A C++ initializer list is a concise way to initialize member variables or base classes using a colon (:) after the constructor definition.
#include <iostream>
using namespace std;
class Point {
public:
int x, y;
Point(int xCoord, int yCoord) : x(xCoord), y(yCoord) {}
};
int main() {
Point p(10, 20);
cout << "Point: (" << p.x << ", " << p.y << ")" << endl;
return 0;
}
What is an Initializer List in C++?
A C++ initializer list is a special syntax used to initialize member variables of a class or structure when an object is created. It serves as an efficient way to set the initial state of an object compared to other initialization methods, especially when dealing with constructors. The primary purpose of using an initializer list is to provide a clean and effective means of initializing member variables when the object is constructed.
Why Use Initializer Lists?
Utilizing initializer lists offers several advantages:
-
Performance Benefits: Initializer lists can lead to better performance since they directly initialize member variables rather than default-constructing them and then assigning values later. This is particularly noticeable for classes that manage resources (like dynamic memory).
-
Enhanced Readability: When using initializer lists, the intent of the initializations becomes clearer and more explicit. This aids in maintaining the code and ensuring that it adheres to good programming practices.
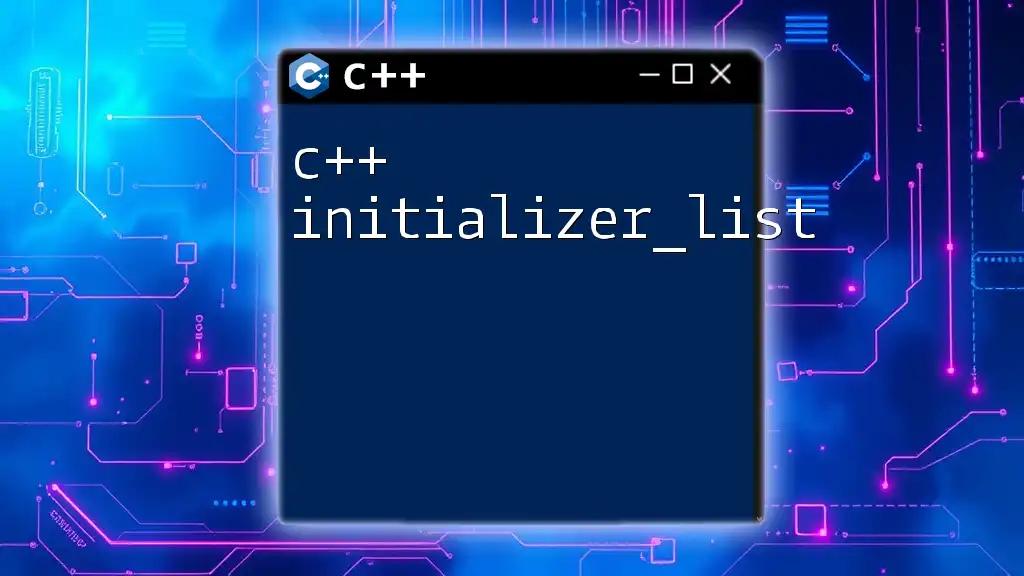
Understanding the Syntax of C++ Initializer Lists
The syntax of a C++ initializer list is relatively straightforward. It consists of a colon followed by a series of member initializations separated by commas. The general structure is as follows:
ClassName(parameter1, parameter2) : member1(value1), member2(value2) {
// constructor body
}
Examples of Initializer List Syntax
Here's a basic example to illustrate the syntax:
class MyClass {
int x;
int y;
public:
MyClass(int a, int b) : x(a), y(b) {}
};
In this snippet, when a `MyClass` object is created, the member variables `x` and `y` are initialized directly with the values of parameters `a` and `b`.

C++ Constructor Initialization List
What is a C++ Constructor Initialization List?
A C++ constructor initialization list is a feature that allows for precise control over how member variables are initialized. This is especially useful when dealing with const members or references that must be initialized at the time of construction.
Common Use Cases for Constructor Initialization List
The constructor initialization list is advisable when:
-
You Are Initializing Constant or Reference Members: Since const and reference members must be initialized upon object construction, initializer lists are required for them.
-
Performance Reasons: It is more efficient to initialize the members directly rather than default-constructing them and then assigning values.
Example of Constructor Initialization List
Here's an example illustrating this concept:
class Point {
int x, y;
public:
Point(int a, int b) : x(a), y(b) {} // Constructor initialization list
};
This example shows how `Point`'s members are set directly through the initializer list, making the creation of `Point` objects efficient.

Member Initialization List in C++
Understanding C++ Member Initialization Lists
A C++ member initialization list performs the same function as a constructor initialization list. The focus here is on clarity regarding the distinction between member initialization during object creation compared to assignment within the constructor's body.
Differences Between Member Initialization Lists and Regular Assignment
Using an initializer list to initialize members is often more efficient. Consider this comparison:
class Example {
int a;
public:
Example(int value) {
a = value; // Regular assignment
}
};
Versus:
class Example {
int a;
public:
Example(int value) : a(value) {} // Initialization list
};
The second option is preferable because it initializes `a` directly.
Code Example of a Member Initialization List
class Rectangle {
int width;
int height;
public:
Rectangle(int w, int h) : width(w), height(h) {}
};
In this example, both `width` and `height` are initialized in the same concise manner.

Using C++ Initializer Lists for Built-in Types
How to Initialize Built-in Types with Initializer Lists
Initializer lists can also simplify the initialization of built-in types. Assigning values directly through the initializer list streamlines the code and maintains performance.
Example of Using Initializer Lists for Built-in Types
class Numbers {
int a;
float b;
public:
Numbers(int x, float y) : a(x), b(y) {}
};
In the `Numbers` class, both `a` and `b` receive their initial values via the initializer list, promoting efficient and clear code.

C++ Initializer Lists with User-defined Types
Working with User-defined Types
One of the most powerful applications of an initializer list is in initializing user-defined types. This allows members that are objects of other classes to be initialized as part of the outer class's constructor.
Example of Initializing User-defined Types in a Constructor
class Point {
int x, y;
public:
Point(int xVal, int yVal) : x(xVal), y(yVal) {}
};
class Line {
Point start;
Point end;
public:
Line(int x1, int y1, int x2, int y2)
: start(x1, y1), end(x2, y2) {}
};
In this example, `Line` initializes two `Point` objects (`start` and `end`) in the constructor via an initializer list. This showcases the ability to concisely initialize complex member variables.

Common Mistakes to Avoid with Initializer Lists
Not Using Initializer Lists When Necessary
One of the most common mishaps is failing to utilize initializer lists, particularly when dealing with `const` members or references. This can lead to compilation errors since these members must be initialized upon construction.
Misunderstanding the Syntax
Syntax errors can occur frequently when using initializer lists, such as missing commas, colons, or using the wrong order. Always ensure that the syntax is adhered to correctly for proper functioning.
Example Illustrating Each Mistake
class Mistake {
const int x; // Must be initialized in the initializer list
public:
Mistake(int value) : x(value) {} // Correct usage
// Mistake(int value) { x = value; } // Incorrect - will not compile
};
In this case, the incorrectly implemented constructor will fail to compile while the initializer list correctly initializes the `const` member.

Conclusion
In summary, C++ initializer lists are an essential feature that enhances both the efficiency and clarity of code. Understanding how and when to use initializer lists can lead to better programming practices and help maintain high-performance code. By practicing with initializer lists, developers can significantly improve their object-oriented programming skills in C++.