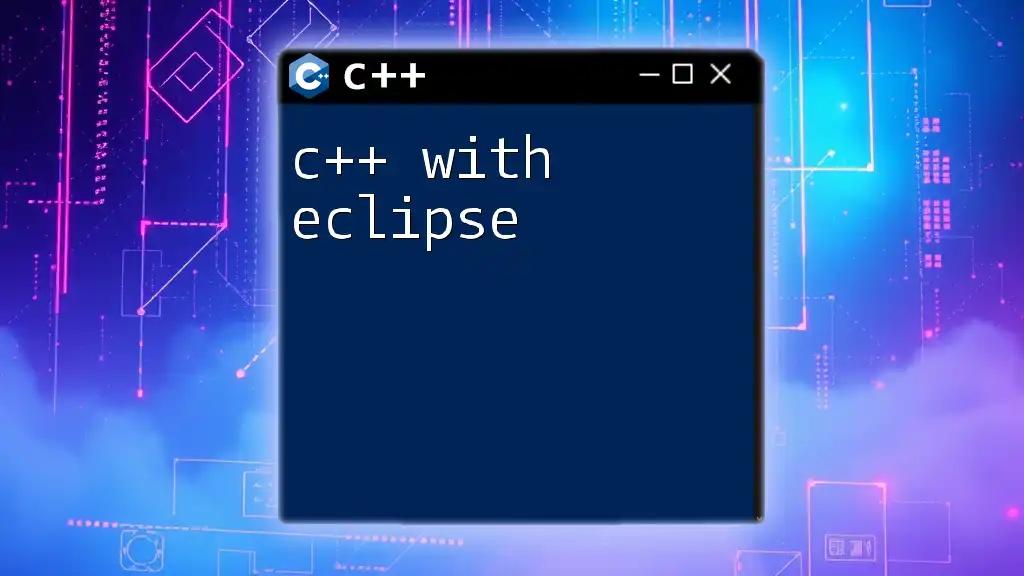
Navigating the Eclipse Environment
Understanding the User Interface
Eclipse is comprised of various components, including:
- Editors: Where you write your code.
- Views: Panels that provide information about projects, files, and more.
- Perspectives: Customized layouts that help focus on different tasks (e.g., coding, debugging).
By understanding these features, you can navigate Eclipse more effectively.
Using the Built-in Features
Code Completion and Syntax Highlighting:
One of the advantages of using an IDE like Eclipse is its code completion feature, which helps you write code faster by suggesting function names and variable types. It also offers syntax highlighting to differentiate between keywords, strings, and comments, making your code more readable.
Error Detection and Debugging Tools:
Eclipse includes robust debugging tools. You can set breakpoints by double-clicking the left margin next to a line number in the editor. Once a breakpoint is set, use the debugger by navigating to Run > Debug. This lets you step through your code line by line, inspect variable states, and diagnose issues efficiently.
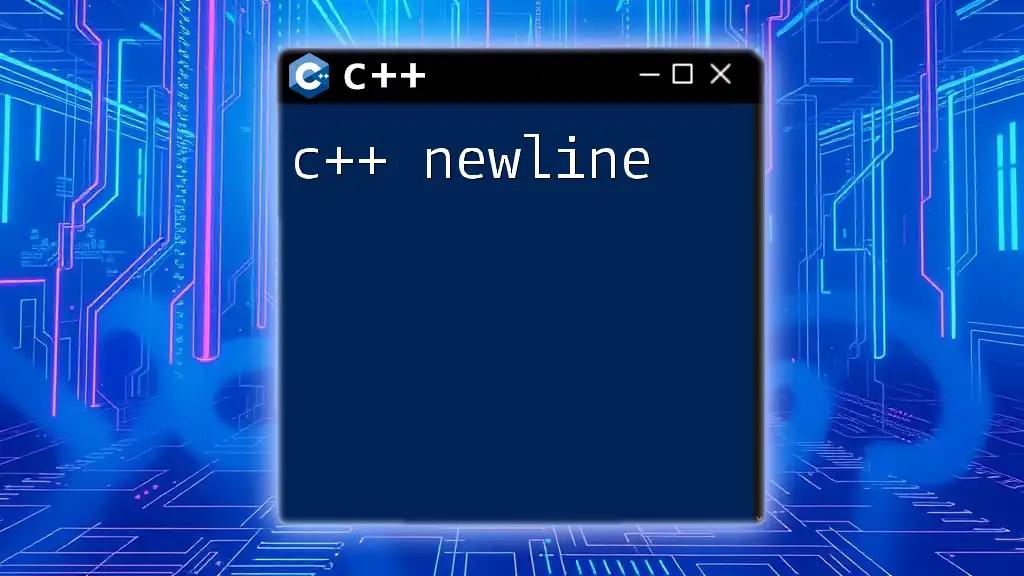
Compiling and Running C++ Code in Eclipse
Build Configurations
Managing your project’s build settings is essential for successful compilation. Eclipse allows you to configure settings for both Debug and Release builds individually. Navigate to your project, right-click, and select Properties. Here, you can adjust optimization levels, include paths, and other compiler settings.
Running Your Program
After writing your code, you can compile and run your program directly from Eclipse. Utilize the Run button (green play icon) found in the toolbar. The output will appear in the Console view at the bottom of the IDE, allowing you to see your program's results seamlessly.
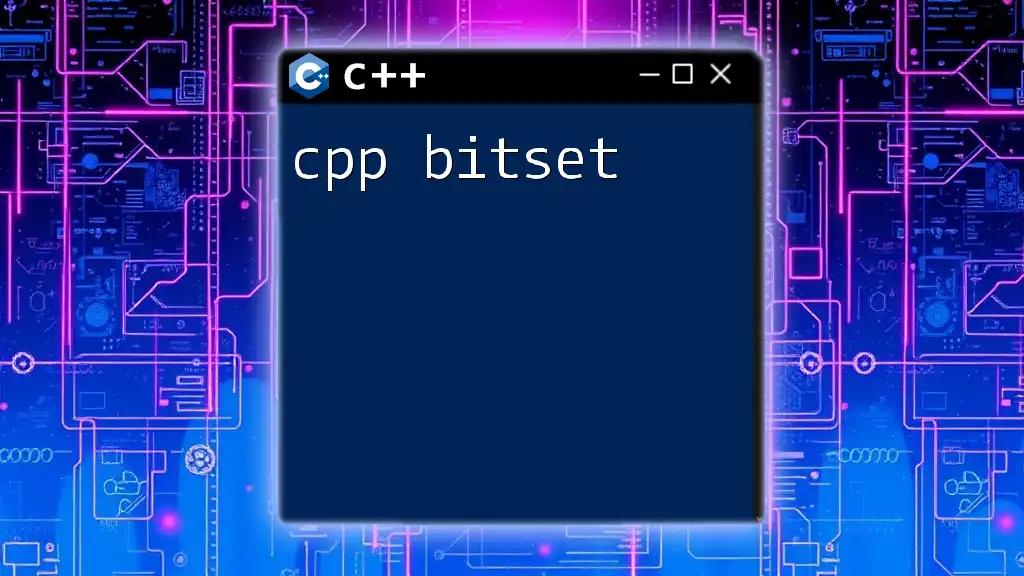
Advanced Features in Eclipse
Using External Libraries
As your C++ projects grow more complex, you may need to link external libraries. This process involves adding library files to your project, allowing you to utilize pre-written functions and classes.
To link libraries:
- Right-click on your project and select Properties.
- Go to C/C++ Build > Settings.
- Under the GCC C++ Linker section, find Libraries, and add the required libraries.
Version Control Integration
Version control is vital for managing changes to your code over time. Eclipse supports integration with Git, which is one of the most popular version control systems. To enable Git in Eclipse:
- Navigate to Help > Eclipse Marketplace and search for "EGit".
- Install the EGit plugin.
- Restart Eclipse, and you can now create repositories, commit changes, and manage branches directly from the Eclipse interface.
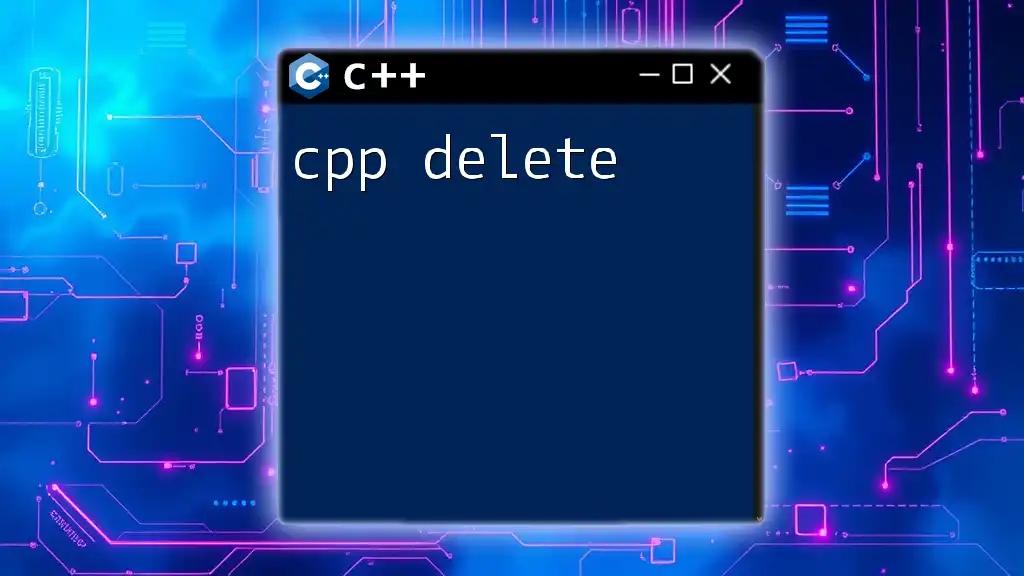
Common Issues and Troubleshooting Tips
Common Errors in Eclipse
While working in Eclipse, you may encounter compilation errors or warnings. Common problems include:
- Missing includes or libraries, which can be resolved by adjusting your project settings.
- Misconfigured build paths; ensure you have correctly set up include directories under project properties.
Debugging Tips for Eclipse
When debugging, start by checking the console output for errors. Utilize Eclipse's built-in debugger to set breakpoints strategically. Sometimes, observing the flow of execution can reveal issues that are not immediately apparent in the code.
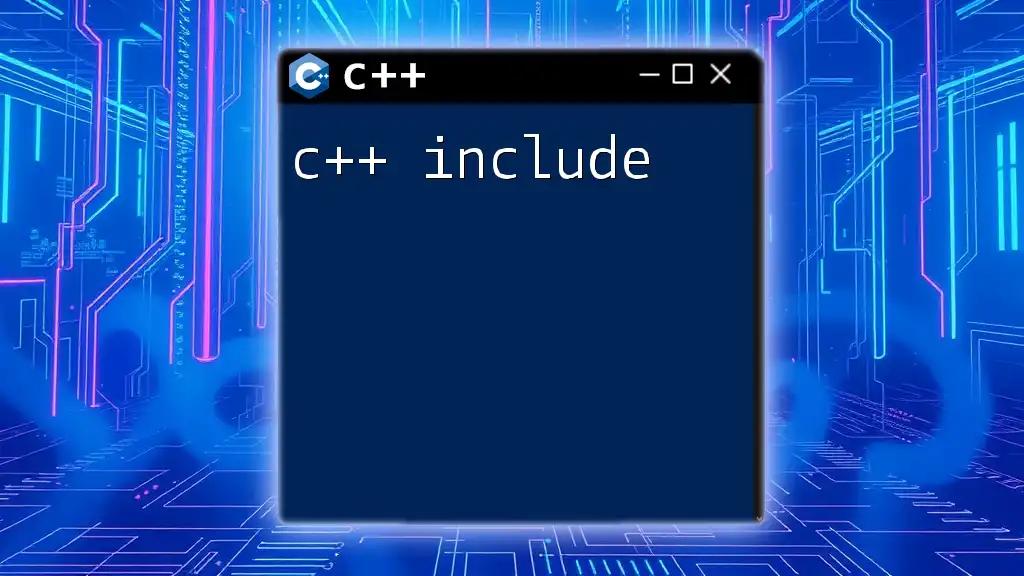
Conclusion
Mastering C++ in Eclipse offers a powerful environment that enhances your programming efficiency. Familiarizing yourself with the IDE's tools can significantly shorten your learning curve and enable you to write cleaner, more effective code. By working through hands-on examples and employing the features discussed, you can become proficient in using C++ and Eclipse.
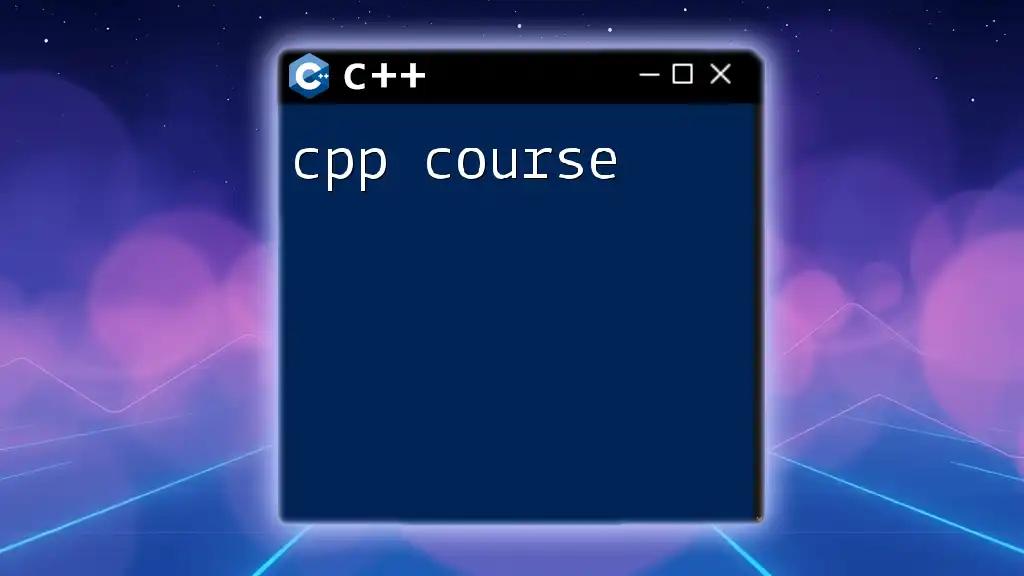
Further Resources
To expand your learning, consider exploring the following resources:
- Recommended Books and Online Courses: Look for popular C++ programming books and online courses that offer practical exercises and deep insights.
- Online Communities and Forums: Engage with other C++ developers in forums or community platforms like Stack Overflow or dedicated Eclipse forums to share knowledge and troubleshoot common programming problems.