To get the current timestamp in C++, you can use the `<chrono>` library along with `<ctime>` to retrieve and print the time in a human-readable format. Here's a simple code snippet demonstrating this:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t currentTime = std::chrono::system_clock::to_time_t(now);
std::cout << "Current Timestamp: " << std::ctime(¤tTime);
return 0;
}
Understanding Timestamps in C++
What is a Timestamp?
A timestamp is a sequence of characters or encoded information that represents a specific date and time. In programming, timestamps are crucial for numerous applications such as logging events, tracking user activity, and performing calculations involving time.
Why Use Timestamps in C++?
Using timestamps in C++ has several practical applications. They help in debugging by recording the exact time when an event occurred, allowing developers to trace issues more effectively. Additionally, timestamps enable the tracking of time intervals, which is essential for timers, schedulers, and performance monitoring. Thus, understanding how to work with timestamps in C++ can enhance the robustness of your applications.
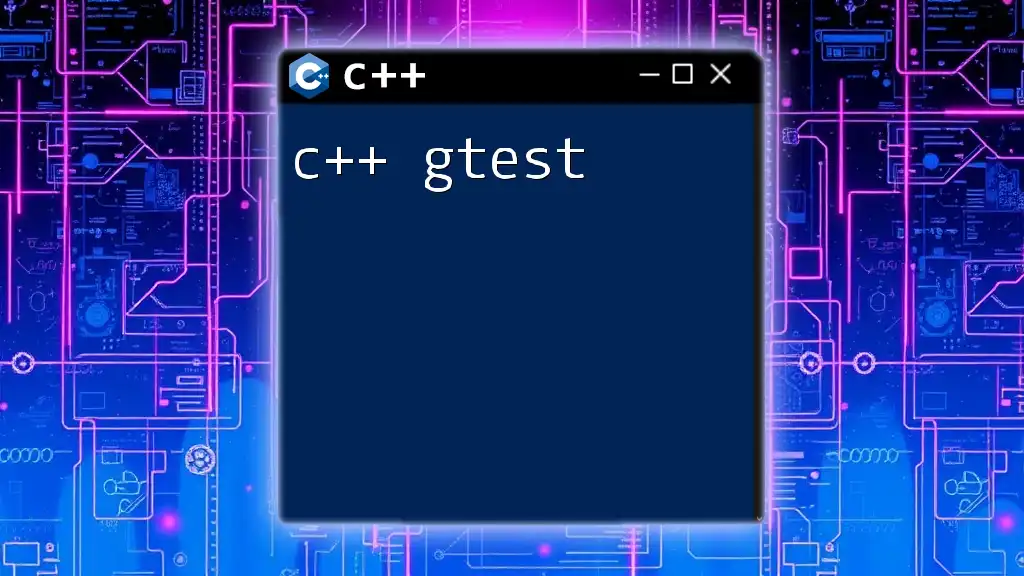
Getting the Current Time in C++
C++ Standard Library & Time Functions
The C++ standard library provides the `<chrono>` header that encompasses several functionalities related to clock and time. This library includes three primary clocks:
- `system_clock`: Represents the wall clock time, which is the time as we know it and is affected by system clock adjustments.
- `steady_clock`: Provides time intervals that are not affected by system clock changes and are therefore suitable for measuring elapsed time.
- `high_resolution_clock`: Typically represents the most precise clock available, often implemented on the basis of `steady_clock`.
With these clocks, getting timestamps in C++ becomes straightforward.
Getting Current Time with `chrono`
Using `chrono::system_clock`
To get the current timestamp in C++, you can leverage the `system_clock`. The following code snippet demonstrates how to retrieve the current timestamp.
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::system_clock::now();
std::cout << "Current time: " << std::chrono::duration_cast<std::chrono::seconds>(now.time_since_epoch()).count() << " seconds since epoch.\n";
return 0;
}
In this code, `now` captures the current time using `system_clock::now()`. The `time_since_epoch()` function gives the duration since the epoch (January 1, 1970), and we convert it into seconds for better readability.
Formatting Current Time
Converting Timestamp to Human-Readable Format
Often, it's beneficial to present timestamps in a human-readable format. By utilizing both `chrono` and `ctime`, you can convert a timestamp into a standard date and time representation:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_c = std::chrono::system_clock::to_time_t(now);
std::cout << "Current local time: " << std::put_time(std::localtime(&now_c), "%F %T") << '\n';
return 0;
}
In this example, we convert the `now` variable into a `std::time_t` object using `to_time_t()`, which represents the time in seconds since the epoch. We then use `std::put_time` with `std::localtime` to format the time into a more readable string.
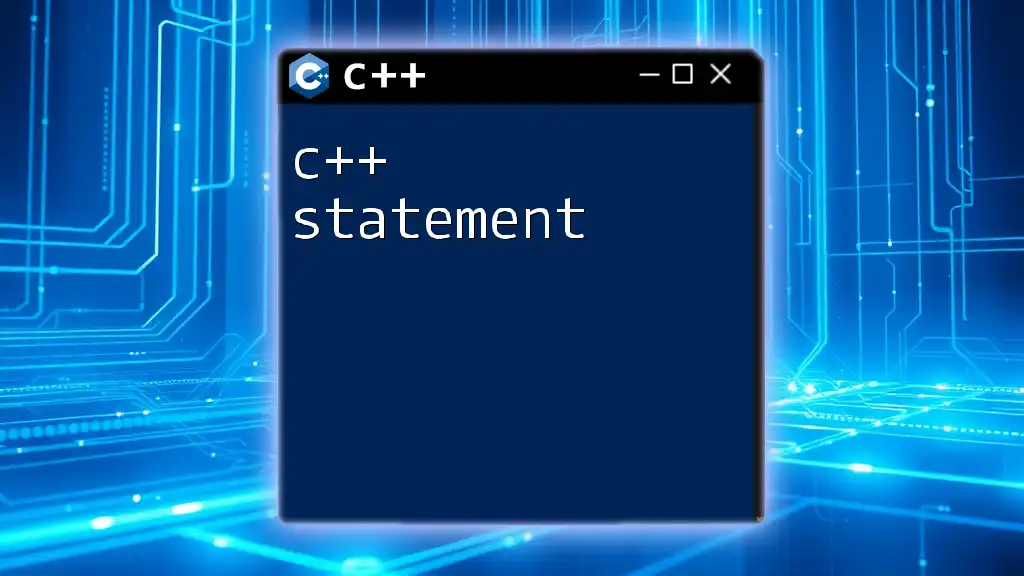
Getting a Timestamp from a Past Time
Using `std::chrono` to Get Past Timestamps
You can also derive timestamps from defined past dates. Here’s how you can create a timestamp for a given date:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
std::tm tm = {};
tm.tm_year = 2021 - 1900; // Year since 1900
tm.tm_mon = 1 - 1; // Month (0-11)
tm.tm_mday = 1; // Day of the month
std::time_t past_time = std::mktime(&tm);
std::cout << "Timestamp for 2021-01-01: " << past_time << '\n';
return 0;
}
In the above code, we define a `std::tm` structure that represents the date January 1, 2021. The year is adjusted by subtracting 1900, and months are zero-based (January is 0). Using `std::mktime`, we convert this structure to a timestamp.
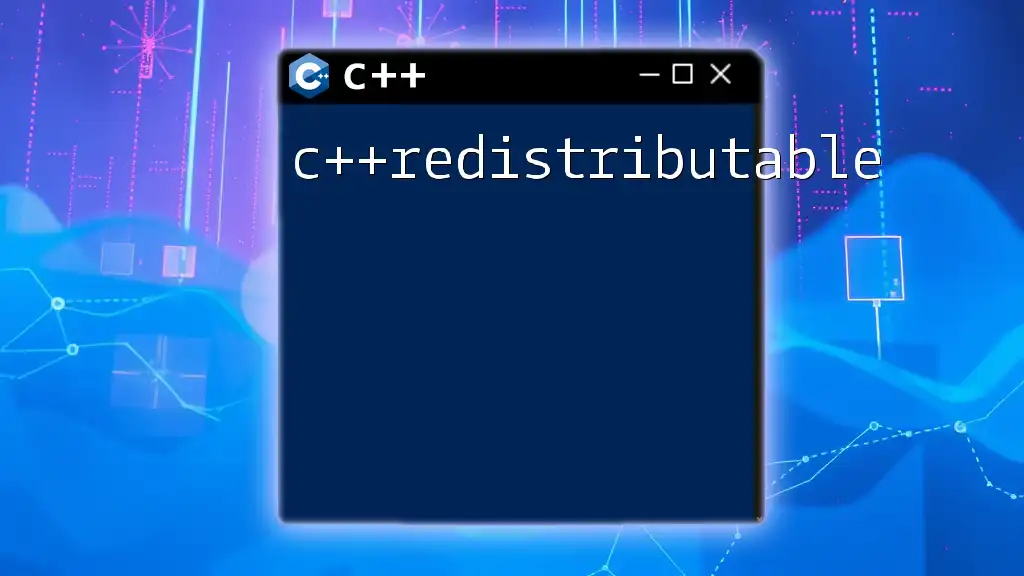
Using C++ for Time Intervals and Timestamps
Measuring Time Intervals
The `steady_clock` can be used for time measurement, providing intervals unaffected by any changes to the system clock. Consider the following example of measuring the execution time of a function:
#include <iostream>
#include <chrono>
void doSomeWork() {
// Simulate processing
for (volatile int i = 0; i < 100000000; ++i);
}
int main() {
auto start = std::chrono::steady_clock::now();
doSomeWork();
auto end = std::chrono::steady_clock::now();
std::chrono::duration<double> elapsed_seconds = end - start;
std::cout << "Time taken: " << elapsed_seconds.count() << " seconds\n";
return 0;
}
This code snippet measures how long the `doSomeWork` function takes to execute. Here, `steady_clock::now()` captures the start and end times, and by subtracting these values, we obtain the elapsed time in seconds.
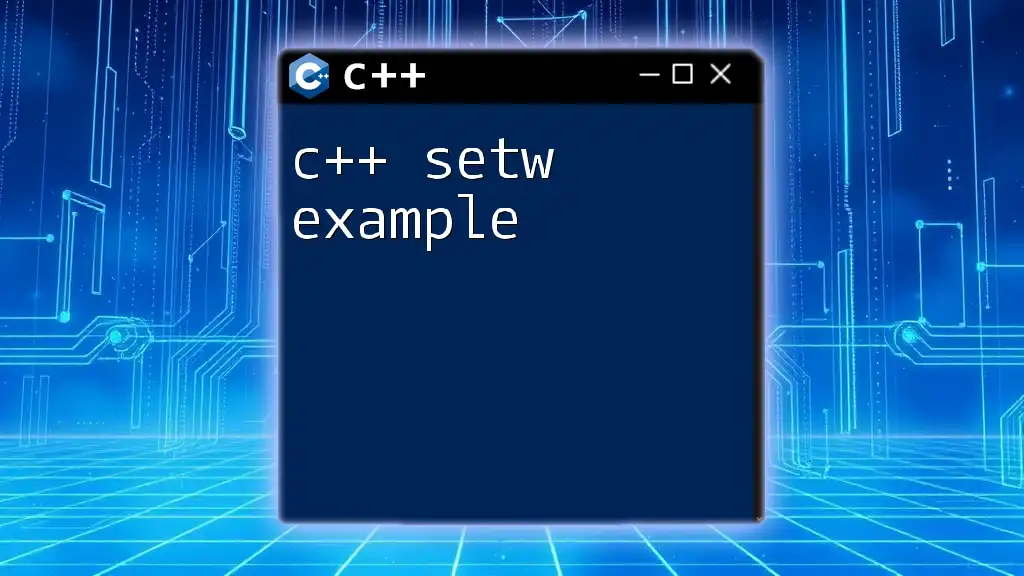
Best Practices for Handling Timestamps in C++
Handling Time Zones
When dealing with timestamps, it's crucial to manage time zones properly. C++ does not natively support time zones, but libraries like date.h or `boost::chrono` provide functionalities to handle time zones effectively. Familiarizing yourself with these libraries can enhance your application's timezone handling.
Avoiding Common Pitfalls
When working with timestamps, be aware of issues like Daylight Saving Time adjustments and leap seconds. These can affect the accuracy of timestamps, especially in logging scenarios. It’s smart to verify that your chosen library or approach correctly accounts for these factors to avoid unexpected results.
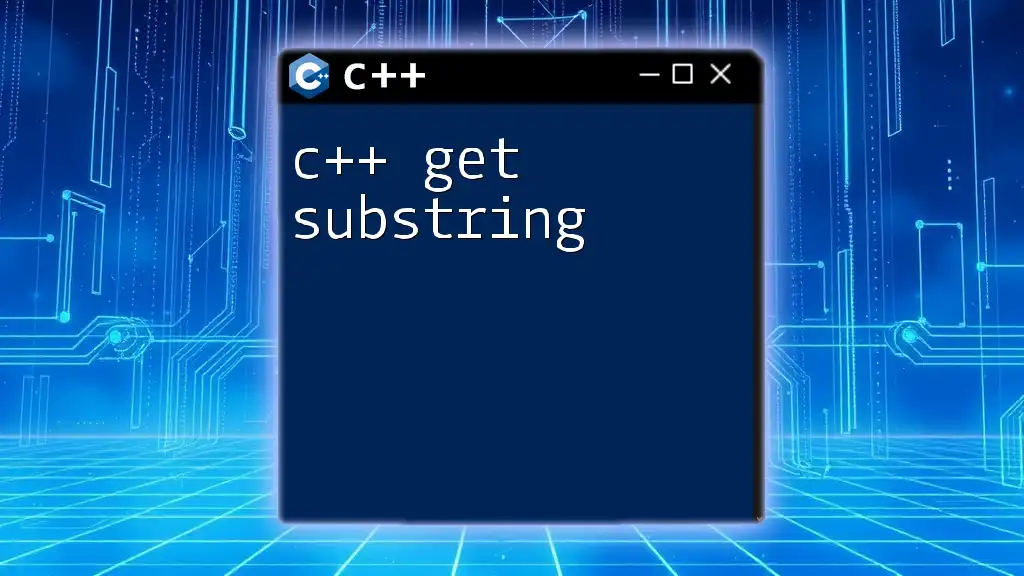
Conclusion
Understanding how to get and manipulate timestamps in C++ significantly boosts your programming capabilities. By mastering the functions provided in the standard library, you can effectively track events, measure execution time, and present time in a readable format. Exploring further into libraries that handle time-related functionalities will further refine your skill set in C++.
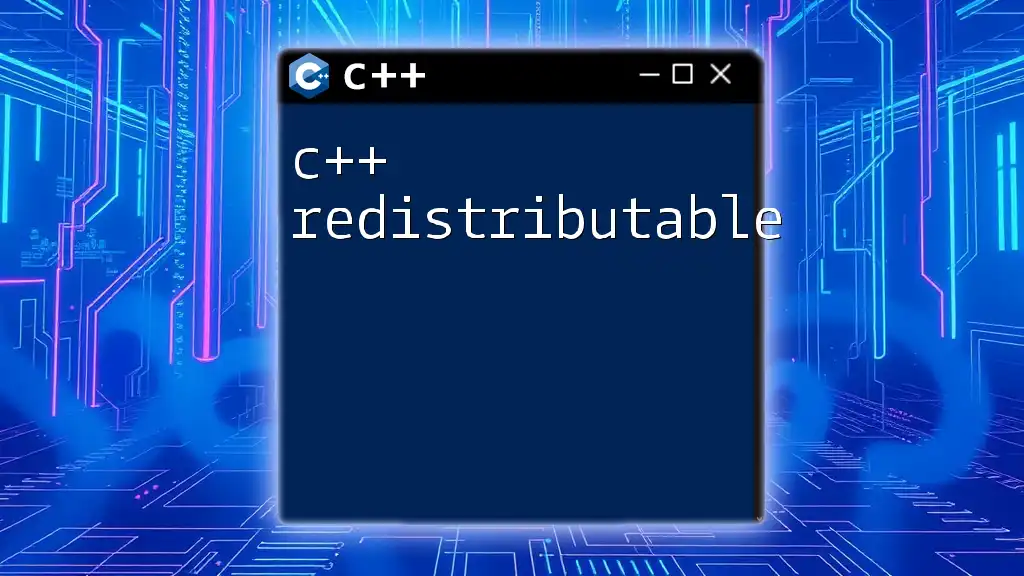
Call to Action
Stay tuned for more insights and tutorials on C++, designed to enhance your programming journey and help you master the intricacies of this powerful language!