The `ceil` function in C++ rounds a floating-point number up to the nearest integer value, returning the result as a double.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cmath>
int main() {
double num = 4.2;
std::cout << "Ceil of " << num << " is " << std::ceil(num) << std::endl;
return 0;
}
What is the Ceil Function in C++?
The ceil function in C++ is a mathematical utility that is used to round a floating-point number up to the nearest integer. This means that if the number has any fractional component, the ceil function will increase the number to the next whole number. For instance, using the ceil function on `2.3` will result in `3`, while using it on `-2.3` will yield `-2`. Understanding this function is crucial, especially in scenarios where rounding up is required.
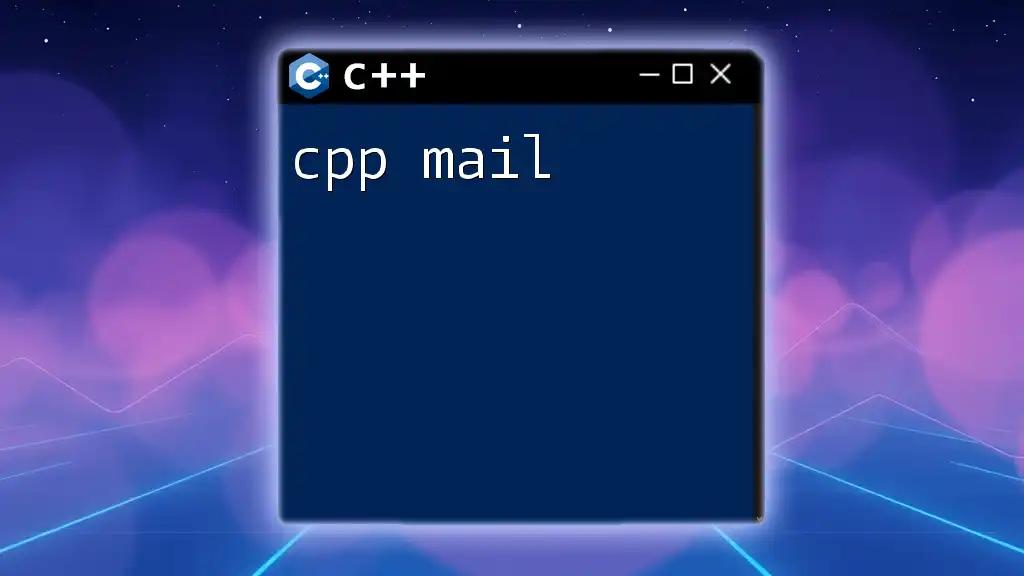
Syntax of the C++ Ceil Function
The syntax of the ceil function is straightforward:
double ceil(double x);
Here, `x` is the number that you wish to round up. It is important to note that ceil returns a type of `double`, even when the result is a whole number.
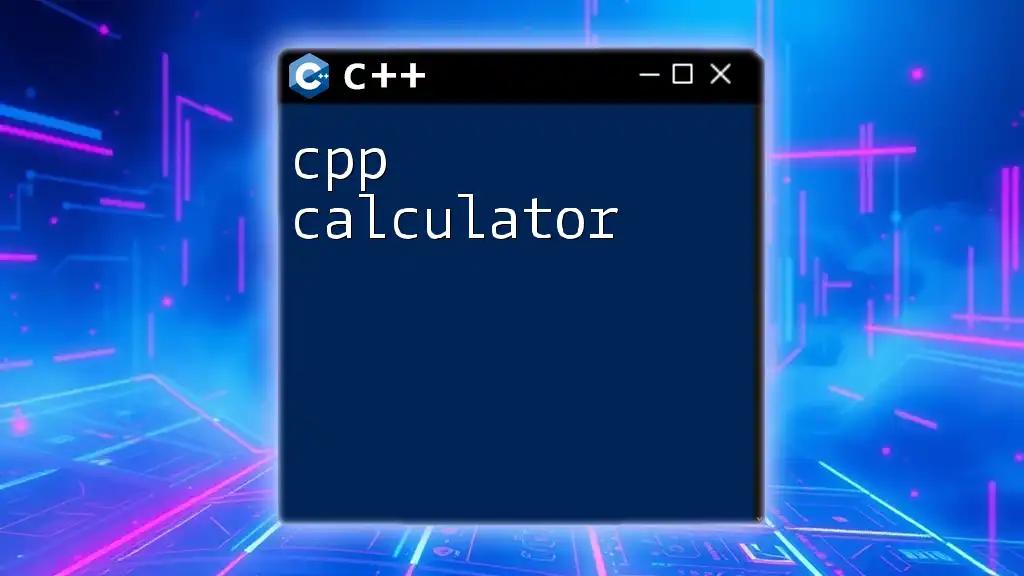
Including the Required Header File
To utilize the ceil function, you must include the `<cmath>` header file at the beginning of your program. This header file contains various mathematical functions, including ceil.
#include <cmath>
Failing to include this header will result in a compilation error when you attempt to use the ceil function, as the compiler won't recognize the function.
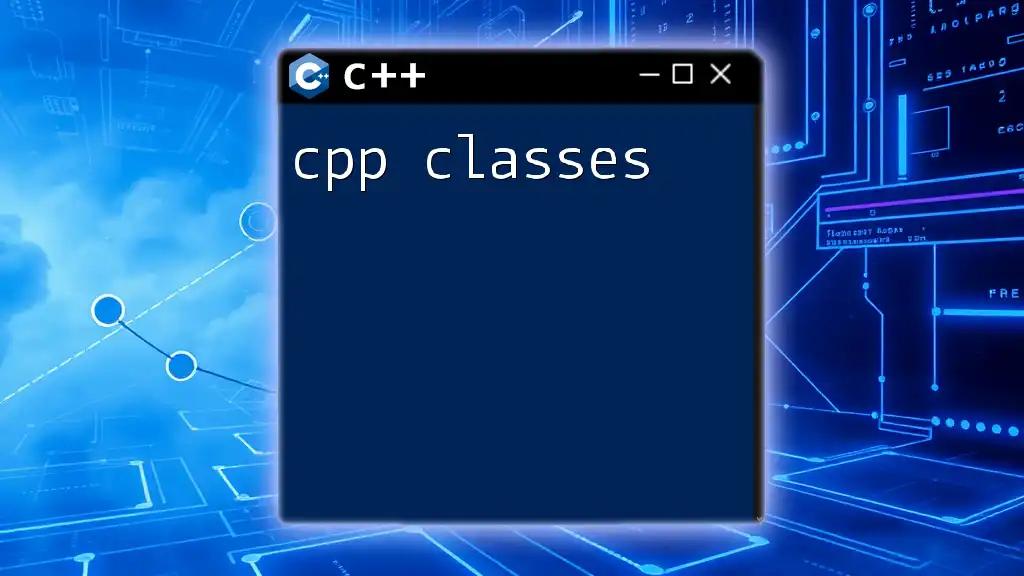
How to Use Ceil in C++
Using the ceil function in your code is quite straightforward. Here’s a step-by-step look at a simple application:
- Include the necessary header files.
- Declare and initialize a floating-point variable that you wish to round up.
- Call the ceil function and store its return value.
- Output the result.
Here’s an example demonstrating this:
#include <iostream>
#include <cmath> // Include the cmath header for mathematical functions
int main() {
double num = 2.3;
double result = ceil(num);
std::cout << "Ceil of " << num << " is " << result << std::endl; // Output: 3
return 0;
}
In this example, `2.3` is rounded to `3`, showcasing the basic functionality of ceil.
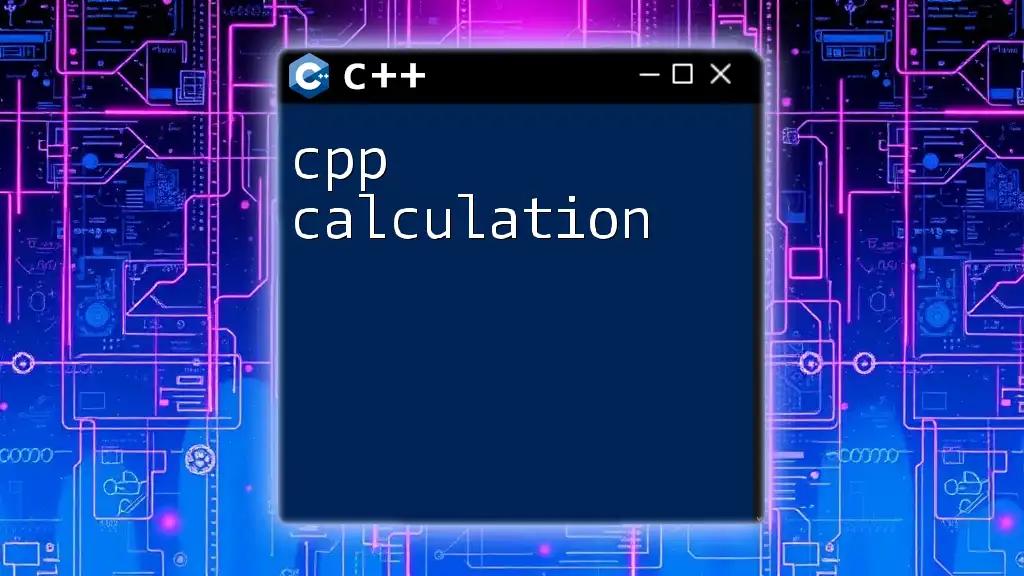
Examples of Using the C++ Ceil Function
Basic Example
To further illustrate the workings of the ceil function, let’s consider another simple example:
double val1 = 1.2;
std::cout << "Ceil(" << val1 << ") = " << ceil(val1) << std::endl; // Output: 2
In this case, `1.2` is rounded up to `2`.
Multiple Examples
Here are several more examples showcasing various inputs to the ceil function:
std::cout << "Ceil(4.8) = " << ceil(4.8) << std::endl; // Output: 5
std::cout << "Ceil(-2.5) = " << ceil(-2.5) << std::endl; // Output: -2
std::cout << "Ceil(0.0) = " << ceil(0.0) << std::endl; // Output: 0
Through these examples, it’s clear that the ceil function consistently rounds towards positive infinity, regardless of whether the input is positive or negative.
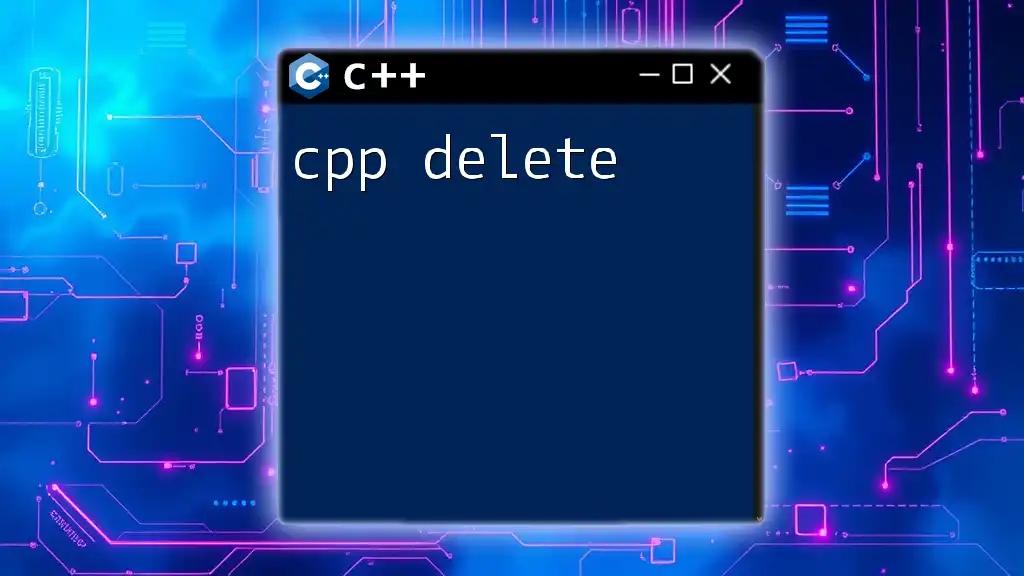
Common Use Cases for the Ceil Function in C++
The ceil function finds its application in various domains. Here are a few common scenarios:
-
Financial Calculations: When calculating payments that cannot be fractional, such as splitting bills among friends or calculating interest rates, using the ceil function ensures that one does not undercharge.
-
Resource Allocation: In programming environments, ceil may be used when determining the required memory blocks or handling pagination in databases to ensure that all items fit appropriately.
-
Gaming Mechanics: Developers may use the ceil function to allocate resources in games. For example, if a character can earn fractional skill points, rounding up may make for a more fair gameplay experience.
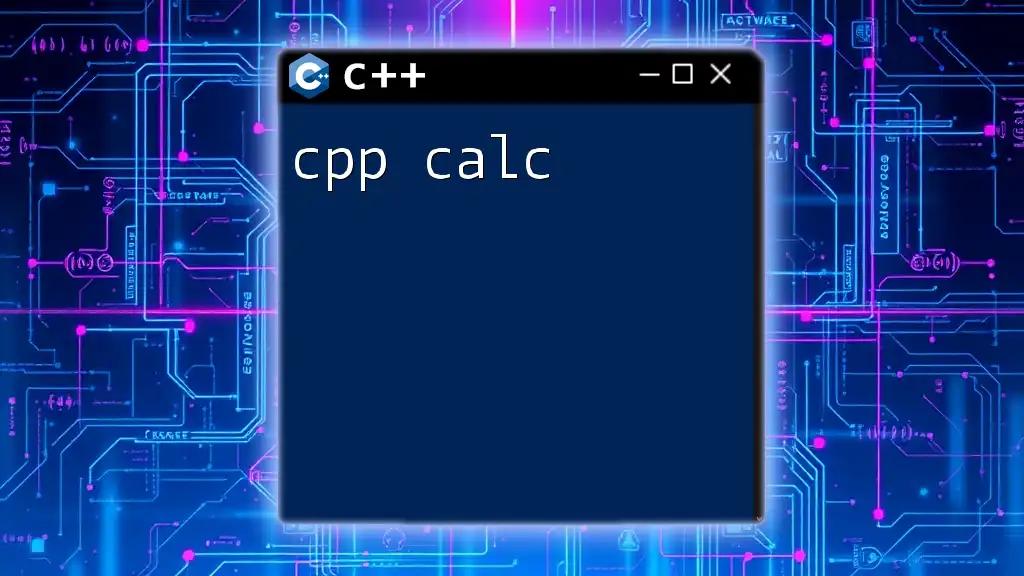
Performance Considerations
When using the ceil function, it’s essential to consider its performance. The ceil function is typically efficient, but comparing it with other rounding methods such as floor, round, or trunc can yield different performance metrics based on your application.
Be mindful of data types as well; for instance, calling ceil on an `int` will require implicit type conversion to `double`, potentially adding a minor overhead. Optimizing your usage according to the data types involved can lead to better performance, particularly in computationally intensive applications.
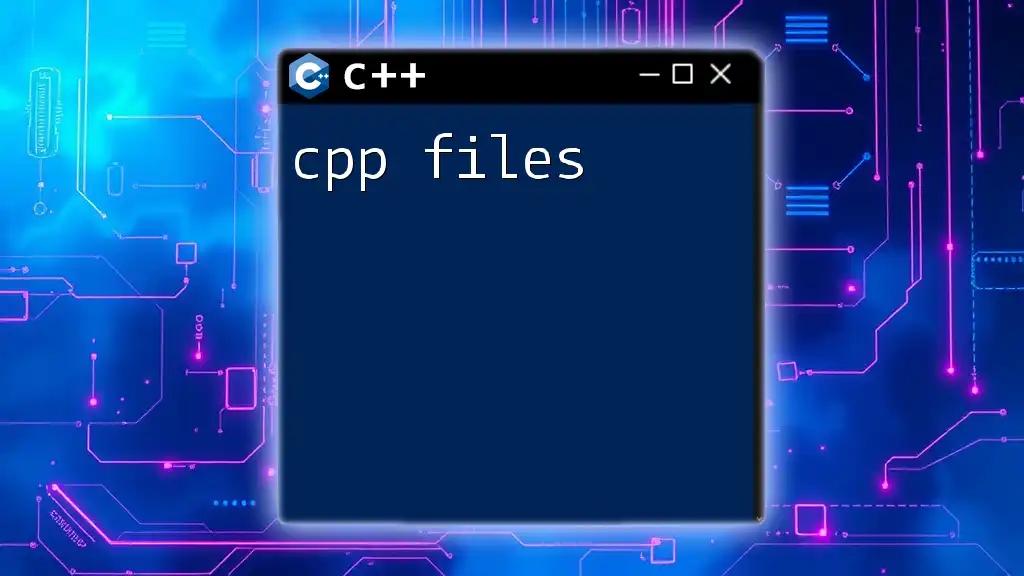
Troubleshooting Common Issues
As you integrate the ceil function into your C++ code, you may encounter a few common issues:
-
Missing Header File: Ensure that you have included `<cmath>`. Omitting this header will lead to an 'undeclared identifier' error.
-
Floating Point Precision: Keep in mind that floating-point numbers can sometimes yield unexpected results due to how they are represented in memory. This can occasionally affect the outcome of the ceil function. Always verify your expectations with direct outputs.
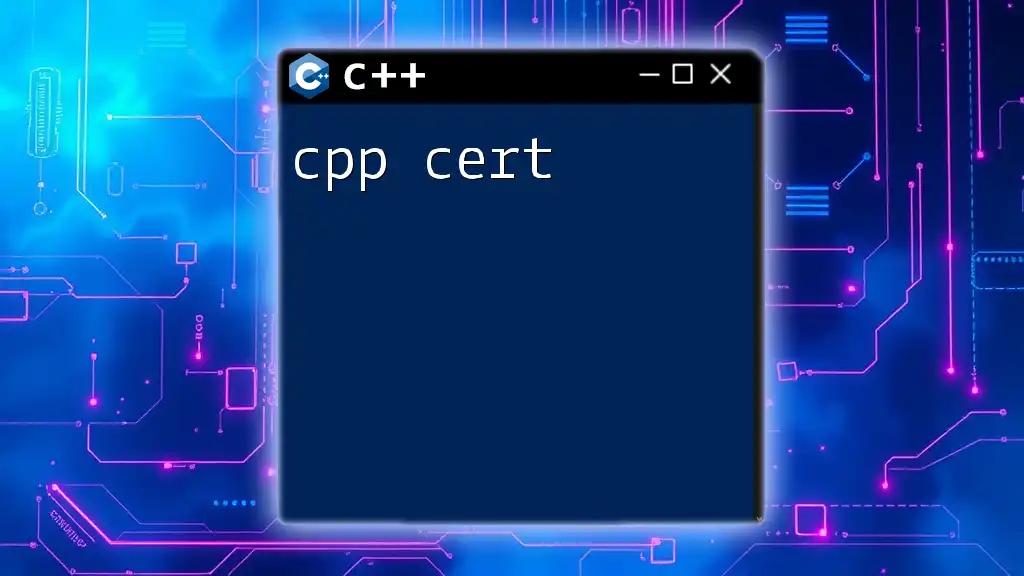
Conclusion
The cpp ceil function is a vital tool in any C++ programmer's toolbox, enabling precise rounding of floating-point numbers. Whether you're working on financial applications, resource allocation, or game development, understanding how to effectively use the ceil function can greatly enhance the reliability and accuracy of your code. Experiment with it in various scenarios to see firsthand how it can simplify mathematical operations in your projects.
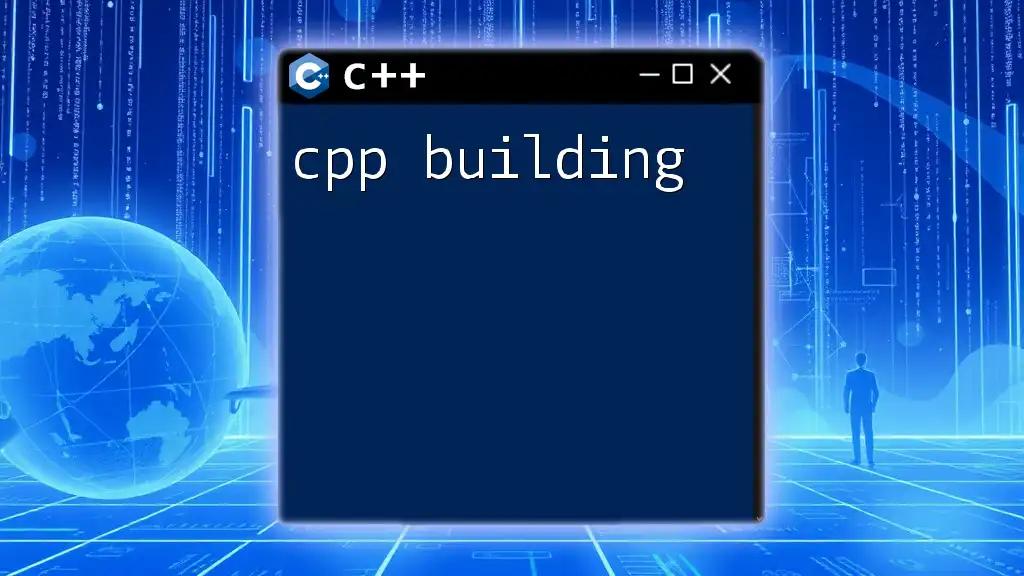
Additional Resources
For further exploration of the ceil function and other related mathematical functions, consider checking out official documentation such as the C++ Standard Library documentation and other reputable C++ programming courses. These resources can provide greater insights and practical exercises to sharpen your C++ skills.