C++ files, commonly with a ".cpp" extension, are source code files that contain C++ program instructions which are compiled to create executable programs.
Here's a simple example of a C++ program in a `.cpp` file:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Files
What are .cpp Files?
.cpp files are the primary source code files for C++ programs. The ".cpp" extension indicates that the file contains C++ code, which is meant to be compiled into executable programs. These files play a crucial role in the development process, as they contain the actual implementation of functionality that is often declared in header files (.h or .hpp).
Understanding the distinction between .cpp files and other file types is key for any C++ developer. While header files are typically used to declare functions, classes, and variables to be shared across multiple .cpp files, .cpp files contain the implementation of these declarations. This separation helps in better organizing code and avoiding compilation issues.
The Role of .cpp Files in C++ Projects
In a typical C++ project, the structure often includes folders for source code, headers, and binaries. The .cpp files act as the main building blocks, holding the logic and functionality required to make the application run. During the compilation process, these .cpp files are transformed into an executable, integrating with the other components of the project.
For instance, when you compile a project, the compiler takes each .cpp file, processes the code for syntax and errors, and generates an object file, which is then linked with other object files and libraries to create an executable.
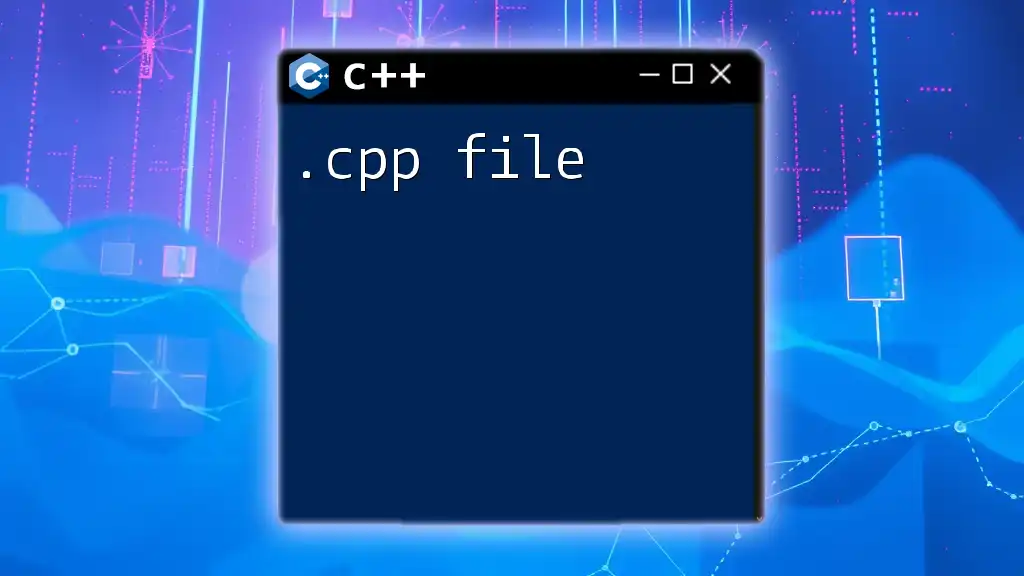
Creating and Managing .cpp Files
Setting Up Your Development Environment
To get started with .cpp files, choose an Integrated Development Environment (IDE) that suits your needs. Popular options include Visual Studio, Code::Blocks, and CLion. You can also use text editors such as Visual Studio Code or Sublime Text.
Creating a new .cpp file is straightforward. In most IDEs, you typically go to the menu and select the option to add a new source file. This action will allow you to choose the .cpp format.
Directory Structure and Organization
When working on larger projects, organization becomes crucial. It’s important to follow best practices for structuring your .cpp files. One common approach is to use separate folders for different modules or libraries within your project. For example, you might have a `src` directory for your .cpp files and an `include` directory for your header files.
A well-structured project directory could look like this:
/MyProject
/src
main.cpp
utils.cpp
/include
utils.h
This structure helps you manage dependencies and maintain the code effectively.
Basic Syntax in .cpp Files
A basic .cpp file typically includes certain essential components: include statements, namespace declarations, and the main function. Here’s a simple example of a minimal C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` allows the program to utilize input and output streams such as `std::cout`. The `main` function serves as the entry point of any C++ program, returning an integer, usually 0 to indicate successful completion.
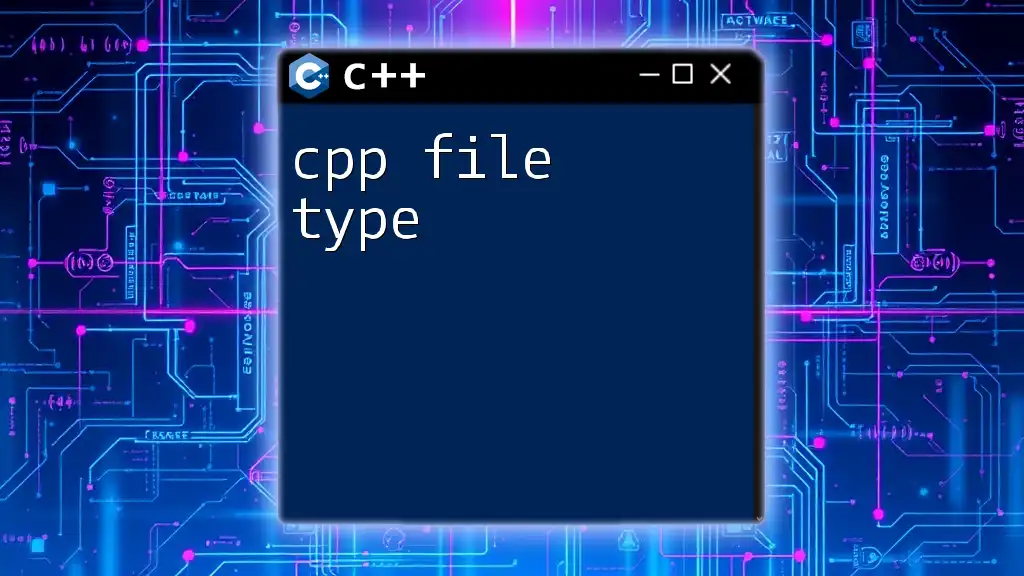
Compiling and Running C++ Files
The Compilation Process Explained
Compiling a .cpp file involves several stages: preprocessing, compilation, assembly, and linking. During preprocessing, directives (like `#include`) are handled. The compilation stage translates your code into assembly language, while the assembly stage creates machine code. Finally, linking combines object files and libraries into a single executable.
How to Compile .cpp Files with Command Line
If you prefer the command line, you can use the GNU Compiler Collection (g++) to compile your .cpp files easily. Here’s how to do it step by step:
- Open your terminal or command prompt.
- Navigate to the directory containing your .cpp file.
- Run the following command to compile your program:
g++ example.cpp -o example
In this command, `example.cpp` is your source file, and `-o example` tells the compiler to name the output executable `example`.
Running Compiled Programs
After compiling, you will have an executable file ready to run. To execute the program, use the command:
./example
This command will run the program, and if everything is correct, you should see the output in your terminal.
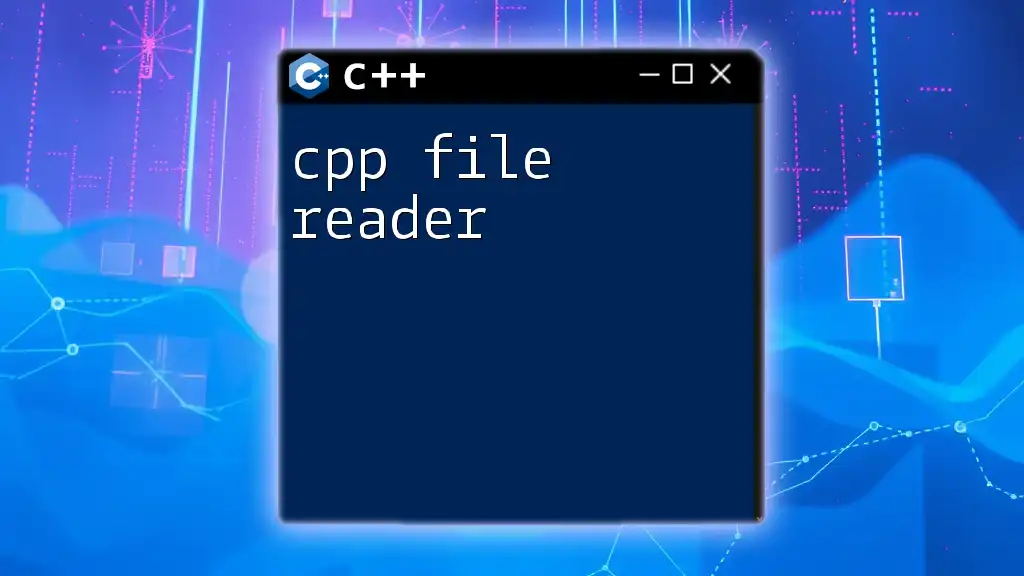
Advanced Concepts Related to .cpp Files
Header Files vs. Source Files
Header files (.h or .hpp) are crucial for C++ programs, as they declare functions, classes, and constants that can be used across multiple .cpp files. Including header files in your .cpp files allows for modular development and promotes code reuse.
To include a header file within a .cpp file, you use the `#include` directive, such as:
#include "myHeader.h"
Using Multiple .cpp Files in a Project
When a project grows beyond a single .cpp file, managing multiple files becomes necessary. Each module of functionality can be placed in its own .cpp file, and these files can be linked together during compilation.
If you have multiple source files, you can compile them together by running:
g++ main.cpp utils.cpp -o program
This command combines both `main.cpp` and `utils.cpp`, leading to the creation of a single executable named `program`.
Debugging Your C++ Code
Debugging is an integral part of programming. You can catch and fix issues in your .cpp files using debugging tools like gdb or built-in IDE debuggers. To debug a program compiled with g++, you can run:
gdb ./program
This command starts the gdb debugger, allowing you to set breakpoints, step through your code, and observe variable values.
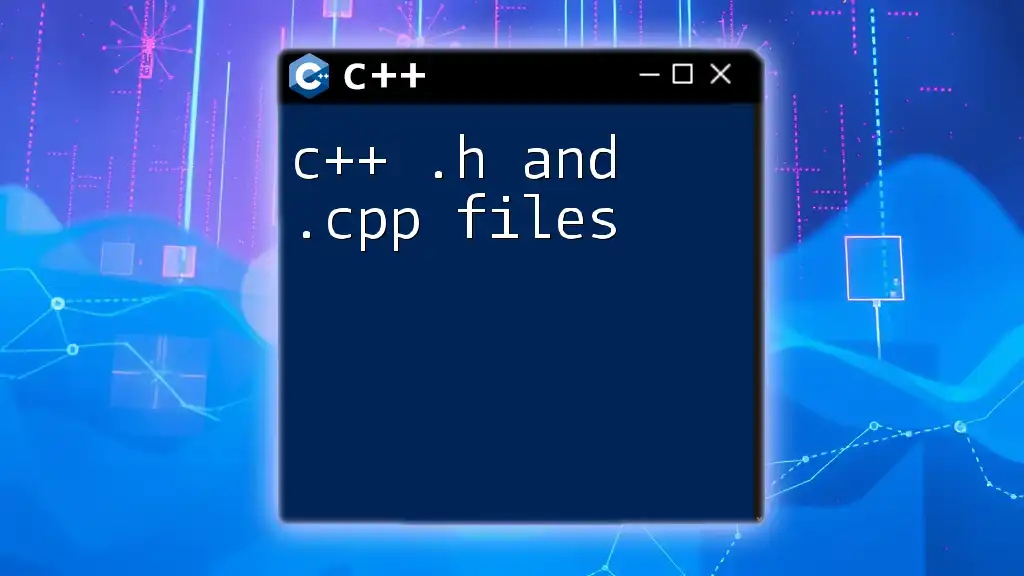
Common Issues with .cpp Files
Compilation Errors and Their Solutions
Compilation errors are commonplace in C++ programming, especially for beginners. Common issues include syntax errors, undeclared variables, or missing headers. Reading the error messages can help pinpoint what needs to be corrected.
For example, if you encounter an error stating a variable is undeclared, double-check your variable spelling and ensure it is declared before use.
Runtime Errors: The Need for Error Handling
Runtime errors occur during program execution and can lead to unexpected behavior or crashes. To manage these errors effectively, implement error handling using `try` and `catch` blocks, which allow your program to respond gracefully to exceptions:
try {
// Code that may throw an exception
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
In this code snippet, the `try` block contains code that might throw an exception, while the `catch` block captures and handles it.
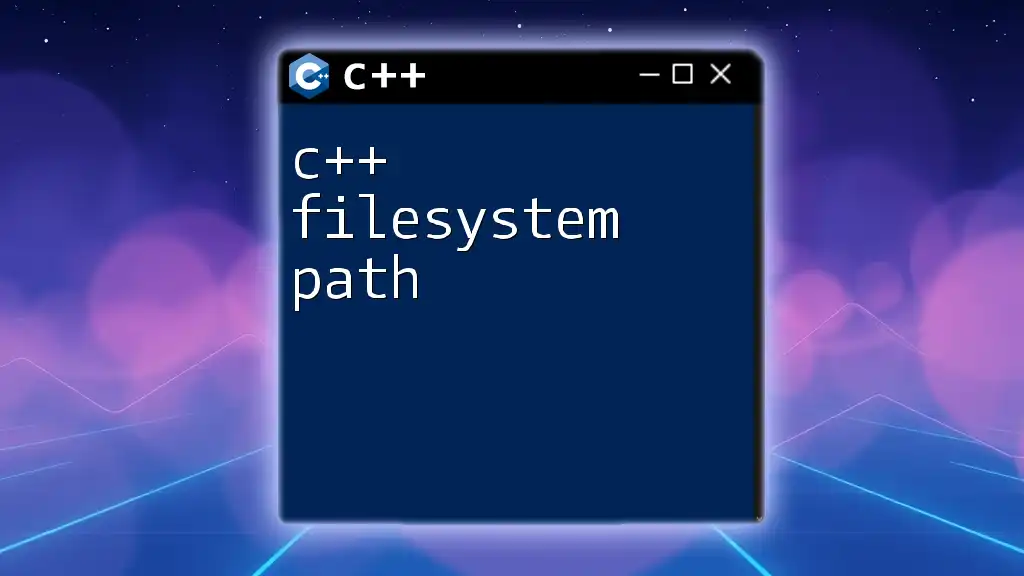
Best Practices for Working with .cpp Files
Coding Standards and Style Guides
Adhering to coding standards is important for maintaining readable and consistent code. Style guides like Google C++ Style and LLVM Style provide valuable insights on naming conventions, formatting, and organization, helping developers write cleaner code.
Comments and Documentation
Adding comments to your .cpp files is vital for improving code readability. Use inline comments for clarity and consider tools like Doxygen to generate documentation from your comments, making it easier to understand how your code works.
Version Control
In any development project, using version control systems like Git is essential for tracking changes, collaborating with others, and maintaining project history. To initialize a Git repository for your C++ project, run the following command in your project directory:
git init
This command sets up a new Git repository, allowing you to start tracking your changes with ease.
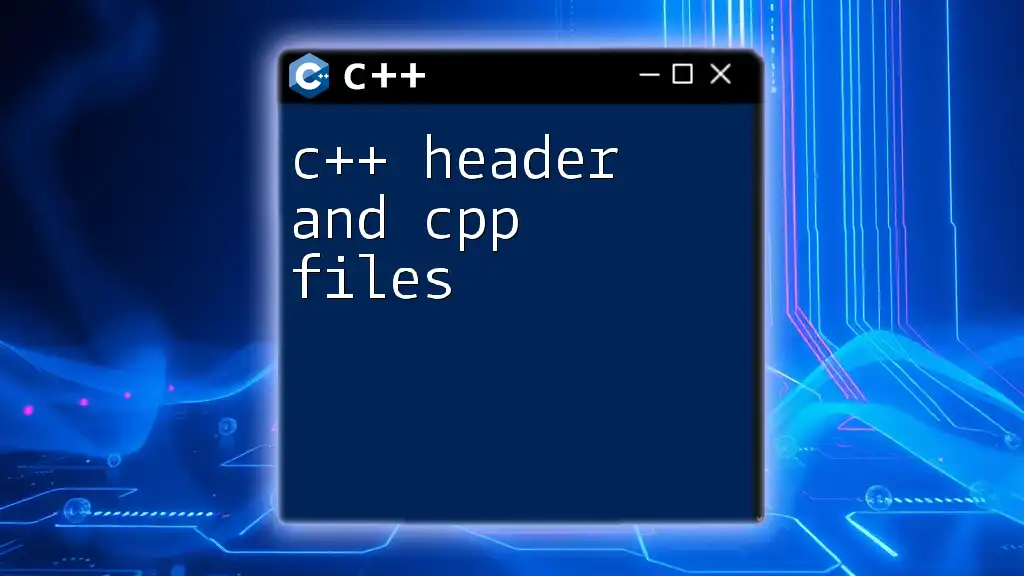
Conclusion
Summary of the Key Takeaways
In conclusion, understanding .cpp files is fundamental for anyone looking to dive into C++ programming. From their creation and management to compiling and debugging, each step is crucial for developing functional, efficient applications.
Call to Action
If you're eager to enhance your C++ skills further, consider joining our courses or webinars. Explore additional resources on C++ programming, and elevate your coding proficiency today!