The "cpp shell" refers to an interactive command-line interface for evaluating and executing C preprocessor commands, allowing users to quickly test and experiment with macro definitions and includes.
#include <iostream>
#define SQUARE(x) ((x) * (x))
int main() {
std::cout << "The square of 5 is: " << SQUARE(5) << std::endl;
return 0;
}
What is C++ Shell?
CPP Shell is an online platform that allows users to write, test, and execute C++ code in a web-based environment. Its importance lies in providing immediate feedback, which is crucial for both beginners and experienced programmers alike. C++ Shell serves as a fantastic tool for learning and experimentation, enabling users to quickly validate their C++ commands and results without the need to set up a complicated local environment.
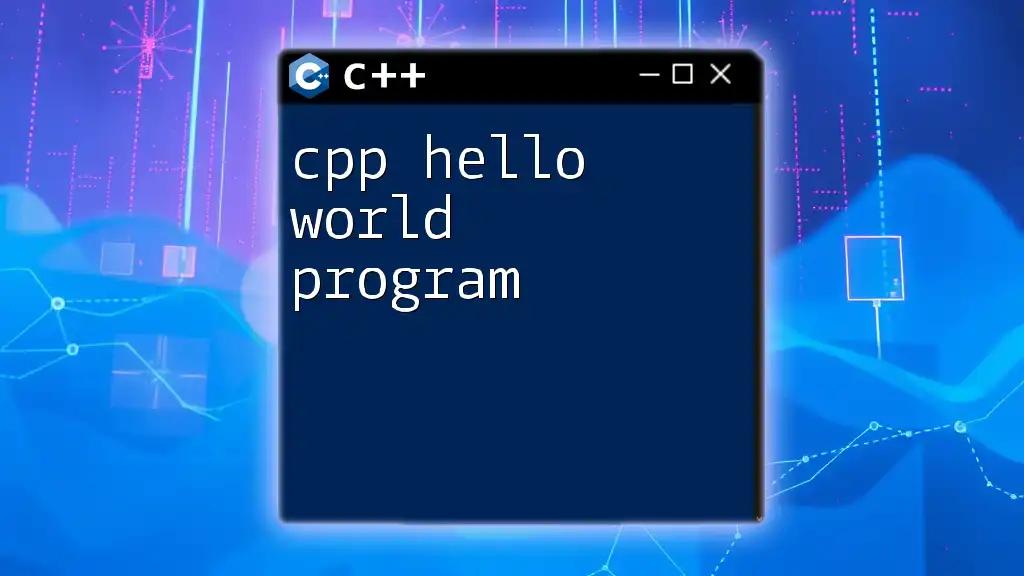
Why Use C++ Shell?
Using C++ Shell offers several benefits for learning and applying C++. It promotes a fast and efficient learning curve, allowing users to experiment with code and receive immediate feedback. By removing the barriers of installation and configuration, C++ Shell allows learners to focus solely on writing and understanding C++ code.

Getting Started with C++ Shell
Setting Up Your Environment
To start using C++ Shell, you can choose either an online compiler or set up a local environment with g++. There are several popular online compilers, like repl.it, cpp.sh, and Ideone. These platforms allow you to run C++ code without installing anything on your machine, making it easier for newcomers to dive right in.
If you prefer a local setup, installing g++ is straightforward. Follow these basic configuration steps:
- Download and install an IDE like Code::Blocks or Visual Studio Code.
- Install the GCC compiler, which includes g++.
- Set the environment path to include the g++ compiler, allowing it to recognize the command from the terminal or console.
Writing Your First C++ Command
A basic C++ program structure is simple but essential. It typically includes headers, a main function, and a return statement.
Here’s a simple C++ program to get you started:
#include <iostream>
int main() {
std::cout << "Hello, C++ Shell!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` allows us to use the input/output stream.
- `int main()` defines the program's starting point.
- `std::cout` is used to output text to the console, and `std::endl` ensures the output is followed by a new line.
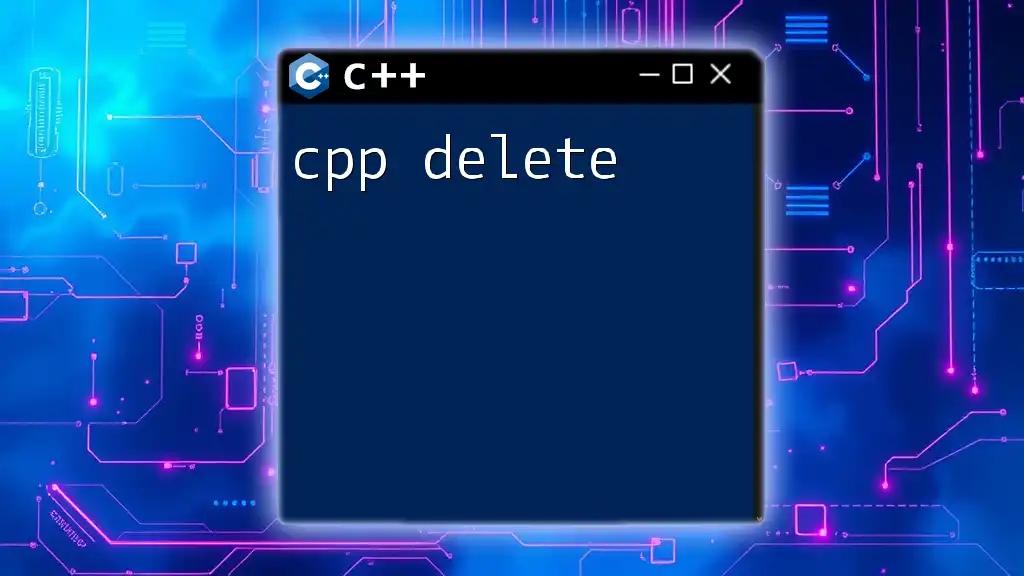
Core Commands in C++ Shell
Input and Output Commands
Understanding how to handle input and output is fundamental in C++. The `std::cout` command is used to display output, while `std::cin` is for accepting user input.
Here’s an example of using output:
std::cout << "Welcome to C++ Shell!" << std::endl;
To gather user input, you would use:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
This snippet prompts the user to enter their age and stores it in the variable `age`. Understanding how to effectively manage input and output is crucial for creating interactive applications.
Control Flow Commands
C++ provides several control flow commands that allow your code to make decisions or iterate over data.
Conditional Statements
A basic if statement checks a condition:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
In this example, if the variable `age` is 18 or older, the console will output "You are an adult."
Looping Constructs
Looping constructs such as for and while enable you to repeat code. Here’s a simple for loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This loop will print the numbers 0 to 4 in the console, demonstrating the basic usage of loops in C++.
Variables and Data Types
Variables store data and are defined with specific data types. Common data types in C++ include `int`, `float`, `char`, and `bool`. Declaring and initializing variables correctly is essential for effective programming.
Here’s how you might declare different types of variables:
float height = 1.75;
int grade = 90;
char initial = 'A';
Choosing the right data type for your variables can enhance performance and reduce memory usage, which is a key consideration in C++ programming.
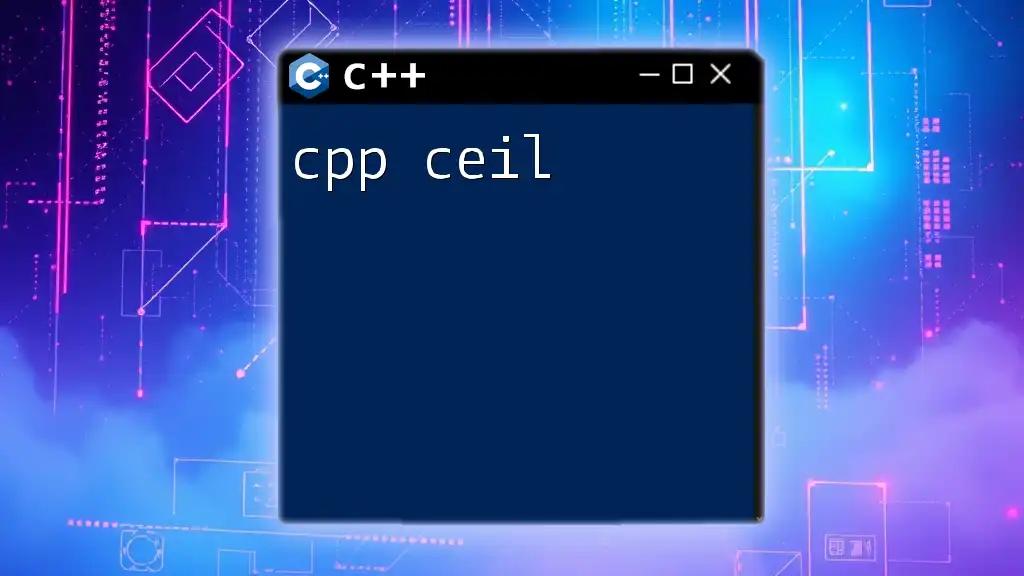
Advanced Topics in C++ Shell
Functions in C++
Functions are blocks of code designed to perform specific tasks. They promote code reusability and can take parameters and return values.
Here’s a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
To call this function, you would use:
int result = add(5, 3);
std::cout << "Sum: " << result << std::endl;
Learning how to create and utilize functions efficiently is a crucial skill in developing larger, more modular programs.
Error Handling
Effective error handling is a cornerstone of robust programming. In C++, you can utilize try-catch blocks to manage exceptions.
Here’s a simple example:
try {
int result = divide(x, y);
} catch (const std::exception& e) {
std::cout << "Error: " << e.what() << std::endl;
}
This structure allows you to anticipate potential issues during runtime and handle them gracefully, ensuring your program doesn’t crash unexpectedly.
Libraries and Includes
C++ leverages libraries to extend its functionality. To use libraries, you include them in your script with the `#include` directive.
Here is an example of including standard libraries:
#include <vector>
#include <string>
These libraries provide additional data structures and capabilities, allowing you to build more complex applications effortlessly.

Practical C++ Shell Examples
Creating a Simple Calculator
Making a basic calculator is an excellent project for understanding C++. Below is an outline of how you might develop one:
-
Declare Functions for Operations:
- Define functions for addition, subtraction, multiplication, and division.
-
User Interface for Input:
- Prompt the user to enter numbers and the operation they wish to perform.
-
Perform Calculation:
- Use conditional statements to determine which operation to execute.
Here’s a simplified code snippet to illustrate:
double add(double a, double b) { return a + b; }
double subtract(double a, double b) { return a - b; }
double multiply(double a, double b) { return a * b; }
double divide(double a, double b) { return (b != 0) ? a / b : throw std::invalid_argument("Cannot divide by zero"); }
By breaking down the application into functions, you can facilitate easier testing and debugging.
Real-World Application of C++ Shell
CPP Shell is not only beneficial for C++ learners but also a versatile tool for programming in other languages. Understanding C++ commands can provide foundational skills that easily translate to languages such as Java and Python. The logic and structuring in programming are often similar, making your learning transferable across different platforms.
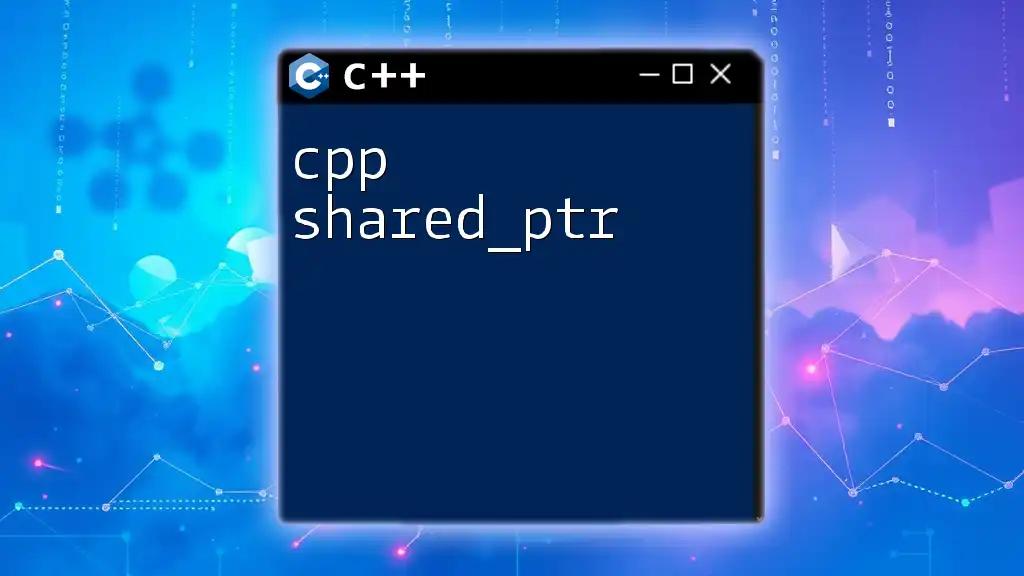
Best Practices for Using C++ Shell
Tips for Writing Efficient C++ Code
When coding in C++, remember the importance of cleanliness and clarity. Write code that is easy to read and maintain. Use meaningful variable names and comment your code to explain complex logic. This practice helps both you and others who may review your code in the future.
Testing and Debugging in C++ Shell
Effective testing and debugging techniques can significantly enhance your development workflow. Use C++ Shell to validate your code incrementally. This way, you can identify issues early and fix them before they escalate. Utilize tools like built-in debuggers or integrated development environments (IDEs) to step through your code and inspect variables in real-time.

Conclusion
In this guide, we have explored the intricacies of the C++ Shell environment, from setting it up to executing complex commands. By understanding the fundamental concepts, such as input/output, control flow, functions, and error handling, you empower yourself to write effective C++ code.
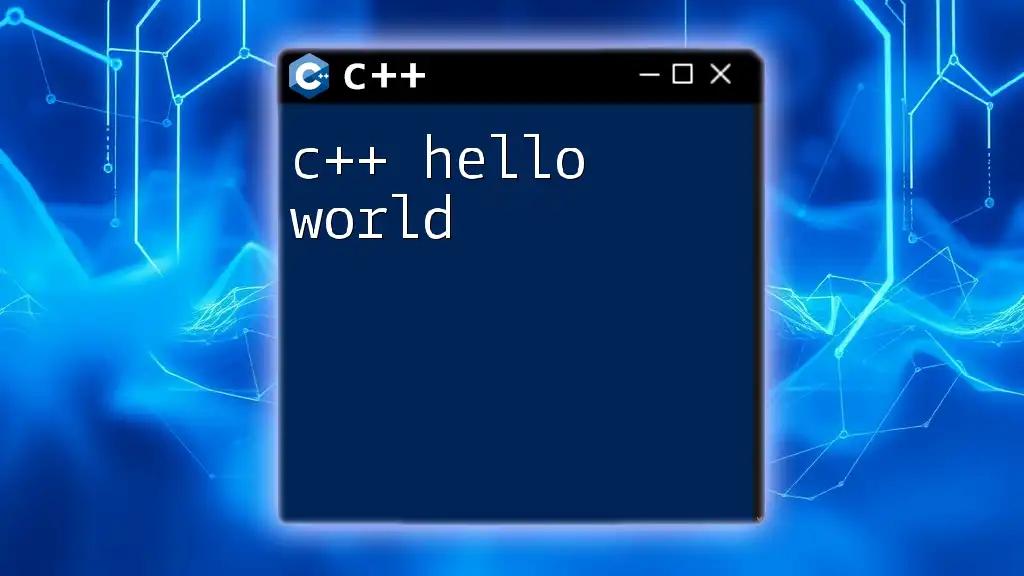
Further Reading and Resources
To further develop your C++ skills, consider diving into books such as "C++ Primer" by Stanley B. Lippman or online resources like C++ reference documentation and coding forums. Regular practice in C++ Shell will reinforce your learning and improve your coding aptitude.
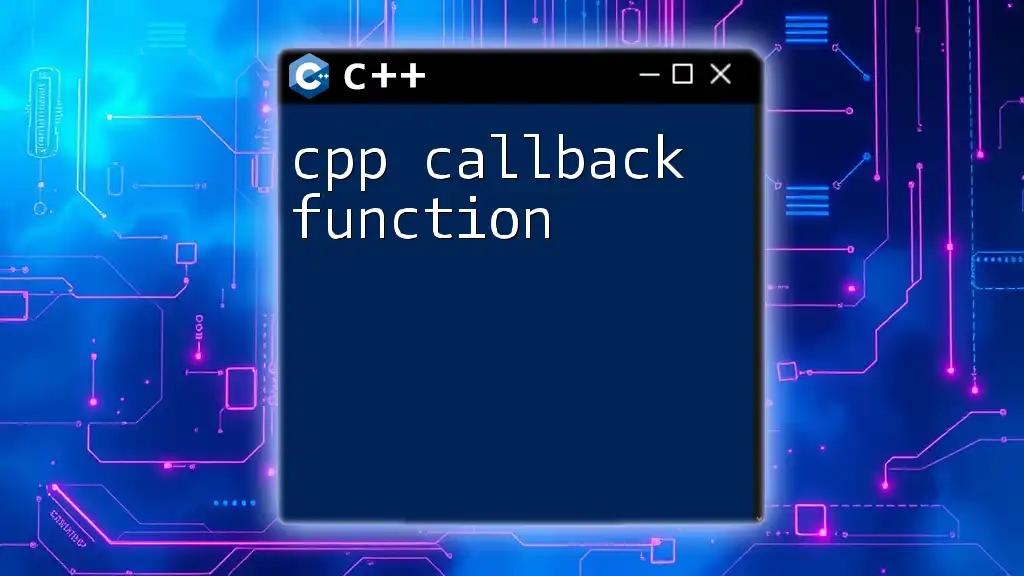
Call to Action
If you're eager to deepen your understanding of C++ and enhance your programming proficiency, subscribe to our newsletter or follow us on our social media platforms. Keep an eye out for workshops and tutorials that can provide you with hands-on experience in using C++ Shell effectively.
About the Author
With extensive experience in C++ programming and a passion for teaching, I encourage learners of all levels to embrace programming challenges. Through tools like C++ Shell, we can unlock the true potential of coding in an accessible way.