In C++, you can execute a Bash command using the `system()` function from the `<cstdlib>` header, allowing you to run command-line operations directly from your C++ program.
#include <cstdlib>
int main() {
system("echo Hello, World!");
return 0;
}
Understanding System Calls in C++
In the C++ programming language, system calls are essential for performing various tasks, including executing external commands. A system call is an interface that allows a program to communicate with the operating system, enabling it to request services such as file manipulation, process control, and executing commands.
One of the most straightforward ways to execute a Bash command from C++ is to use the `system()` function. This function provides a simple interface to the command shell, allowing you to run commands as if you were typing them directly into the terminal.
The Role of the `system()` Function
The `system()` function takes a string representing the command and passes it to the shell for execution. The syntax is as follows:
int system(const char *command);
This function returns an integer value that indicates the success or failure of the command executed. A return value of `0` typically means that the command executed successfully, while any non-zero value often indicates an error.
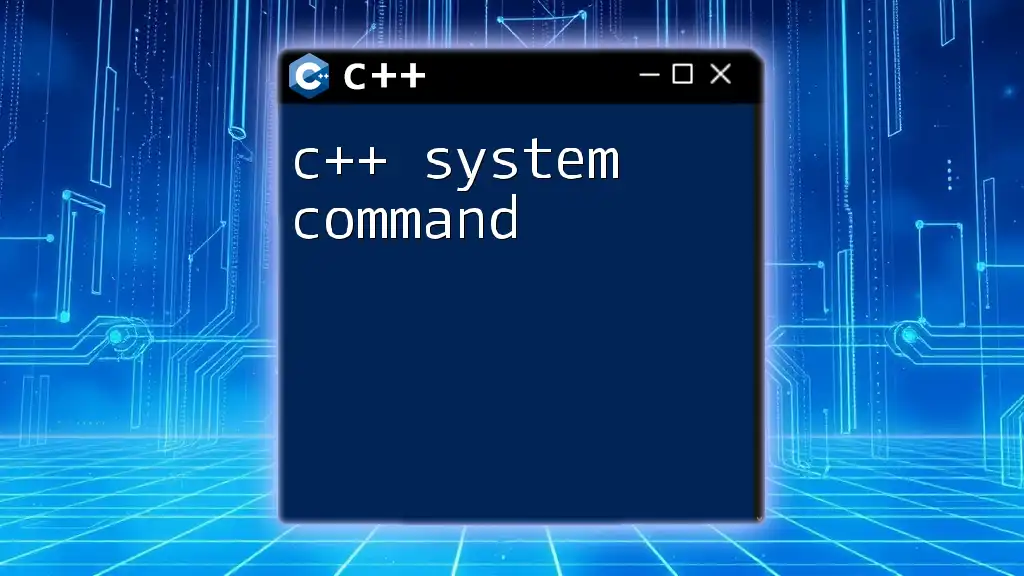
Executing Bash Commands Using `system()`
Simple Command Execution
To execute a basic Bash command, you can simply use the `system()` function in your C++ code. For example, if you want to list the files and directories in the current directory using the `ls` command, your code would look like this:
#include <cstdlib>
int main() {
system("ls");
return 0;
}
When this code is run, it will display the contents of the current directory directly in the console.
Advanced Command Execution
Command with Arguments
To execute commands that require arguments, you can pass the command string with the necessary parameters. For instance, to create a new directory called `new_directory` using the `mkdir` command, you can write:
int main() {
system("mkdir new_directory");
return 0;
}
This command will execute properly if you have permissions to create a directory in the current path. If the directory already exists or if permissions are insufficient, it may return a non-zero exit status indicating an error.
Piping and Redirection
C++ can also be used to execute more complex shell commands that involve piping and redirection. For example, to filter the output of the `ls` command for files containing the word "file," you can use:
int main() {
system("ls | grep 'file'");
return 0;
}
This command demonstrates how to use the output of one command as input to another using the pipe (`|`). The `grep` command will only display lines from the `ls` output that contain the specified string, offering an excellent illustration of how to leverage shell capabilities within C++.
Error Handling with `system()`
When using `system()`, it's crucial to handle potential errors. The function returns an integer that you can use to check whether the command was executed successfully. You can implement error handling as follows:
int status = system("command");
if (status == -1) {
// Handle error
}
This simple check allows you to respond appropriately if the command fails. Always check the return status to understand how well your command executed and to account for any issues.
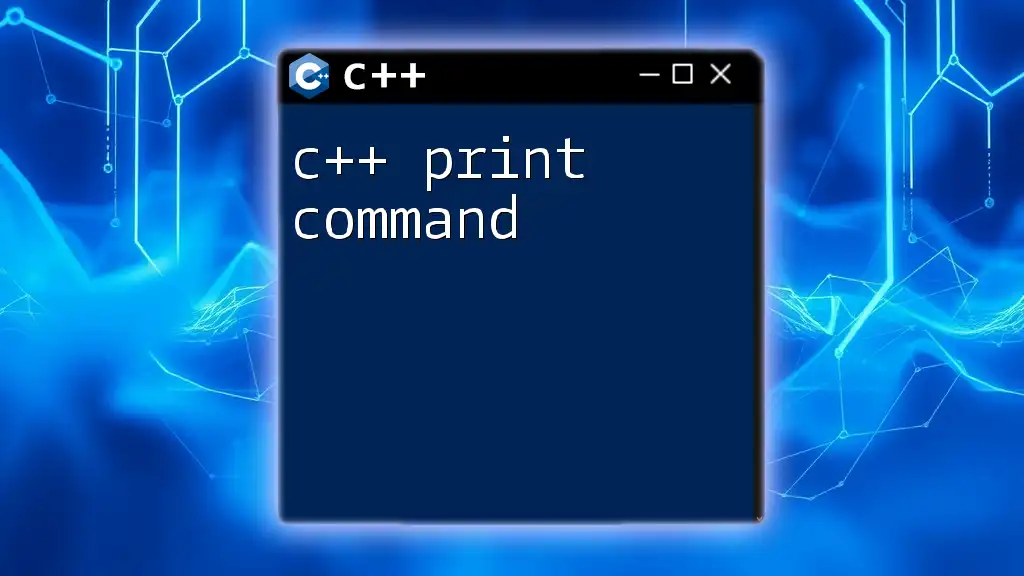
Using `popen()` for More Control
An Introduction to `popen()`
While `system()` is straightforward, it lacks control over command execution, especially when handling input or output. The `popen()` function provides a more flexible solution that lets you read output from commands or write input to them.
Reading Command Output
If you want to read the output of a command in your C++ program, you can use `popen()`. Here’s an example that captures the output of the `ls` command:
#include <cstdio>
int main() {
FILE *fp;
char buffer[128];
fp = popen("ls", "r");
if (fp == nullptr) {
// Handle error
return -1;
}
while (fgets(buffer, sizeof(buffer), fp) != nullptr) {
printf("%s", buffer);
}
pclose(fp);
return 0;
}
In this example, `popen()` opens a process by creating a pipe, allowing you to read from the `ls` command. The `while` loop reads each line from the command's output until there’s no more data, which is then printed to the console. Always ensure to close the pipe using `pclose()` to avoid resource leaks.
Writing Commands to Input
You can also use `popen()` to supply input to commands. For example, to sort strings and write to the sort command's input, you can do the following:
FILE *fp = popen("sort", "w");
fprintf(fp, "c\nb\na\n");
pclose(fp);
In this case, the `sort` command will process the strings "c," "b," and "a," sorting them and outputting "a," "b," and "c." This demonstrates how you can program interactions with command-line utilities directly from your C++ code.
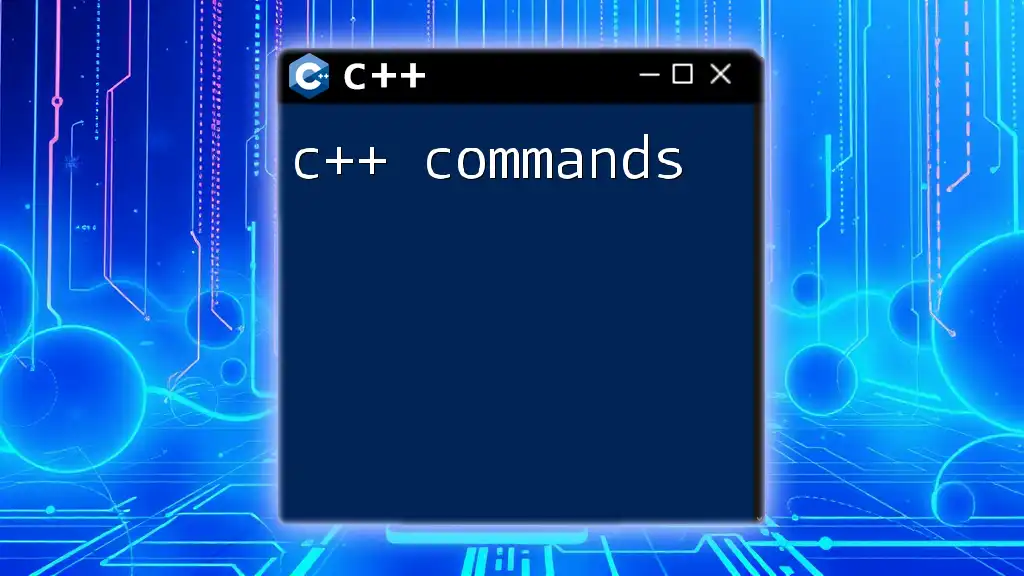
Securing Command Execution
Executing commands outside your program can be risky, particularly concerning security vulnerabilities like shell injection attacks. Here are some best practices:
-
Sanitize Input: Never pass untrusted user input directly to a shell command. Always sanitize and validate the data to prevent malicious exploitation.
-
Avoid Shell Built-ins: When possible, prefer direct system calls or libraries over shell commands to reduce potential risks.
By being aware of these concerns, you can significantly enhance the security of your applications when executing Bash commands.
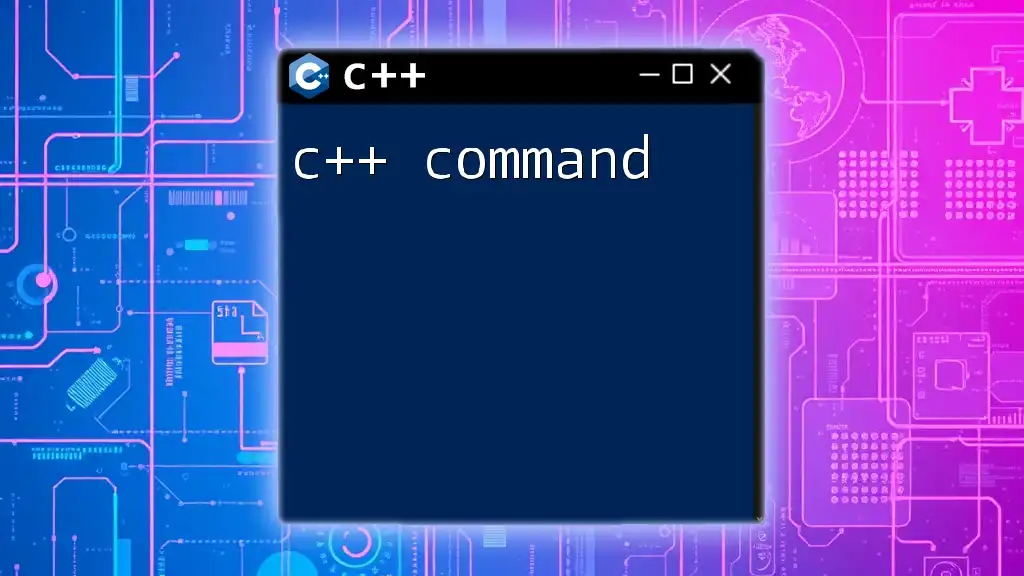
Conclusion
Understanding how to execute Bash commands in C++ provides powerful capabilities for interacting with your operating system, from file manipulation to complex command execution. By utilizing functions like `system()` and `popen()`, you can efficiently harness this functionality while also ensuring to implement proper error handling and security measures.
By practicing these techniques, you’ll become more adept at utilizing external commands seamlessly within your C++ programs, opening up a world of possibilities for automation and dynamic process control.