In C++, you can throw an exception with a custom message using the `throw` keyword followed by an exception object that contains the message, like this:
throw std::runtime_error("This is an error message.");
What is Exception Handling?
Exception handling is a crucial feature in programming that allows developers to manage errors and exceptional conditions more effectively. It enables a program to catch errors gracefully, maintain control flow, and prevent crashes, enhancing the robustness of your application.
Importance
The significance of exception handling in C++ cannot be overstated. It helps improve the maintainability of your code by separating error handling from regular logic and provides a clear mechanism for signaling problems.
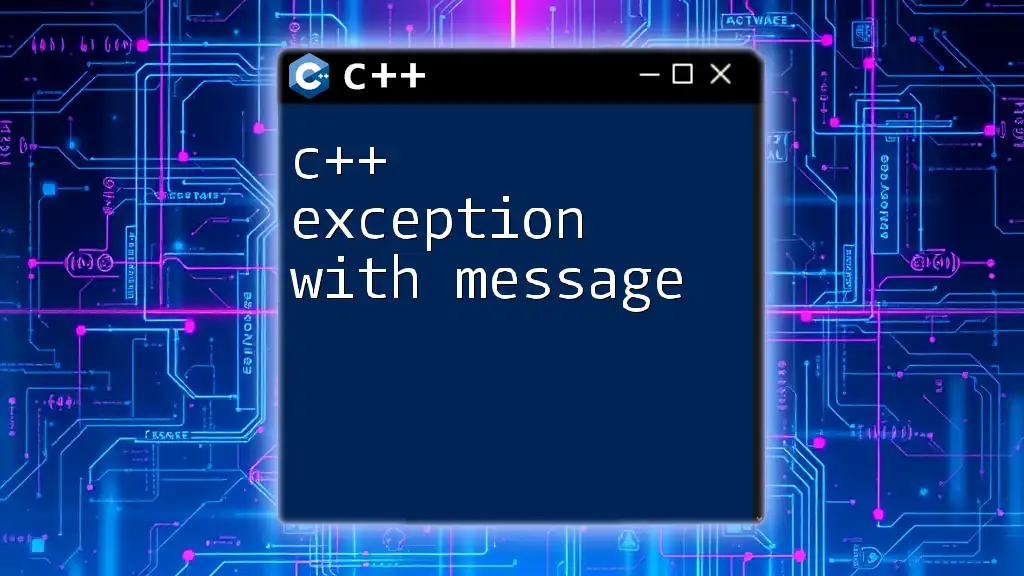
Overview of C++ Exceptions
Standard Exception Hierarchy
In C++, exceptions are organized in a standard hierarchy defined by the Standard Template Library (STL). The base class for all exceptions is `std::exception`, from which various specialized exception classes derive. These built-in exceptions include:
- `std::runtime_error`
- `std::logic_error`
- `std::bad_alloc`
Understanding this inheritance hierarchy allows developers to effectively catch and manage exceptions.
Built-in Exception Classes
C++ provides several built-in exception classes that developers can utilize to capture errors that are specific to their operations or the standard library. For instance, `std::runtime_error` is often used for runtime errors that can occur during program execution, while `std::logic_error` indicates errors in logic that could have been caught earlier in the program's execution.
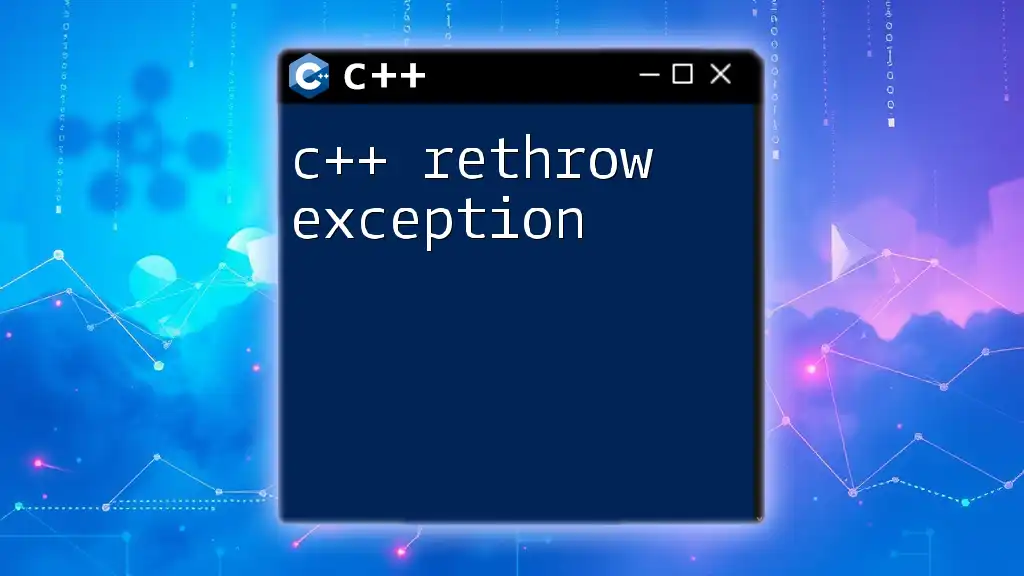
Understanding the `throw` Keyword
What is the Throw Statement?
The `throw` keyword is used in C++ to signal an exception. When an error condition occurs, you can use `throw` followed by an object that describes the exception.
Syntax
The basic syntax of a throw statement is as follows:
throw exception_object;
When to Use Throw?
You should use the `throw` statement when an operation fails and you cannot recover from the error. Common scenarios include:
- Invalid user input
- Failed memory allocations
- Errors in file handling
Best Practices
While throwing exceptions is essential for robust error handling, it should be done judiciously. Adhering to best practices, such as only throwing exceptions for exceptional conditions and ensuring that exceptions contain meaningful information, is crucial for maintaining clean and understandable code.
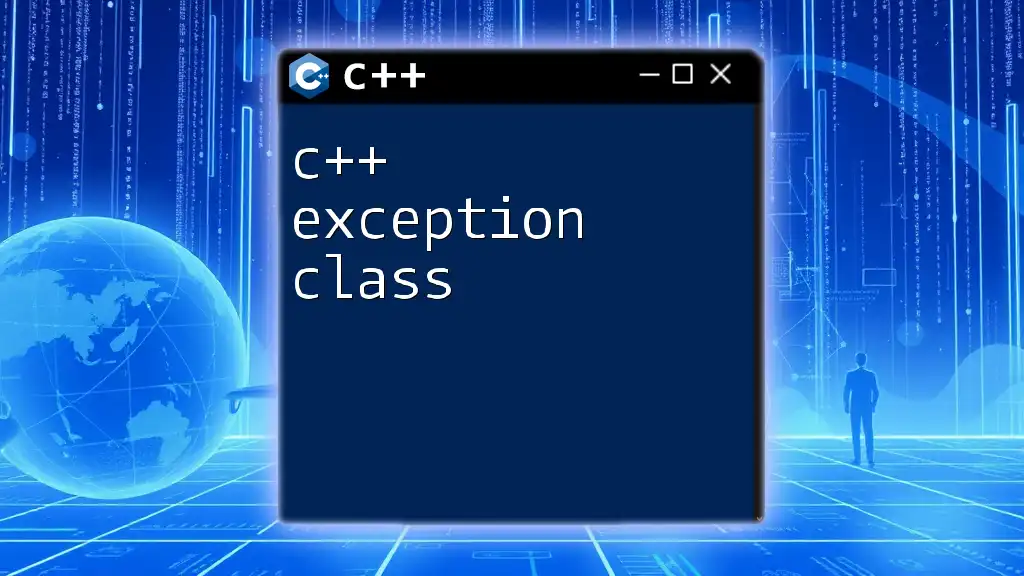
Constructing Exception Messages
Importance of Exception Messages
Providing clear and detailed exception messages is essential for effective debugging. Well-constructed messages can significantly enhance the clarity of the error, making it easier for developers to identify and fix issues.
Creating Custom Exception Messages
When an exception is thrown, the message passed along with it should be informative. A good exception message typically includes:
- The nature of the error
- Relevant data from the context
- Guidance or suggestions for resolution
By following these guidelines, you can create exception messages that significantly improve maintainability.
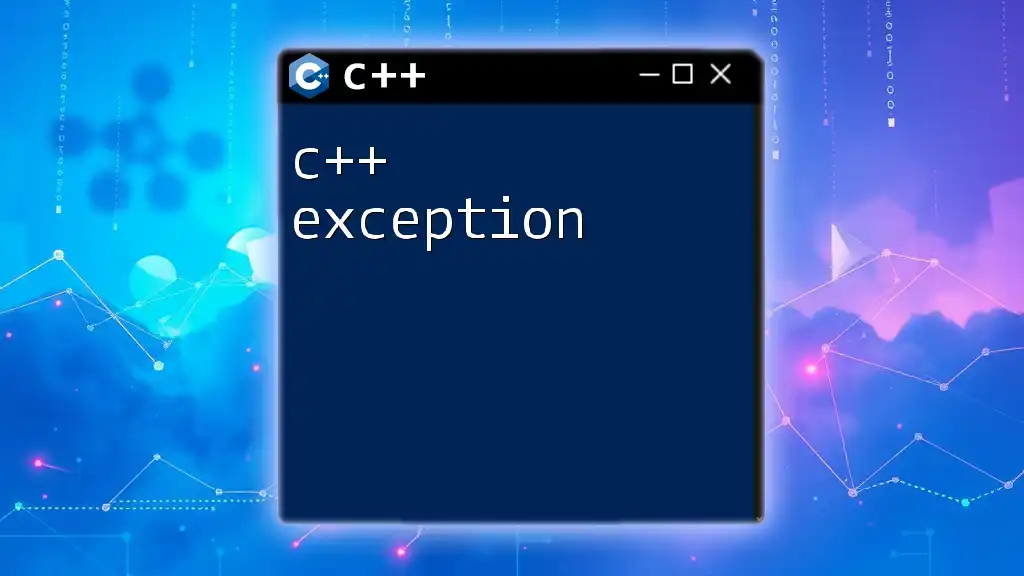
How to Throw an Exception with a Custom Message
Basic Syntax of Throwing an Exception
Throwing an exception with a message involves using one of the standard or custom exception classes. Here’s an example using `std::runtime_error`:
throw std::runtime_error("This is a runtime error message");
Using Custom Exception Classes
Creating your own exception classes can help encapsulate specific error conditions that are unique to your application.
What are Custom Exceptions?
Custom exceptions derive from `std::exception` and allow for more tailored error handling.
Creating a Custom Exception Class
To create a custom exception, define a new class, and override the `what()` method to return a message. Here’s an example:
class MyCustomException : public std::exception {
private:
std::string message;
public:
MyCustomException(const std::string& msg) : message(msg) {}
const char* what() const noexcept override {
return message.c_str();
}
};
Throwing a Custom Exception
You can now throw your custom exception like this:
throw MyCustomException("This is a custom exception message");
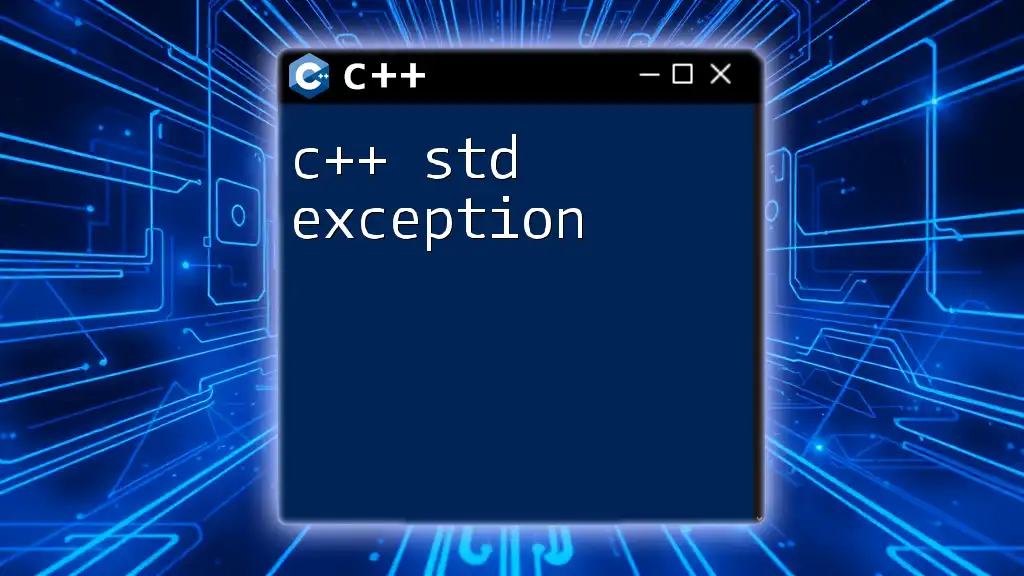
Catching Exceptions
Basic Catch Blocks
Using a `try` block allows you to monitor code that might throw an exception. When an exception occurs, it can be caught in a corresponding `catch` block.
Syntax of Catch
Here’s a simple example:
try {
// Code that may throw an exception
} catch (const std::runtime_error& e) {
std::cerr << "Caught runtime error: " << e.what() << std::endl;
}
Catching Custom Exceptions
Custom exceptions can also be caught using their specific class. Here’s how you do it:
try {
throw MyCustomException("This is a custom error!");
} catch (const MyCustomException& e) {
std::cerr << "Caught custom exception: " << e.what() << std::endl;
}
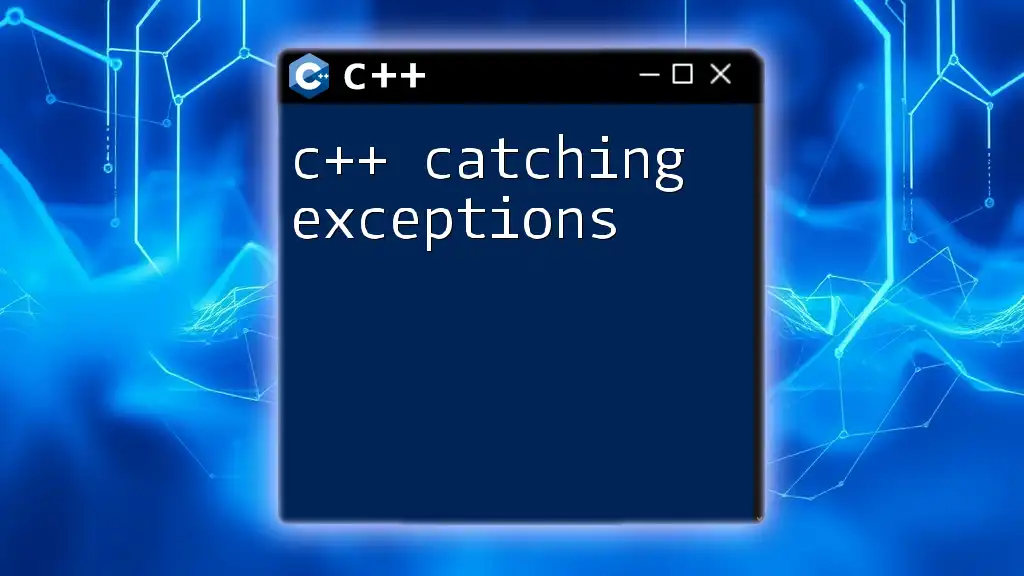
Best Practices for Exception Handling
Avoiding Overusing Exceptions
While exceptions are powerful, overusing them can lead to performance issues, particularly in high-frequency or critical performance sections of your code. Instead, consider using error codes for predictable conditions and reserve exceptions for truly exceptional circumstances.
Documenting Exceptions
It is vital to document which exceptions a function may throw. This practice not only aids other developers who might use your code but also enhances the clarity of your design intent.
Catching By Reference
Catching exceptions by reference (`const std::exception&`) is a best practice as it avoids unnecessary copies of the exception object, improving efficiency and maintaining the original state of the exception.
catch (const std::exception& e)
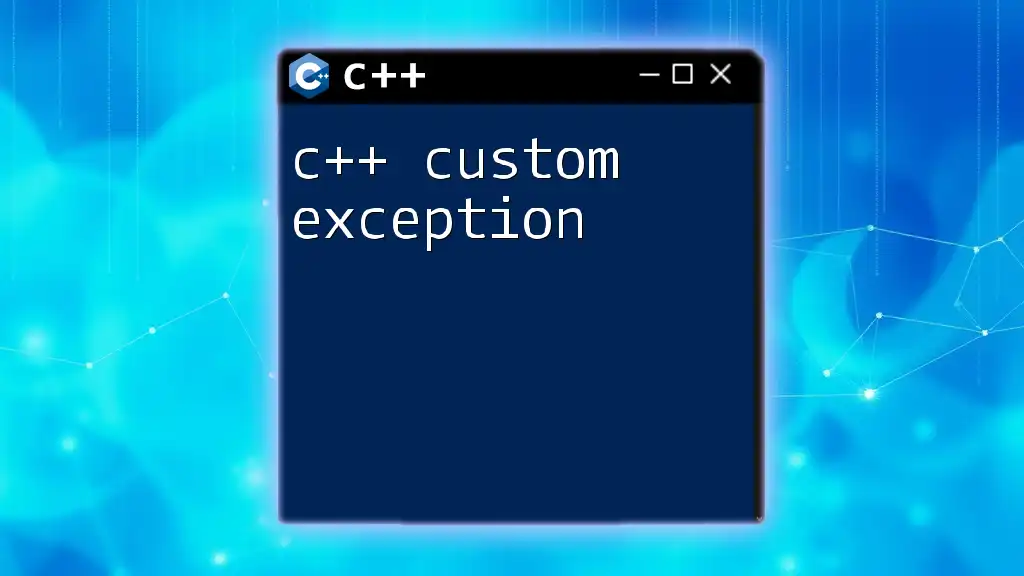
Conclusion
In this article, we explored the concept of c++ throw exception with message. From understanding the importance of exception handling to effectively throwing and catching exceptions, we emphasized the need for clarity in messaging and best practices.
Effective error handling is a critical component of writing robust software. By implementing these practices, you not only enhance the quality of your code but also provide better experiences for those who use or maintain it.
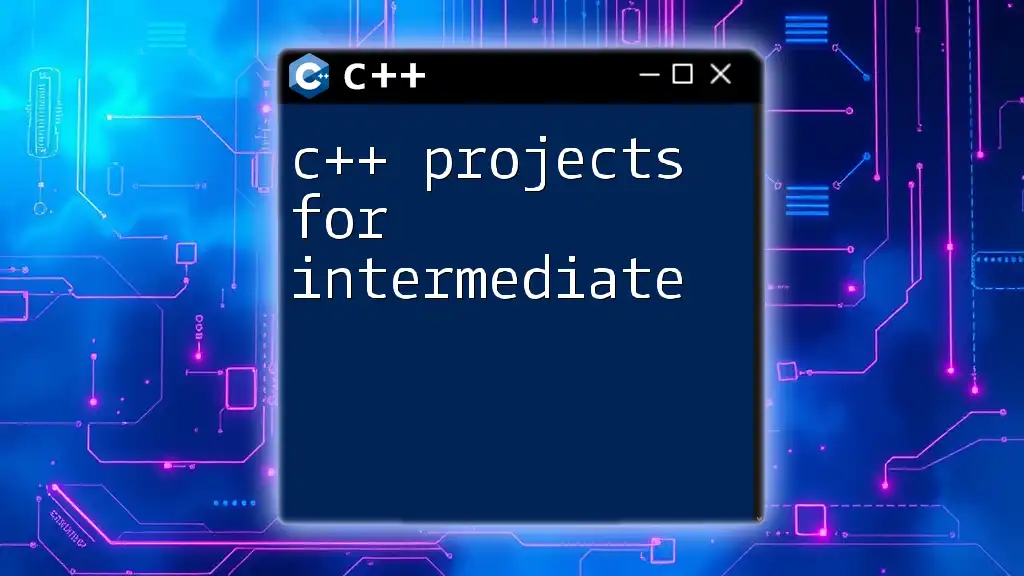
Additional Resources
For readers interested in furthering their knowledge of C++ and exception handling, explore the official C++ documentation, relevant online tutorials, and coding communities where knowledge sharing thrives. Recommended readings could include specialized books on C++ programming and error handling strategies.