A "cpp student" refers to individuals who are learning to program in C++ by practicing essential commands and syntax to enhance their coding skills.
Here's a simple code snippet as an example of a basic C++ program that outputs "Hello, World!":
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful, high-performance programming language that is widely used in various domains such as software development, game development, and system programming. Developed by Bjarne Stroustrup in the 1970s, C++ integrates object-oriented programming features into the C language, providing more flexibility and efficiency. As a C++ student, understanding its unique features compared to other languages like Java or Python is crucial. While C is procedural and simpler, C++ adds object-oriented capabilities, allowing for better data abstraction and code organization.
Setting Up Your C++ Environment
To start your journey as a C++ student, setting up your development environment is your first step. You will need a compiler and an Integrated Development Environment (IDE). Popular choices include:
- Microsoft Visual Studio
- Code::Blocks
- Dev-C++
Once installed, you can write your first program. Here’s a simple example of a Hello World program that showcases the basic structure of a C++ application:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code includes the iostream library and contains the main function, the entry point of every C++ program. It outputs the text "Hello, World!" to the console.
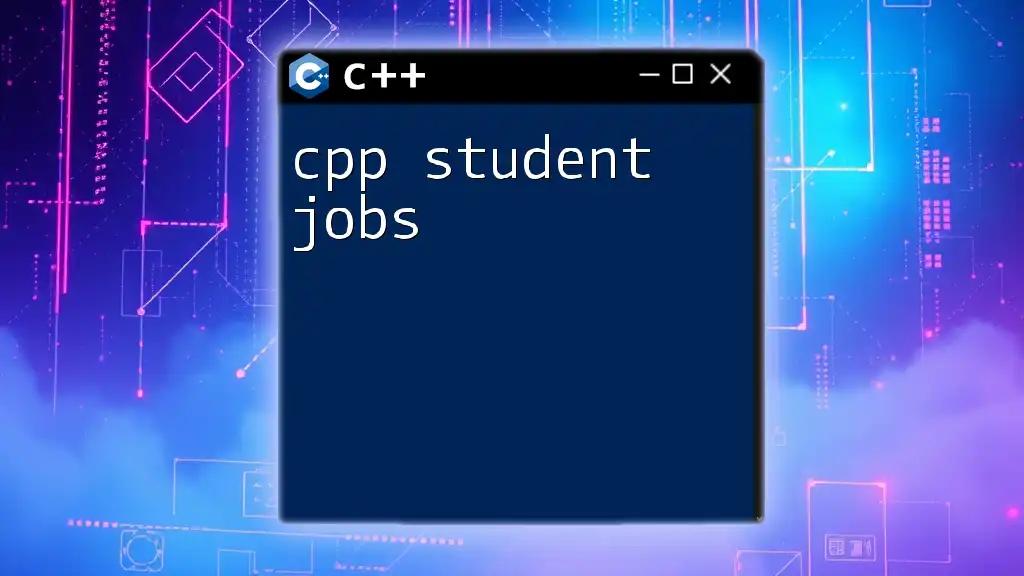
Core Concepts of C++
Variables and Data Types
Understanding variables and data types is fundamental for any C++ student. C++ supports various data types, each designed for different kinds of data. Common data types include:
- int for integers
- float for floating-point numbers
- char for single characters
- string for sequences of characters
Here’s how you can declare variables in C++:
int age = 25;
float height = 5.9;
char initial = 'A';
std::string name = "Alice";
It's important to understand the scope and lifetime of variables, which dictate where variables can be accessed in your code.
Control Structures
Conditional Statements
As a C++ student, mastering conditional statements is vital. These statements control the flow of execution based on given conditions. Here’s how you can use `if`, `else if`, and `else`:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
This code snippet checks the age and prints whether the person is an adult or a minor.
Loops: For, While, and Do-While
Loops allow you to execute code multiple times, making them essential for tasks like iterating over arrays or collections. Here’s a brief overview of different types of loops:
For Loop:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
This code will print numbers from 0 to 4.
While Loop:
int count = 0;
while (count < 5) {
std::cout << count << " ";
count++;
}
This loop continues until `count` reaches 5.
Functions
Defining and Calling Functions
Functions are crucial for code reusability and organization. Here’s how you can define and call a function in C++:
int add(int a, int b) {
return a + b;
}
std::cout << add(5, 10) << std::endl; // Outputs 15
This code defines a function `add`, which takes two integers and returns their sum.
Function Overloading
C++ allows you to have multiple functions with the same name as long as they differ in parameters (type or number). This is known as function overloading and can simplify your code:
int add(int a, int b) { /*...*/ }
double add(double a, double b) { /*...*/ }
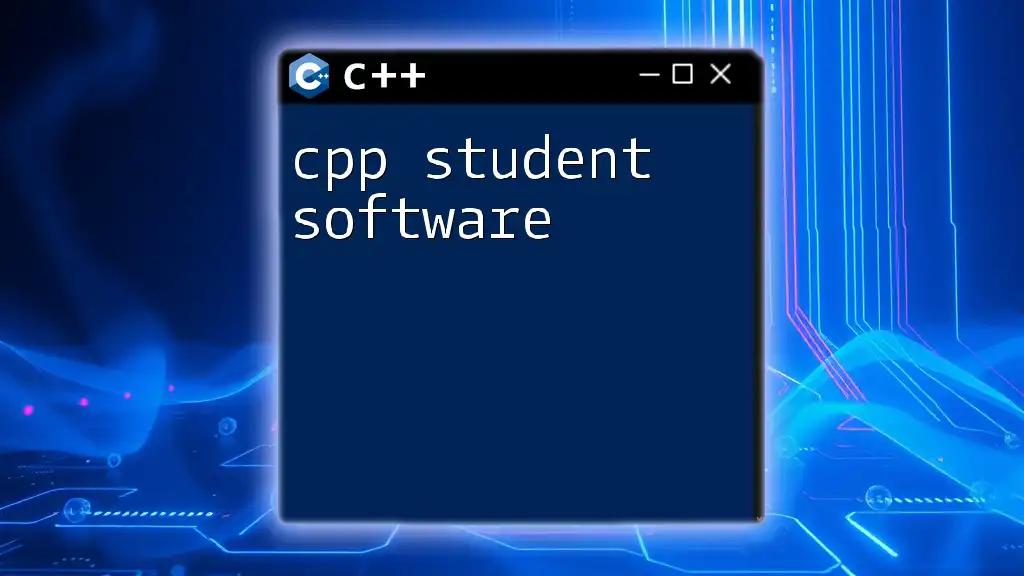
Object-Oriented Programming (OOP) in C++
Classes and Objects
As a C++ student, you’ll quickly encounter object-oriented programming, which is built around the concept of classes and objects. A class defines a blueprint for objects. Here’s a simple example:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Dog myDog;
myDog.bark(); // Outputs "Woof!"
Inheritance and Polymorphism
Inheritance allows a class to inherit properties and methods from another class, promoting code reuse. Here’s how it works in C++:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Cat : public Animal {
public:
void meow() {
std::cout << "Meow!" << std::endl;
}
};
Cat myCat;
myCat.eat(); // Outputs "Eating..."
Polymorphism allows for methods to do different things based on the object that it is acting upon, offering substantial flexibility in code implementation.
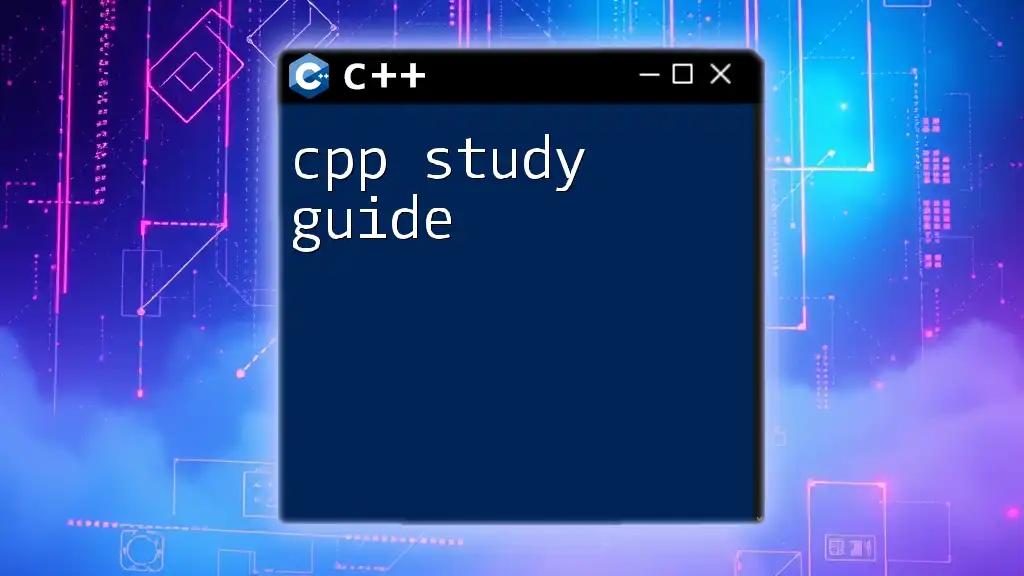
Best Practices for C++ Students
Writing Clean and Maintainable Code
For effective programming, it is vital to adopt good coding practices as a C++ student. Always strive for clean and maintainable code:
- Use meaningful variable names.
- Organize your code with proper indentation.
- Comment your code judiciously to explain complex logic.
Debugging Techniques
Learning how to debug effectively can significantly enhance your coding skills. Debugging tools such as integrated debuggers in IDEs can help you track down issues in your code. Be aware of common pitfalls:
- Segmentation faults: often caused by accessing invalid memory.
- Infinite loops: caused by incorrect loop conditions.
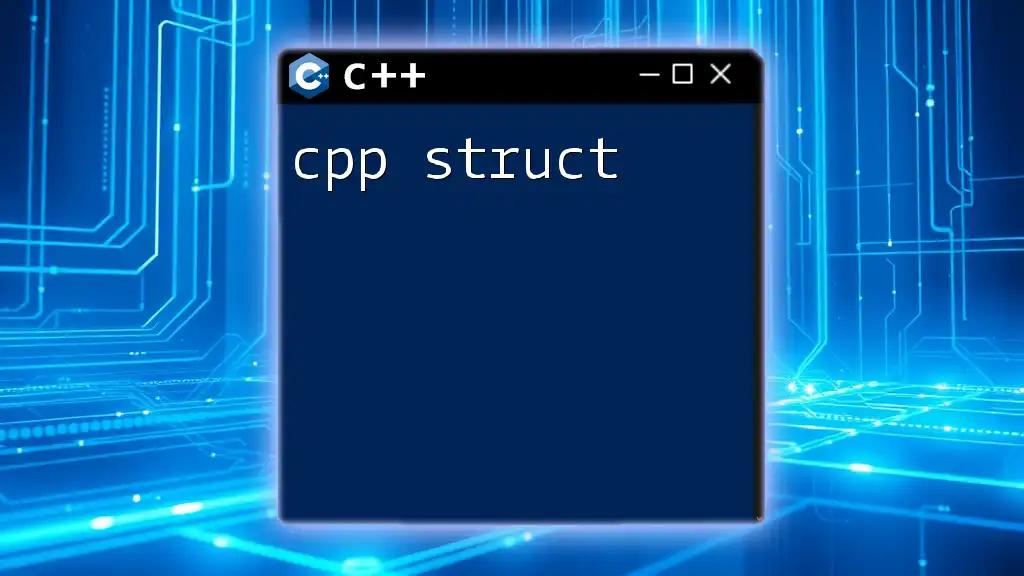
Resources for C++ Students
Books and Online Courses
To further your C++ knowledge, explore various books and online courses. Some recommended materials include:
- "Programming: Principles and Practice Using C++" by Bjarne Stroustrup
- "Effective C++" by Scott Meyers
Platforms like Codecademy, Coursera, and edX offer structured courses that can supplement your learning experience.
Community and Support
Joining programming communities like Stack Overflow, GitHub, and Reddit can provide invaluable support. Engaging with fellow C++ learners and experienced developers can help answer questions, solve problems, and deepen your understanding of C++.
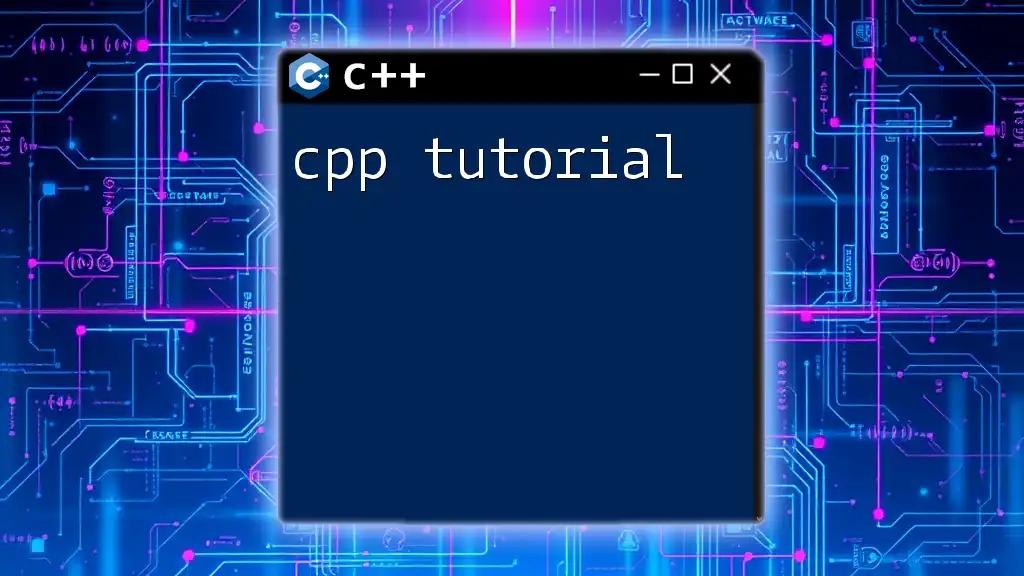
Conclusion
Being a C++ student involves continuous learning and practice. By mastering the concepts outlined in this guide—from fundamental programming structures to advanced OOP principles—you can develop a strong foundation in C++. Embrace the challenge of learning C++, and don’t hesitate to reach out for support and resources as you progress.
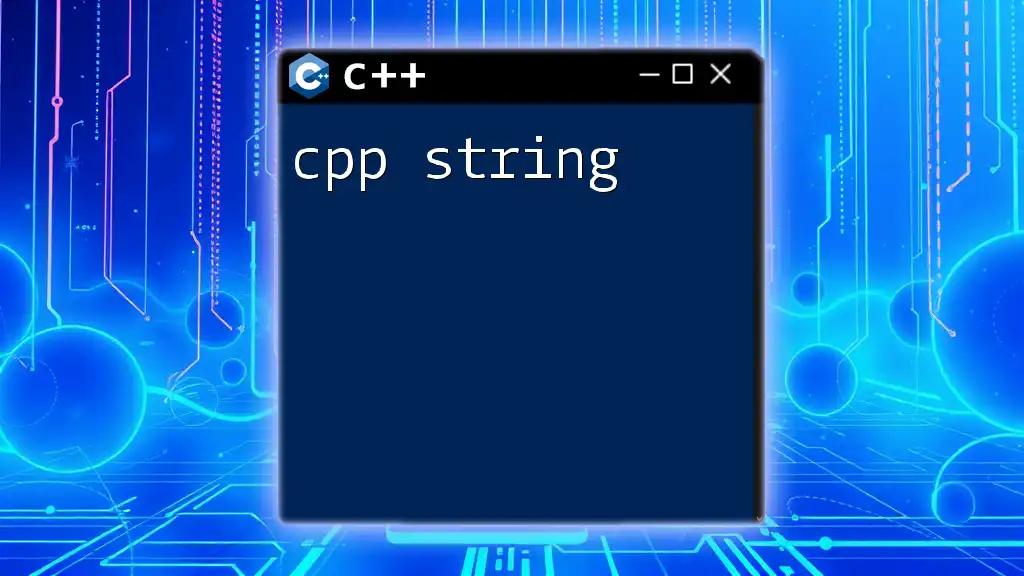
FAQs
As you grow in your understanding of C++, you'll encounter common questions and misconceptions. Don’t hesitate to explore these queries, as they lead to critical learning opportunities and deepen your grasp of this powerful programming language.

Call to Action
Take the next step in your C++ learning journey by enrolling in our courses and workshops, specifically designed to accelerate your understanding and mastery of C++. Whether you're beginning your C++ journey or looking to enhance your existing skills, we have tailored resources to meet your needs!