In C++, you can print a vector's elements using a simple loop or the `std::copy` function along with an output stream, as shown below:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
// Method 1: Using a loop
for (const auto& val : vec) {
std::cout << val << " ";
}
std::cout << std::endl; // New line for better output formatting
// Method 2: Using std::copy
std::copy(vec.begin(), vec.end(), std::ostream_iterator<int>(std::cout, " "));
return 0;
}
Understanding C++ Vectors
What is a Vector in C++?
In C++, a vector is a part of the Standard Template Library (STL) that allows you to create a dynamic array capable of resizing itself when elements are added or removed. Unlike regular arrays, vectors can grow and shrink in size, providing flexibility that is essential in modern programming.
Vectors are particularly useful for handling collections of data where the exact number of elements might not be known ahead of time, making them a foundational component in C++ programming.
How to Declare and Initialize a Vector in C++
Declaring a vector in C++ is straightforward. You can create empty vectors or initialize them with values. Here is the syntax for both methods:
#include <vector>
std::vector<int> numbers; // Empty vector
std::vector<int> initializedVector = {1, 2, 3, 4, 5}; // Initialized vector
The above code illustrates how to declare a vector of integers. You can also replace `int` with other data types, such as `double`, `char`, or even user-defined types.
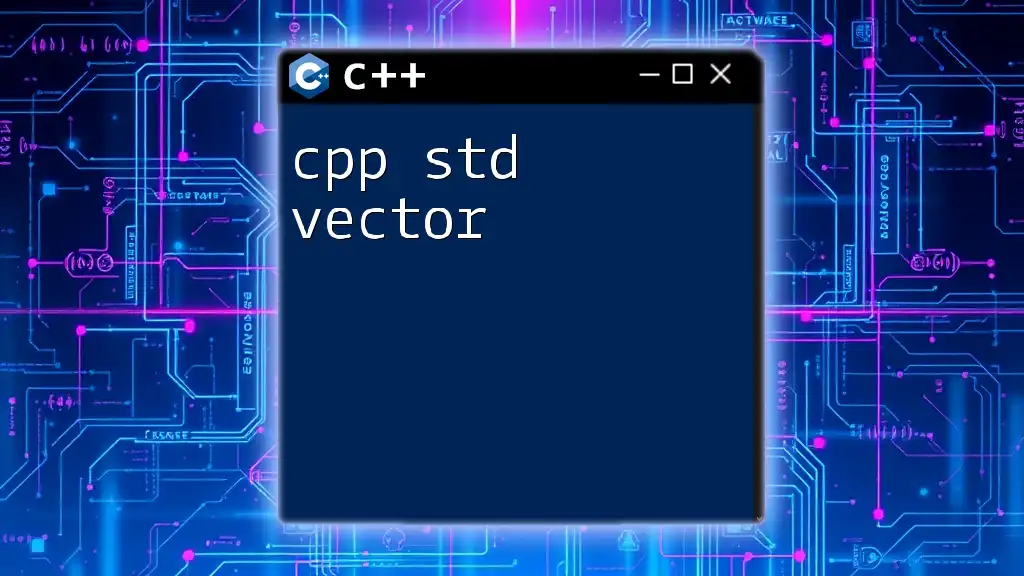
How to Print a Vector in C++
Basics of Printing in C++
To print the contents of a vector, you utilize the `cout` object along with the insertion operator `<<`. This operator allows you to stream output to the console. A simple output statement looks like this:
std::cout << "Hello, World!" << std::endl;
This fundamental understanding of `cout` sets the groundwork for printing vectors.
How to Print the Elements of a Vector in C++
Using a Simple Loop
One of the most common ways to print the contents of a vector is to use a conventional `for` loop. Here's how it can be done:
for (int i = 0; i < myVector.size(); ++i) {
std::cout << myVector[i] << " ";
}
std::cout << std::endl; // New line for better readability
In this example, `myVector.size()` returns the number of elements in the vector, and the loop iterates through each index to output its value.
Using a Range-based For Loop
With C++11, you can simplify the process of printing vectors using the range-based for loop. This approach is not only cleaner but also less prone to errors. Here’s an example:
for (const auto& element : myVector) {
std::cout << element << " ";
}
std::cout << std::endl; // New line after printing
This method iterates through each element directly, eliminating the need for an index variable.
How to Print Vector in C++ with Index
Printing the elements along with their indices can be helpful for debugging or for displaying a more informative output. Here's how you can do that:
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << "Element at index " << i << ": " << myVector[i] << std::endl;
}
This loop provides context to each printed element, making it easier for readers to understand where each value is positioned within the vector.
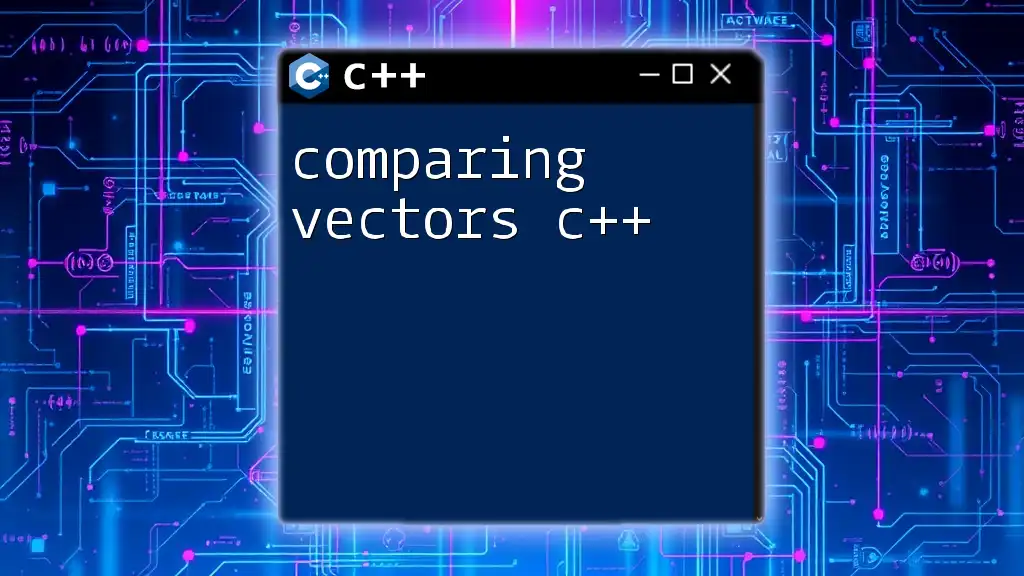
Formatting Output When Printing a Vector C++
Printing Separators
When printing a list of elements, you might want to format the output more clearly by adding separators, such as commas or spaces. Here’s an example of how to achieve this:
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myVector[i];
if (i < myVector.size() - 1) {
std::cout << ", "; // Print a comma except for the last element
}
}
This code ensures that the output is tidy and readable by adding a comma after each element except the last one.
Using Functions to Print Vectors
To promote code reuse and clarity, you can define a function specifically for printing vectors. This is beneficial when dealing with multiple vectors in the same program:
void printVector(const std::vector<int>& vec) {
for (const auto& element : vec) {
std::cout << element << " ";
}
std::cout << std::endl;
}
This function takes a vector as an argument and prints its elements, allowing you to call `printVector(myVector);` wherever needed.
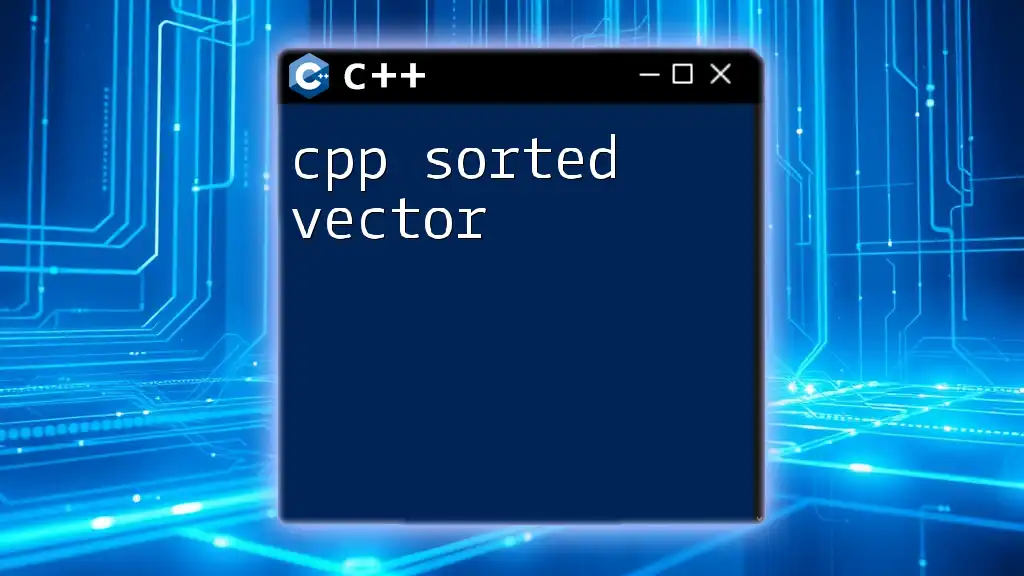
Advanced Printing Techniques for Vectors in C++
Printing Vectors of Different Data Types
Vectors are not limited to integers; they can also hold various data types. For instance, if you want to create a vector of strings, the approach remains largely the same:
std::vector<std::string> stringVector = {"Hello", "World"};
for (const auto& str : stringVector) {
std::cout << str << " ";
}
This capability allows you to handle collections of mixed data types or to create vectors of custom objects, enhancing your programming versatility.
Using Algorithms for Printing
C++ offers a comprehensive set of algorithms within the STL that can simplify tasks like printing. The `for_each` algorithm can be effectively used for this purpose:
#include <algorithm>
#include <iostream>
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::for_each(myVector.begin(), myVector.end(), [](int element) {
std::cout << element << " ";
});
std::cout << std::endl; // Final newline for output clarity
This approach encapsulates the printing logic within a lambda function, demonstrating the power and flexibility of C++'s features.
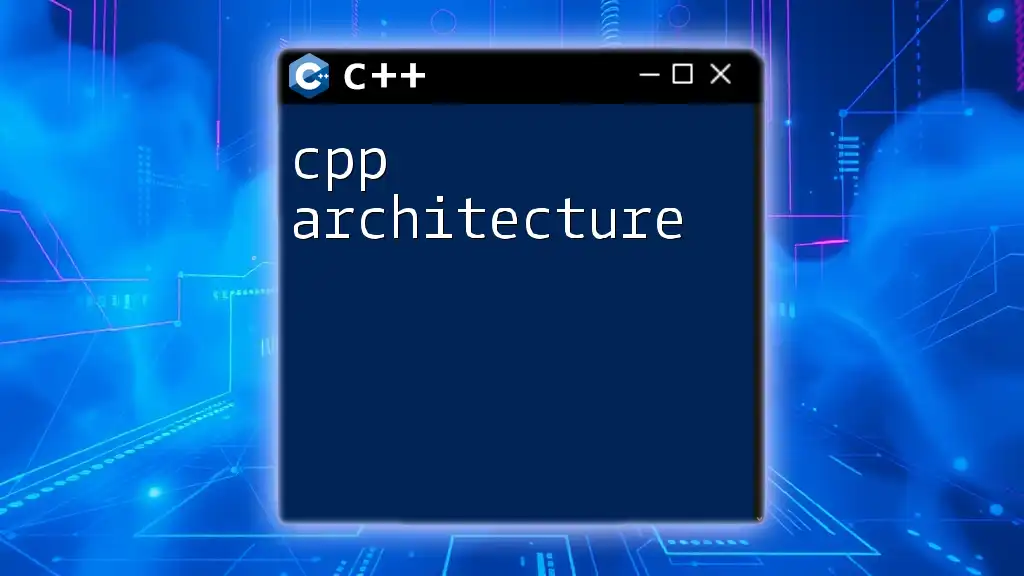
Troubleshooting Common Issues
Vector Is Empty
One common issue you may encounter is trying to print an empty vector. It’s essential to check if the vector has elements before attempting to print it:
if (myVector.empty()) {
std::cout << "The vector is empty." << std::endl;
} else {
printVector(myVector);
}
This safeguard prevents misleading outputs and ensures that your program handles empty vectors gracefully.
Out-of-Bounds Access
Another potential pitfall is accessing elements outside the vector's range. If you attempt to access an element using an invalid index, it can lead to undefined behavior. Always ensure your indices are valid:
if (index >= 0 && index < myVector.size()) {
std::cout << "Element: " << myVector[index] << std::endl;
} else {
std::cout << "Index out of bounds." << std::endl;
}
Employing such checks can save you from frustrating programming errors.
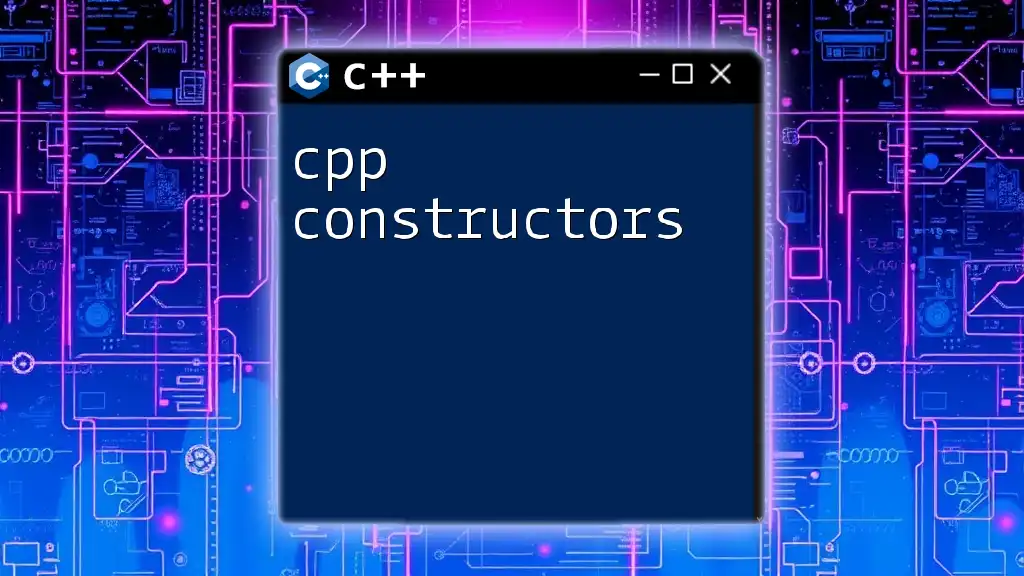
Conclusion
Printing vectors in C++ is a fundamental skill that enhances your ability to debug and visualize data effectively. Through various methods—such as loops, functions, and algorithms—you can choose the approach that best suits your needs. Whether you print elements with indices, format your output neatly, or handle different data types, mastery of these techniques will significantly impact your programming efficiency.
Continue to practice these methods and explore additional resources to further enhance your understanding of C++ vectors and their manipulation. Happy coding!