C++ classes are user-defined data types that enable the encapsulation of data and functions, allowing for organized and reusable code.
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
Understanding the Basics of C++ Classes
What is a Class in C++?
A class is a user-defined data type that allows you to combine data and functions into a single unit. This concept forms the backbone of object-oriented programming (OOP), which provides a way to structure software in a more manageable and modular fashion. C++ classes encapsulate data for the object and provide methods for manipulating that data, making your programs more organized and easier to maintain.
In C++, classes also bring the concept of abstraction and inheritance into play, allowing developers to model real-world entities and relationships effectively.
Key Components of a C++ Class
Attributes (data members) and methods (member functions) are the core components of a class. Attributes define the state of an object, while methods define its behavior.
Attributes
Attributes represent the properties of a class. For example, if we have a `Car` class, attributes might include `make`, `model`, and `year`.
Methods
Methods are functions that operate on the class' attributes. For instance, a method might display the car's information or calculate its age based on the current year.
Access Specifiers
In C++, you can control the visibility of your class members using access specifiers:
- Public: Members are accessible from outside the class.
- Private: Members are accessible only within the class.
- Protected: Members are accessible within the class and by derived classes.
Example: Access Specifiers in a Class
class MyClass {
public:
int publicAttribute; // Accessible everywhere
private:
int privateAttribute; // Accessible only within MyClass
};

How to Make a C++ Class
Defining a C++ Class
Defining a class in C++ is relatively straightforward. It starts with the `class` keyword, followed by the class name and its body enclosed in curly braces. The body contains the attributes and methods.
Example: Basic Class Definition
class Car {
public:
string make;
string model;
int year;
void displayInfo() {
cout << year << " " << make << " " << model << endl;
}
};
Creating Objects from a Class
Once you've defined a class, you can create objects (instances) from it. An object represents a specific item defined by the class, such as a particular car.
Example: Creating Objects
int main() {
Car myCar; // Create an object of Car class
myCar.make = "Toyota"; // Set the make attribute
myCar.model = "Corolla"; // Set the model attribute
myCar.year = 2022; // Set the year attribute
myCar.displayInfo(); // Output: 2022 Toyota Corolla
return 0;
}

Advanced Concepts in C++ Classes
Constructors and Destructors
In C++, constructors are special member functions that are called when an object of a class is instantiated. They help initialize the object. Destructors, on the other hand, clean up when an object goes out of scope or is deleted.
Here are the types of constructors to know:
- Default Constructor: No parameters.
- Parameterized Constructor: Takes parameters to initialize an object in a specific way.
- Copy Constructor: Used to create a new object as a copy of an existing object.
Example: Constructor and Destructor
class Book {
public:
string title;
// Constructor
Book(string t) : title(t) {}
// Destructor
~Book() {
cout << title << " destroyed." << endl;
}
};
Static Members in C++ Classes
Static members are shared among all objects of the class. This means that they maintain a single copy and are not tied to any specific instance. Static members can be both attributes and methods.
Example: Static Member Example
class Counter {
public:
static int count; // Static member
Counter() {
count++; // Increment count for each object created
}
};
int Counter::count = 0; // Initialize static member
Inheritance in C++ Classes
Understanding Inheritance
Inheritance allows one class (derived class) to inherit properties and methods from another class (base class), enabling code reuse and establishing hierarchical relationships in your code.
Base and Derived Class Example
Using inheritance, you can create a `Vehicle` class (base) and derive a `Bike` class from it.
Example: Implementing Inheritance
class Vehicle {
public:
void start() {
cout << "Vehicle started." << endl;
}
};
class Bike : public Vehicle {
public:
void pedal() {
cout << "Pedaling the bike." << endl;
}
};

Best Practices for Using C++ Classes
Naming Conventions
When defining C++ classes, adhere to naming conventions to enhance readability and maintainability. Use uppercase letters for class names (e.g., `Car`) and camel case for member functions (e.g., `displayInfo`).
Documentation and Comments
Include comments in your code to explain the purpose of your classes and methods. Good documentation significantly aids future code maintenance and enhances collaboration with other developers. Consider using tools like Doxygen for automatically generating documentation from annotated source code.
Performance Considerations
Understanding the memory implications of classes is crucial. When you copy objects, especially large ones, consider defining copy constructors and assignment operators to manage resources effectively. Prefer passing objects by reference when appropriate to avoid unnecessary copying.

Conclusion
C++ classes serve as a fundamental building block in programming, enabling developers to encapsulate data and behavior efficiently. By mastering the concepts of classes, including encapsulation, inheritance, and polymorphism, you can structure your code in a way that is both efficient and easy to understand. Experimenting with various class structures will deepen your understanding and enrich your programming skills in C++.

Additional Resources
Consider diving deeper into C++ documentation and recommended books to enhance your knowledge of C++ classes. Online courses focusing on object-oriented programming in C++ are also excellent resources for expanding your skills.
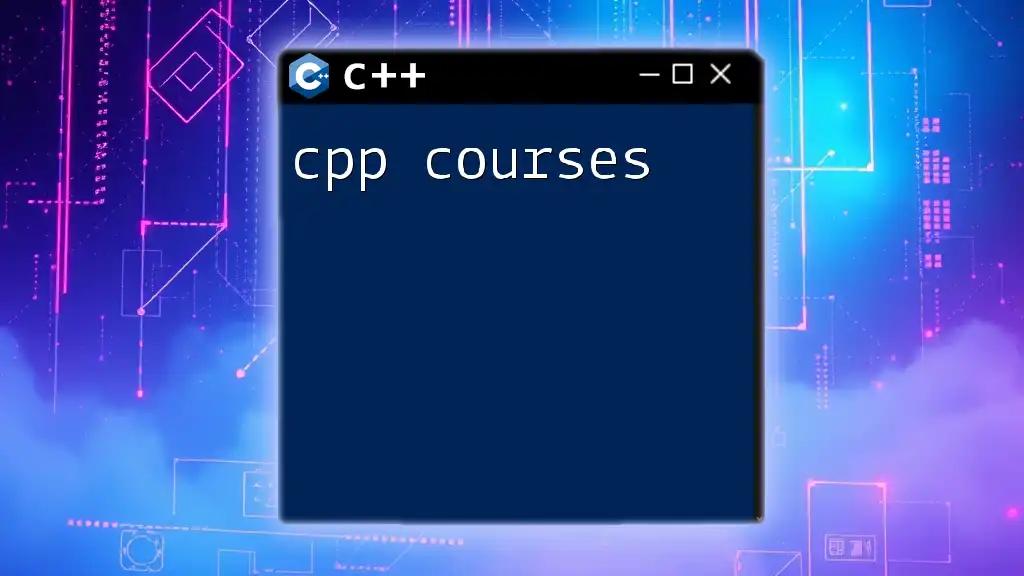
FAQs
What are the advantages of using classes in C++?
Using classes allows for better organization of code, code reuse through inheritance, data encapsulation, and easier maintenance.
How do I manage memory for class objects in C++?
Memory management can be managed using constructors, destructors, and smart pointers to prevent memory leaks.
Can a class inherit from multiple classes in C++?
Yes, C++ supports multiple inheritance, where a class can inherit from more than one base class.
What is the difference between an object and a class in C++?
A class is a blueprint for creating objects, while an object is an instance of a class with specific data and functionality defined by the class.