A C++ callback function is a function that is passed as an argument to another function, allowing for customizable behavior during execution.
Here's a simple code snippet demonstrating a callback function in C++:
#include <iostream>
// Callback function declaration
void callbackFunction(int x) {
std::cout << "Callback function called with value: " << x << std::endl;
}
// Function that accepts a callback
void performOperation(int value, void (*callback)(int)) {
// Perform some operation (e.g., just printing value)
std::cout << "Performing operation with value: " << value << std::endl;
// Call the callback function
callback(value);
}
int main() {
// Calling performOperation with a callback
performOperation(10, callbackFunction);
return 0;
}
Understanding the Basics of Callbacks
What is a Callback?
A callback function is a function that is passed into another function as an argument and is then invoked within that outer function. This allows for flexible and reusable code, making it possible to customize actions in response to certain events. Think of callbacks as a way to pass behavior around in your code, much like passing a recipe to someone who will cook the dish when they are ready.
How Callback Functions Work in C++
The mechanics of cpp callback functions involve two key components: registration and invocation. When you register a callback, you are effectively telling the program, "When this event occurs, call this function." When the specified event occurs, the program executes the registered callback.
In most implementations, you can use function pointers to facilitate this process. A function pointer is a variable that holds the address of a function, allowing it to be called at a later time. Understanding how to create and utilize function pointers is vital for mastering callbacks in C++.
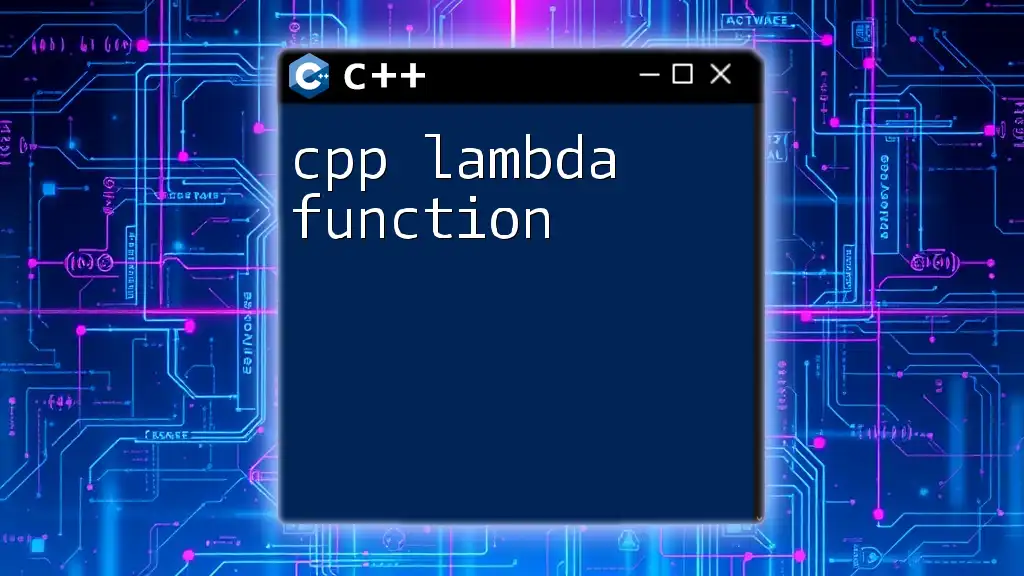
Types of Callback Functions
Function Pointer Callbacks
Function pointer callbacks are the most straightforward type of callback. They declare a function pointer that points to a function with a specific signature.
Here's an example demonstrating function pointer callbacks:
#include <iostream>
void myCallbackFunction() {
std::cout << "Callback called!" << std::endl;
}
void executeCallback(void (*callback)()) {
callback();
}
int main() {
executeCallback(myCallbackFunction);
return 0;
}
In this code, `myCallbackFunction` is passed to `executeCallback`, which calls the callback function, producing the output: `"Callback called!"`.
Member Function Callbacks
When dealing with classes in C++, you may need to work with member functions as callbacks. This requires a slight modification in how you declare and call the callback.
The following example illustrates how to use member function callbacks:
class MyClass {
public:
void myMemberFunction() {
std::cout << "Member Function Callback called!" << std::endl;
}
void executeCallback(void (MyClass::*callback)()) {
(this->*callback)();
}
};
int main() {
MyClass obj;
obj.executeCallback(&MyClass::myMemberFunction);
return 0;
}
In this case, the `executeCallback` method takes a pointer to a member function of the class. The output will be: `"Member Function Callback called!"`.
Lambda Expressions as Callbacks
Lambda expressions are a more modern C++ feature that allows for the creation of inline anonymous functions. This can lead to cleaner, more concise callback implementation, particularly when the callback logic is either very simple or specific to the context it's used in.
Here’s how to use a lambda function as a callback:
#include <iostream>
#include <functional>
void executeCallback(std::function<void()> callback) {
callback();
}
int main() {
executeCallback([]() {
std::cout << "Lambda Callback called!" << std::endl;
});
return 0;
}
The introduction of the lambda function provides significant flexibility. The output in this case is: `"Lambda Callback called!"`.
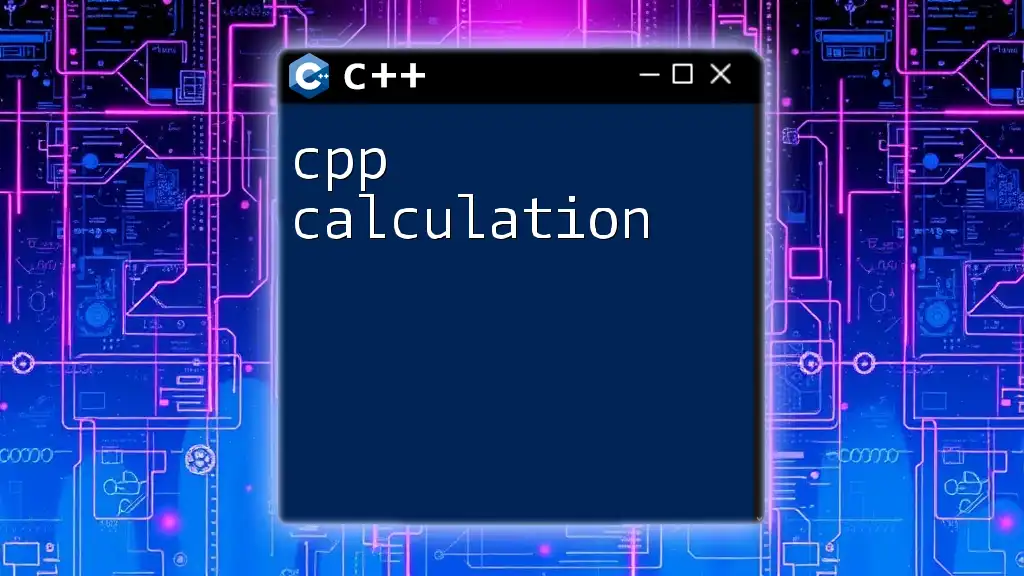
Practical Applications of C++ Callback Functions
Event Handling
One of the most prominent applications of cpp callback functions is in event-driven programming. Here, callbacks are essential for handling events in graphical user interfaces (GUIs) or in situations where actions depend on user input.
For example, when a button is clicked in a GUI, the associated callback function for that click event is invoked, allowing specific actions to occur in response to the user's actions.
Asynchronous Programming
Callbacks play a critical role in asynchronous programming, where operations occur separately from the main thread to avoid blocking the user interface. For instance, when downloading data from the internet, a program can continue executing while waiting for data to be retrieved. Once the download is complete, a callback is triggered to process the data received, ensuring a smooth user experience.
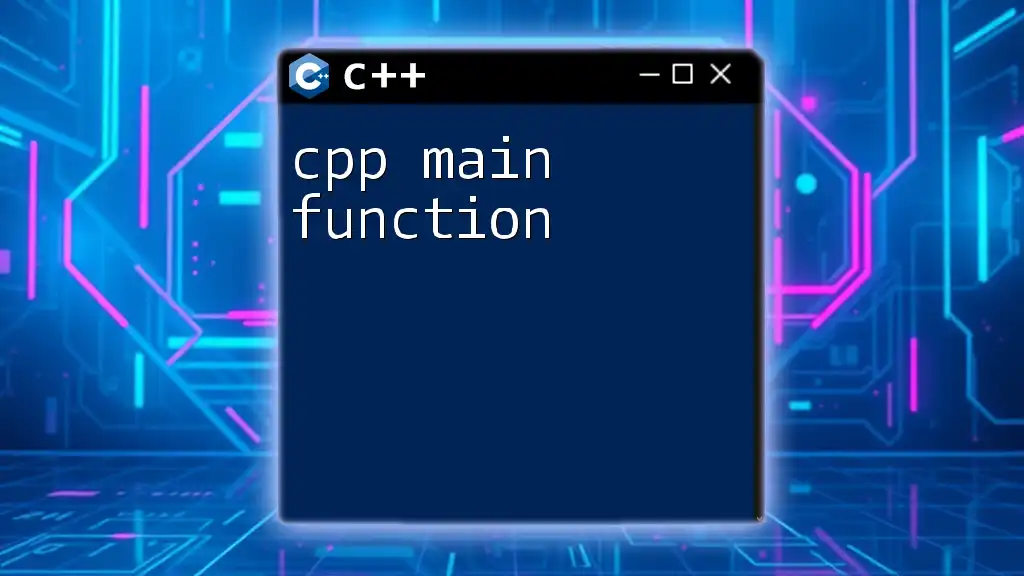
Advantages and Disadvantages of Using Callback Functions
Advantages
The use of cpp callback functions comes with several advantages:
- Code Modularity and Reusability: Callbacks allow you to write more reusable code since you can pass different callback functions to achieve different behaviors with the same codebase.
- Improved Maintainability and Readability: By isolating pieces of logic into separate functions, your program becomes easier to read and maintain.
Disadvantages
However, there are some drawbacks as well:
- Potential for Callback Hell: When callbacks are nested too deeply, the code can become hard to read and maintain, a situation often referred to as "callback hell."
- Managing Memory and Lifetime of Callbacks: It can be challenging to manage lifetimes correctly, especially when callbacks outlive the objects they reference, leading to dangling pointers.
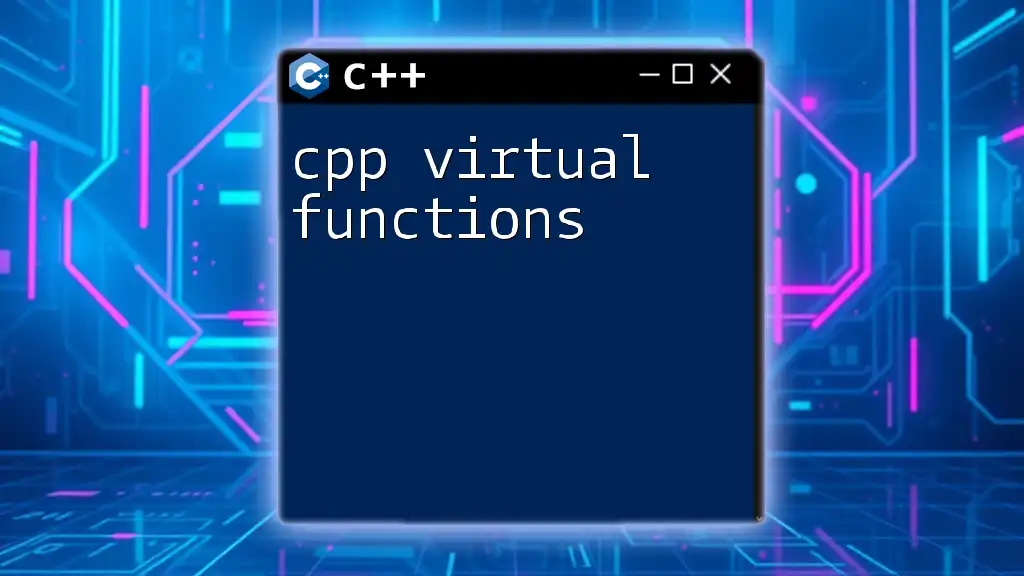
Best Practices for Implementing Callbacks in C++
Using Smart Pointers
To mitigate the issues of managing lifetimes, it’s crucial to use smart pointers. Smart pointers like `std::shared_ptr` and `std::unique_ptr` help automatically manage the memory of callback functions, ensuring that resources are properly released.
For example:
#include <iostream>
#include <memory>
#include <functional>
void executeCallback(std::function<void()> callback) {
callback();
}
int main() {
std::shared_ptr<int> resource = std::make_shared<int>(42);
executeCallback([resource]() {
std::cout << "Callback with resource: " << *resource << std::endl;
});
return 0;
}
This use of smart pointers ensures that the resource will be valid and managed correctly, avoiding issues associated with manual memory management.
Keeping Callbacks Simple
It's vital to keep your callback implementations simple and digestible. Complex, multi-layered callbacks can reduce clarity and complicate debugging. Always aim for simplicity and clarity in your callback designs. Try to limit the number of arguments necessary and ensure that the callback's responsibilities are well-defined.
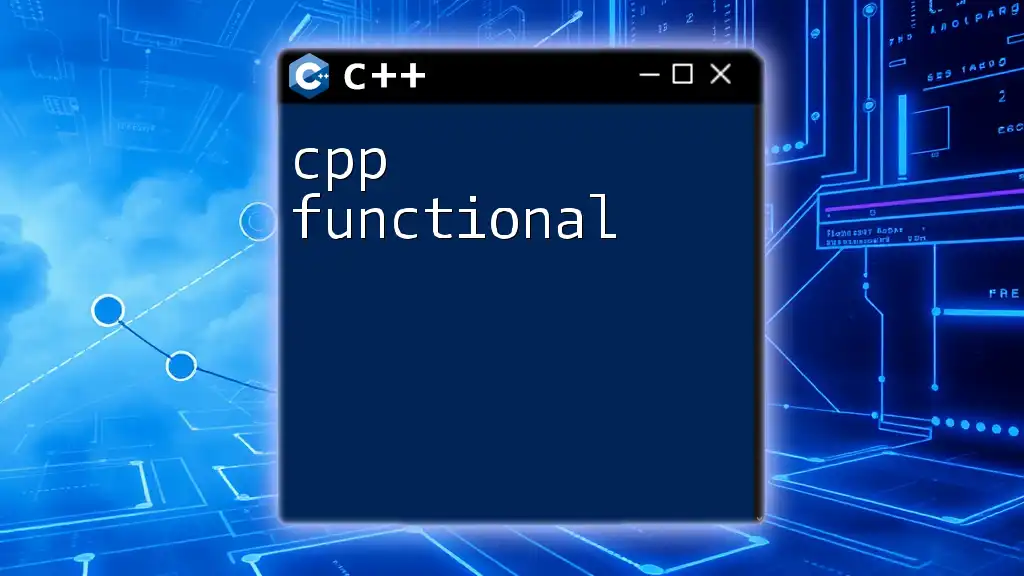
Conclusion
In summary, understanding cpp callback functions broadens your capability as a C++ programmer, allowing for significant flexibility in your code architecture. By mastering different types of callbacks—including function pointer callbacks, member function callbacks, and lambda expressions—you can significantly improve the responsiveness and modularity of your applications. Remember to consider the advantages and disadvantages of callbacks and adopt best practices like using smart pointers and keeping your codes simple to write better, more maintainable C++.