To initialize a vector in C++, you can use the following syntax to create a vector and optionally set its size and initial values.
#include <vector>
std::vector<int> myVector(5, 10); // Initializes a vector of size 5 with all elements set to 10
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can resize itself automatically when elements are added or removed. It is part of the C++ Standard Library and provides a convenient and efficient way to manage a collection of elements. Unlike traditional arrays, vectors:
- Manage their own storage: They can grow or shrink in size.
- Provide built-in functions: Vectors come equipped with several member functions that allow for easy manipulation of the elements, such as adding, removing, and accessing data.
Why Use Vectors?
Using vectors offers numerous advantages over static arrays:
- Dynamic sizing: Vectors can adjust their size during runtime, making them exceptionally flexible.
- Ease of use: With built-in functions, vectors streamline operations such as insertion and deletion.
- Memory management: Vectors allocate memory intelligently, reducing the likelihood of memory leaks that can occur with traditional arrays.
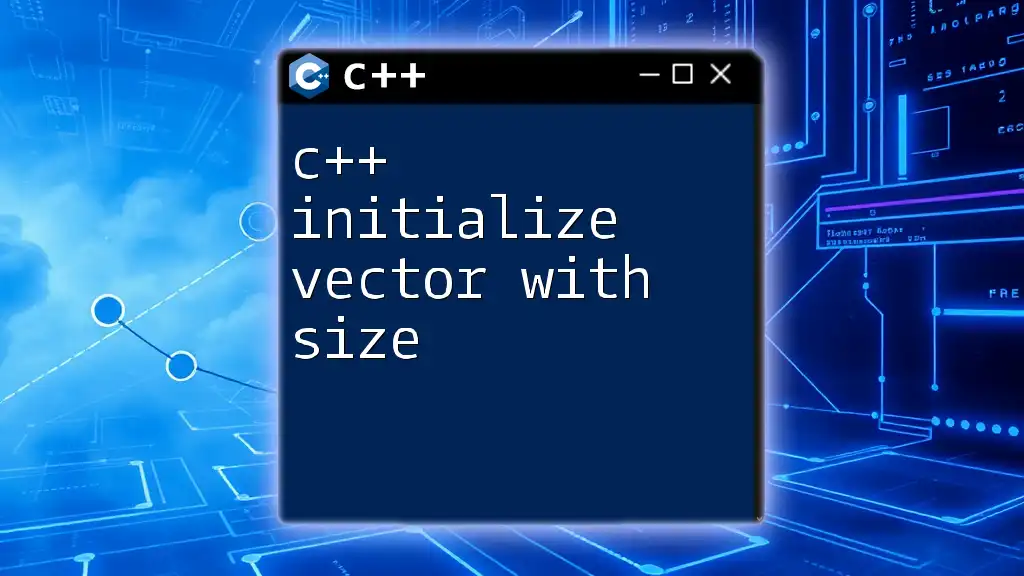
Declaring a Vector in C++
How to Declare a Vector
When declaring a vector, you must specify the type of elements it will hold. Here’s the syntax for declaring a vector:
std::vector<DataType> vectorName;
For example:
#include <vector>
std::vector<int> intVector; // Declaration of an empty integer vector
std::vector<std::string> stringVector; // Declaration of an empty string vector
Vector Declaration with Reserve
Declaring a vector with `reserve` demonstrates foresight by allocating memory for a specified number of elements upfront. This method enhances performance by minimizing the number of reallocations that occur as elements are added.
Example:
std::vector<int> intVector;
intVector.reserve(10); // Reserve space for 10 integers
In this case, space for ten integers is allocated, but the vector has no elements until values are explicitly added.
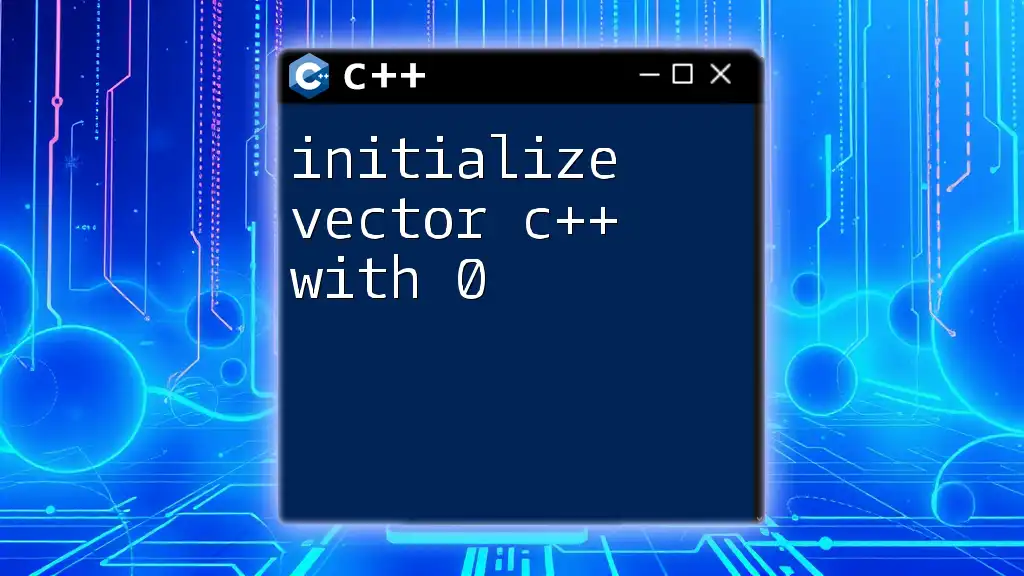
Initializing a Vector in C++
Default Initialization
You can initialize an empty vector simply by declaring it without specifying a size or initial values.
Example:
std::vector<int> intVector; // Empty vector initialized
This creates an empty vector ready for future use without preallocated memory.
Initializing a Vector with a Size
You might sometimes want to create a vector with a predetermined size, filling it with default values (typically 0 for numeric types).
Example:
std::vector<int> intVector(5); // Vector with 5 elements, each initialized to 0
In this instance, `intVector` is instantiated with five integer elements, all initialized to zero.
Initializing a Vector with Specific Values
One of the most powerful features of vectors is their ability to be initialized with specific values using an initializer list.
Example:
std::vector<int> intVector = {1, 2, 3, 4, 5}; // Vector initialized with values
This initialization succinctly sets the vector's elements to a predefined list.
Using Fill Constructor
The fill constructor allows you to create a vector with a specific size, all filled with the same value.
Example:
std::vector<int> intVector(5, 10); // Vector of 5 elements initialized to 10
Here, each of the five elements in `intVector` is initialized to the value `10`, demonstrating the convenience of the fill constructor for repeated values.
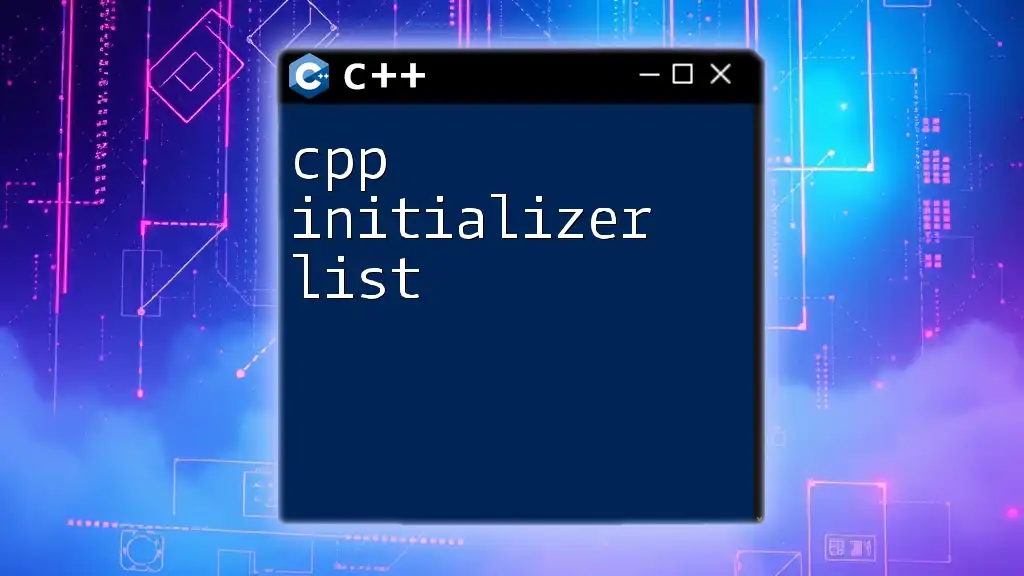
Advanced Vector Initialization Techniques
Using std::vector's Assign Method
The `assign()` method of vectors provides an alternative way to initialize or modify the contents of a vector. Using `assign()`, you can populate a vector with repeated values or a range from another container.
Example:
std::vector<int> intVector;
intVector.assign(5, 2); // Vector with 5 elements set to 2
This code snippet creates a vector populated with five `2`s, showcasing how `assign()` offers flexibility in vector initialization.
Initializing a Vector from Another Container
Vectors can also be constructed from other existing containers like arrays and other vectors, allowing for easy copying of data.
Example:
std::vector<int> source = {1, 2, 3};
std::vector<int> intVector(source.begin(), source.end()); // Copy initialization
In this case, `intVector` is initialized by copying the elements from the `source` vector using iterators, which is both efficient and straightforward.
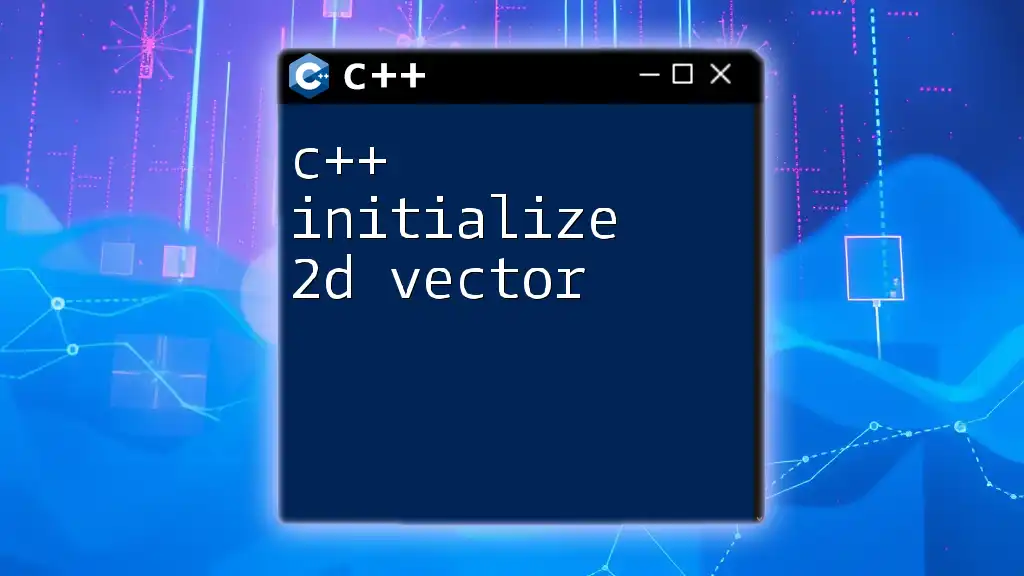
Common Issues and Troubleshooting
Common Mistakes
When working with vectors, a few common pitfalls to watch out for include:
- Forgetting to include the vector header: Always remember to include `<vector>` in your source files.
- Using uninitialized vectors: Attempting to access or modify vectors that haven't been properly initialized can lead to unexpected behavior.
Debugging Vector Errors
Understanding common vector-related errors can save time. Here are a few tips for debugging:
- Use assertions to verify the size of a vector before accessing elements.
- Check for out-of-range errors when using methods like `at()` or `operator[]`.
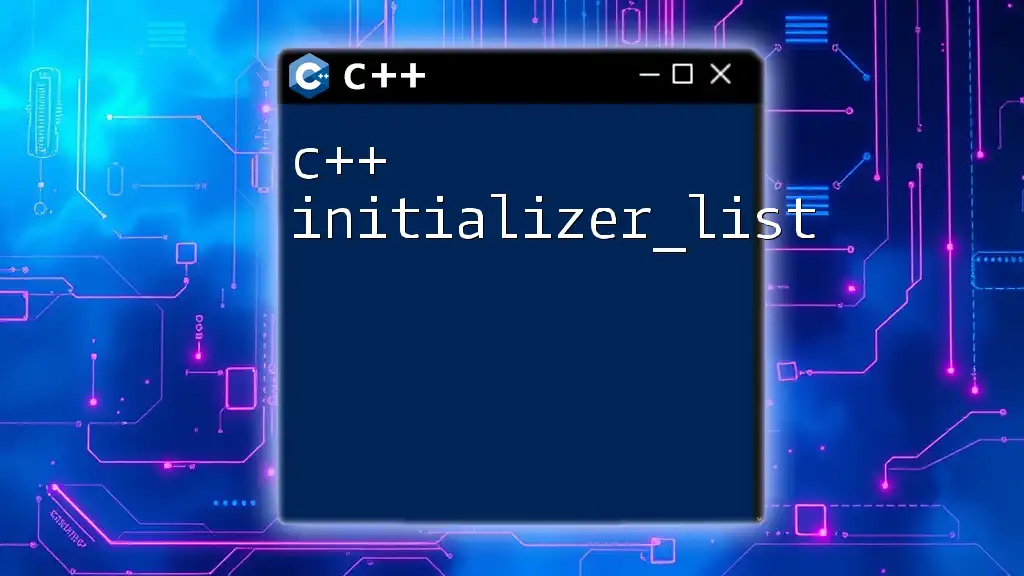
Conclusion
In this article, we explored how to cpp initialize vector effectively, covering different methods and scenarios. Understanding vector initialization is fundamental for leveraging the flexibility and power of C++ vectors in your programming.
Practice the various initialization techniques discussed, and you’ll find that vectors can greatly enhance your code's efficiency and readability. Don't hesitate to share your experiences or ask questions as you delve deeper into the world of C++ vectors.