The error "expected initializer before token" in C++ typically occurs when the compiler encounters a syntax issue, such as a missing type declaration or incorrect usage of variables or functions.
Here's a simple example illustrating this error:
#include <iostream>
int main() {
int number; // Declare variable
numbe = 5; // Error: expected initializer before token 'numbe'
std::cout << number;
return 0;
}
Understanding C++ Syntax
What is Syntax in Programming?
In programming, syntax refers to the set of rules that define the combinations of symbols that are considered to be correctly structured statements in a programming language. Syntax is fundamental because it not only dictates how code is written but also affects its functionality and behavior. If the syntax is incorrect, the compiler will throw errors, preventing the code from executing properly.
Common Syntax Errors in C++
C++ developers frequently encounter various syntax errors. Understanding these errors is crucial to writing efficient code. Among these errors, the "expected initializer before token c++" is a common issue that can halt the compilation process. Identifying and correcting these errors saves developers time and frustration.

What Does "Expected Initializer Before Token" Mean?
Definition of the Error
The error message "expected initializer before token" indicates that the compiler came across a token—such as a variable name, keyword, or symbol—that it did not expect at that point in the code. In simple terms, the compiler expected an initialization or declaration before a particular token, but it encountered something different.
Typical Scenarios Leading to This Error
Several scenarios may lead to this error, including:
- Missing semicolons at the end of statements.
- Misplaced brackets or braces that disrupt code blocks.
- Incorrect function declarations that don't follow syntax rules.
- Use of undeclared variables, making it impossible for the compiler to recognize them.

Analyzing the Error Message
Breakdown of the Error Message
Understanding the components of the error message can help developers pinpoint the issue:
- Expected Initializer: This segment indicates that the compiler was waiting for a value or definition.
- Before Token: The 'token' refers to the specific item in your code that caused the confusion.
Finding the Source of the Error
When you see this error, it's essential to:
- Check Line Numbers: The error message usually indicates a line number. Begin your search there.
- Recognize Patterns: Identify any common coding mistakes that may have led to this error, such as inconsistent coding style or improper use of syntax.

Common Causes and How to Fix Them
Missing Semicolons
One of the most frequent causes of this error is forgetting to add a semicolon at the end of a statement. For example, consider the following snippet:
int main() {
int x = 10
return 0;
}
The missing semicolon after `int x = 10` will trigger the "expected initializer before token" error. The corrected code should read:
int main() {
int x = 10;
return 0;
}
Misplaced Brackets or Braces
Another common error involves misplaced brackets or braces, which can disrupt the code's structure. For instance:
if (x > 5) {
cout << "X is greater than 5" // Missing brace
// Unclosed if statement
The absence of a closing brace leads to confusion for the compiler. The corrected version is:
if (x > 5) {
cout << "X is greater than 5";
}
Incorrect Function Declarations
When a function is declared incorrectly, this could lead to the error in question. For example, the following code is problematic:
void myFunction( // Missing parameter type
{
cout << "Hello, World!";
}
Without a proper function definition, the compiler will not recognize this function. The corrected code should be structured as follows:
void myFunction() {
cout << "Hello, World!";
}
Using Undeclared Variables
Attempting to use a variable that has not been declared will also raise this error:
cout << myVariable; // myVariable is not declared
In this case, `myVariable` needs to be declared before use. For example:
int myVariable = 10;
cout << myVariable;
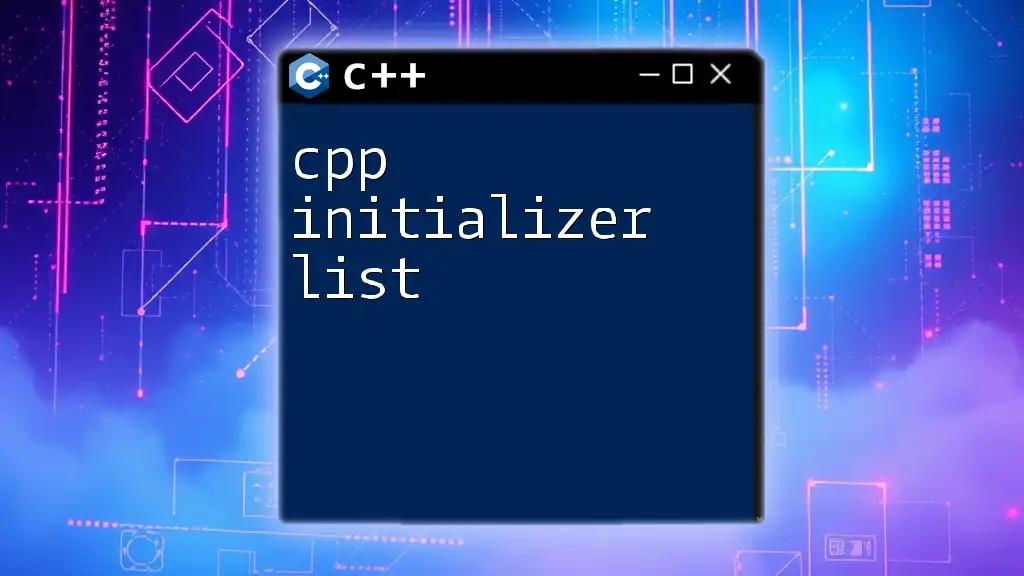
Best Practices to Avoid Syntax Errors
Code Structuring
Proper code structuring is essential for readable and maintainable code. Break down complex logic into smaller, manageable functions, and use consistent indentations. Such practices not only enhance performance but also minimize errors.
Regular Code Reviews
Engaging in peer reviews or pair programming can lead to innovative solutions and identify possible errors. Having another set of eyes assess your code can help in recognizing common pitfalls.
Using an Integrated Development Environment (IDE)
Utilizing IDEs can greatly facilitate coding. Modern IDEs come with integrated debugging tools, syntax highlighting, and error detection features. These resources make it easier to spot syntax errors before compilation, reducing the chances of encountering the "expected initializer before token c++" error.

Conclusion
The "expected initializer before token c++" error is a common yet manageable issue encountered in C++ programming. By grasping fundamental syntax rules and recognizing typical scenarios that lead to this error, you can dramatically improve your coding skills. Remember, syntax errors are a natural part of the development process. Continuous learning and practice will bolster your understanding of C++ and its nuances, enabling you to write cleaner and more efficient code.

Additional Resources
For those seeking to deepen their understanding of C++, consider exploring online courses, recommended books, and various platforms dedicated to programming education. Engaging with these resources can empower you further on your programming journey.
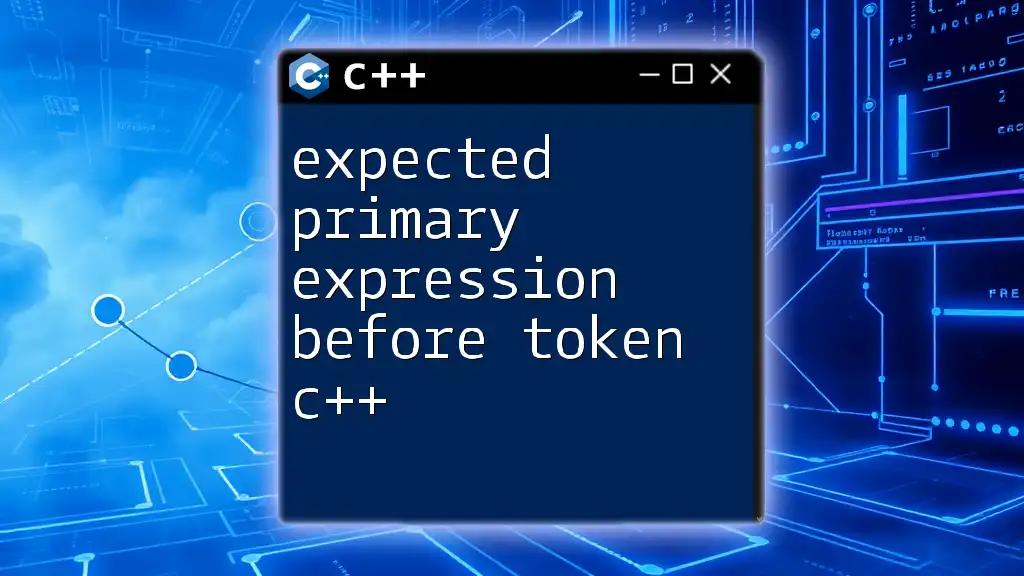
Call to Action
If you're excited to advance your C++ skills and learn more about syntax and error handling, be sure to follow our training sessions. Together, we can enhance your coding proficiency and make you a more confident programmer!