In this post, we will provide concise C++ practice questions to help you sharpen your programming skills efficiently.
#include <iostream>
using namespace std;
int main() {
// Question: Write a program to calculate the sum of two integers.
int a, b;
cout << "Enter two integers: ";
cin >> a >> b;
cout << "Sum: " << (a + b) << endl;
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful, high-level programming language widely used for building software applications. Developed by Bjarne Stroustrup, it adds object-oriented programming features to the C programming language, making it suitable for both low-level programming and high-level software development. C++ is known for its efficiency, flexibility, and performance benefits, which is why it remains popular in system/software development, game development, and in performance-critical applications.
Importance of C++ Practice
Practicing C++ commands and concepts is essential for several reasons:
-
Reinforcing Concepts: Regularly engaging with practice questions solidifies your understanding and helps you internalize how various components of C++ work.
-
Preparation for Interviews and Exams: Many technical interviews, especially in top tech companies, include coding assessments. Practicing C++ questions will prepare you for those challenges, increasing your confidence and competence.
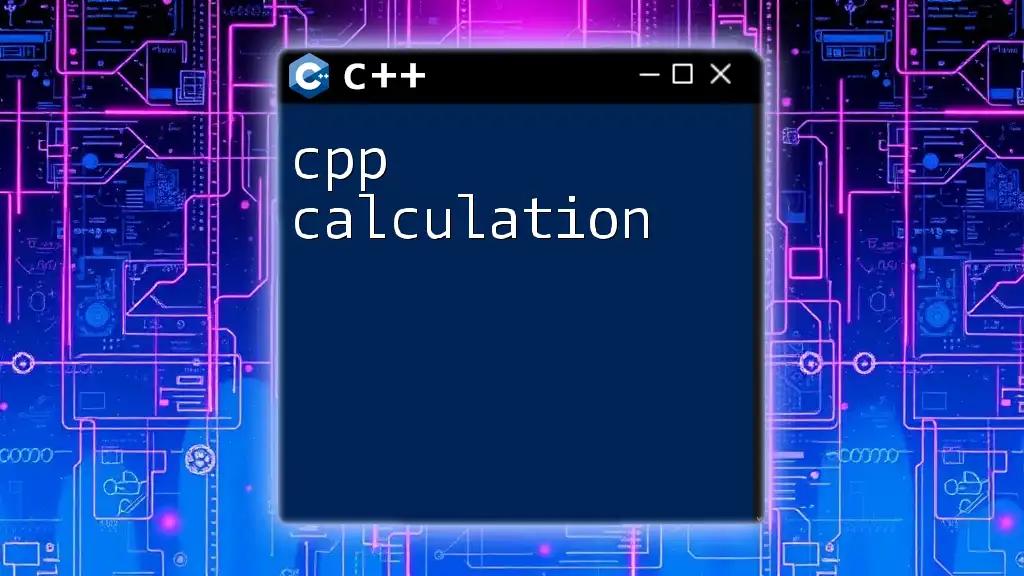
Types of C++ Practice Questions
Conceptual Questions
Conceptual questions assess your understanding of core principles of C++. These questions often require you to explain concepts rather than write code. For instance, you may be asked:
Discuss the difference between pointers and references.
Pointers are variables that hold the memory address of another variable, whereas references are an alias for another variable. Understanding these differences is crucial as they affect memory management and variable manipulation in C++.
Coding Questions
Coding questions test your ability to solve problems using actual code. Practicing these questions enhances your logical thinking and proficiency in syntax. An example would be:
Write a function to reverse a string in C++.
Here’s a simple implementation:
#include <iostream>
#include <string>
using namespace std;
string reverseString(const string &str) {
string reversed(str.rbegin(), str.rend());
return reversed;
}
In this code, we utilize the reverse iterator to create a new string that is the reverse of the input. This highlights the power of C++ string manipulation.
Debugging and Error Correction Questions
These questions provide you with a piece of code that contains bugs or logical errors. Your task is to identify the issues and correct them. For example:
Consider the following code that is supposed to calculate the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
if (n <= 1)
return 1;
else
return n * factorial(n-1);
}
int main() {
cout << "Factorial of 5 is: " << factorial(5) << endl;
return 0;
}
If the code were to input a negative number, it would lead to a stack overflow. Correcting such issues is part of becoming a skilled programmer.
Advanced C++ Questions
Advanced questions challenge you with complex concepts like inheritance, polymorphism, and templates. A common query would be:
Explain how templates work in C++.
Templates allow you to create generic functions or classes that can operate with any data type. Here’s a basic example:
#include <iostream>
using namespace std;
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << "Sum of integers: " << add(5, 3) << endl;
cout << "Sum of doubles: " << add(5.5, 3.2) << endl;
return 0;
}
In this code, `add` is a template function that can take parameters of any type, demonstrating the versatility and reusability offered by C++ templates.
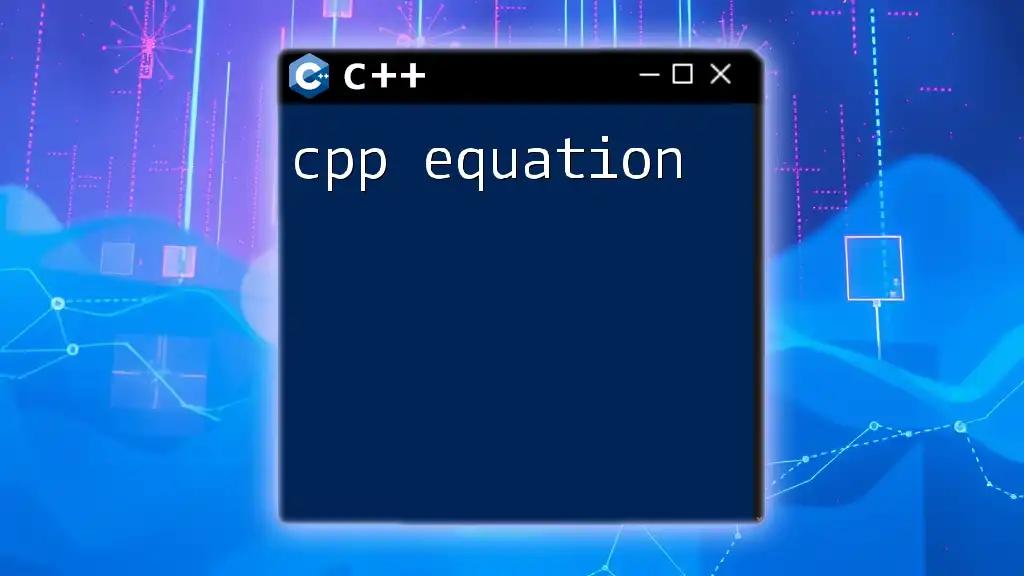
Example C++ Practice Questions
Basic Level Questions
Variables and Data Types
At a basic level, practice questions may look like this:
Declare an integer variable and initialize it.
The code would be:
int number = 10;
Understanding the concept of variable declaration and initialization forms the foundation of programming.
Intermediate Level Questions
Control Structures
As you progress, you will encounter questions involving control structures, such as:
Write a program using a loop to find the sum of the first ten integers.
Example implementation:
#include <iostream>
using namespace std;
int main() {
int sum = 0;
for (int i = 1; i <= 10; i++) {
sum += i;
}
cout << "The sum of the first ten integers is: " << sum << endl;
return 0;
}
This example illustrates the use of loops and fundamental arithmetic operators.
Advanced Level Questions
Object-Oriented Programming
Advanced questions will test your comprehension of OOP principles. Consider this query:
How do you create a class in C++?
Here’s a simple example:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
In this code, we define a class named `Dog` with a public method `bark()`, showcasing encapsulation and the basics of an object-oriented design.

Exploring Resources for C++ Practice
Online Platforms
Many online platforms offer a variety of C++ practice questions. Websites like LeetCode, HackerRank, and Codecademy provide structured practice, covering basic to advanced topics, thereby becoming invaluable resources for learners at any level.
Books and Study Materials
In addition to online resources, several books focus on C++ programming that include practice sections. Look for titles that emphasize exercises and problem-solving, providing a more comprehensive learning experience.
Community and Forums
Participating in online communities and forums is crucial for growth as a programmer. Platforms like Stack Overflow and Reddit not only allow you to ask questions but also enable you to learn from others' experiences and solutions. Engaging with a community fosters collaboration and can significantly enhance your understanding.
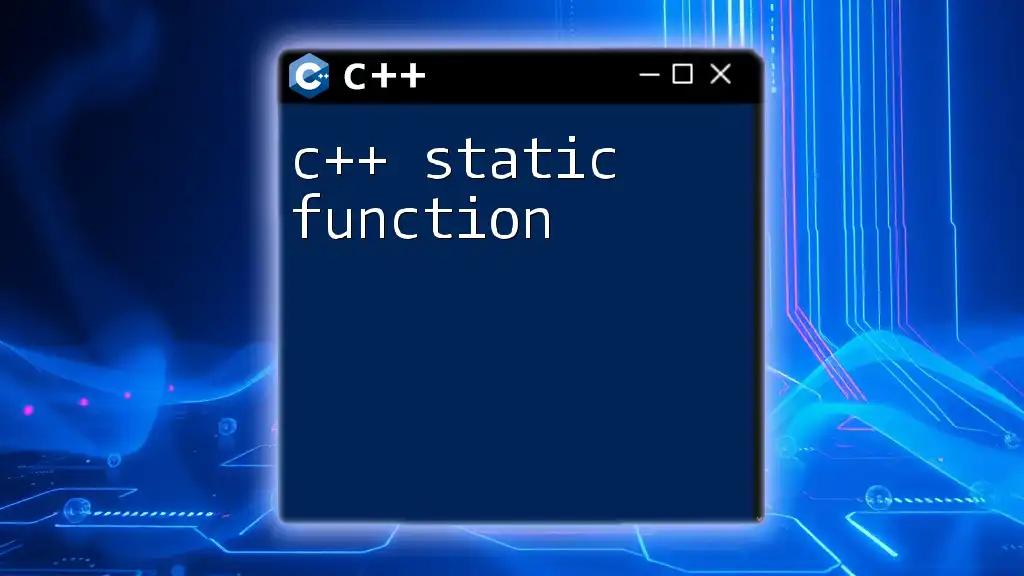
Strategies for Effective Practice
Time Management
Creating a consistent practice schedule is key to mastering C++. Rather than trying to cram information, regular practice ensures that you stay engaged and retain what you learn. Set aside dedicated time each day or week for C++ practice questions.
Analyzing Solutions
After solving problems, take the time to analyze the solutions, especially for questions you struggled with. Understanding the correct approach and debugging your own errors will deepen your learning and improve your problem-solving skills.
Building a Portfolio
Consider documenting your C++ projects. This approach not only reinforces your skills but also provides tangible proof of your programming capabilities. Creating a portfolio that showcases your projects can be a significant advantage during job interviews.
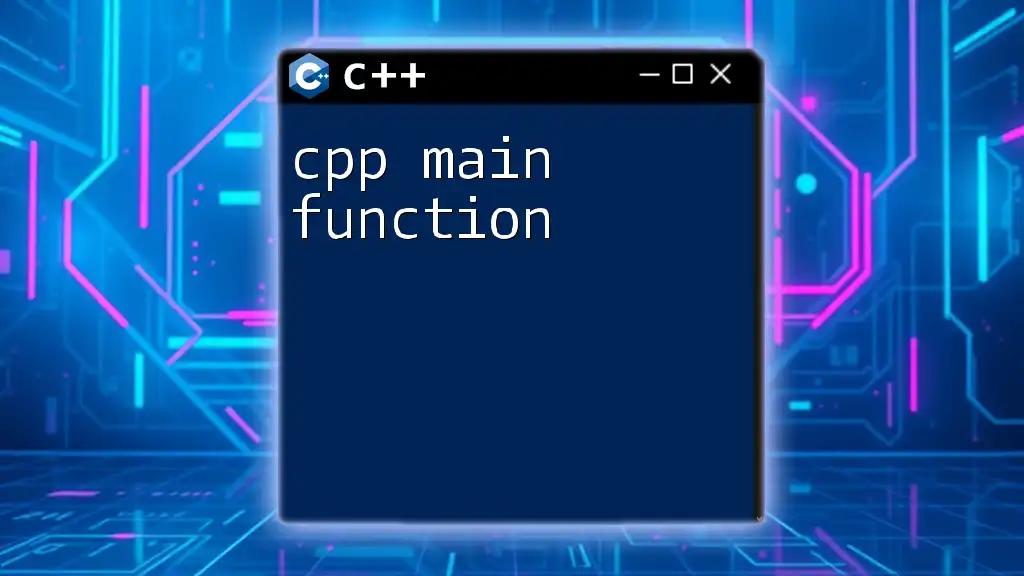
Conclusion
In summary, consistent practice with C++ practice questions is pivotal to reinforcing your knowledge of the language. By understanding and engaging with both basic and complex concepts through varied types of questions, you prepare yourself for real-world applications, technical interviews, and a successful career in programming.
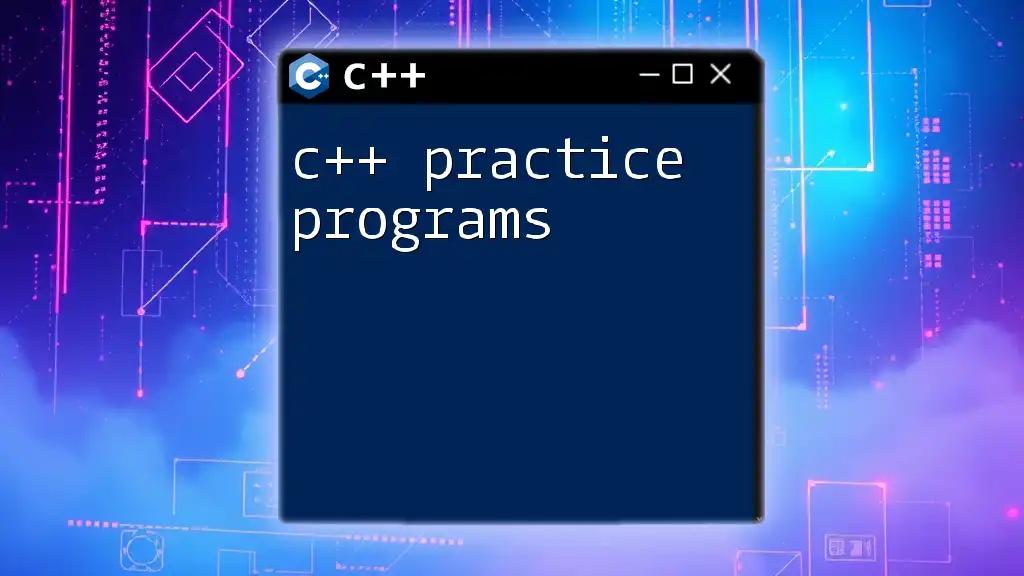
Call to Action
Start your C++ journey today by seeking out resources, joining communities, and committing to regular practice. The more you engage with C++ practice questions, the more proficient you will become!