The error "C++ expression must be a modifiable lvalue" occurs when you attempt to modify a value that is not an assignable location in memory, such as a constant or a temporary value.
Here's an example demonstrating this error:
#include <iostream>
int main() {
const int x = 10; // x is a constant and cannot be modified
x = 20; // Error: expression must be a modifiable lvalue
std::cout << x << std::endl;
return 0;
}
What is a Modifiable Lvalue?
Definition of Lvalue and Rvalue
In C++, expressions can be categorized primarily into lvalues and rvalues. An lvalue is an expression that refers to a memory location, which allows for modifications. On the contrary, an rvalue represents a temporary value that does not occupy a specific memory address you can modify.
For example, in the line of code:
int x = 10; // x is an lvalue, 10 is an rvalue
`x` can be changed later in the code, while `10` is a literal constant and thus is a temporary value (rvalue).
Characteristics of Modifiable Lvalues
A modifiable lvalue is not just any lvalue; it is one that can be both read from and written to. There are two types of lvalues: modifiable and non-modifiable.
- Modifiable lvalues allow you to change their value during program execution.
- Non-modifiable lvalues are often defined using the `const` keyword, signaling that their values cannot be changed once set.
For instance:
const int y = 20; // Non-modifiable lvalue
y = 30; // Error: 'y' is not modifiable
In this case, attempting to assign a new value to `y` leads to a compilation error, showcasing the restrictions imposed by constancy.
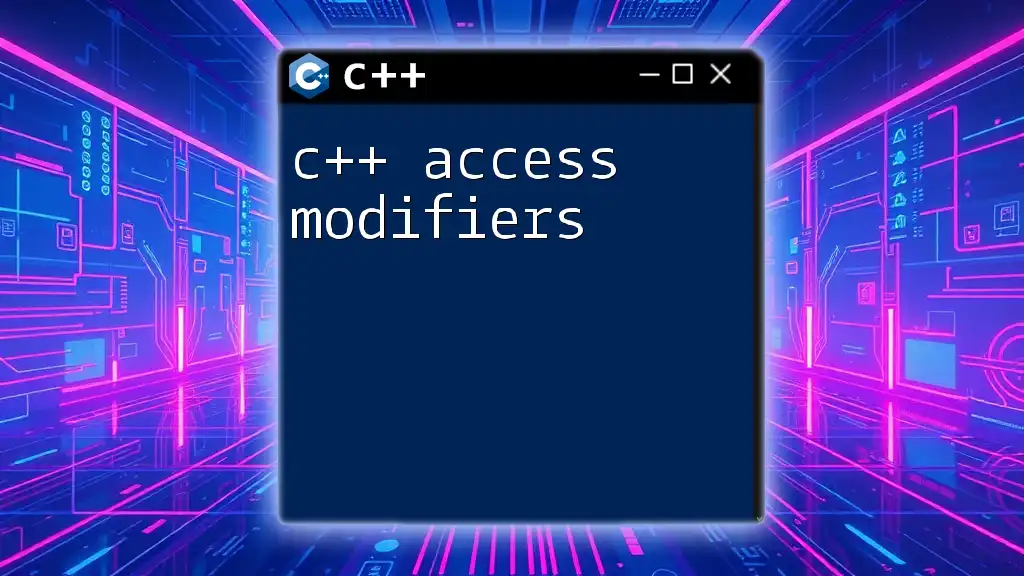
Situations Leading to the Error
Common Scenarios
During coding in C++, you may encounter the "expression must be a modifiable lvalue" error under certain conditions. Some common scenarios arise from attempting to assign a value to non-modifiable lvalues or using temporary objects.
For example, assigning to a non-modifiable lvalue:
const int z = 5;
z = 10; // Error
Here, attempting to assign `10` to `z` results in an error as `z` has been declared as `const` and cannot be modified.
Another common error occurs when trying to assign a value to a temporary object:
(x + 1) = 5; // Error: (x + 1) is a temporary (rvalue)
In this example, the expression `(x + 1)` produces a temporary result and thus cannot be treated as an lvalue.
Why These Errors Occur
Understanding the rules imposed by the C++ language around lvalues and rvalues is crucial. The C++ compiler prevents modifications to expressions that do not conform to rules of modifiability for both efficiency and logical consistency, preserving the integrity of constants and temporaries.
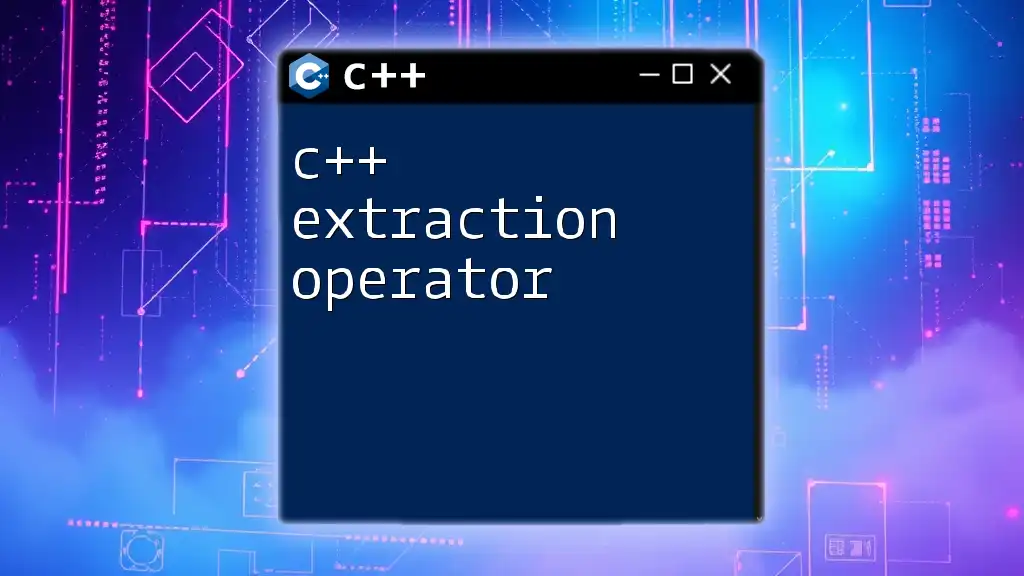
How to Identify Modifiable Lvalues
Syntax for Understanding Lvalues and Rvalues
To effectively differentiate between lvalues and rvalues, you can use `decltype`, a powerful feature in C++. By employing it, you can ascertain the nature of an expression in terms of modifiability:
decltype(x) a; // 'a' is of type int, an lvalue
By examining the result of `decltype`, you can quickly determine whether a variable can be modified.
Best Practices for Ensuring Modifiable Lvalues
- Variable Declaration: Always declare variables without the `const` qualifier if you intend to modify them later.
- Temporary Objects: Be cautious with expressions that yield temporary objects. Remember that operations like addition (`+`) or multiplication (`*`) return rvalues.
By practicing these guidelines, you can avoid many common pitfalls related to modifiable lvalues in C++.
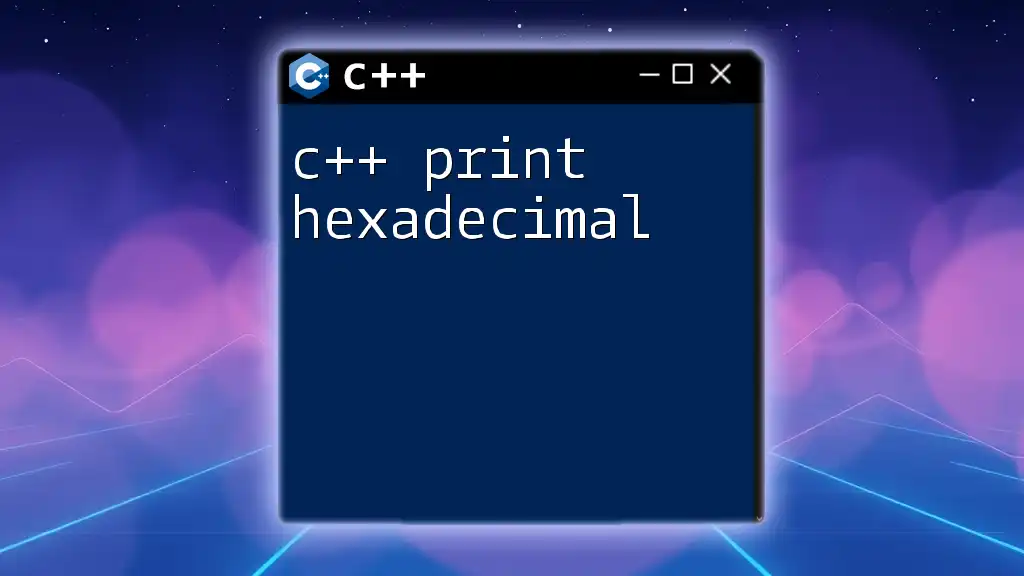
Examples of Expressions and Their Lvalue Status
Example Code Snippets
Let's explore some clear examples to illustrate the concept further.
Modifiable Lvalues
int a = 10; // 'a' is modifiable
a += 5; // OK: 'a' can be modified
In this case, `a` can be modified freely, confirming its status as a modifiable lvalue.
Non-Modifiable Lvalues
const int b = 20; // 'b' is non-modifiable
b += 5; // Error: cannot modify a const variable
Here, the inability to modify `b` clearly demonstrates that it is considered non-modifiable due to its `const` designation.
Analyzing the Code with Comments
Understanding why certain expressions are classified as lvalues or rvalues is pivotal. Using comments in your code can clarify these points:
int num = 100; // 'num' is an lvalue
num += 10; // 'num' can be modified, valid operation
const int constNum = 200; // 'constNum' is a non-modifiable lvalue
constNum += 50; // Error: cannot modify a const variable
Descriptive comments help to aid comprehension for both you and any collaborators.
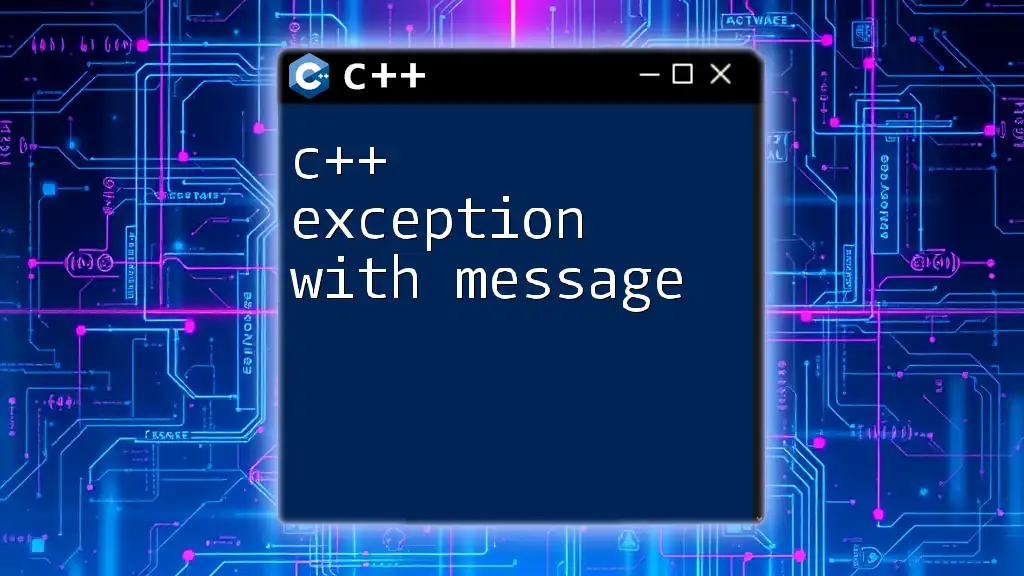
How to Fix "Expression Must Be a Modifiable Lvalue" Errors
Steps to Resolve the Error
When you encounter the “expression must be a modifiable lvalue” error, consider the following steps to resolve it:
- Review Variable Declaration: Examine the variable's declaration to ensure it is not marked as constant.
- Change the Variable Type: If you need a variable to be modifiable, remove the `const` qualifier.
- Use Pointers or References: If you need to modify an object indirectly, consider using pointers or references.
Practical Examples of Fixing the Error
Consider the following problematic code:
const int c = 30;
c = 40; // Error
To correct this error, you can modify your variable as follows:
int c = 30; // Removed const to allow modification
c = 40; // No Error now, as 'c' is modifiable
This change ensures that `c` can be changed without causing a compilation error.
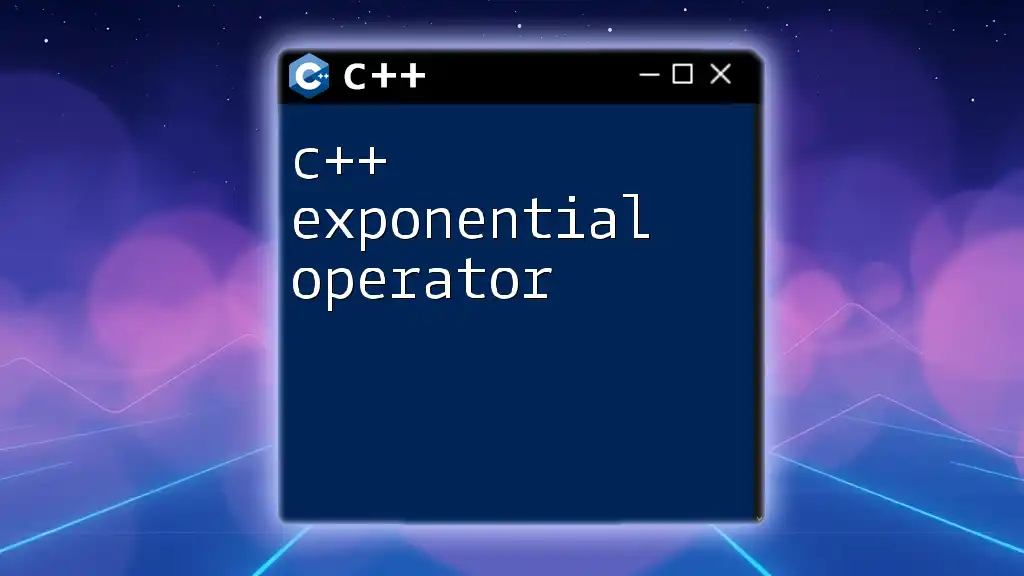
Conclusion
Understanding the intricacies of C++ expression must be a modifiable lvalue is vital for effective programming. Recognizing lvalues and rvalues will help you write clearer, more efficient code. Practice identifying these expressions will equip you with the necessary knowledge to avoid common pitfalls.
Engage with the material, ask questions, and continuously explore the depth of C++—the journey to mastering this language is as important as the destination.