C++ documentation provides essential information on the language's syntax, libraries, and usage, allowing developers to quickly understand and implement C++ commands efficiently.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Documentation
What is Documentation?
Documentation refers to a collection of instructions, descriptions, or explanations related to a certain topic. In the realm of programming, documentation serves as a crucial tool for understanding, maintaining, and using code effectively. There are several types of documentation in programming:
-
Inline Documentation: This consists of comments embedded directly within the code and helps explain the purpose and functionality of various segments.
-
External Documentation: This includes user manuals, API references, and tutorials meant to provide comprehensive insights into the entire system or library.
Types of C++ Documentation
Inline Documentation
Inline documentation is crucial for the immediate understanding of code. It provides context for someone reading the code without needing to reference external resources. For example:
// Function to calculate the sum of two integers
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
Importance: Inline comments clarify logic and purpose, helping to reduce confusion and mistakes during later edits.
API Documentation
API documentation is essential for developers using libraries or frameworks. It outlines the functionalities available, how to use them, and provides examples of expected input/output. Tools like Doxygen and Sphinx are commonly used to create and maintain API documentation dynamically.
For example, a sample API documentation entry for a class might look like this:
/**
* @class Calculator
* @brief A simple calculator class
*
* This class provides basic arithmetic operations.
*/
class Calculator {
public:
/// Adds two doubles
double add(double a, double b);
/// Subtracts second double from the first
double subtract(double a, double b);
};
User Guides & Tutorials
User guides and tutorials help end users understand how to utilize specific functionality within a software application. Typical components of a user guide include setup instructions, example use cases, and troubleshooting tips. A well-structured user guide often contains:
- Step-by-step instructions for various tasks
- Code snippets to illustrate usage
- Best practices for optimal usage

Importance of C++ Documentation
Enhances Code Maintainability
Well-written documentation significantly enhances code maintainability. When future developers (or even the original authors) refer to their previous work, clear documentation aids comprehension and streamlines updates. For example, consider two snippets:
Poorly Documented Code:
void f(int x) {
// Do stuff
int y = x * 2;
}
Well-Documented Code:
// Multiplies the input by 2 and stores the result in y
void doubleValue(int x) {
int y = x * 2; // y now holds double the input
}
In the second example, future developers can understand the purpose of the function at a glance.
Facilitates Collaboration
Documentation plays a vital role in collaborative projects, allowing team members to stay informed about code functionality and design choices. Whether sharing a codebase with colleagues or contributing to open-source projects, well-structured documentation ensures everyone is on the same page. For instance, project wikis or README files can serve as common resources for team insights.
Acts as a Learning Resource
For newer developers, comprehensive documentation can bridge the gap in their understanding. An example is the documentation provided for libraries like Boost or Qt, which often comes with clear explanations, examples, and tutorials that minimize the learning curve.
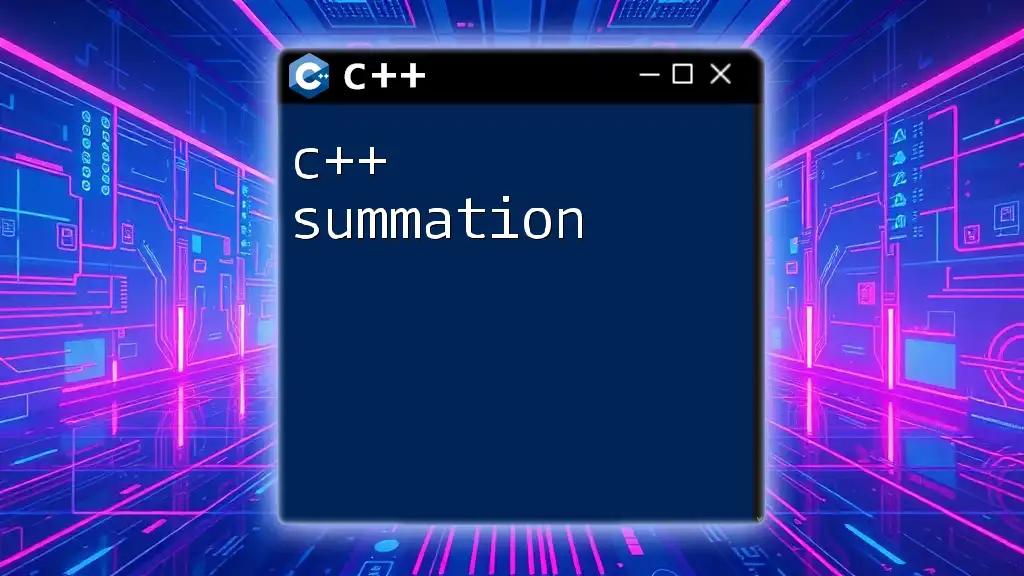
Components of Good C++ Documentation
Consistency
Consistency in style is crucial when creating documentation. Abiding by specific naming conventions and formatting guidelines across comments and documentation enhances readability. Establishing a style guide for your project helps maintain uniformity and clarity.
Clarity and Conciseness
Effective documentation should prioritize clarity and conciseness. This means avoiding overly verbose descriptions while ensuring the necessary information is present. Consider the following example:
Redundant Comment:
// This function returns the sum of two integers
int sum(int a, int b) {
return a + b;
}
Concise Comment:
// Returns the sum of two integers
int sum(int a, int b) {
return a + b;
}
Code Examples and Snippets
Documenting code examples can provide invaluable context. For example, when charting the usage of a function, ensure you include calls and their expected outputs:
// Example usage of sum function
int main() {
int result = sum(5, 3); // result is 8
return 0;
}
Here, you show how the `sum` function operates in a practical context.
Use of Visual Aids
Visual aids like flowcharts or diagrams can illustrate complex algorithms or workflows. These visuals help break down complicated information into digestible formats. For example, a flowchart explaining the steps of a sorting algorithm could clarify its operations far beyond textual explanations.
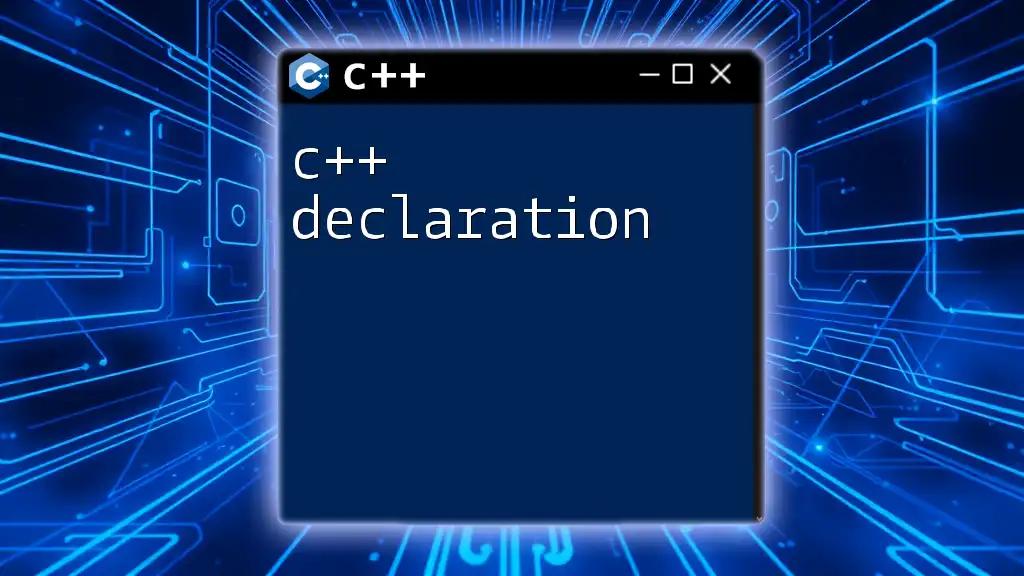
Tools for Creating C++ Documentation
Doxygen
Doxygen is a powerful documentation generator primarily designed for C++. It parses comments in the source code and produces comprehensive documentation in various formats, including HTML and LaTeX.
To set up Doxygen for a C++ project:
- Install Doxygen: Follow installation instructions as per your operating system.
- Create a Doxyfile: This configuration file will define how Doxygen generates your documentation.
- Annotate your code: Use specific comment formats to annotate your code adequately.
- Run Doxygen: Execute the Doxygen command to generate the documentation based on your code comments.
Example of Doxygen annotation:
/**
* @brief Multiplies two integers
*
* @param a First integer
* @param b Second integer
* @return Product of a and b
*/
int multiply(int a, int b) {
return a * b;
}
Sphinx
Sphinx is a documentation generator that’s widely used in the Python community but can be adapted for C++ projects too.
To utilize Sphinx:
- Install Sphinx: Ensure you have Python installed, then install Sphinx using pip.
- Initialize Sphinx in your project: Run `sphinx-quickstart` to set up a structure.
- Write documentation: Use reStructuredText or Markdown to create documents.
- Build the documentation: Execute the Sphinx build command to generate static HTML documentation.
Example of a basic Sphinx documentation entry:
.. module:: my_module
:synopsis: Provides basic arithmetic functions.
.. function:: multiply(a, b)
Multiplies two integers.
:param a: First integer to multiply.
:param b: Second integer to multiply.
:returns: The product of a and b.
Other Documentation Tools
While Doxygen and Sphinx are popular, other tools like Javadoc and GitHub Pages also serve useful purposes. They may offer different formats and qualities of output, so developers should assess them based on their specific project needs.
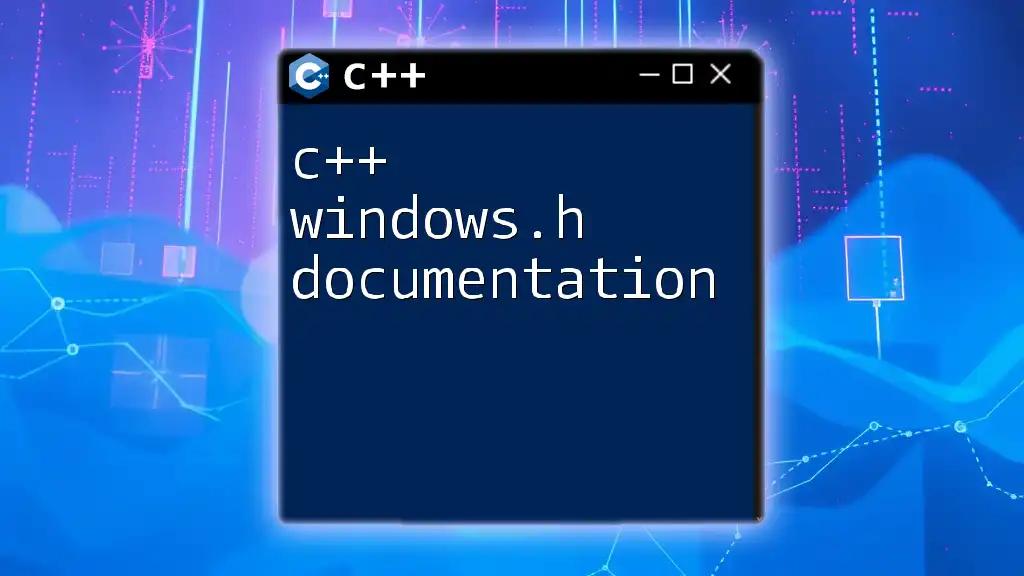
Best Practices for Effective C++ Documentation
Write for Your Audience
Understanding the audience is crucial. Tailor your documentation to match the knowledge level and needs of your users, be it novice programmers or experienced developers.
Regular Updates
A common pitfall in software development is stagnant documentation. Regularly review and update your documentation to reflect new features, deprecated functionalities, and changes in design. One effective strategy may involve scheduling periodic reviews to assess the accuracy of your documentation.
Utilize Examples and Tutorials
Concrete examples can clarify complex functions or classes. Structure tutorials to showcase common use cases, ensuring readers derive practical implications from theoretical concepts.
Encourage Community Contributions
Open-source projects benefit from community contributions to the documentation. Establish clear guidelines for contributions, and promote collaboration on platforms like GitHub, where others can suggest edits or improvements to the documentation.

Conclusion
C++ documentation is a vital component of programming that enhances maintainability, fosters collaboration, and serves as a crucial learning resource. By implementing best practices and utilizing effective tools, developers can create comprehensive documentation that not only serves immediate needs but also evolves alongside the codebase. Prioritizing documentation will streamline future development processes and ultimately lead to more robust software.
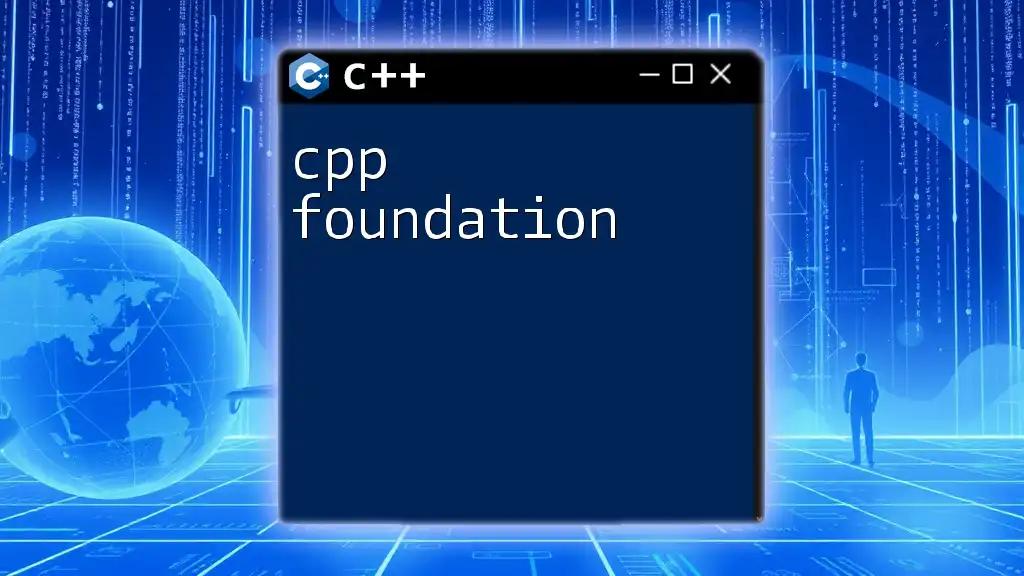
Further Reading and Resources
For those wishing to deepen their understanding of C++ documentation, consider exploring the following resources:
- Books on documentation practices and programming patterns
- Online articles that focus on documentation tools and techniques
- Forums and communities dedicated to C++ programming

FAQ Section
Common Questions About C++ Documentation
What is the best practice for writing comments? Aim to be clear, concise, and purposeful. Comments should explain why the code exists rather than what it does, which should be apparent from the code itself.
How often should documentation be reviewed or updated? Review documentation regularly, especially when changes are made to the code. Consider implementing a schedule, such as every quarter, or whenever a new feature is added.
Are there specific formatting guidelines for C++ documentation? While guidelines may vary by project, adopting a consistent style for comments (like using Doxygen annotations) and adhering to a chosen documentation standard can enhance clarity and usability.