A "C++ segmentation fault (core dumped)" error occurs when a program tries to access a memory location that it's not allowed to, often due to dereferencing a null or invalid pointer.
#include <iostream>
int main() {
int* ptr = nullptr; // Initialize a null pointer
std::cout << *ptr; // Dereference the null pointer, causing a segmentation fault
return 0;
}
Understanding Segmentation Faults
What is a Segmentation Fault?
A segmentation fault occurs when a program tries to access a memory segment that it is not allowed to. In the context of C++, memory management is crucial since it involves direct manipulation of pointers and memory addresses. When a program attempts to access an invalid memory segment, the operating system interrupts it, resulting in an error and typically displaying the message "Segmentation fault (core dumped)."
When Does a Segmentation Fault Occur?
Common scenarios that lead to segmentation faults include dereferencing null pointers, accessing elements out of bounds, or using uninitialized pointers. For instance, if you attempt to access a memory location that the program is not allocated, you will encounter a segmentation fault.
The Role of Core Dumps
A core dump is a file that captures the memory image of a process at a specific point, usually when it crashes. It can be analyzed to determine the cause of the fault. Core dumps are immensely helpful for debugging, allowing developers to gain insights into the state of the program at the moment the segmentation fault occurred. Analyzing such dumps helps identify bad memory accesses or logical errors in the code.
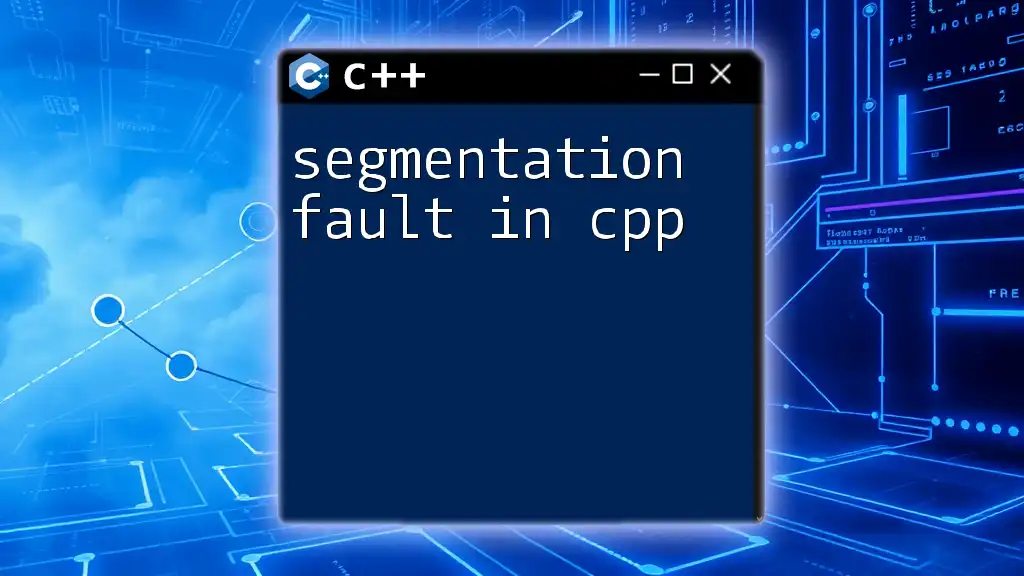
Common Causes of Segmentation Faults in C++
Dereferencing Null Pointers
One of the most straightforward causes of a segmentation fault is attempting to dereference a null pointer. A null pointer does not point to any valid location in memory; thus, dereferencing it leads to a crash. Here’s an example:
int* ptr = nullptr;
// This will cause a segmentation fault
std::cout << *ptr << std::endl;
Out-of-Bounds Array Access
In C++, arrays have fixed sizes, and accessing an index beyond the allocated limits will cause undefined behavior, most often resulting in a segmentation fault. For example:
int arr[5] = {1, 2, 3, 4, 5};
// This will cause a segmentation fault
std::cout << arr[10] << std::endl;
Use of Uninitialized Pointers
Using pointers before they have been initialized is another common pitfall that leads to segmentation faults. An uninitialized pointer might point to a random location, and accessing it is akin to a gamble with undefined results. Consider this example:
int* ptr; // Pointer declared but not initialized
// This will cause a segmentation fault
std::cout << *ptr << std::endl;
Memory Mismanagement
Double Free or Corruption
Double freeing memory occurs when a program attempts to free a pointer that has already been released. Doing so corrupts the program's memory management structure, leading to a potential segmentation fault:
int* ptr = new int(5);
delete ptr;
delete ptr; // This will cause a segmentation fault
Stack Overflow
Stack overflow often occurs due to excessive recursion. Each function call adds a layer to the call stack, and if recursion is uncontrolled, it will eventually exhaust the stack space, leading to a segmentation fault. Here’s a simple demonstration:
void recursiveFunc() {
recursiveFunc(); // Infinite recursion leading to segmentation fault
}
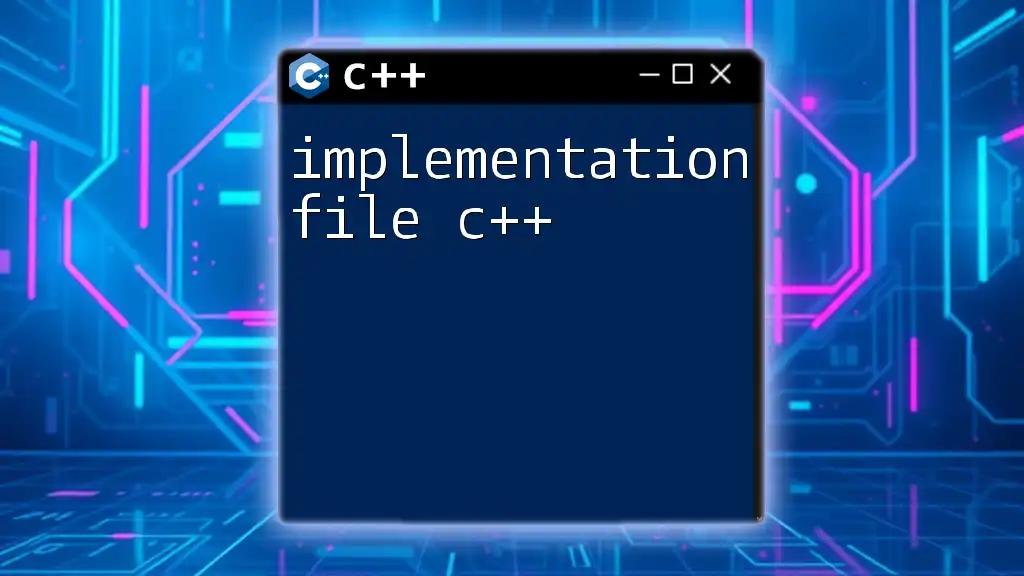
Identifying Segmentation Faults
Common Debugging Tools
When it comes to debugging segmentation faults, various tools can help identify the culprit. Some popular ones include gdb (GNU Debugger) and Valgrind. Gdb is particularly powerful for real-time debugging, allowing developers to step through the code line by line.
How to Use gdb to Debug Segmentation Faults
Using gdb can help pinpoint the exact line where the segmentation fault occurs. Here’s how to use it:
-
Compile your program with debugging symbols:
g++ -g your_program.cpp -o your_program
-
Start gdb with your program:
gdb ./your_program
-
Run your program inside gdb:
run
-
Once it crashes, you can backtrace to see where it went wrong:
backtrace
Analyzing Core Dumps
To analyze a core dump, you first need to enable core dumps in your system. You can do this by running:
ulimit -c unlimited // Enable core dumps
After running your program, a core file will be generated when a segmentation fault occurs. You can analyze it with gdb by executing:
gdb ./your_program core
This command will allow you to inspect the memory state at the time of the crash, helping you identify where the error originated.
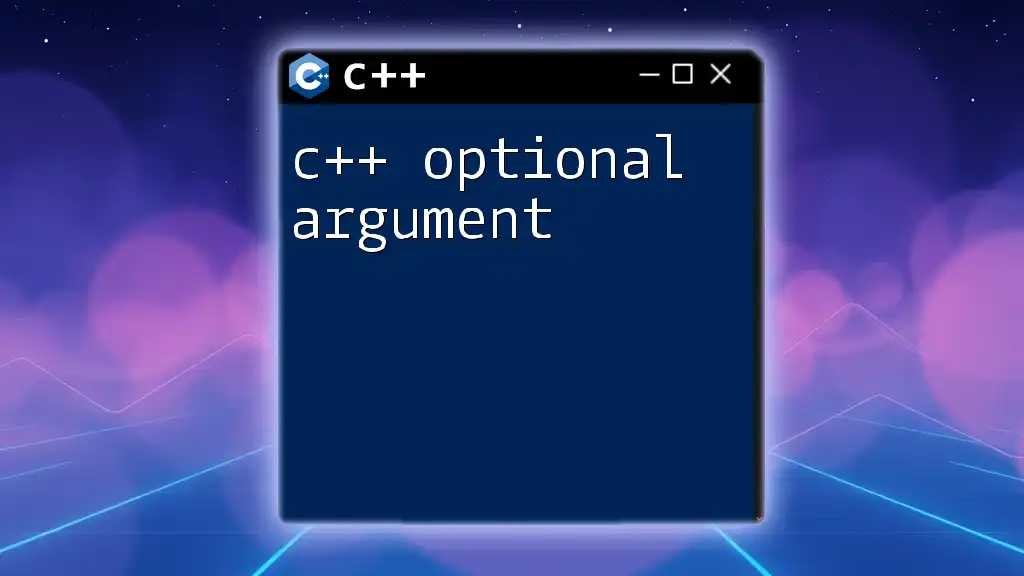
Preventing Segmentation Faults
Best Practices in C++ Programming
Preventing segmentation faults starts with best practices in coding. Always ensure pointers are initialized before use and check for nulls before dereferencing them. Another effective way to manage memory is by using smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, which automatically manage resource deallocation.
Writing Safe Code with C++ Standard Library
Utilizing the C++ Standard Library (STL) significantly reduces the risk of segmentation faults. Containers like `std::vector` and `std::array` automatically manage memory boundaries, making your code much safer:
std::vector<int> vec = {1, 2, 3};
// Using .at() provides bounds checking
std::cout << vec.at(2) << std::endl; // Safe access
Utilizing Compiler Warnings and Static Analysis Tools
Compiler warnings are an essential aspect of C++ development. Use flags such as `-Wall` and `-Wextra` to catch potential issues at compile time. Additionally, static analysis tools like the Clang Static Analyzer can help identify vulnerabilities in your code before execution.
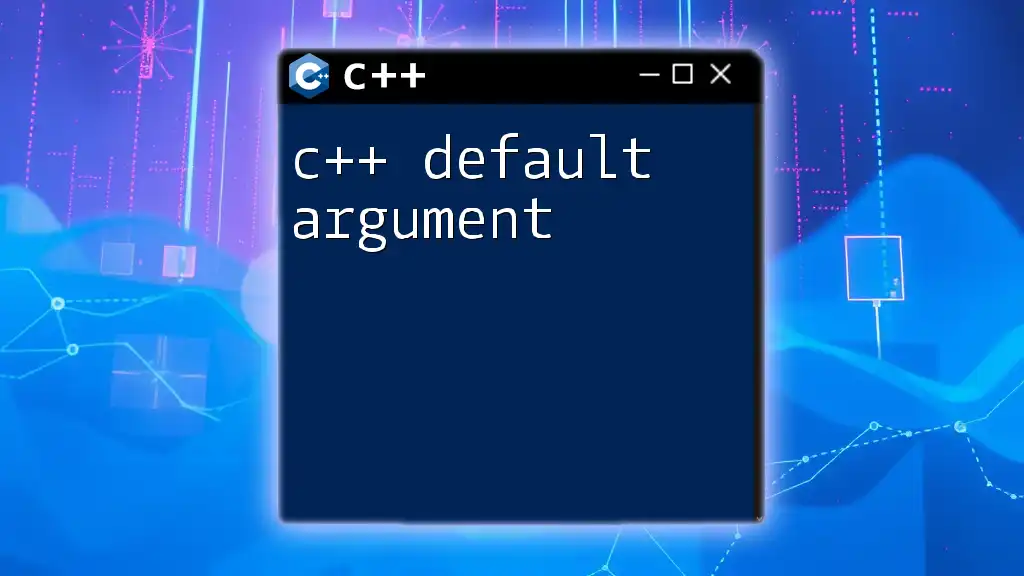
Conclusion
Summary of Key Points
Understanding what triggers a C++ segmentation fault core dumped error is crucial for any programmer. By recognizing common causes and using effective debugging methods, you can prevent these faults from disrupting your work. Applying best practices in memory management and using robust tools can lead to more reliable C++ applications.
Additional Resources
For further exploration of these topics, consider examining popular programming books, online courses, and engaging with communities focused on C++ development. Official C++ documentation and tutorials are invaluable resources for ongoing learning.
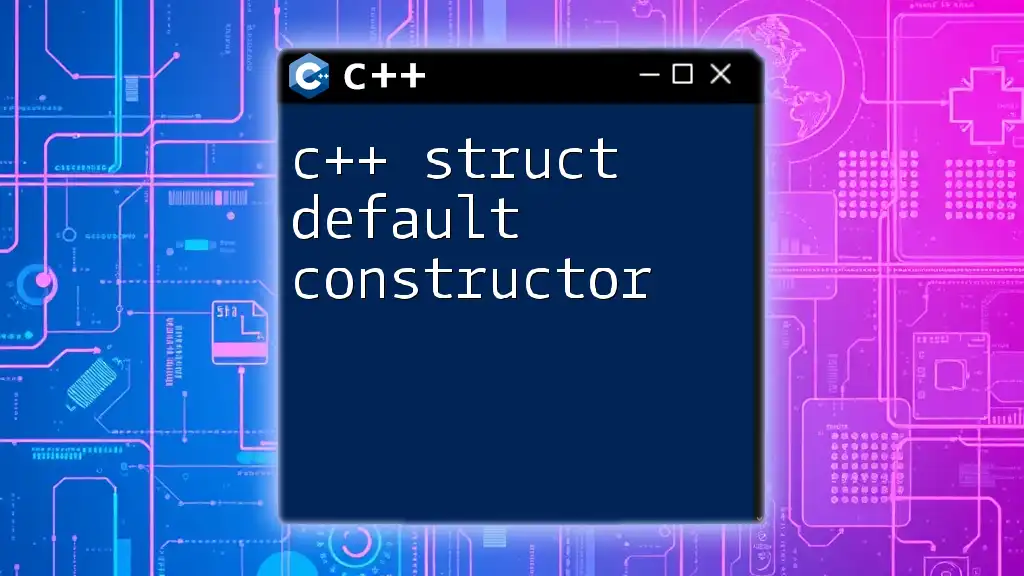
Call to Action
Take this knowledge to heart and practice your C++ skills regularly. Understanding and preventing segmentation faults is a step toward becoming a proficient C++ programmer. Share your experiences with segmentation faults or reach out for assistance in the comments section!