A sorted vector in C++ is a dynamic array that maintains its elements in a particular order, allowing efficient insertion, deletion, and retrieval, and can be achieved using the `std::sort` function from the `<algorithm>` header.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {3, 1, 4, 1, 5};
std::sort(nums.begin(), nums.end());
for(int num : nums) {
std::cout << num << " ";
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can grow or shrink in size. Unlike traditional arrays, which have a fixed size, vectors can accommodate varying amounts of data, making them one of the most used data structures in C++. Their main characteristics include:
- Dynamic sizing: Automatically adjusts to accommodate elements.
- Contiguous storage: Elements are stored in memory contiguously, allowing easy access.
Vectors provide several advantages over arrays, such as ease of use, built-in functionalities, and automatic memory management, which make them a preferred choice for many programming tasks.
Basic Operations on Vectors
Vectors support a variety of operations, including:
- Creating a vector: You can initialize a vector with specific sizes and types.
- Adding and removing elements: Functions like `push_back()` and `pop_back()` enable dynamic modifications to the vector.
- Accessing elements: You can quickly access elements using the subscript operator (e.g., `vec[i]`).
Understanding these basic operations is crucial before diving into more advanced applications, such as managing a cpp sorted vector.
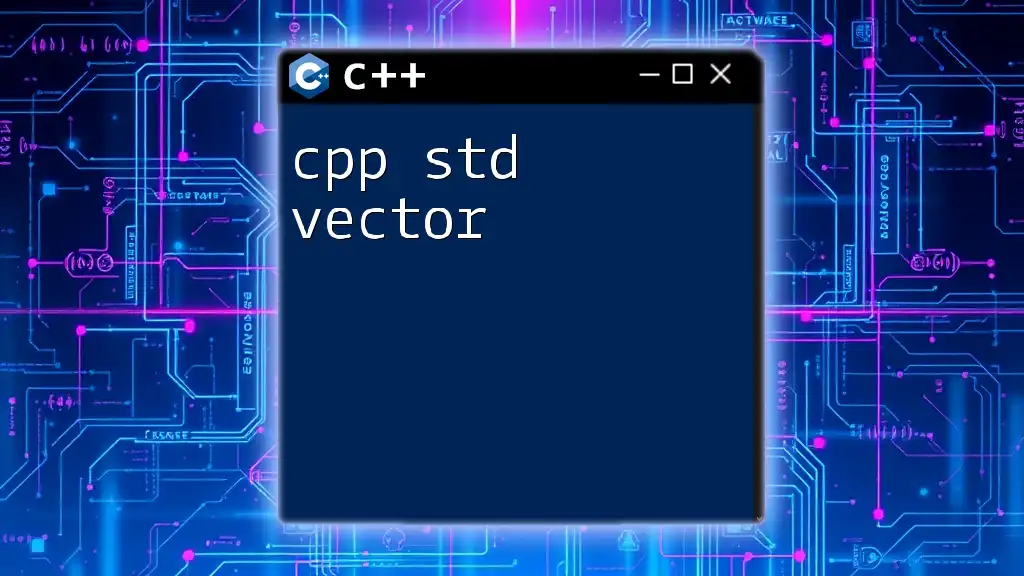
Introduction to Sorting in C++
Importance of Sorting
Sorting is a fundamental concept in computer science and programming. It facilitates efficient searching, organizing, and data retrieval. Common scenarios requiring sorting include:
- Organizing data for display or reporting.
- Preparing datasets for binary search algorithms, which require sorted data for optimal performance.
- Enhancing the overall readability and usability of data by presenting it in a logical order.
The Standard Library in C++
C++ provides a powerful Standard Library that includes the `<algorithm>` header, which contains several built-in functions, including sorting algorithms. The `std::sort` function is one of the most commonly used tools for sorting vectors efficiently and effectively.
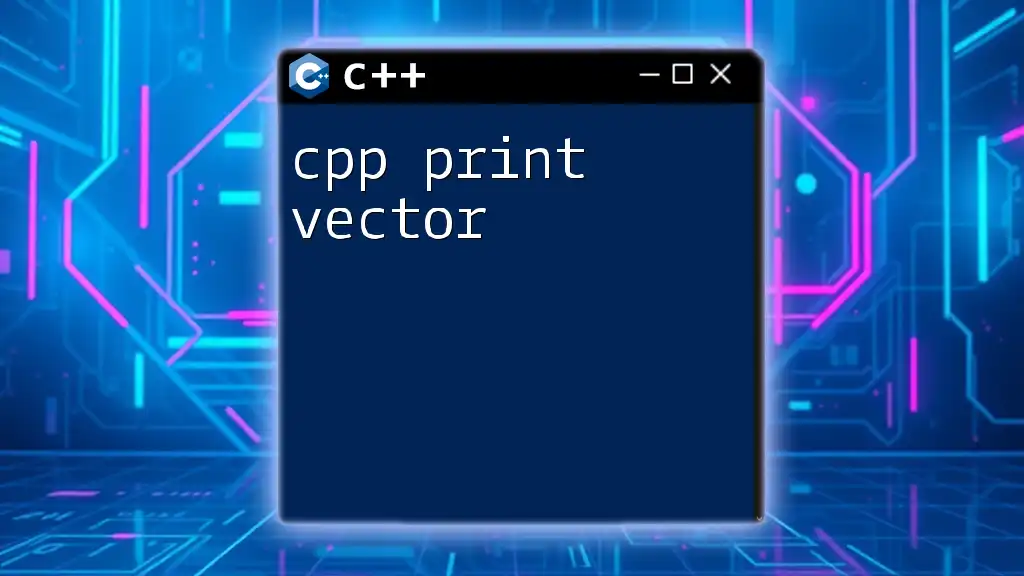
C++ Sorted Vector: What You Need to Know
Defining a Sorted Vector
A sorted vector is simply a vector whose elements are in a specific order—typically ascending or descending. Maintaining sorted order can improve data retrieval and management, especially when working with data-driven applications or algorithms.
Creating a Sorted Vector in C++
To create a sorted vector in C++, you can utilize the `std::sort` function. Here's how to initialize a sorted vector:
- First, declare a vector and populate it with unsorted values.
- Then, call the `std::sort` function to arrange the elements in the desired order.
Example: Basic Sorted Vector Creation
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {4, 1, 3, 9, 2};
// Sorting the vector
std::sort(nums.begin(), nums.end());
// Displaying the sorted vector
for (int num : nums) {
std::cout << num << " "; // Output: 1 2 3 4 9
}
return 0;
}
In this example, the vector `nums` is sorted from lowest to highest using `std::sort`, demonstrating the basic process of creating a cpp sorted vector.
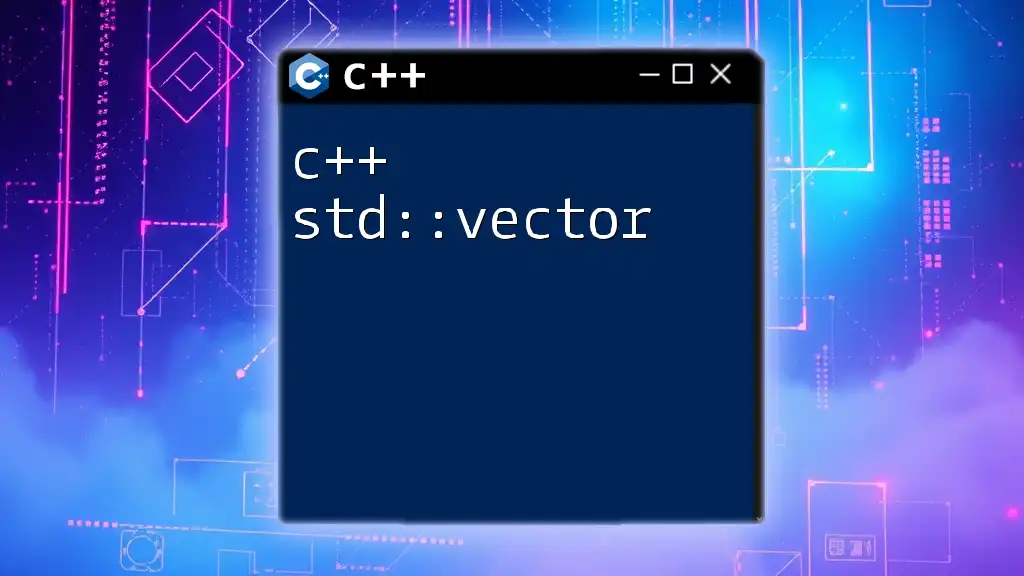
Using `std::sort` to Sort Vectors
How to Sort a Vector in C++
The `std::sort` function has a straightforward syntax:
std::sort(iterator_begin, iterator_end);
- iterator_begin: Starting point of the vector you want to sort.
- iterator_end: Ending point of the vector.
Example: Sorting a Vector of Integers
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {4, 1, 3, 9, 2};
// Sorting the vector
std::sort(nums.begin(), nums.end());
// Displaying the sorted vector
for (int num : nums) {
std::cout << num << " "; // Output: 1 2 3 4 9
}
return 0;
}
This code uses `std::sort` to sort a vector of integers. Note how the entire vector is sorted in a single function call, a testament to the power and simplicity of C++'s Standard Library.
Sorting Custom Objects
You can extend the functionality of `std::sort` to sort vectors containing custom objects. For this, you must define a comparison function that determines how two objects should be compared.
Example: Sorting a Vector of Objects Based on a Member Variable
#include <iostream>
#include <vector>
#include <algorithm>
class Person {
public:
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
bool compareAge(const Person &a, const Person &b) {
return a.age < b.age; // Sort by age in ascending order
}
int main() {
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}, {"Charlie", 35}};
// Sorting using a custom comparison function
std::sort(people.begin(), people.end(), compareAge);
// Displaying the sorted vector of people
for (const auto &person : people) {
std::cout << person.name << " " << person.age << std::endl;
// Output: Bob 25, Alice 30, Charlie 35
}
return 0;
}
In this example, the `compareAge` function determines the sort order based on each person's age. By utilizing custom comparison, you're able to sort complex data types efficiently.
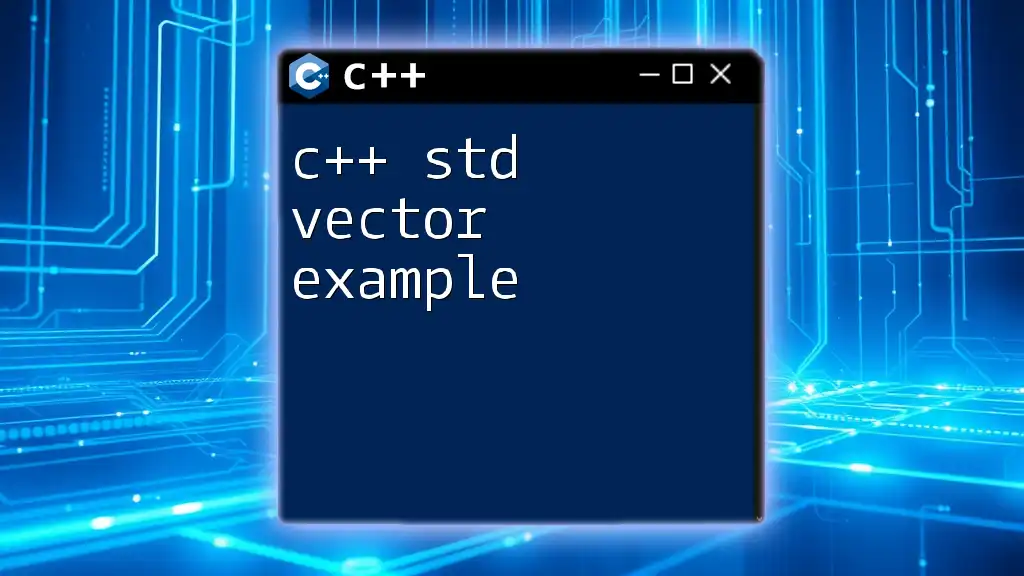
Maintaining a Sorted Vector
Inserting Elements into a Sorted Vector
Maintaining a sorted vector requires care when inserting new elements to ensure that the order is preserved. Instead of merely appending new values to the end, you can use `std::lower_bound` to find the correct position for the new element.
Example of Inserting While Maintaining Order
#include <iostream>
#include <vector>
#include <algorithm>
void insertInOrder(std::vector<int> &vec, int value) {
auto it = std::lower_bound(vec.begin(), vec.end(), value);
vec.insert(it, value); // Inserts value at the correct position
}
int main() {
std::vector<int> sortedVec = {1, 3, 5, 7};
// Inserting a new element while maintaining order
insertInOrder(sortedVec, 4);
// Displaying the updated sorted vector
for (int num : sortedVec) {
std::cout << num << " "; // Output: 1 3 4 5 7
}
return 0;
}
In this code snippet, the `insertInOrder` function dynamically inserts an integer into its correct position in the sorted vector. This process keeps the vector sorted after each insertion.
Removing Elements from a Sorted Vector
When you need to remove elements from a sorted vector, it’s essential to maintain its order. You can accomplish this using `std::remove_if` in conjunction with `std::erase`.
Example of Removing an Element
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> sortedVec = {1, 2, 3, 4, 5};
// Removing the number 3 from the sorted vector
sortedVec.erase(std::remove(sortedVec.begin(), sortedVec.end(), 3), sortedVec.end());
// Displaying the updated sorted vector
for (int num : sortedVec) {
std::cout << num << " "; // Output: 1 2 4 5
}
return 0;
}
This example effectively demonstrates how to remove elements while ensuring the integrity of a cpp sorted vector. The `std::remove` function shifts the elements and the `erase` function removes the unwanted entries.
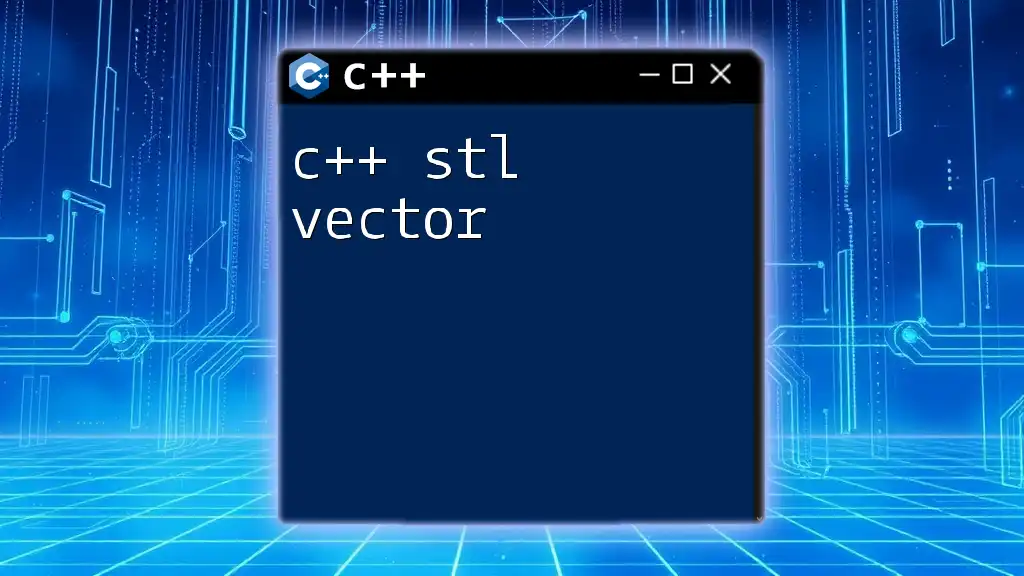
Conclusion
Recap of C++ Sorted Vectors
To sum up, understanding and working with cpp sorted vectors can significantly enhance your programming efficiency. From creating a sorted vector using `std::sort`, to maintaining order during insertions and deletions, mastering these techniques will improve your handling of data—making your programs not only faster but also more organized.
Next Steps
To further enhance your skills in C++, consider exploring advanced data structures, performance optimization techniques, and more intricate sorting algorithms. Engaging with practice exercises will solidify your knowledge and prepare you for real-world programming challenges.
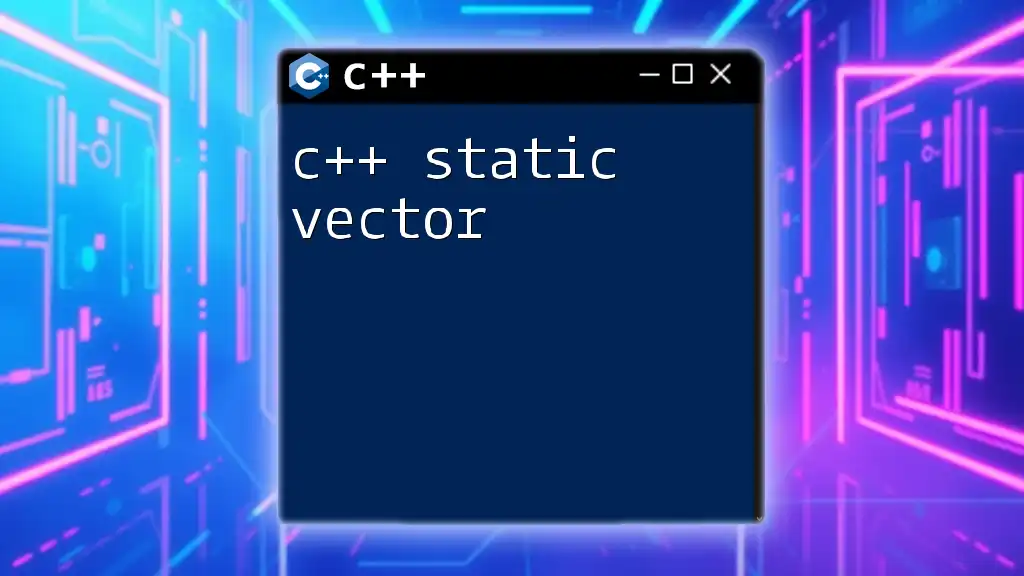
Additional Resources
Online Tools and References
For hands-on practice, consider utilizing online C++ coding platforms such as repl.it or cppreference.com to deepen your understanding of sorting vectors and other structures.
Community and Support
Joining programming forums and communities like Stack Overflow and Reddit can also be beneficial. These platforms are excellent for seeking help, sharing ideas, and collaborating with fellow C++ enthusiasts on various projects.