C++ architecture refers to the overall structure and organization of a C++ program, including elements like classes, objects, and functions that work together to define how the code executes.
Here's a simple example of defining a class in C++:
#include <iostream>
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
What is CPP Architecture?
CPP architecture encompasses the fundamental concepts, methodologies, and guidelines that govern how C++ programs are structured and managed. Understanding CPP architecture is crucial for developing efficient, maintainable, and scalable C++ applications. It brings clarity to the complex interactions within code, leading to improved software quality.
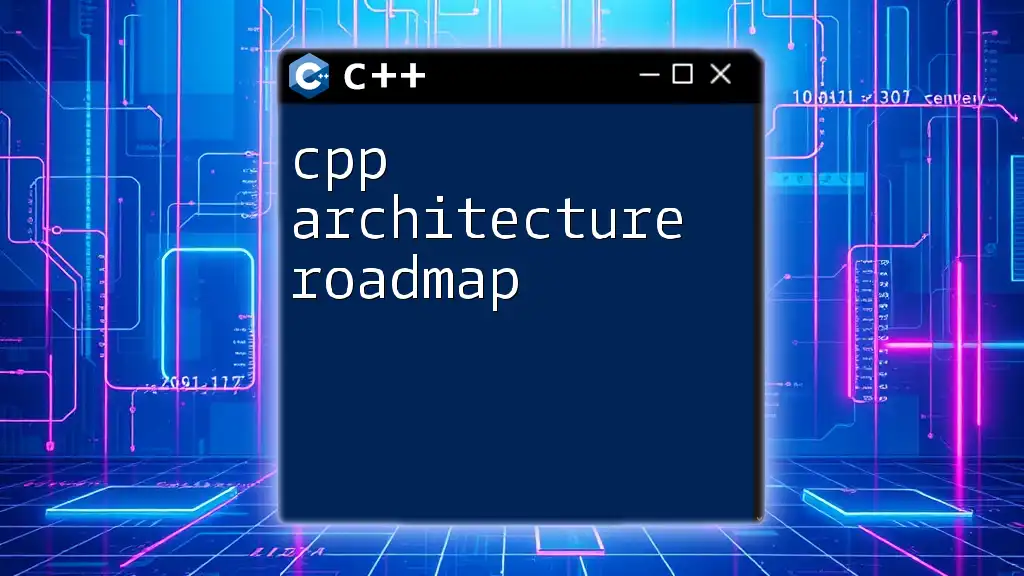
Why Learn CPP Architecture?
Learning CPP architecture equips developers with the tools necessary to enhance performance and manage resources effectively. With a solid grasp of these concepts:
- You can write code that runs faster and makes better use of memory.
- You improve the readability and maintainability of your projects, enabling easier team collaboration and future enhancements.
- Understanding architectural patterns allows you to find and fix issues more quickly, ultimately leading to more reliable software.
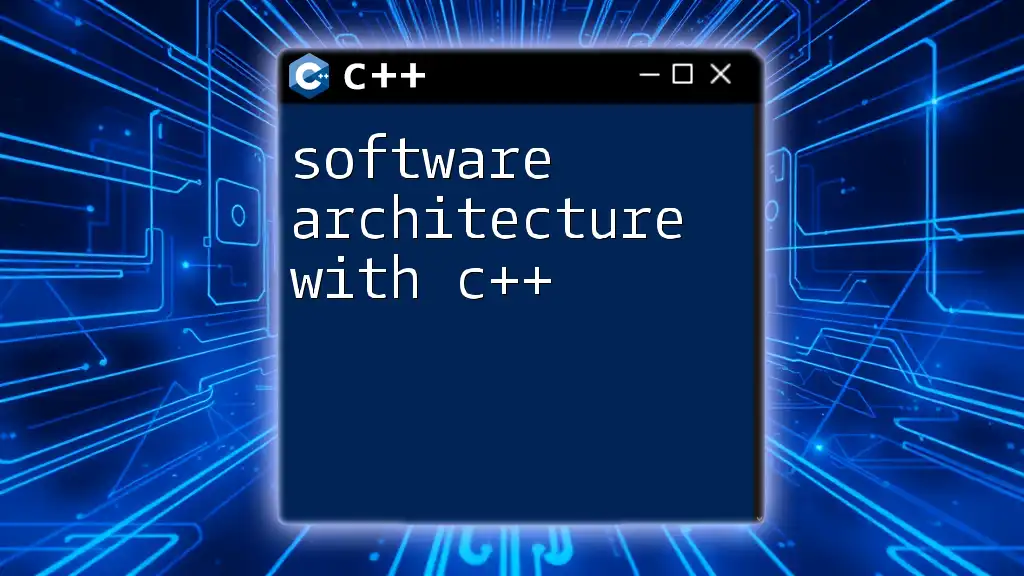
Core Concepts of CPP Architecture
Understanding Object-Oriented Programming (OOP) in C++
Object-Oriented Programming (OOP) is a programming paradigm central to C++ architecture. It emphasizes the use of "objects," which can represent real-world entities and encapsulate data and behavior.
Key OOP Principles
-
Encapsulation: Bundling data (attributes) and methods (functions) that operate on the data into a single unit called a class. This restricts direct access to some of the object’s components.
- Example:
class Car { private: string engineType; public: void setEngineType(string type) { engineType = type; } string getEngineType() { return engineType; } };
-
Inheritance: Allowing a new class (derived class) to inherit properties and methods from an existing class (base class). This promotes code reuse.
- Example:
class Vehicle { public: void start() { /* start vehicle */ } }; class Car : public Vehicle { public: void honk() { /* honk car */ } };
-
Polymorphism: The ability to treat different classes through the same interface, enabling a single function to work in different ways.
- Example:
class Animal { public: virtual void sound() { /* generic sound */ } }; class Dog : public Animal { public: void sound() override { /* bark */ } };
Memory Management in C++
C++ allows fine-grained control over memory usage, making it vital to understand static versus dynamic memory allocation.
Static vs. Dynamic Memory Allocation
-
Static Allocation: Memory is allocated at compile time. The lifetime is tied to the scope in which the variable is defined. Examples include global variables and variables declared within functions.
-
Dynamic Allocation: Memory is allocated at runtime using `new`, allowing for flexible memory management but requiring diligent cleanup to avoid memory leaks.
- Example:
int* ptr = new int; // Dynamic allocation delete ptr; // Free memory
Smart Pointers
C++ provides smart pointers to manage memory automatically and reduce the risk of memory leaks.
-
std::unique_ptr: Manages exclusive ownership of an object; when the pointer goes out of scope, it automatically deletes the associated object.
-
std::shared_ptr: Allows multiple pointers to share ownership of an object. The object is deleted when the last `shared_ptr` goes out of scope.
-
Example of using `std::unique_ptr`:
#include <memory>
class Car {
// Car implementation
};
std::unique_ptr<Car> carPtr(new Car());
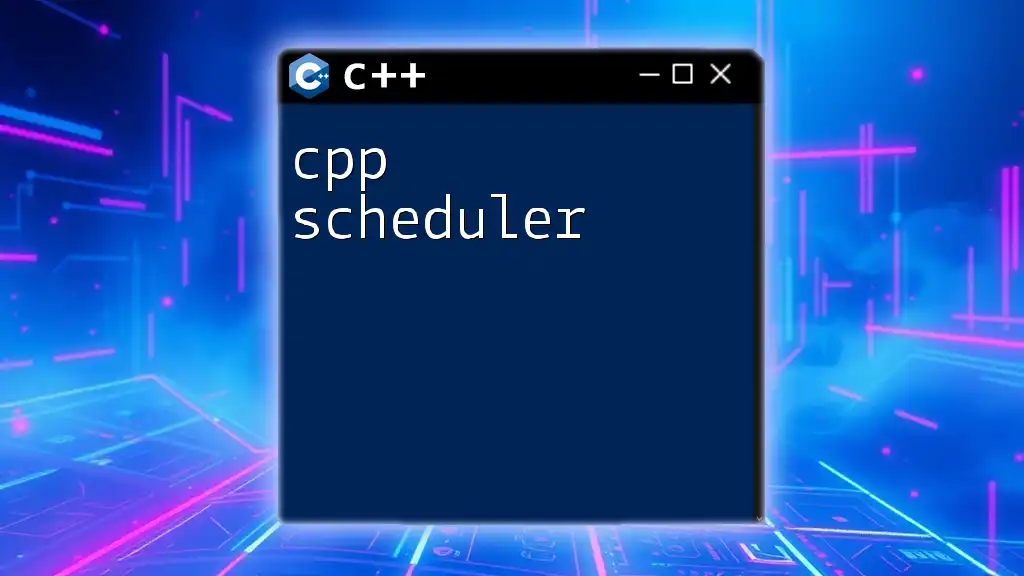
Design Patterns in CPP
Design patterns provide generalized solutions to common design problems. They enhance code organization and promote best practices.
Common Design Patterns
Creational Patterns
Creational patterns manage object creation. For instance:
- Singleton Pattern: Ensures that a class has only one instance and provides a global point of access.
- Example:
class Singleton { public: static Singleton& getInstance() { static Singleton instance; return instance; } // Other methods... private: Singleton() {} };
Structural Patterns
These patterns focus on object composition, allowing classes and objects to work together. Examples include:
- Adapter Pattern: Allows incompatible interfaces to work together.
- Composite Pattern: Lets you compose objects into tree structures to represent part-whole hierarchies.
Behavioral Patterns
Behavioral patterns focus on how objects interact. Examples include:
- Observer Pattern: Enables one object (the subject) to notify others (observers) automatically when its state changes.
- Strategy Pattern: Lets you define a family of algorithms, encapsulating them to make them interchangeable.
Example of a Design Pattern
Implementation of the Observer Pattern
- Define a subject class and observer interface. The subject maintains a list of observers, notifying them when a state change occurs.
class Subject {
private:
std::vector<Observer*> observers;
public:
void attach(Observer* observer) {
observers.push_back(observer);
}
void notify() {
for (auto observer : observers) {
observer->update();
}
}
};
// Observer interface
class Observer {
public:
virtual void update() = 0;
};
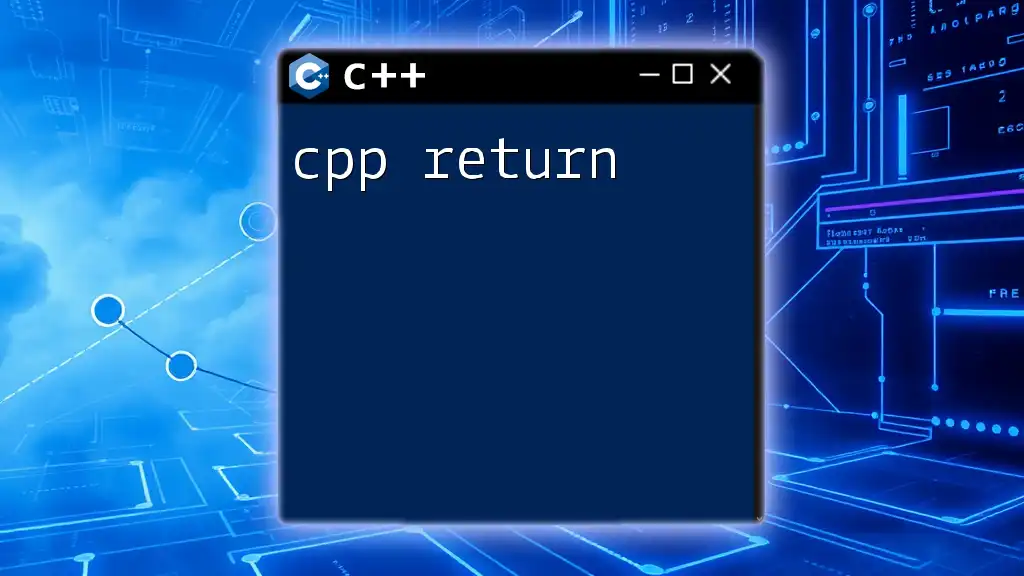
The Role of Templates in C++
Templates allow for the creation of generic functions and classes, enabling code reuse and type safety.
What are Templates?
Templates enable writing code without needing to specify all types explicitly.
-
Function Templates: Allow functions to operate with any data type.
- Example:
template <typename T> T add(T a, T b) { return a + b; }
-
Class Templates: Allow defining a class with generic types.
- Example:
template <typename T> class Box { T item; public: void setItem(T newItem) { item = newItem; } T getItem() { return item; } };
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful set of C++ template classes to create data structures and algorithms.
Common Containers in STL
- Vectors: Resizable arrays that allow fast random access.
- Lists: Doubly-linked lists; efficient for insertions/deletions.
- Maps: Associative arrays that store key-value pairs, allowing efficient lookups.
Example of using a `vector`:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4};
numbers.push_back(5); // Adding an element
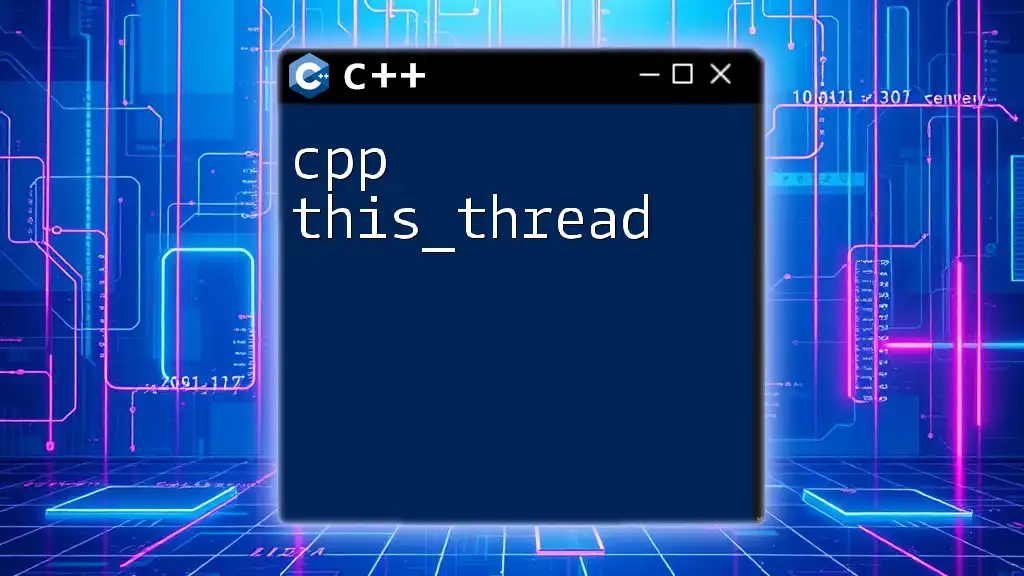
Concurrency in C++
Concurrency allows multiple threads to execute simultaneously, significantly enhancing performance for multi-core processors.
Multithreading Basics
Multithreading improves the efficiency of CPU usage, allowing processes to run concurrently.
Creating Threads in CPP
C++ offers the `<thread>` library for creating and managing threads.
- Example of thread creation:
#include <thread>
#include <iostream>
void threadFunction() {
std::cout << "Thread is running." << std::endl;
}
std::thread t(threadFunction);
Synchronization Primitives
With multiple threads running, we face the risk of data races. Synchronization primitives help mitigate this risk.
- Mutexes: Used to ensure that only one thread can access a resource at a time.
- Locks: Provide a safer, easier way to manage mutexes.
Example of using a mutex:
#include <mutex>
std::mutex mtx;
void safePrint() {
mtx.lock();
std::cout << "Thread-safe operation." << std::endl;
mtx.unlock();
}
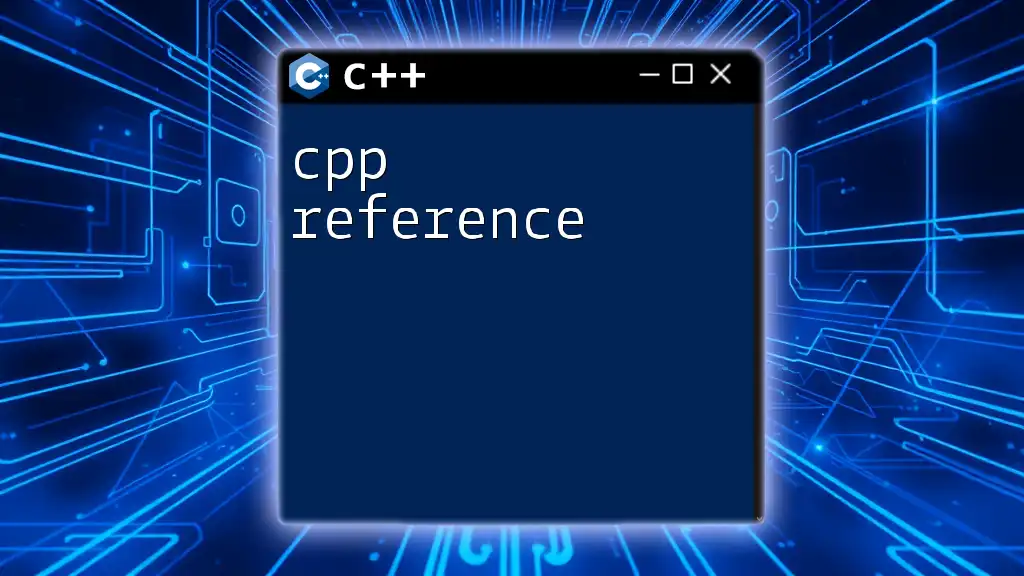
Advanced Topics in CPP Architecture
Understanding advanced aspects of CPP architecture helps in creating robust applications at scale.
Compiler and Build Systems
The C++ compiler translates your code into machine code, allowing it to run on hardware. Understanding how the compilation works can lead to better optimization of your code.
Build Systems
Building large C++ projects can be complex. Build systems like CMake manage project dependencies and facilitate the compilation process. CMake works across different platforms, automatically generating appropriate build configurations.
Performance Optimization Techniques
Optimization involves improving the performance of C++ code through various strategies.
-
Code Optimization Strategies: Prioritize using more efficient algorithms and data structures, reducing the complexity of your code.
-
Profiling and Debugging: Use profiling tools like Valgrind or gprof to analyze where bottlenecks are occurring in your code. Basic debugging techniques include using print statements or more advanced debugging tools to step through code execution.
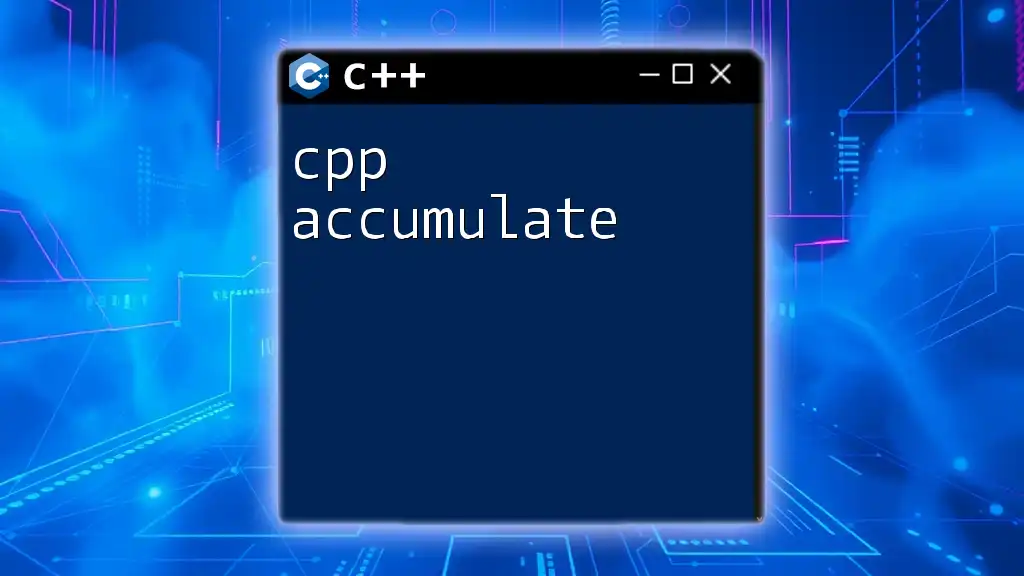
Conclusion
Understanding CPP architecture is essential for anyone looking to excel in C++ programming. By mastering the principles of OOP, memory management, design patterns, templates, concurrency, and advanced topics, you can build robust software solutions. This knowledge not only enhances your ability to write efficient and maintainable code but also prepares you for tackling complex programming challenges.
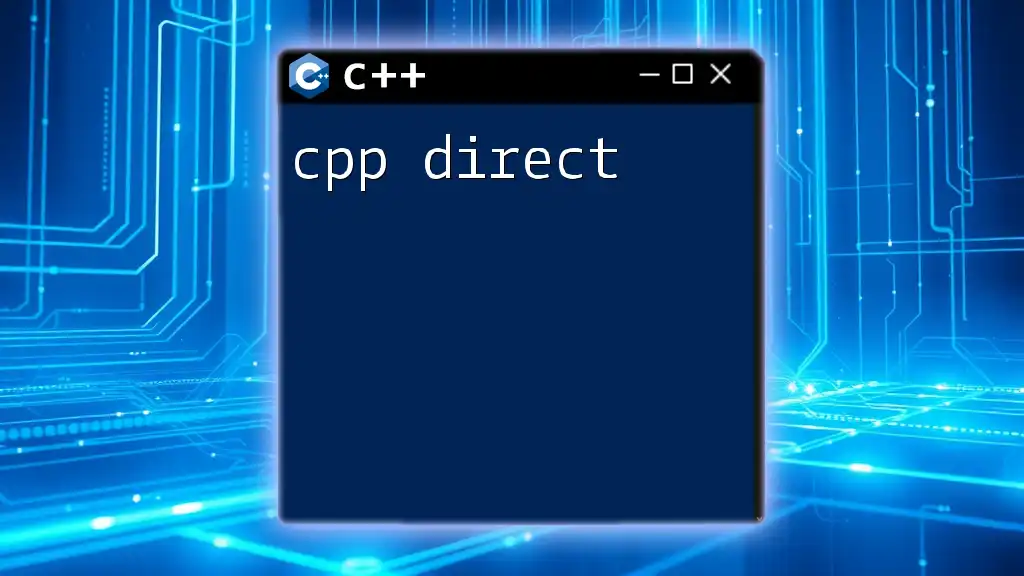
Additional Resources
Delve deeper into these topics using books, online tutorials, and collaborative coding platforms. Invest time in practice and continual learning, as mastery in C++ architecture opens up vast opportunities in software development.
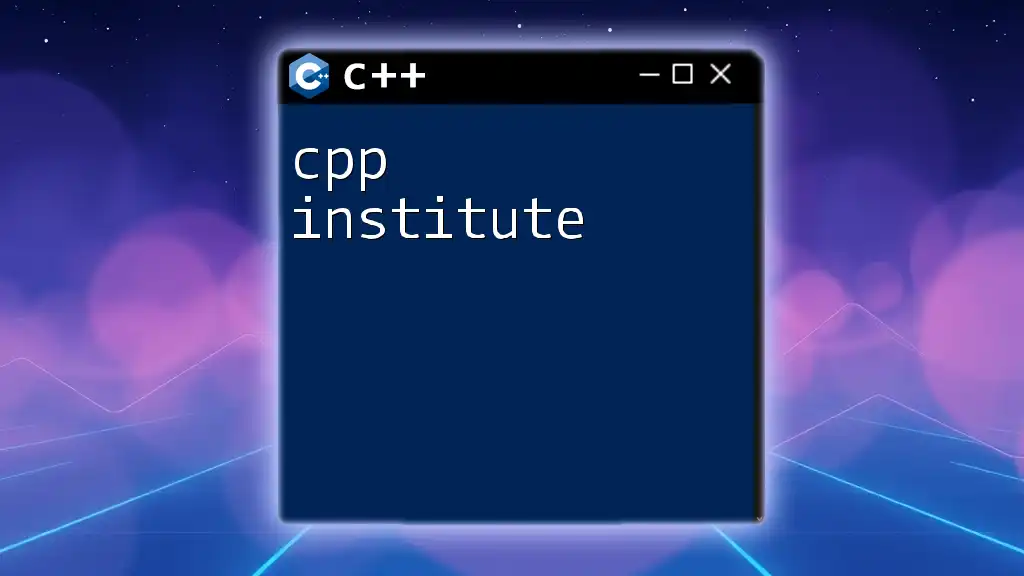
Call to Action
Join our workshops to gain hands-on experience in mastering C++ architecture. Engage with experts and fellow enthusiasts, and take your programming skills to the next level!