In C++, the `std::expected` feature is a proposed standard library utility that encapsulates the result of operations that may succeed or fail, providing a means to handle errors more effectively without relying solely on exceptions.
Here's a simple example of how you might use `std::expected`:
#include <iostream>
#include <expected>
std::expected<int, std::string> divide(int numerator, int denominator) {
if (denominator == 0) {
return std::unexpected("Division by zero error");
}
return numerator / denominator;
}
int main() {
auto result = divide(10, 0);
if (result) {
std::cout << "Result: " << *result << std::endl;
} else {
std::cout << "Error: " << result.error() << std::endl;
}
return 0;
}
This code snippet shows how to use `std::expected` to handle a division operation that could fail due to division by zero.
What is `std::expected`?
`std::expected` is a class template introduced to provide a robust mechanism for handling operations that can either succeed with a value or fail with an error. Its design is aimed at improving the clarity and maintainability of programs by reducing reliance on error codes or exceptions.
When using traditional error handling techniques, developers often find that error codes can lead to unclear and cluttered code, while exceptions may introduce complexity in control flow. `std::expected` elegantly resolves these issues by encapsulating the result of an operation along with any associated error state in a single object.
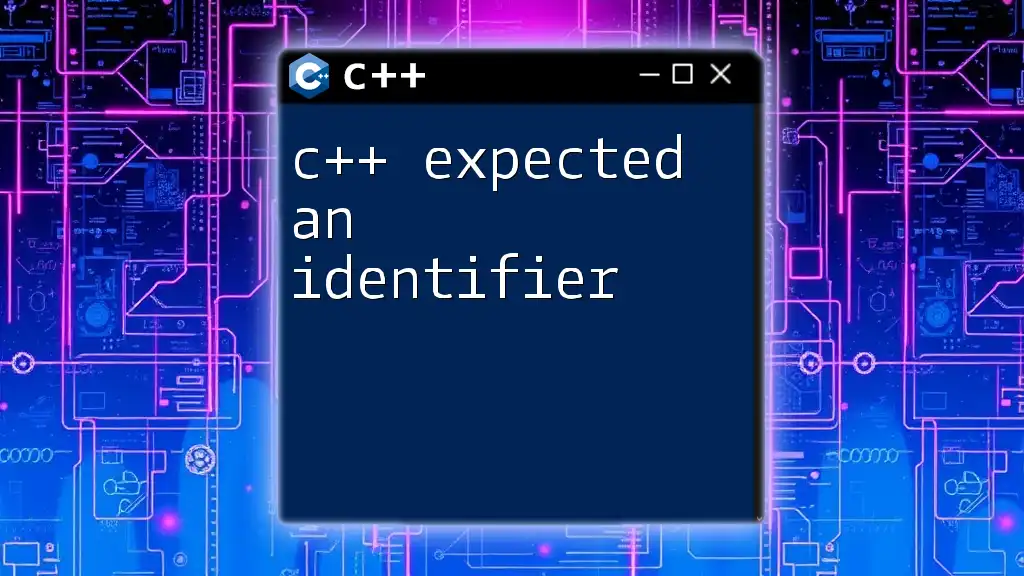
Why Use `std::expected`?
Using `std::expected` offers several advantages:
-
Clarity and Conciseness: By returning a single object that represents either a successful result or an error, `std::expected` simplifies the control flow of programs. There's no need to check error codes manually; the presence or absence of a value in the `expected` object indicates the success or failure.
-
Better Error Management: `std::expected` allows you to propagate errors without throwing exceptions, making it easier to reason about code behavior and reducing side effects.
-
Readable and Maintainable Code: Code that uses `std::expected` can be easier to follow, making it simpler to understand the success/failure states of operations without digging through exception handling constructs.
Comparing Traditional Error Handling Techniques
Error Codes: Error codes are a commonly used technique but come with their own set of challenges:
- Advantages: Simple to implement and do not disrupt the program flow.
- Disadvantages: They require manual checks after each operation and can lead to missed errors if programmers forget to check them.
Exceptions: C++ supports exception handling, but it has its downsides:
- Pros: Exceptions allow separation of error handling from regular code flow.
- Cons: They can make code difficult to follow and introduce additional complexity in managing resource cleanup.
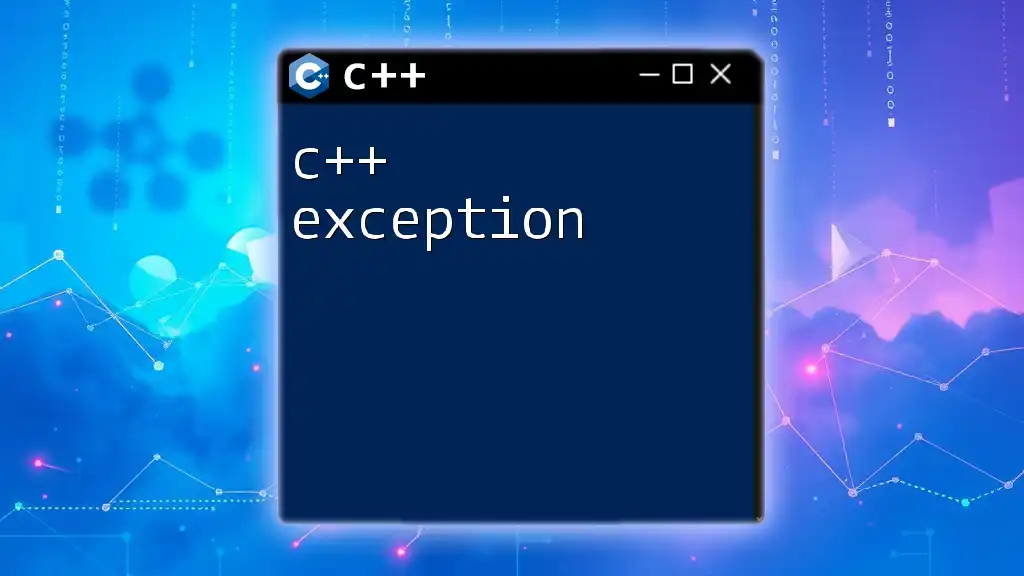
The Basics of `std::expected`
The `std::expected` class template is defined in the C++ standard library, allowing you to specify two types: one for the successful result (denoted as `T`) and another for the error state (denoted as `E`). This provides a clean and unified way to handle function outcomes.
The basic syntax looks like this:
std::expected<T, E>
Templates and Type Parameters
When declaring an `expected` object, you define the types that represent success and error. Here’s a simple example demonstrating how to declare an `expected` object that returns an integer or an error message:
#include <expected>
#include <string>
#include <iostream>
std::expected<int, std::string> divide(int a, int b) {
if (b == 0)
return std::unexpected("Division by zero");
return a / b;
}
In this example, the function `divide` checks if the denominator is zero. If it is, it returns a `std::unexpected` instance containing the error message; otherwise, it returns the result of the division.
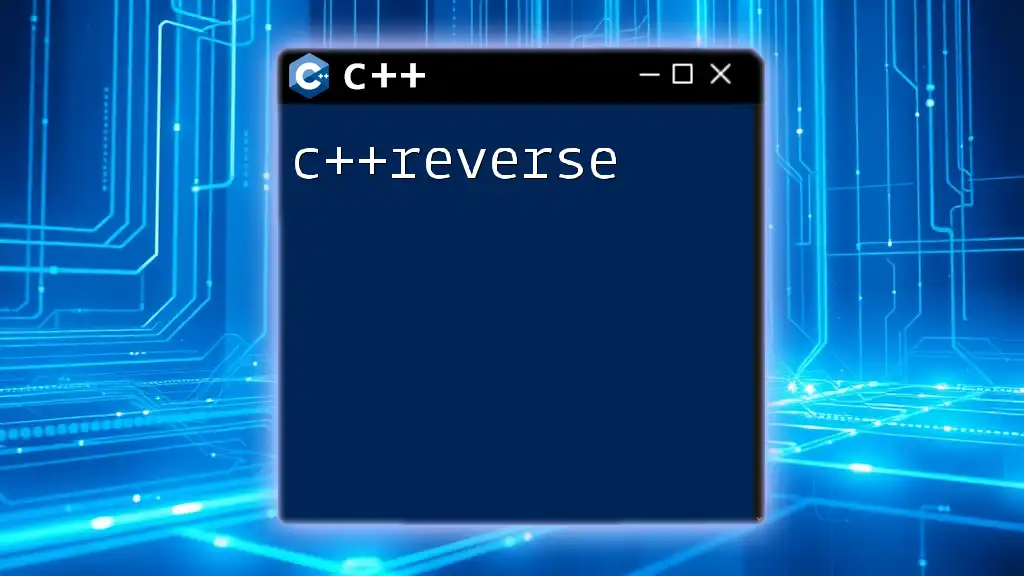
How to Use `std::expected` in Your Code
To use `std::expected`, you can create instances of expected outcomes in your functions. When a function returns an `expected` object, you can handle its value or error state in a straightforward manner.
Example: Using `std::expected` for a Simple Function
Let’s implement a function that adds two integers safely:
std::expected<int, std::string> safe_add(int a, int b) {
if (a > INT_MAX - b) return std::unexpected("Integer overflow occurred");
return a + b; // Result if successful
}
To handle the result of the function, you can use the following pattern:
auto result = safe_add(100, 200);
if (result.has_value()) {
std::cout << "Result is: " << result.value() << "\n";
} else {
std::cout << "Error: " << result.error() << "\n";
}
Here, `has_value()` checks if the operation was successful, while `value()` retrieves the result. If an error occurred, `error()` fetches the error message, keeping the control flow clean and understandable.
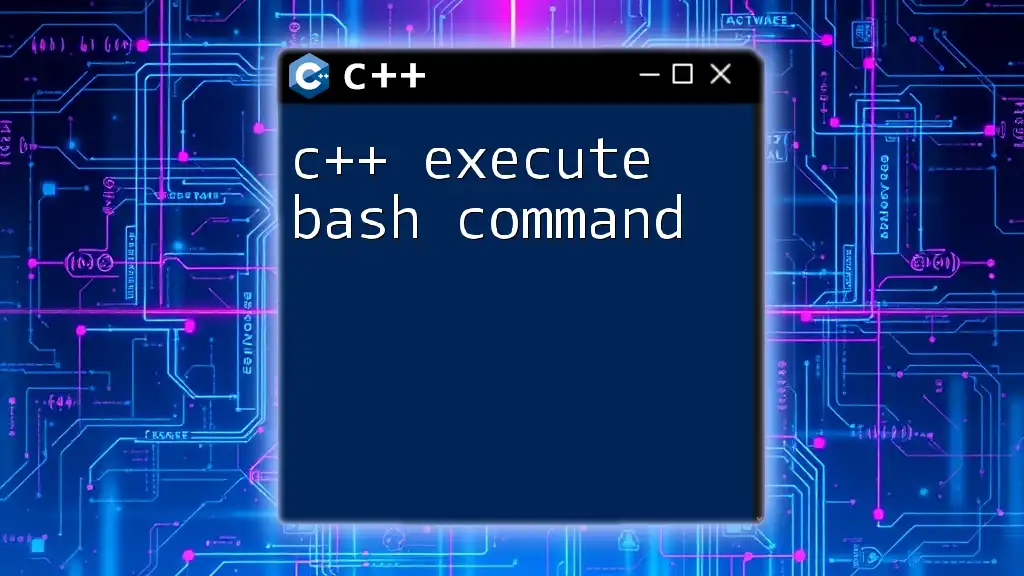
Best Practices for Using `std::expected`
To maximize the benefits of `std::expected`, keep these best practices in mind:
-
Use `std::expected` when you want to represent a function that can return a valid result or an error. It’s a clear way to denote the potential for failure directly in the return type.
-
Prefer using `std::expected` over exceptions when you want to manage errors in a more explicit manner.
-
Combine `std::expected` with `std::optional`. If a function may not return an error but could return an "empty" or "non-existent" value, you might consider using `std::expected<T, void>`.
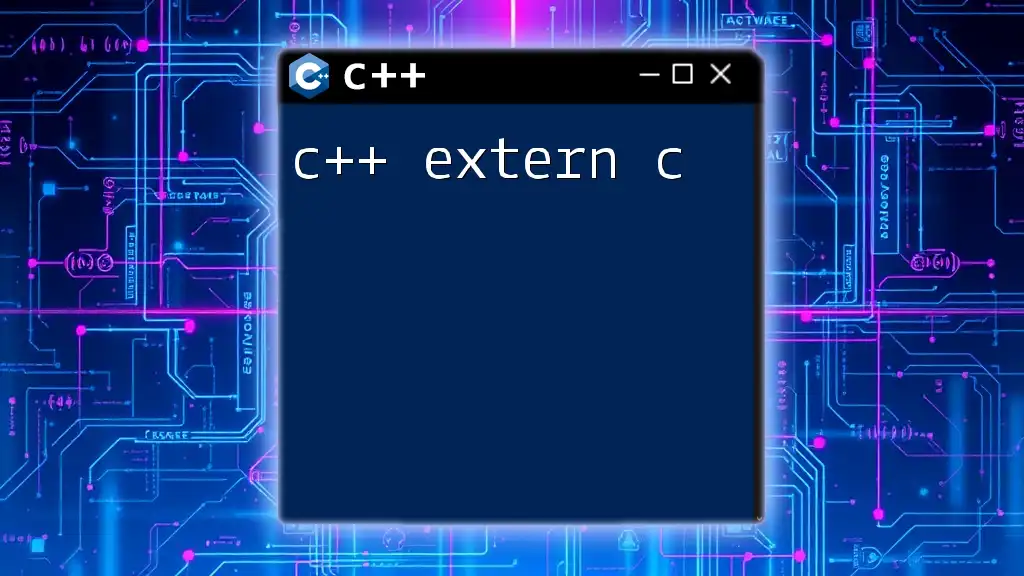
Advanced Uses of `std::expected`
Chaining Operations
A powerful feature of `std::expected` is that it allows for chaining operations. When a function's output is another operation that may also fail, you can directly chain these calls without messy error handling.
std::expected<int, std::string> process_input(int input) {
if (input < 0) return std::unexpected("Negative input");
// Mock processing logic
return input * 10;
}
auto result = divide(process_input(5).value(), 2);
Error Handling Strategies
When dealing with multiple `expected` results in a chain, Error handling can be approached elegantly through:
- Early Returns: Immediately terminate the processing pipeline when an error is encountered.
- Mapping Error Handling: Use mapping to transform or handle the errors in a more sophisticated way.
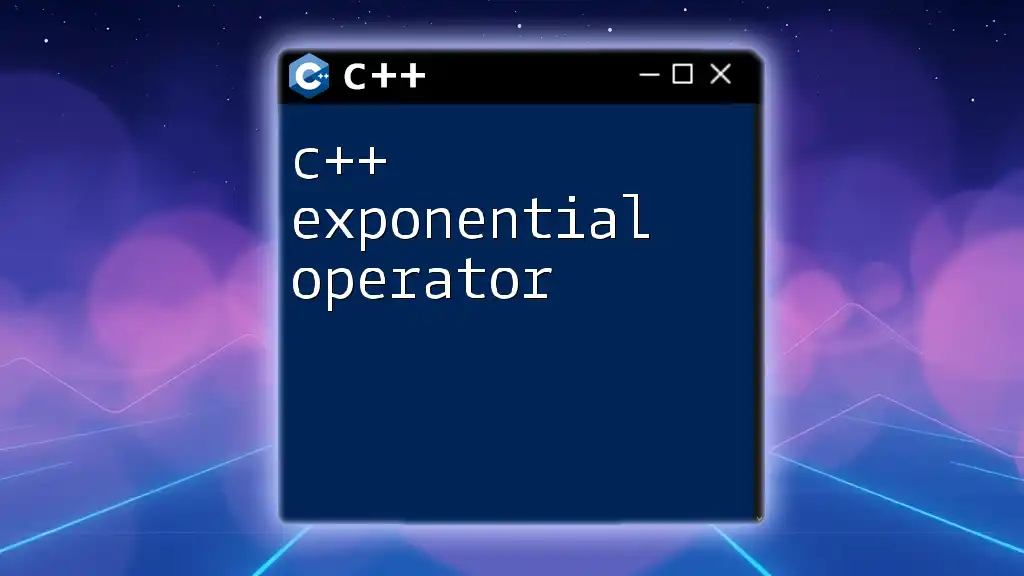
Common Pitfalls and How to Avoid Them
As with any feature, `std::expected` has its pitfalls:
-
Forgetting to Check the Result: Always check for success/failure using `has_value()` or similar checks. Skipping these could leave errors unhandled.
-
Excessive Coupling: Try not to tightly couple the operations with expected states, which could hinder flexibility. Keep function interfaces clean.
-
Ignoring `std::unexpected`: Mismanaging the error by treating it as a regular value can result in silent failures. Always treat `std::unexpected` cases as specialized handling paths.
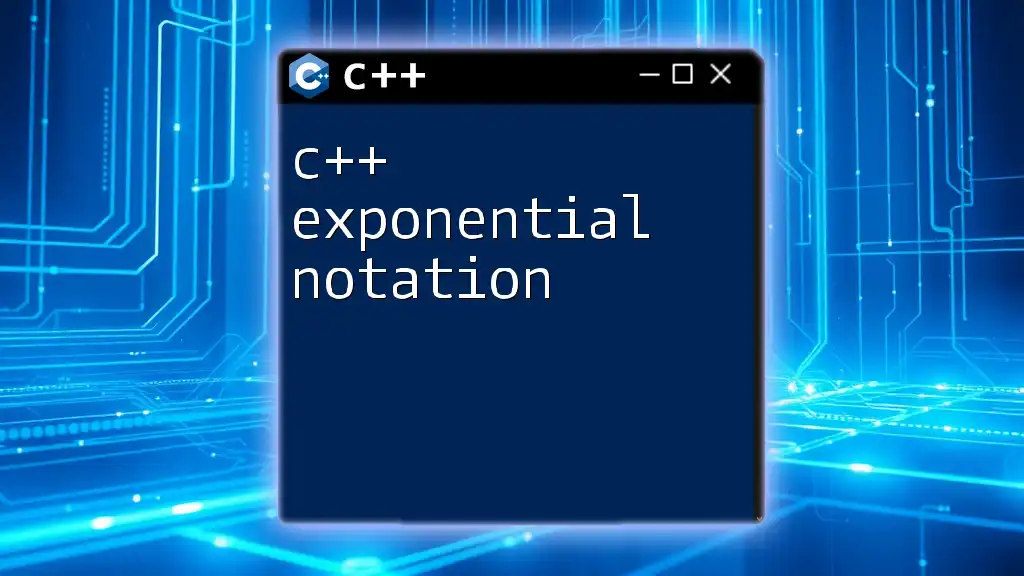
Conclusion
In summary, `std::expected` is a powerful tool for handling operations that can fail, offering a clearer and more manageable way to handle errors compared to traditional techniques like error codes and exceptions. By incorporating `std::expected` into your C++ programming practices, you cultivate clearer, more maintainable code that gracefully handles success and failure states. Embrace it in your next projects for a more robust error management strategy.
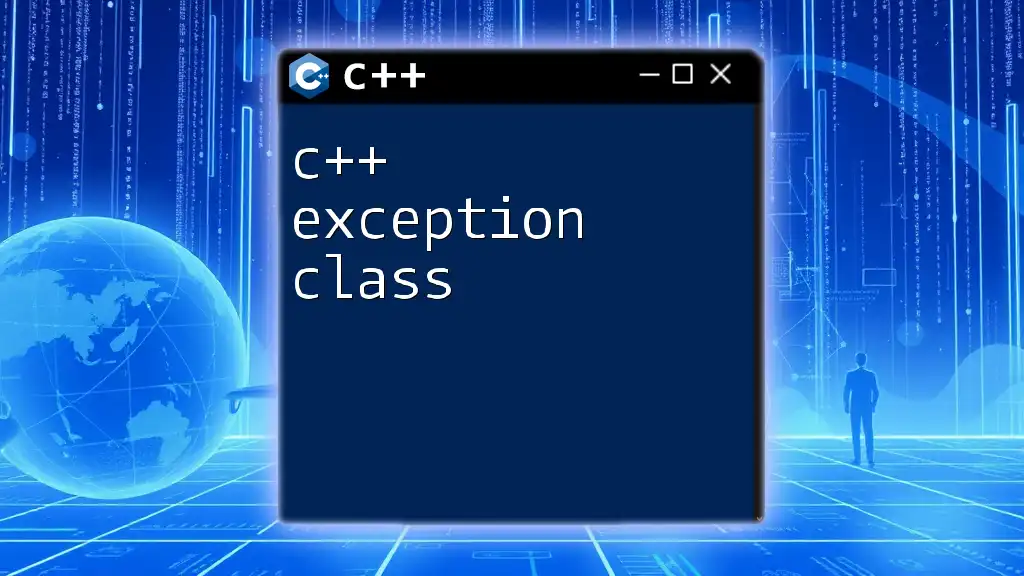
Additional Resources
To delve deeper into `std::expected`, consider exploring:
- The latest C++ standards that introduce additional features.
- Online documentation and community discussions surrounding best practices in error handling in modern C++.
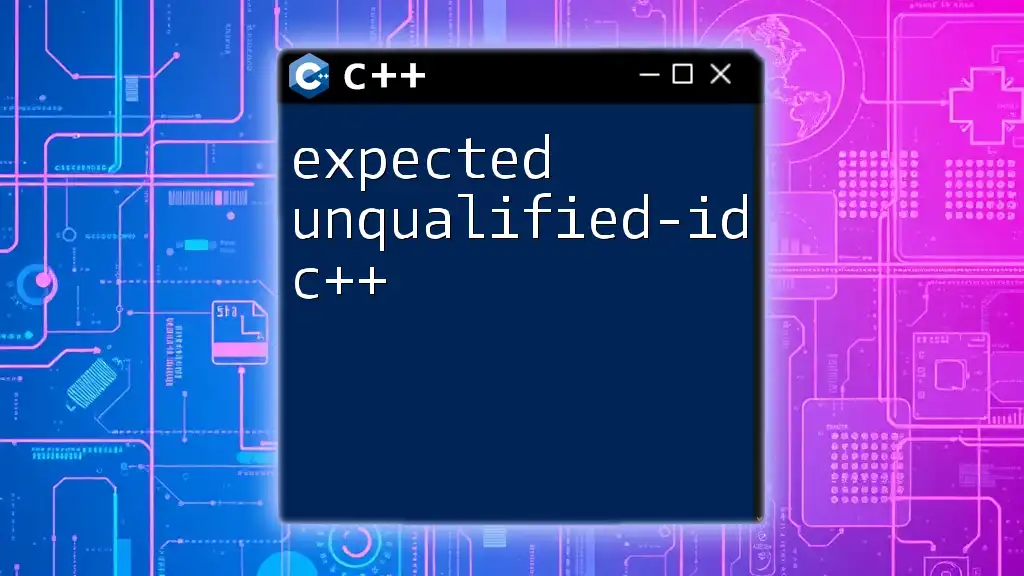
Call to Action
We invite you to share your experiences with using `std::expected` and suggest topics for future articles! Engaging with the community helps us all grow as developers and enhance our coding practices.