In C++, header files (`.h`) are used to declare the interfaces of classes and functions, while implementation files (`.cpp`) contain their definitions and logic.
Here’s a simple example demonstrating this:
// File: example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
class Example {
public:
void sayHello();
};
#endif // EXAMPLE_H
// File: example.cpp
#include <iostream>
#include "example.h"
void Example::sayHello() {
std::cout << "Hello, World!" << std::endl;
}
Understanding C++ Files: The Basics
What is a C++ File?
A C++ file is essential for writing, compiling, and executing C++ programs. These files serve various purposes and are commonly split into two types: `.h` files and `.cpp` files. The key difference lies in their functions; while `.h` files are used for declarations, `.cpp` files are used for implementations.
Why Use `.h` and `.cpp` Files?
The primary benefit of using `.h` and `.cpp` files is to facilitate modular programming. By separating declarations from definitions, you improve the readability, reusability, and maintainability of your code. This structure allows different parts of the program to be developed independently while ensuring a clean interface for interaction.
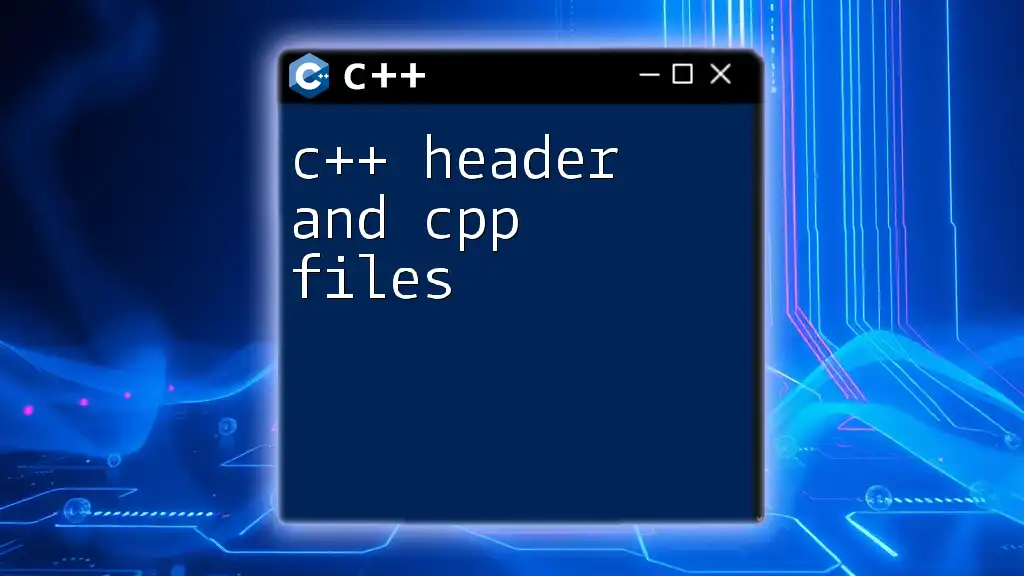
The Structure of C++ Files
The `.h` File: Interface Definition
What Goes Inside a Header File?
A header file typically contains:
- Class declarations: Defines the attributes and methods.
- Function prototypes: Outlines functions without providing their implementations.
- Constants and macros: Allows constant values to be reused.
This organization clarifies the public interface, making it easier for other files to interact with its contents without needing implementation details.
Example of a Simple Header File
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
class Example {
public:
void doSomething();
};
#endif // EXAMPLE_H
In this example, `#ifndef`, `#define`, and `#endif` create header guards, preventing multiple inclusions of the same header in a single compilation unit, which is crucial in avoiding compilation errors.
The `.cpp` File: Implementation Details
What Happens in a Source File?
A source file contains the actual definitions for the functions declared in the header file, implementing the functionalities. When working with source files, it is also essential to include the relevant header files, ensuring that you have access to the declarations needed for the definitions.
Example of a Simple Source File
// example.cpp
#include "example.h"
#include <iostream>
void Example::doSomething() {
std::cout << "Doing something!" << std::endl;
}
In this code snippet, `#include "example.h"` pulls in the interface defined in the header, allowing the `doSomething` function to be completed.
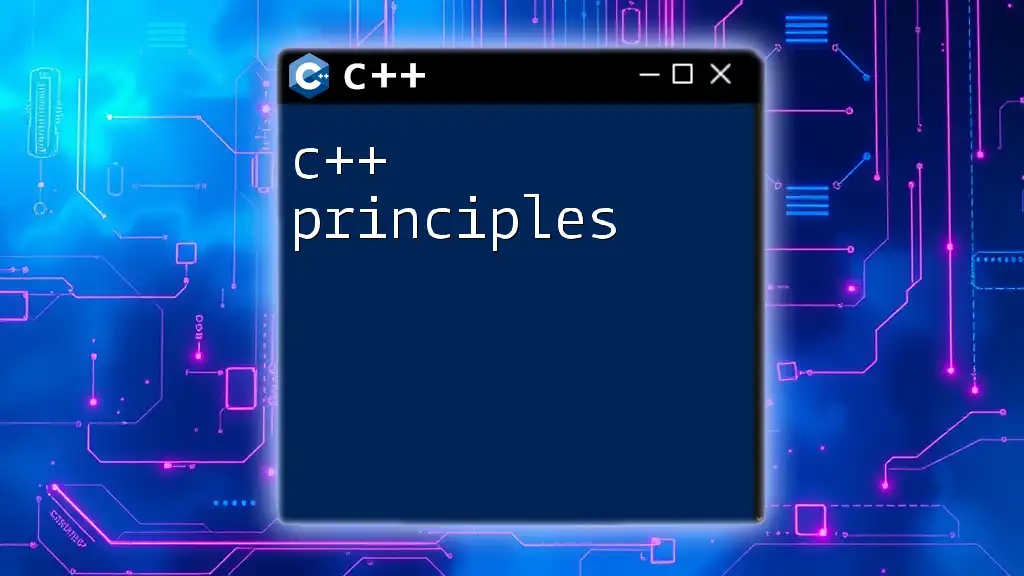
Creating a C++ Project with `.h` and `.cpp` Files
Organizing Your C++ Project
Creating a well-structured directory for your C++ project is crucial for managing complexity. Here are some best practices:
- Separate folders for headers and source files: Keeps the project organized.
- Consistent naming conventions: Use clear and descriptive names for files and classes.
This organization simplifies the navigation of your codebase and enhances collaboration when working in teams.
Compiling and Linking C++ Files
Compilation Process
When handling C++ files, the compilation process goes through several steps:
- The C++ preprocessor processes directives (like `#include`).
- The compiler compiles the `.cpp` files and generates object files.
- The linker combines all the object files into a final executable.
It’s important for header guards to be included in your header files, as they prevent the C++ preprocessor from including the same file multiple times, which can lead to conflicts.
Practical Example of Compilation
To compile a project with multiple C++ files, you can use:
g++ main.cpp example.cpp -o my_program
In this command, `main.cpp` is usually the entry point of your program and serves as a launching point for your application.
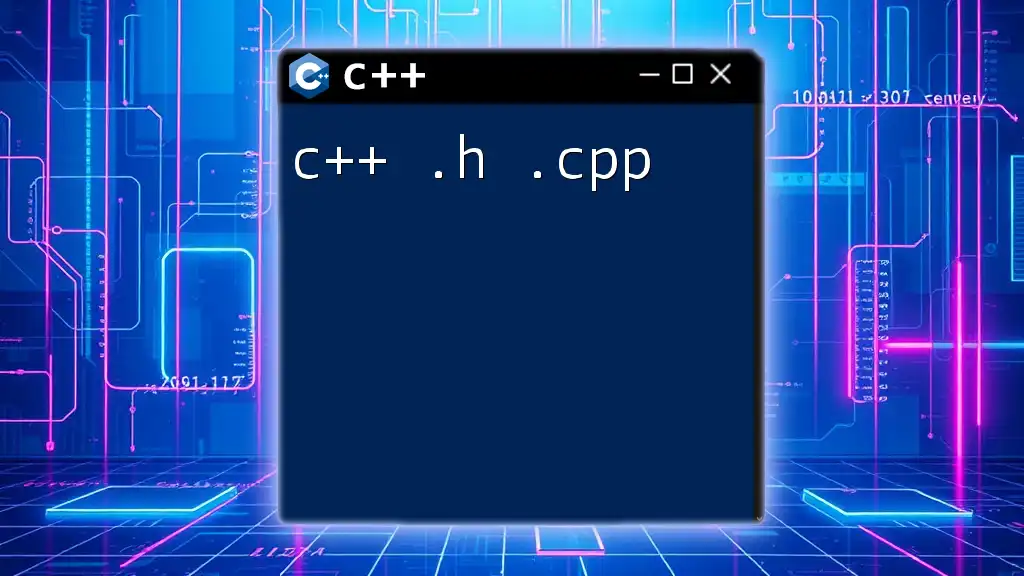
Advanced Topics in C++ File Management
Forward Declarations vs. Includes
Forward declarations are declarations of classes or functions that inform the compiler that a type exists before its actual definition appears. This technique can significantly reduce dependencies and improve compilation times, especially in large projects.
Best Practices for Header Files
When creating header files, consider the following best practices:
- Use inline functions for small, performance-critical functions to reduce overhead.
- Carefully manage static and extern variables based on their scope and usage.
- Group related classes and functions within the same header file to encapsulate functionality better.
Using Namespaces
Namespaces are a vital feature in C++ that helps organize code and prevent name clashes. You can define a namespace in your `.h` and `.cpp` files to wrap related functionalities.
Example:
// example.h
namespace MyNamespace {
class Example {
public:
void doSomething();
};
}
// example.cpp
#include "example.h"
#include <iostream>
void MyNamespace::Example::doSomething() {
std::cout << "Doing something in MyNamespace!" << std::endl;
}
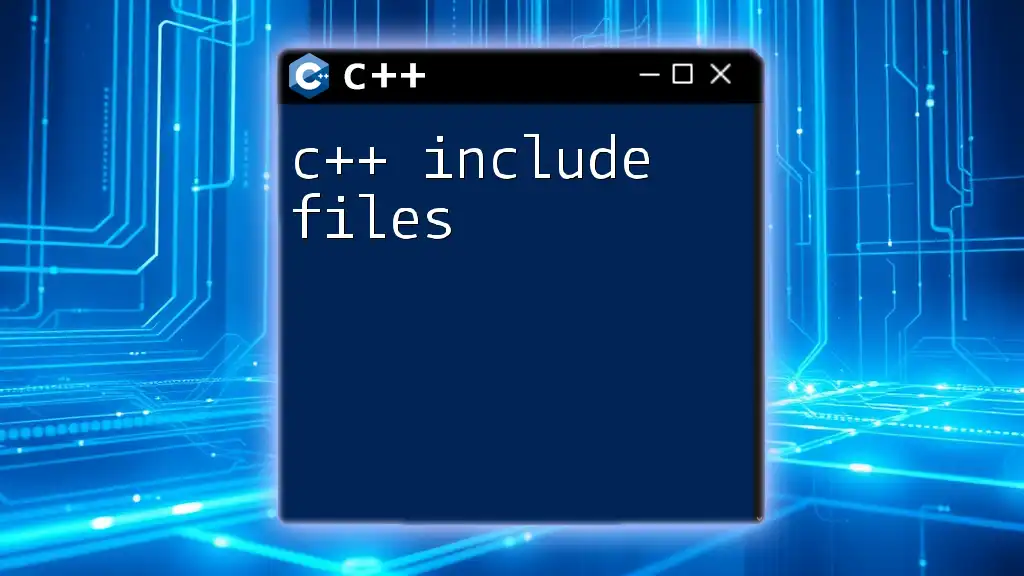
Common Issues and Debugging Tips
Common Mistakes with `.h` and `.cpp` Files
Even seasoned developers may encounter common pitfalls:
- Multiple header inclusions can lead to redefinition errors.
- Incomplete or incorrect inclusion of necessary headers can result in unresolved references during linking.
Debugging Compilation Errors
When facing compilation errors related to `.h` and `.cpp` files:
- Utilize compiler flags to provide more detailed error messages.
- Review the order of header inclusions to ensure dependencies are correctly managed.
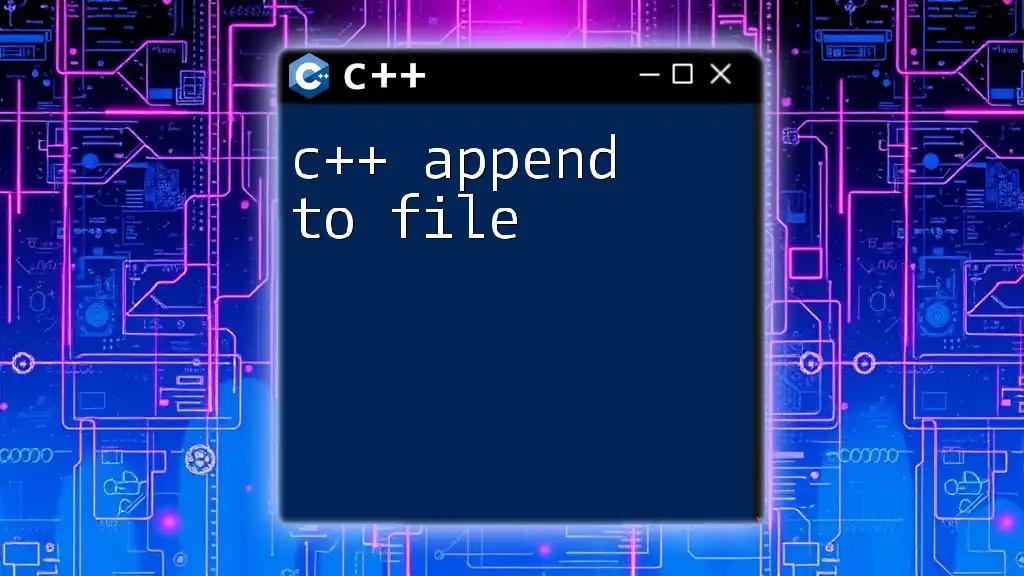
Conclusion
In summary, understanding the roles of C++ `.h` and `.cpp` files is crucial for effective C++ programming. By separating declarations and definitions, you not only enhance code organization but also improve maintainability. Practicing these concepts through projects will solidify your understanding and skills in C++. Remember that the journey of mastering C++ is ongoing, and hands-on experience is essential for true proficiency.
Additional Resources
To further develop your knowledge on C++ programming and file management, consider exploring recommended books, websites, and online courses specific to these topics.