C++ examples are practical snippets of code that demonstrate specific functionalities or commands within the C++ programming language, allowing learners to grasp concepts quickly and effectively.
Here’s a simple example that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Basic C++ Syntax
What is Syntax in C++?
In C++, syntax refers to the rules that define the structure of the programs. Understanding syntax is crucial, as it dictates how code lines should be written for them to be successfully compiled and executed. Small errors in syntax can lead to compilation failures or unpredictable behavior in programs.
Example of Basic Syntax
Consider the following simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, we break down its components:
- `#include <iostream>`: This line tells the compiler to include the standard input-output stream library, enabling the use of `std::cout` for printing to the console.
- `int main()`: This defines the main function where the program execution begins.
- Inside the curly braces `{}`, we have the actual code executed: `std::cout << "Hello, World!" << std::endl;`, which prints "Hello, World!" to the console.
- `return 0;` indicates successful termination of the program.
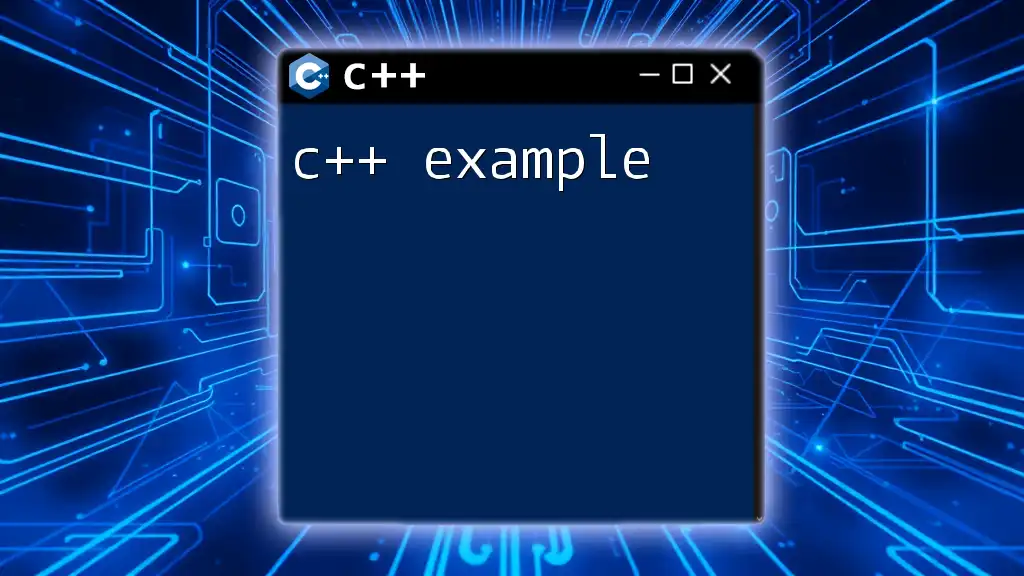
Variables and Data Types in C++
Declaring Variables
In C++, declaring a variable means specifying its data type and optionally initializing it. Variables act as containers for holding data that can be manipulated by the program.
Common Data Types in C++
Some common data types in C++ include:
- int: Represents integers (whole numbers).
- double: Represents floating-point numbers (numbers with decimals).
- char: Represents a single character.
- bool: Represents boolean values (`true` or `false`).
Here is an example demonstrating variable declaration:
int age = 25;
double salary = 50000.50;
char initial = 'A';
bool isCPPFun = true;
In this code, we declare an integer variable `age`, a double variable `salary`, a character variable `initial`, and a boolean variable `isCPPFun`, showcasing multiple data types in a single snippet.
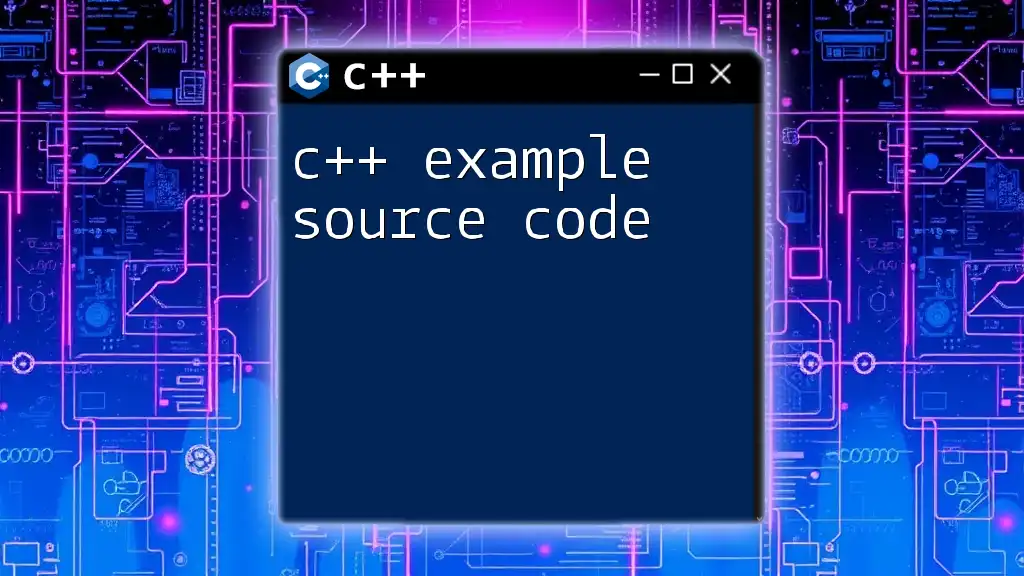
Control Structures in C++
Conditional Statements
Conditional statements in C++ allow the program to make decisions based on certain conditions. The most common ones include `if`, `else if`, and `else`.
Here’s an example of a conditional statement:
int num = 10;
if (num > 0) {
std::cout << "Positive Number";
} else {
std::cout << "Non-Positive Number";
}
In this case, the program evaluates `num`. If it’s greater than 0, it prints "Positive Number." Otherwise, it prints "Non-Positive Number."
Loops in C++
Loops are fundamental for executing a block of code multiple times. The two significant types in C++ are `for` loops and `while` loops.
For Loop
The `for` loop is used for iterating over a sequence, such as a range of numbers. Here is a simple implementation:
for (int i = 0; i < 5; ++i) {
std::cout << i << std::endl;
}
This loop prints the numbers 0 through 4, demonstrating how to control the execution flow effectively.
While Loop
The `while` loop continues executing as long as a specified condition remains true. Here's how it works:
int i = 0;
while (i < 5) {
std::cout << i << std::endl;
++i;
}
In this snippet, the loop continues to print `i` until it reaches 5, making it a useful control structure for scenarios where the number of iterations is unknown beforehand.
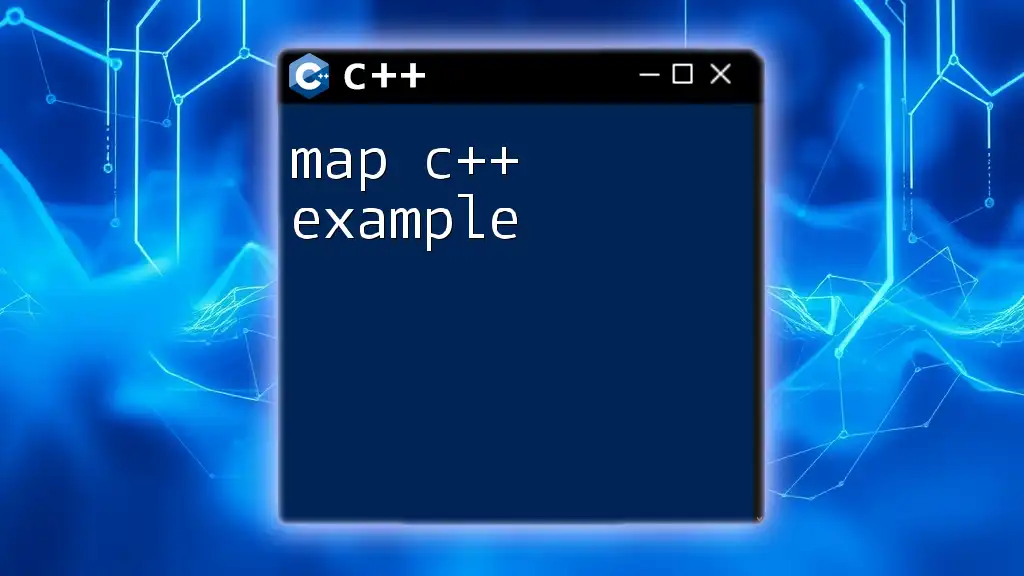
Functions in C++
Defining Functions
Functions are blocks of code designed to perform specific tasks, promoting code modularity and reusability.
Here is an example of a function definition:
int add(int a, int b) {
return a + b;
}
This `add` function takes two integers as parameters and returns their sum.
Using Functions
To call a function, simply use its name and pass the required parameters. Here's how you can utilize the `add` function:
std::cout << add(3, 4); // Output: 7
The output demonstrates how functions encapsulate behavior, making the code easier to read and maintain.
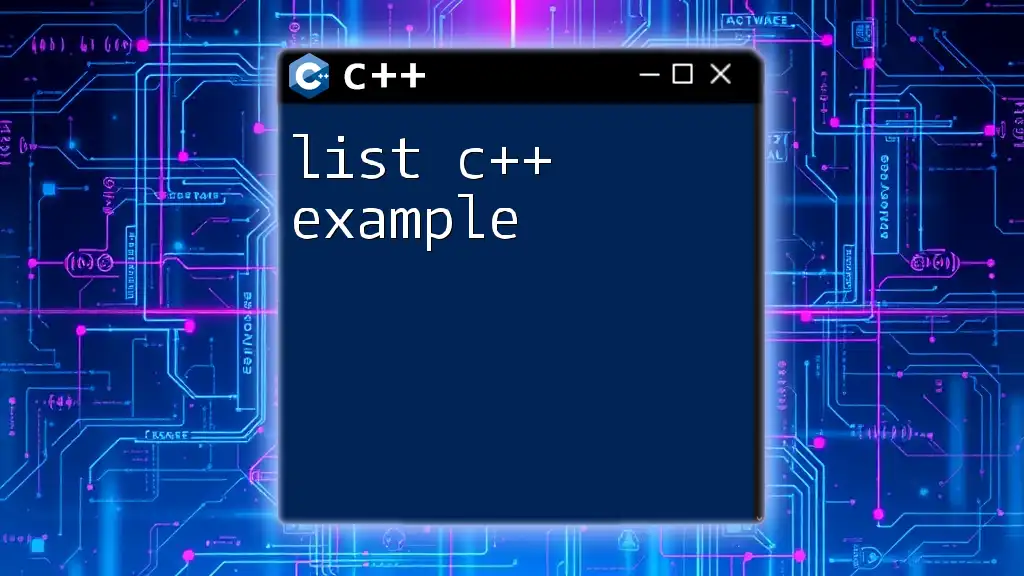
Working with Arrays and Vectors
Introduction to Arrays
Arrays are collections of data items of the same type. They provide a way to store multiple elements in a single variable.
Here’s a simple array declaration:
int numbers[5] = {1, 2, 3, 4, 5};
This declares an array named `numbers` that holds five integers.
Using Vectors
Vectors are dynamic arrays that can resize themselves, making them more flexible compared to fixed-size arrays.
Here's how to declare and utilize a vector:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
Vectors allow you to add or remove elements dynamically, further enhancing the functionality available in arrays.
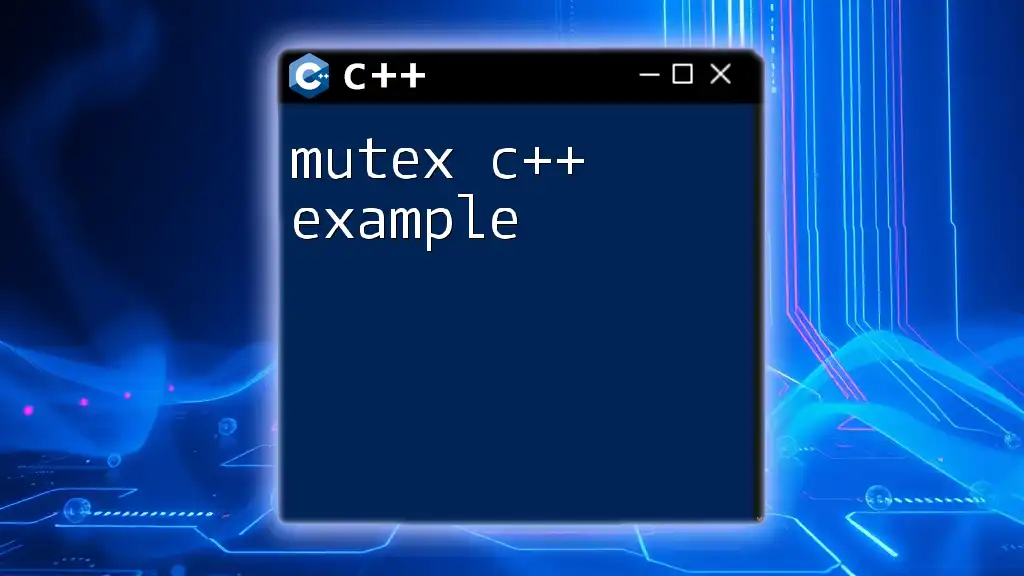
Object-Oriented Programming in C++
Understanding Classes and Objects
C++ is an object-oriented programming language, and classes and objects are fundamental concepts. A class is a blueprint for creating objects and encapsulates data for the object.
Here’s an illustration of class definition:
class Car {
public:
void start() {
std::cout << "Car started" << std::endl;
}
};
In this example, `Car` is a class that contains a public method `start()`, which prints a message when invoked.
Inheritance and Polymorphism
Inheritance allows a class to inherit properties and methods from another class, promoting code reusability.
Here’s an example:
class Vehicle {
public:
void move() { std::cout << "Moving"; }
};
class Bike : public Vehicle {
public:
void ringBell() { std::cout << "Bell rings"; }
};
In this case, `Bike` inherits from `Vehicle`, allowing it to use its methods while adding its own. This demonstrates one of the core principles of object-oriented programming in C++.
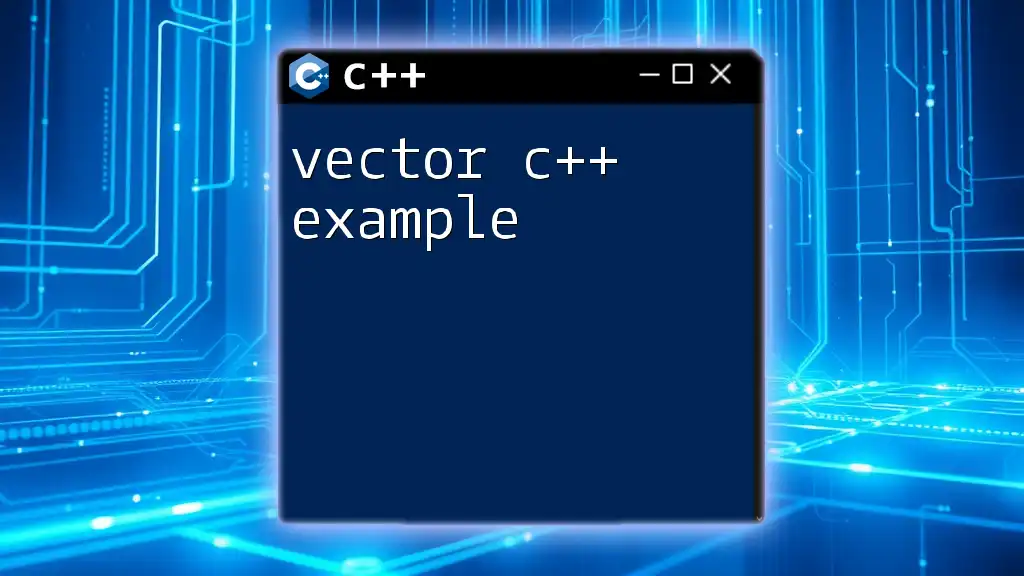
Conclusion
This guide provided a comprehensive overview of C++ examples covering basic syntax, variables, control structures, functions, arrays, and object-oriented programming. By understanding and experimenting with these examples, you can deepen your C++ knowledge and enhance your programming skills. Don't hesitate to try these code snippets in your development environment, as hands-on practice is vital for mastering C++.