You can initialize a C++ vector with a specific size by using the constructor that takes the size as an argument, optionally setting an initial value for its elements.
#include <vector>
std::vector<int> myVector(10, 0); // Initializes a vector of size 10 with all elements set to 0
What is a Vector in C++?
Definition
In C++, a vector is a dynamic array that can change in size as elements are added or removed. It is part of the Standard Template Library (STL) and provides an easier and safer way to handle arrays. Unlike traditional arrays, where the size must be known at compile time, vectors can be resized during runtime.
Advantages of Using Vectors
Vectors come with numerous benefits that make them preferable to arrays in many scenarios:
- Dynamic Sizing: Vectors automatically resize themselves when elements are added or removed, which leads to more flexible and efficient code.
- Built-in Functions: They include numerous built-in functions for manipulation, such as `push_back`, `pop_back`, and `insert`.
- Easier Memory Management: Vectors handle memory allocation automatically, significantly reducing the risk of memory leaks and buffer overflows.
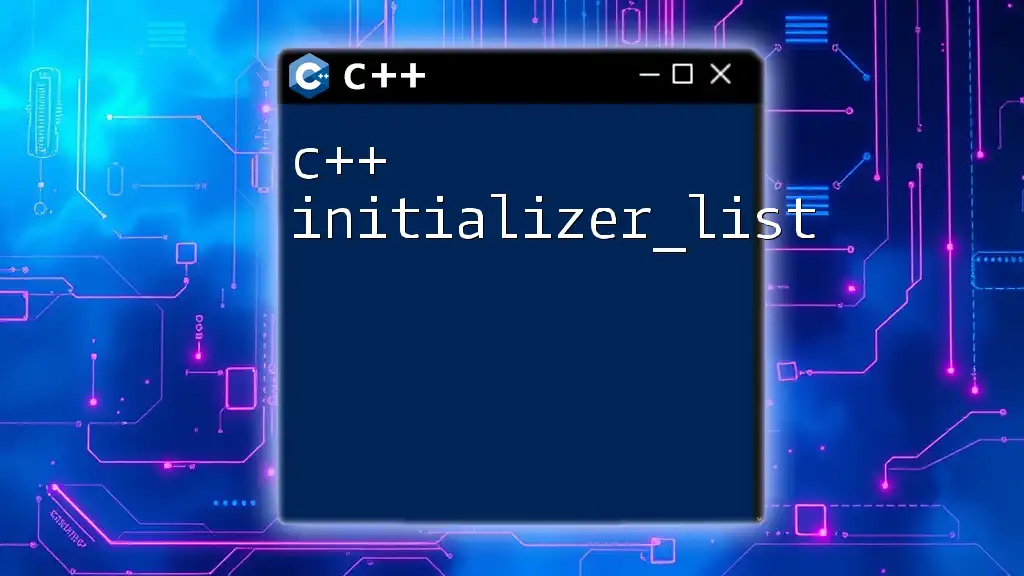
How to Initialize a Vector with Size
Syntax Overview
To initialize a vector with size, you use the following syntax:
std::vector<type> vectorName(size);
Where `type` is the data type of elements stored in the vector, `vectorName` is the name you assign to the vector, and `size` is the number of elements you want to initialize.
Using Default Initialization
When you initialize a vector with a specified size but no values, C++ defaults the elements to zero (for fundamental data types). This is known as default initialization.
Here's an example:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec(5); // Initializes a vector of size 5 with default values (0)
for (const auto& elem : vec) {
std::cout << elem << " "; // Output: 0 0 0 0 0
}
return 0;
}
In the above code, we have initialized a vector of size 5. All elements default to `0`.
Using Value Initialization
You can also initialize all elements of a vector to a specific value upon its creation. This is known as value initialization. The syntax for this is:
std::vector<type> vectorName(size, value);
Here's how it looks in practice:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec(5, 10); // Initializes a vector of size 5 with all elements set to 10
for (const auto& elem : vec) {
std::cout << elem << " "; // Output: 10 10 10 10 10
}
return 0;
}
In this example, a vector of size 5 is created, where every element is initialized to `10`. This is particularly useful when a specific starting value is required.
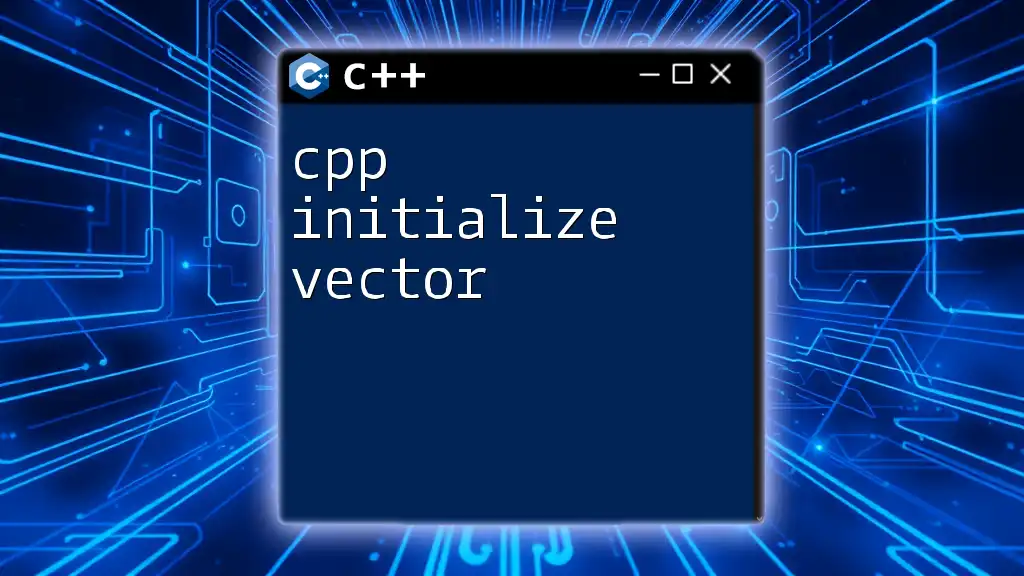
Important Considerations When Initializing Vectors
Memory Management
One of the significant advantages of vectors is their dynamic memory management. When elements are added, the vector automatically allocates memory and adjusts its size accordingly. However, keep in mind that frequent resizing can lead to performance overhead, especially if the vector scales repeatedly.
Properly managing vector size ensures optimal performance and minimizes memory reallocation.
Vector Size vs. Capacity
The size of a vector refers to the number of elements currently held, while capacity refers to the total amount of space allocated for the vector in memory. Vectors automatically manage their capacity, but understanding this distinction is vital, especially when optimizing performance.
Here’s a code snippet that illustrates the difference:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec(5);
std::cout << "Size: " << vec.size() << ", Capacity: " << vec.capacity() << std::endl;
return 0;
}
When this code runs, it displays the vector's current size and capacity. The vector’s capacity may be greater than its size to accommodate future growth without reallocating memory.
Resizing Existing Vectors
If you need to change the size of an already initialized vector, you can use the `resize()` method. This is incredibly powerful for adjusting the size of a vector based on your program's needs without creating a new vector.
Here's how to do it:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec(5);
vec.resize(10, 1); // Resizes the vector to size 10, with new elements initialized to 1
for (const auto& elem : vec) {
std::cout << elem << " "; // Output: 0 0 0 0 0 1 1 1 1 1
}
return 0;
}
This code snippet illustrates how to safely increase the size of a vector and initialize new elements to a specific value (`1` in this case).
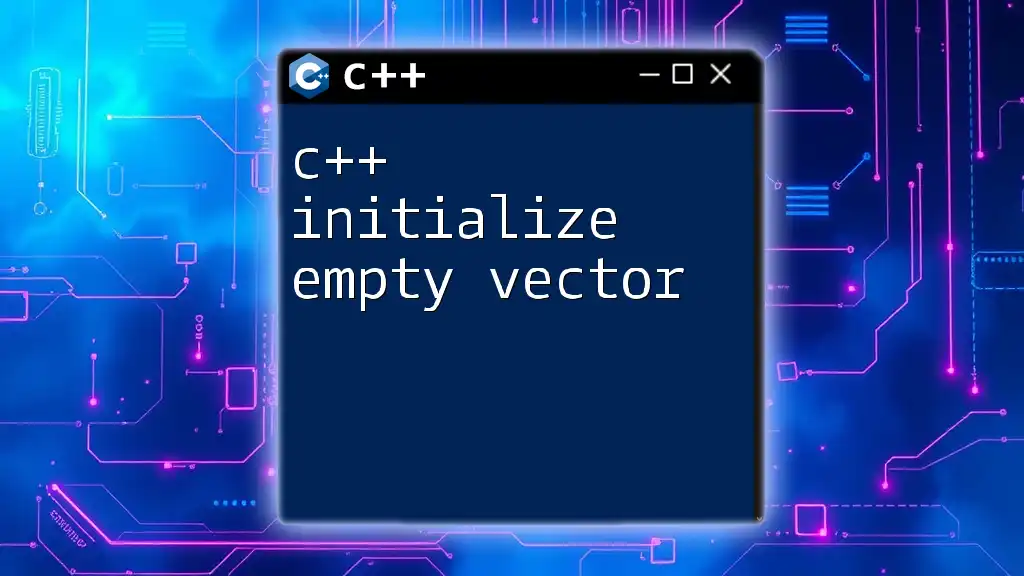
Common Use Cases for Initializing Vectors
Storing User Input
Vectors are often employed to store user input where the amount of data isn't predetermined. For instance, when reading scores or entries from users, you can initialize a vector of a specific size based on the expected input.
Working with Multi-Dimensional Vectors
When dealing with multi-dimensional data (like matrices), you can initialize a vector of vectors. This provides a convenient way to represent and manipulate table-like structures in C++.
Here’s an example of initializing a 2D vector with specified sizes:
#include <vector>
#include <iostream>
int main() {
std::vector<std::vector<int>> matrix(3, std::vector<int>(4, 0)); // 3 rows and 4 columns initialized to 0
for (const auto& row : matrix) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl; // Output: 0 0 0 0 (three times)
}
return 0;
}
In the above example, a 2D vector is initialized with 3 rows and 4 columns, where each entry is set to `0`. This is handy for algorithms that require matrix manipulations.
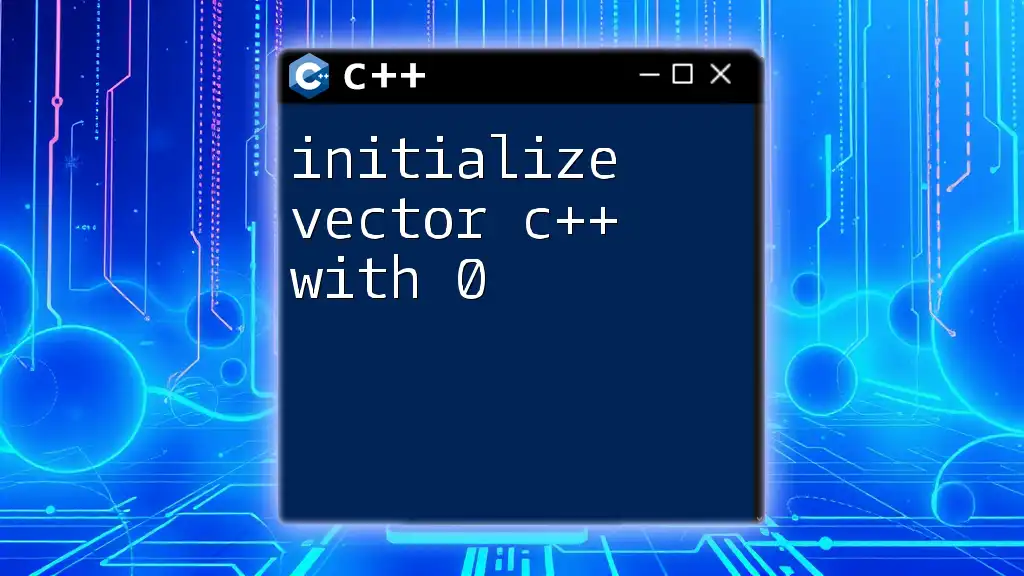
Conclusion
In this guide, we have explored various approaches to c++ initialize vector with size. We discussed default and value initialization, the significance of memory management, and the differences between size and capacity. Additionally, we looked at practical use cases, including how to manage user inputs and multi-dimensional data structures.
As you continue to experiment with vectors in C++, take advantage of their flexibility and built-in functionalities. The more you work with them, the more proficient you will become at utilizing this powerful data type.
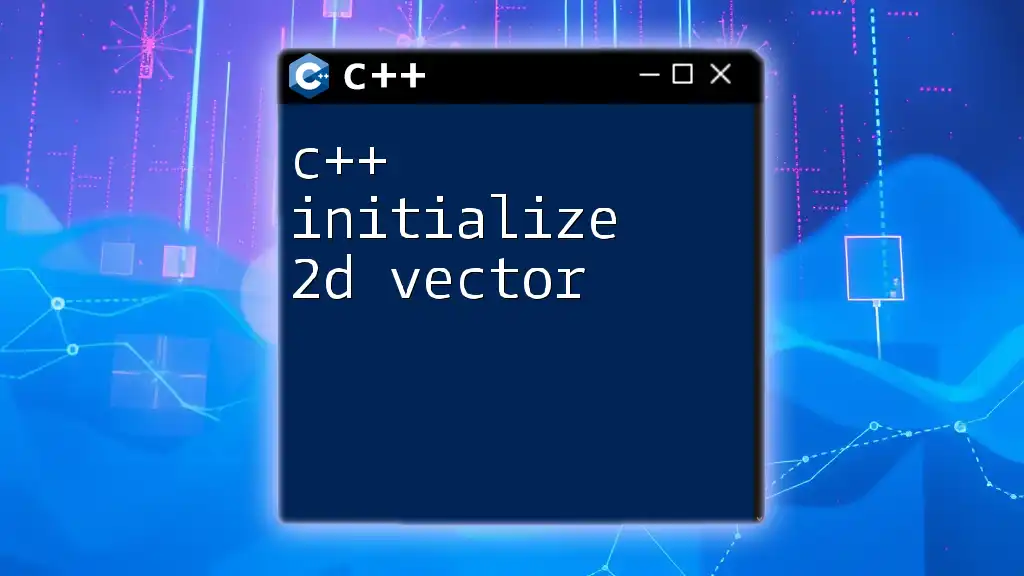
Additional Resources
For further learning, consider diving into these resources that expand on vector manipulations and C++ programming:
- Recommended books and online courses
- Links to C++ documentation and tutorials
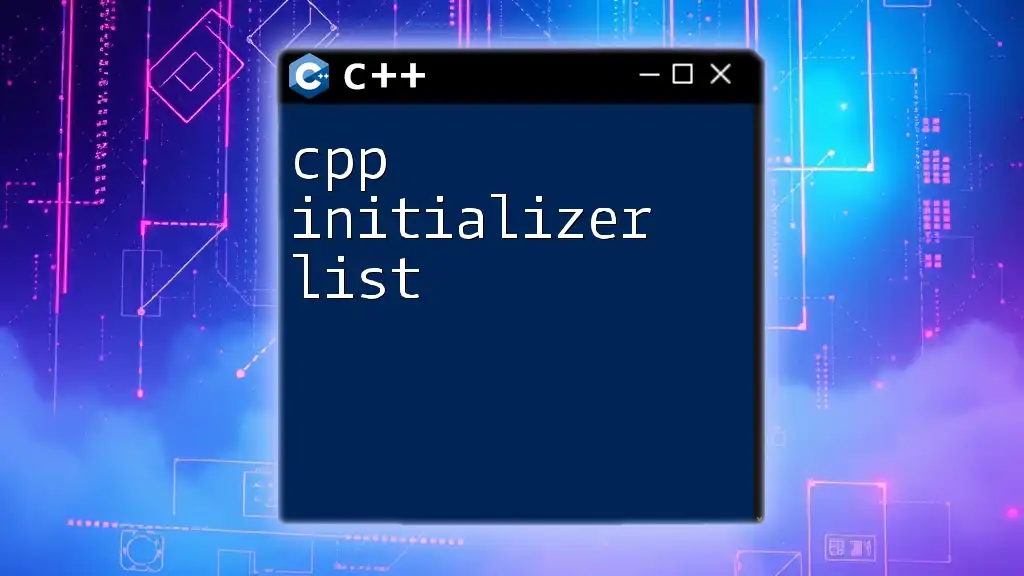
Frequently Asked Questions
What happens if I don't initialize a vector with size?
If you don’t specify a size when you declare a vector, it will be created as an empty vector. Elements can be added later using functions like `push_back`, but it's often beneficial to know the anticipated size for better performance.
Can I change the size of an existing vector after initialization?
Yes, vectors allow dynamic resizing. You can use the `resize()` method to adjust the size of a vector at runtime, making them an excellent choice for programs with fluctuating data needs.