To effectively use C++ commands, you need to have a C++ compiler and a basic understanding of programming concepts such as variables, control structures, and syntax.
Here’s an example of a simple C++ program that demonstrates the basic structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful, high-performance programming language that combines features of both high-level and low-level languages. Originally developed by Bjarne Stroustrup at Bell Labs in the early 1980s, C++ has evolved significantly over the years. Its design allows programmers to develop complex applications with added efficiency. Key features such as object-oriented programming, generic programming, and low-level memory manipulation set C++ apart in the programming world.
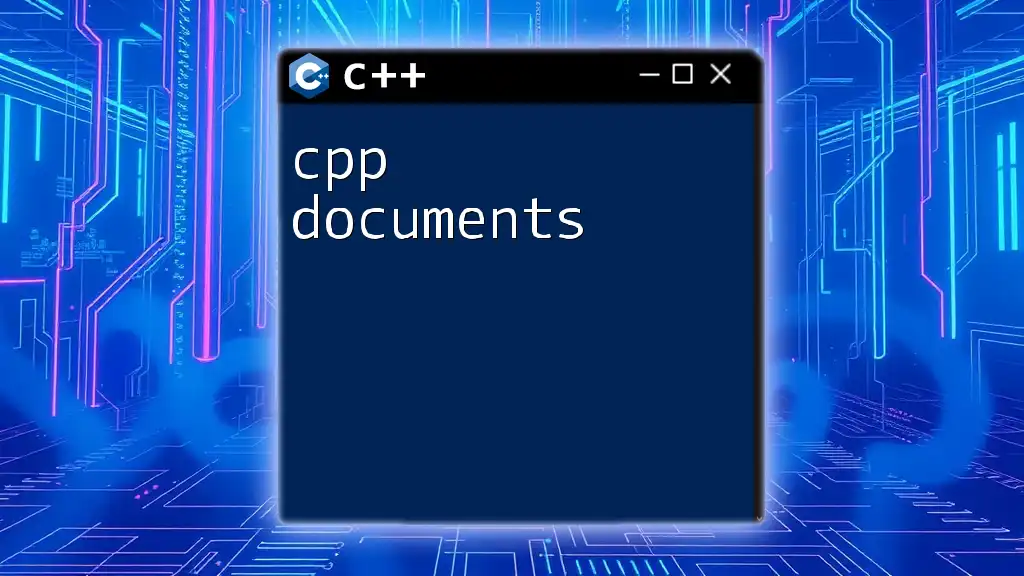
C++ Language Requirements
Compiler
A compiler is a crucial component in the process of developing C++ programs. It transforms the human-readable C++ code into machine code that the computer can execute. Understanding how to work with a compiler is essential for any C++ developer.
Role of the Compiler in C++
The compiler checks your code for syntax errors and translates your code into an executable format. Without a compiler, C++ programs cannot run.
Some popular compilers include:
- GCC (GNU Compiler Collection)
- Clang
- MSVC (Microsoft Visual C++)
Example: Basic Program Compilation Process
When you write a simple C++ program, you'll need to compile it with a command like this:
g++ hello.cpp -o hello
The `g++` command indicates you are using the GCC C++ compiler, `hello.cpp` is the source file, and `-o hello` specifies the output file name.
Standard Library
The C++ Standard Library is a powerful collection of classes and functions that help you avoid reinventing the wheel. This library includes components for input/output operations, string manipulations, memory management, and standard algorithms.
Importance of Including the Standard Library in Your Project
Using the Standard Library allows for more efficient code development, as it provides well-tested implementations of common data structures and algorithms.
Code Example: Including Headers and Using Standard Library Features
Here’s a simple example of using the C++ Standard Library:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1};
std::sort(numbers.begin(), numbers.end());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This program sorts a vector of integers and prints the sorted result.
Code Development Environment
Integrated Development Environment (IDE)
An IDE is a comprehensive software application that provides tools for software development. An IDE typically consists of a code editor, a compiler, a debugger, and other useful features.
Popular C++ IDEs:
- Visual Studio: A robust IDE with excellent debugging and GUI design capabilities.
- Code::Blocks: A free, open-source IDE designed for C++ programming.
- Eclipse: Known primarily for Java, but has strong support for C++ through plugins.
Benefits of Using an IDE for C++ Programming
An IDE streamlines the development process, offering features such as syntax highlighting, code completion, and project management. This makes coding faster and tends to reduce errors.
Example: Setting Up a Simple C++ Project in an IDE
To create a new project in Visual Studio:
- Open the IDE and select "Create a new project."
- Choose “Console Application” in the template section.
- Name your project and select a location.
- Start coding in the automatically created `main.cpp`.
Text Editors
In contrast to full-fledged IDEs, text editors give you a lighter alternative for C++ development. They typically lack integrated compilation and debugging features but are flexible and customizable.
Popular Text Editors for C++:
- Sublime Text: Fast, lightweight, with powerful features.
- Visual Studio Code: Free, open-source, and has extensive plugin support.
- Atom: A hackable and flexible text editor.
Customizing Your Text Editor for C++ Development
Most text editors allow you to install plugins that add syntax highlighting and build commands specifically for C++. For example, in Visual Studio Code, you can install the C/C++ extension from Microsoft, which enhances debugging and IntelliSense capabilities.
Operating System Compatibility
C++ is designed to be cross-platform. However, C++ code might behave differently depending on the operating system due to differences in compilers and available libraries.
Understanding How C++ Code Interacts with Different OS
When working on Windows, you might use MSVC, while on Linux, GCC is common. Being aware of these differences can help you write more portable code.
Example: Using Preprocessor Directives for OS-Specific Code
You can use preprocessor directives to compile different code based on the operating system:
#ifdef _WIN32
// Windows-specific code
#else
// Other OS code (e.g., Linux, Mac)
#endif
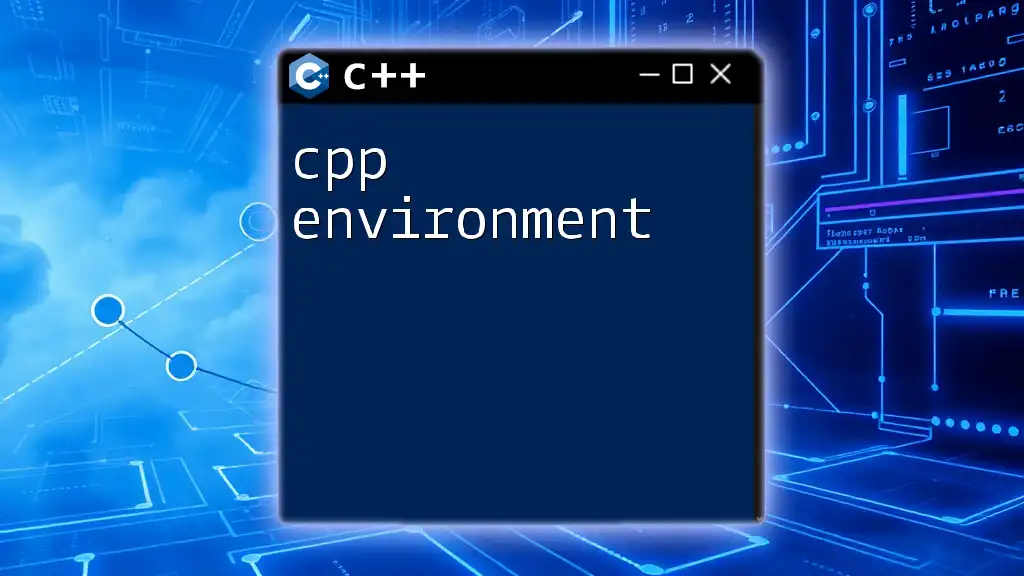
Coding Standards
Best Practices for C++ Coding
Coding standards are vital for maintaining readability and manageability of your code. Following established conventions helps you and your team to work together more effectively.
Overview of Common C++ Coding Guidelines:
- Use descriptive names for variables and classes.
- Maintain consistent formatting and indentation.
- Limit the complexity of functions to keep them understandable.
Example: A Simple Class Adhering to Coding Standards
class Rectangle {
private:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() {
return width * height;
}
};
This class uses clear naming conventions and encapsulates its data.
Comments and Documentation
Comments play a significant role in making C++ code maintainable. They help you convey intent, explain business logic, and make it easier for others (and future you) to understand your code.
Types of Comments in C++:
- Single-line comments: Use `//` for short explanations.
- Multi-line comments: Use `/* ... */` for longer descriptions.
Importance of Documentation
Writing clear documentation is essential. Using tools like Doxygen can automate the generation of documentation from your annotated code, making it easier for others to follow.
Example: Documenting a Function Properly
/**
* Calculates the area of a rectangle.
* @param width The width of the rectangle.
* @param height The height of the rectangle.
* @return The area of the rectangle.
*/
double calculateArea(double width, double height) {
return width * height;
}
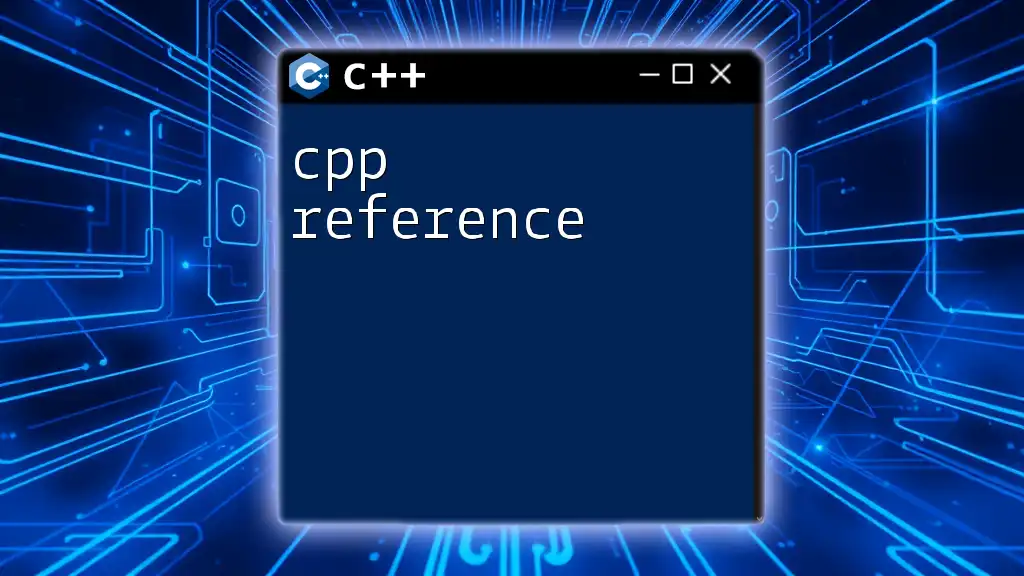
Required Knowledge and Skills
Fundamental Programming Concepts
Before diving deep into C++, it's important to have a solid understanding of fundamental programming concepts. This includes understanding variables, data types, control structures, and more.
Overview of Necessary Knowledge:
- Variables and Data Types: Know how to declare and use integers, floats, strings, etc.
- Control Structures: Mastering loops (for, while) and conditional statements (if-else, switch) is crucial.
Example: Basic C++ Program Using These Concepts
#include <iostream>
int main() {
int num = 10;
if (num > 5) {
std::cout << "Number is greater than 5." << std::endl;
}
return 0;
}
Object-Oriented Programming (OOP) Concepts
C++ is primarily known for its support of Object-Oriented Programming (OOP). Understanding OOP principles is critical for effective C++ programming.
Explanation of OOP Principles Relevant to C++:
- Encapsulation: Keeping data safe within classes.
- Inheritance: Creating new classes based on existing ones.
- Polymorphism: Allowing functions to work in different ways based on their input.
Example: A Simple Class Demonstrating OOP Principles
class Animal {
public:
virtual void speak() {
std::cout << "Animal speaks." << std::endl;
}
};
class Dog : public Animal {
public:
void speak() override {
std::cout << "Woof!" << std::endl;
}
};
In this example, we see both inheritance and polymorphism in action.
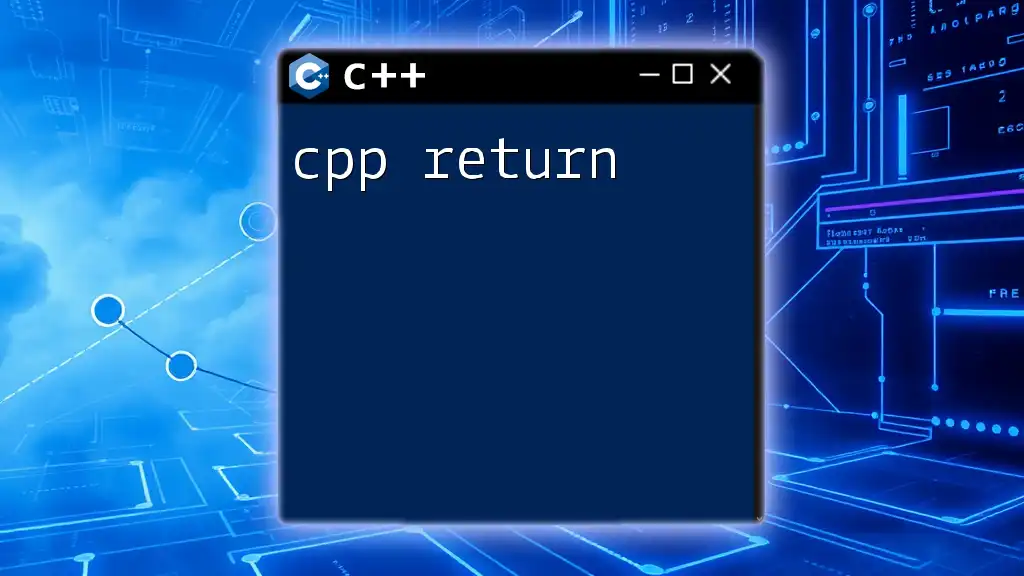
Additional Resources
Books and Online Courses
To deepen your understanding of C++, consider exploring various books and online learning platforms. Some recommended texts include:
- "C++ Primer" by Lippman: A great start for beginners.
- "Effective C++" by Scott Meyers: Offers best practices and insights for better C++ programming.
Additionally, platforms like Coursera, Udacity, and Udemy offer courses tailored to C++ for various skill levels.
Community and Forums
Never underestimate the power of community. Engaging with others in the C++ programming space can provide invaluable support and insight.
Importance of Community Support: Forums and online communities can help solve problems and share knowledge.
Popular C++ Forums and Communities:
- Stack Overflow: An excellent place for troubleshooting and asking programming questions.
- C++ Subreddit: A lively community with discussions, questions, and resources.
- Cplusplus.com Forum: A dedicated place for C++ developers to connect and share ideas.
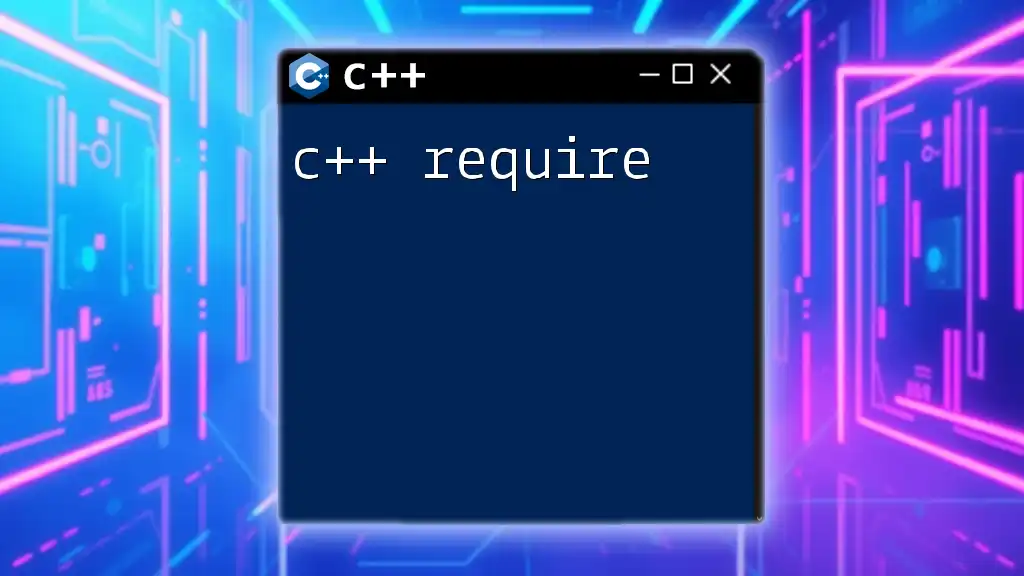
Conclusion
Understanding C++ requirements is fundamental for anyone looking to excel in C++ programming. The combination of a solid compiler, effective usage of the Standard Library, a conducive coding environment, awareness of coding standards, and an understanding of fundamental programming concepts will serve you well as you embark on your C++ journey. Start practicing, experiment with your code, and unlock the extensive capabilities that C++ has to offer. Happy coding!