In C++, exceptions allow you to handle errors gracefully, and you can throw an exception with a message using the `throw` keyword followed by a `std::runtime_error` to provide a clear explanation of the issue.
Here's an example:
#include <iostream>
#include <stdexcept>
int main() {
throw std::runtime_error("An error occurred while processing the request.");
return 0;
}
Understanding Exceptions in C++
What is an Exception?
In C++, an exception is a runtime error that occurs during the execution of a program, disrupting the flow of control. Unlike conventional errors, exceptions provide a structured way to manage unexpected situations, allowing developers to respond appropriately.
Types of Exceptions
In C++, exceptions can be categorized into two main types:
- Standard exceptions: Predefined exception classes provided by the C++ Standard Library, such as `std::runtime_error` and `std::out_of_range`.
- User-defined exceptions: Custom exception classes created to handle specific error situations unique to an application.
Understanding when to use standard exceptions versus user-defined exceptions is crucial for effective error management.
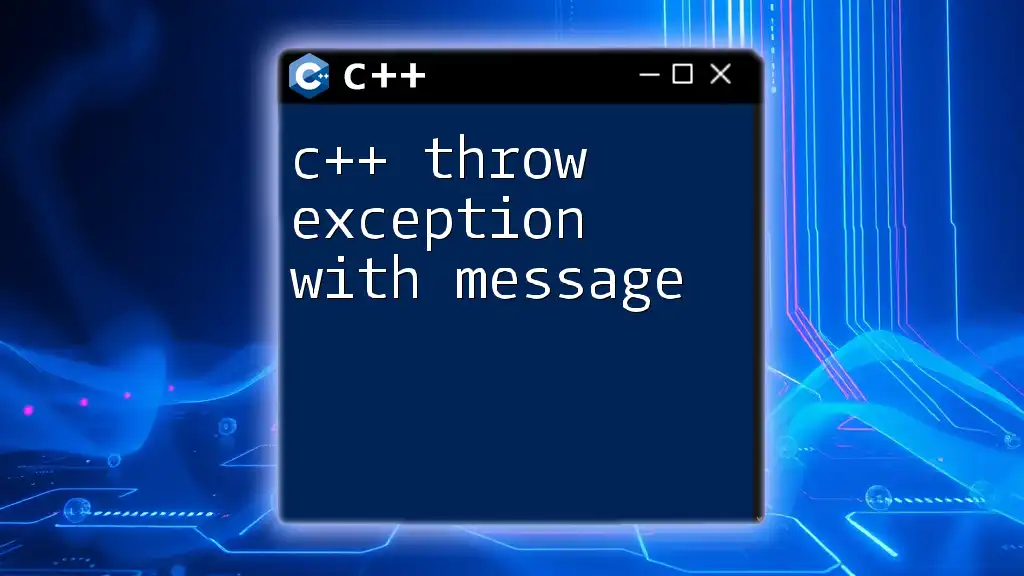
The Exception Handling Mechanism in C++
Try-Catch Blocks
Exception handling in C++ relies on try-catch blocks. The syntax is straightforward:
try {
// Code that may throw an exception
} catch (const std::exception& e) {
// Code to handle the exception
}
When a block of code within the `try` section throws an exception, control is transferred to the corresponding `catch` block, preventing the program from crashing. This mechanism allows developers to maintain stability in their applications.
Throwing Exceptions
To signal an error condition, you can throw an exception using the `throw` keyword followed by an instance of an exception class. For example:
throw std::runtime_error("Error message");
It’s essential to encapsulate meaningful messages within these exceptions, making it easier to understand the issue when it occurs.
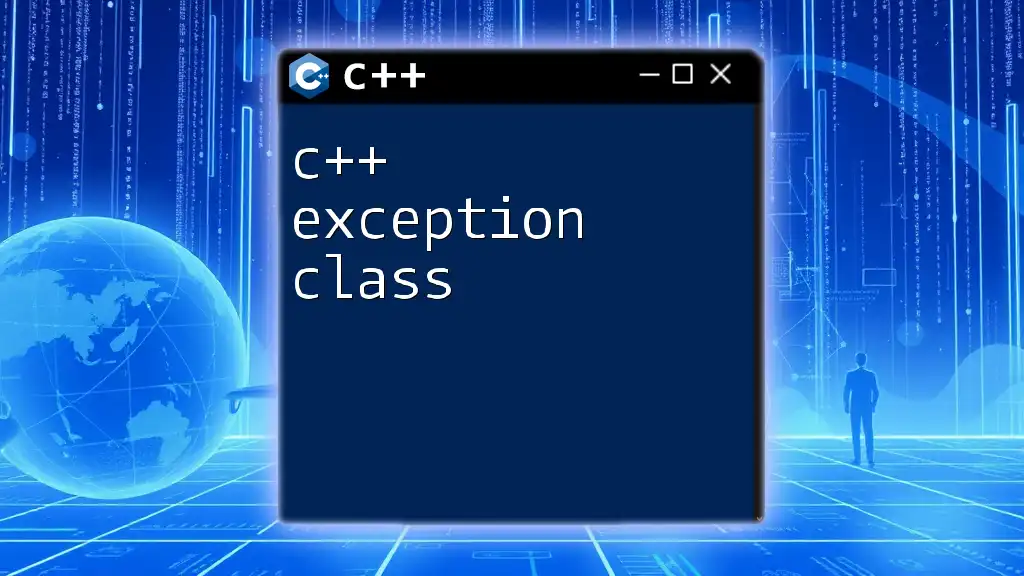
Creating Custom Exception Classes
Why Create Custom Exception Classes?
While standard exceptions cover many scenarios, there are cases where they may not suffice. Creating custom exception classes allows developers to provide specific context and details about errors tailored to their applications.
Structure of a Custom Exception Class
A basic custom exception class typically includes a constructor that accepts a message and inherits from `std::exception`. Here’s an example of how to structure such a class:
#include <exception>
#include <string>
class CustomException : public std::exception {
public:
explicit CustomException(const std::string& message) : msg_(message) {}
virtual const char* what() const noexcept { return msg_.c_str(); }
private:
std::string msg_;
};
This custom class enables developers to throw exceptions with context-sensitive messages, enhancing error handling in their applications.
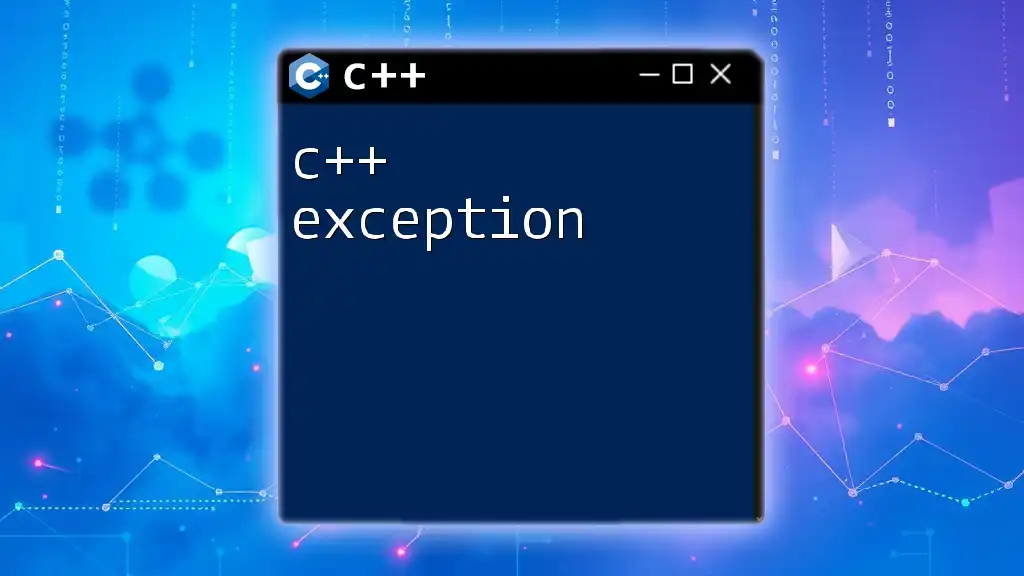
Catching Exceptions with Messages
Using Standard Exceptions
Here's how to throw and catch a standard exception with a message:
try {
throw std::runtime_error("A runtime error occurred!");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl; // Output: A runtime error occurred!
}
This demonstrates the ease of managing standard exceptions equipped with messages that provide crucial information about the error.
Catching Custom Exceptions
Custom exceptions can also be thrown and caught similarly. Here’s an example:
try {
throw CustomException("This is a custom error message.");
} catch (const CustomException& e) {
std::cout << e.what() << std::endl; // Output: This is a custom error message.
}
This showcases the power of custom exceptions in communicating specific issues clearly and effectively.
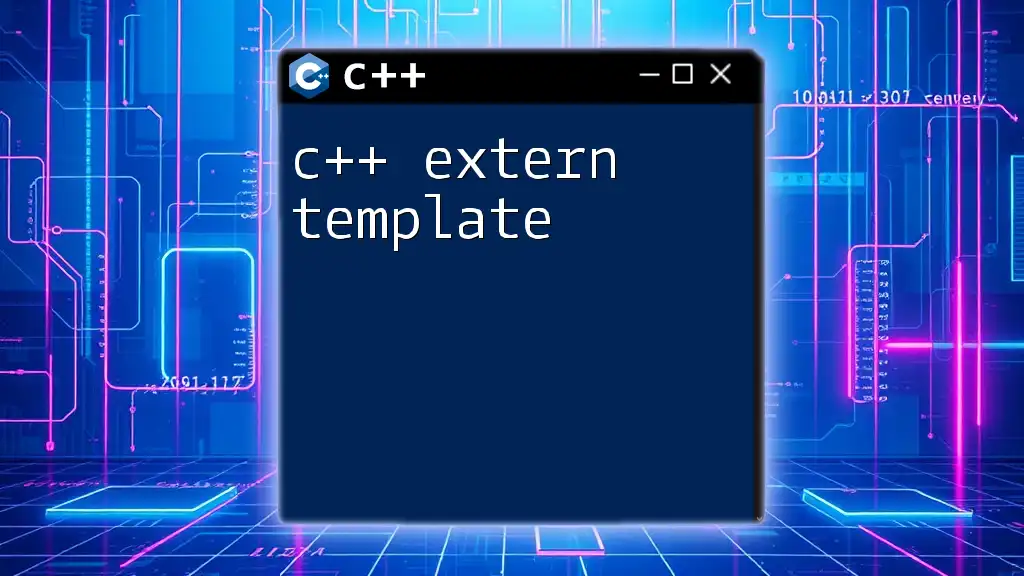
Best Practices for Exception Messages
Writing Clear and Concise Messages
When developing exception messages, clarity and conciseness are vital. Avoid technical jargon, and focus on articulating the problem in a way that’s easily understandable for users and developers alike.
Carriage Return and Special Characters
Care should also be taken when incorporating special characters in exception messages. C++ handles formatting characters, such as newline characters, gracefully. For instance:
throw std::runtime_error("Error: Invalid input\nPlease try again.");
This method improves the readability of messages, especially when printed to logs or the console.
Logging Exceptions
In addition to displaying messages to users, it's beneficial to log exceptions for debugging purposes. Here’s a simple example of logging an exception message to a file:
std::ofstream log_file("error_log.txt", std::ios::app);
log_file << e.what() << std::endl;
Logging provides a history of errors, enabling developers to trace issues back to their source effectively.
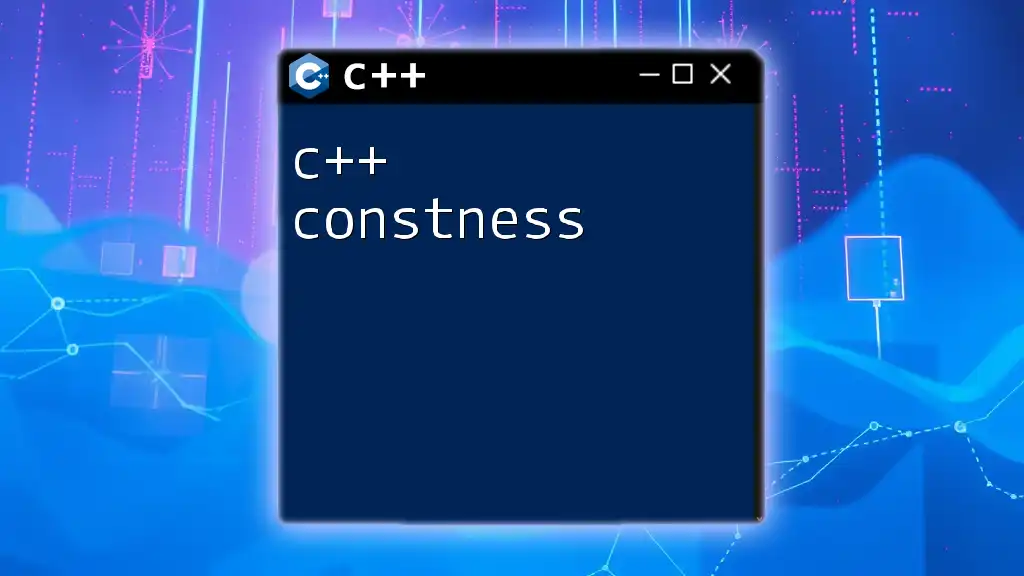
Common Pitfalls to Avoid
Overusing Exceptions
One significant pitfall to avoid is the overuse of exceptions. They should not be used for standard control flow. Using exceptions for non-exceptional situations can lead to performance degradation and make code harder to read. Instead, consider other error handling mechanisms, such as return values or error codes.
Ignoring Exception Messages
Ignoring the importance of exception messages can be detrimental. Each exception contains critical information that can help diagnose issues. Developers must not only throw exceptions but also ensure they are handled and logged efficiently.
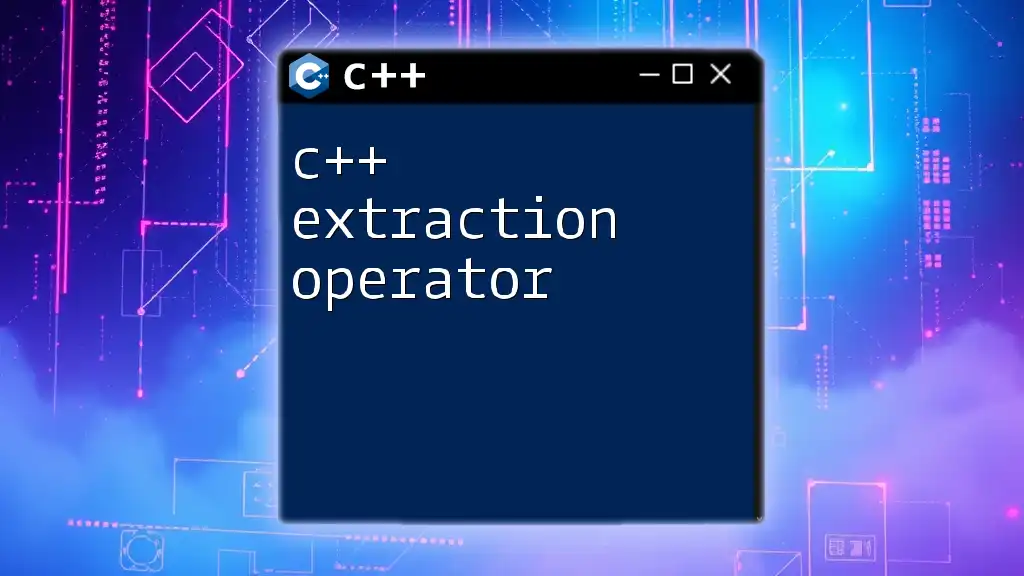
Conclusion
In summary, mastering C++ exceptions with messages is essential for robust error handling and maintaining application stability. By effectively throwing and catching exceptions, including writing meaningful messages, and creating custom exception classes, developers can enhance their C++ programming skills significantly. Practicing these concepts will yield applications that are prepared to handle unexpected situations smoothly.
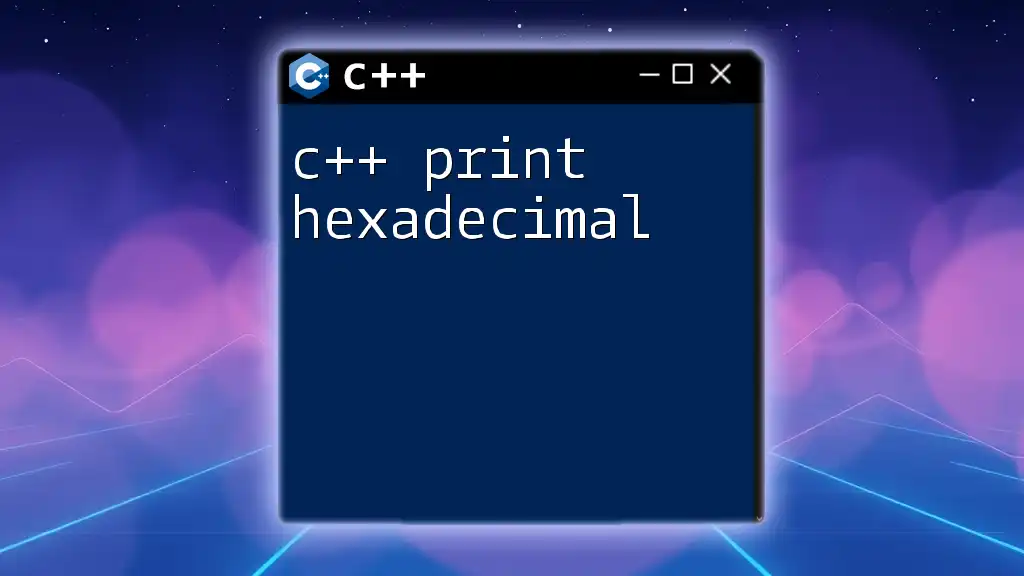
Additional Resources
For further reading on C++ exception handling, consider checking reputable online resources, documentation, and books dedicated to C++ programming. These will help deepen your understanding and encourage best practices in exception management.