C++ syntax refers to the set of rules that define the combinations of symbols and tokens that are considered to be correctly structured C++ programs.
Here's a simple example of C++ syntax that demonstrates a basic `hello world` program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Basic C++ Syntax Rules
Structure of a C++ Program
Every C++ program has a fundamental structure centered around the main function, which serves as the entry point. The syntax for the main function is crucial as it dictates where execution begins. Here’s a simple example:
int main() {
return 0;
}
In this snippet, `int` indicates the return type of the function, which is an integer. The `return 0;` statement signifies successful execution of the program.
Case Sensitivity
C++ is case-sensitive, meaning that identifiers with the same letters but different cases are considered distinct. For instance, `Variable` and `variable` would be two separate identifiers. This feature plays a vital role in maintaining clarity and avoiding confusion in code.
Statements and Expressions
Understanding the difference between statements and expressions is essential in mastering C++ syntax. A statement is a complete unit of execution that performs an action, while an expression is a piece of code that evaluates to a value.
Example:
int x = 10; // Statement
x + 5; // Expression
In this case, `int x = 10;` is a statement that declares a variable, while `x + 5;` is an expression that computes a value.
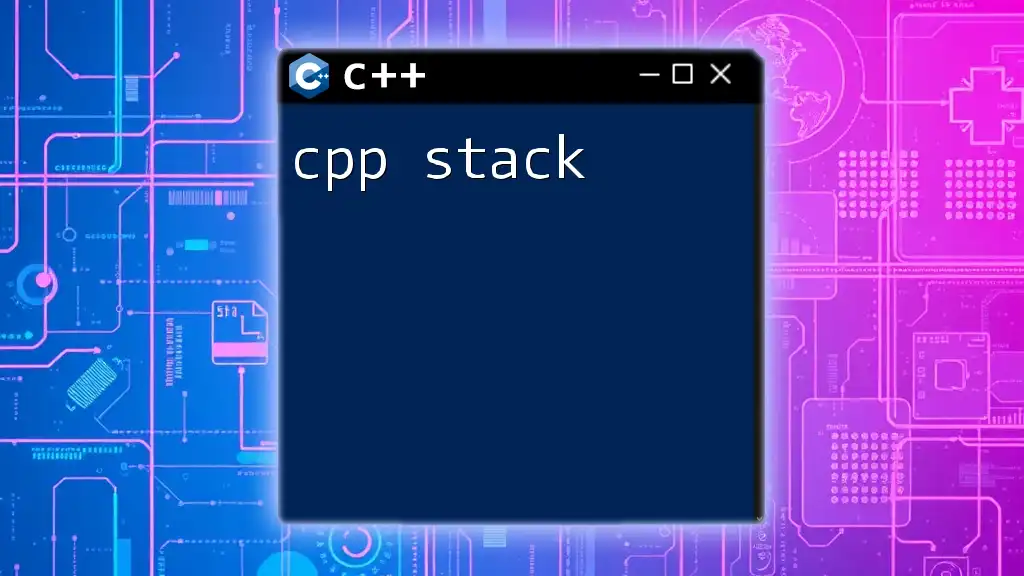
Variables and Data Types
Declaring Variables
Variables must be declared before they are used, which involves specifying the type of data they will store. The syntax for declaring variables is straightforward:
int number; // Integer
double price; // Double
char letter; // Character
This declaration tells the compiler what kind of data the variable will hold, setting the groundwork for proper memory allocation and type safety.
Data Types in C++
C++ offers a variety of built-in data types, categorized into fundamental types, which are crucial for efficient programming:
- `int`: Represents integers (whole numbers).
- `float`: Represents single-precision floating-point numbers.
- `double`: Represents double-precision floating-point numbers, allowing more precision.
- `char`: Represents a single character.
Example:
int age = 25; // Integer
float height = 5.8; // Float
char grade = 'A'; // Character
These types form the backbone of data manipulation in C++.
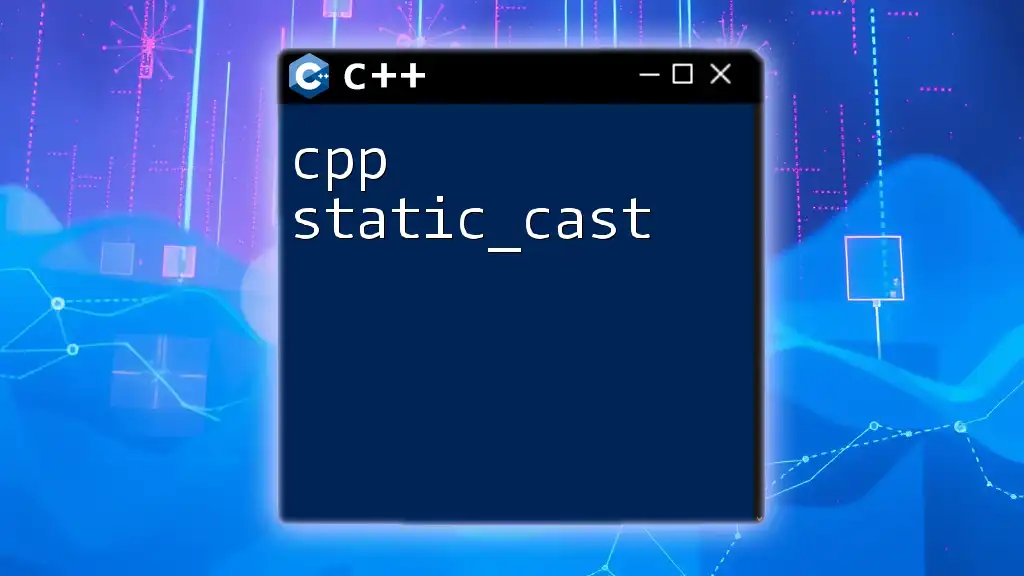
Control Structures
Conditional Statements
Conditional statements allow the program to make decisions based on certain conditions. The most common forms are `if`, `else if`, and `else`.
Example:
if (age > 18) {
cout << "Adult";
} else {
cout << "Minor";
}
This structure checks if the condition is true or false, executing different blocks of code based on the evaluation.
Loops
Loops allow code to run repeatedly based on a condition. The three main types of loops in C++ are `for`, `while`, and `do-while` loops.
Example of a `for` loop:
for (int i = 0; i < 5; i++) {
cout << i;
}
In this example, the loop iterates five times, printing the value of `i` during each iteration.
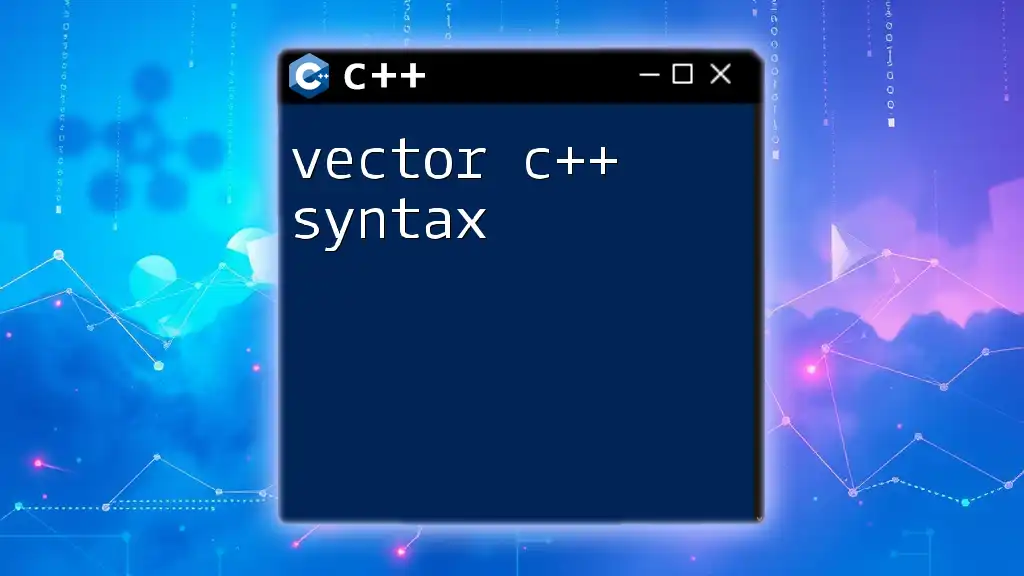
Functions in C++
Defining Functions
Functions in C++ enable code reusability and organization. The general syntax for defining a function consists of a return type, function name, parameters, and the function body.
Example:
int add(int a, int b) {
return a + b;
}
Here, `int add(int a, int b)` declares a function named `add` that takes two integer parameters and returns their sum.
Function Overloading
C++ allows multiple functions to have the same name with different parameters—a feature known as function overloading. This enhances flexibility and clarity in function use.
Example:
int add(int a, int b);
double add(double a, double b);
In this instance, there are two `add` functions that operate on different data types.
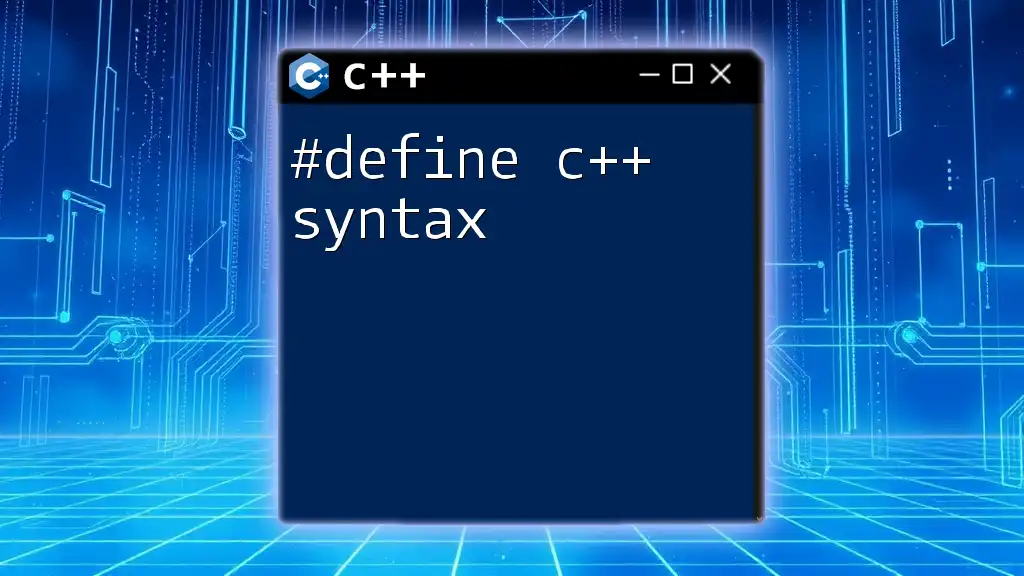
Object-Oriented Syntax
Classes and Objects
C++ is an object-oriented programming language, meaning it allows the creation of classes and objects. A class serves as a blueprint for creating objects, encapsulating both data and functions.
Example:
class Car {
public:
string color;
void drive();
};
In this code, a class named `Car` is defined with an attribute (`color`) and a member function (`drive()`), demonstrating the principles of encapsulation.
Constructor and Destructor
Constructors and destructors are special member functions in a class, handling initialization and cleanup processes, respectively.
Example:
class Car {
public:
Car() { /* constructor */ } // Initializes a Car object
~Car() { /* destructor */ } // Cleans up
};
The constructor executes when an object is created, setting initial states, while the destructor runs when the object is destroyed, cleaning up resources.
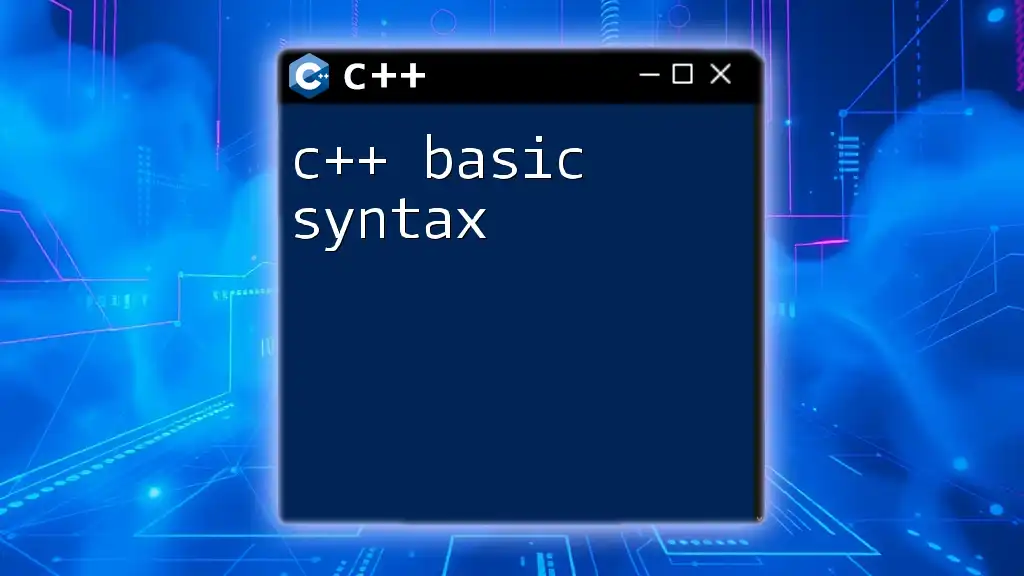
Exception Handling
Try, Catch, and Throw
C++ includes robust mechanisms for error handling through exception handling. The syntax involves the keywords `try`, `catch`, and `throw`.
Example:
try {
throw "An error occurred";
} catch (const char* msg) {
cout << msg;
}
The `try` block contains code that might throw an exception, while the `catch` block handles the exception, allowing the program to recover gracefully from unexpected errors.
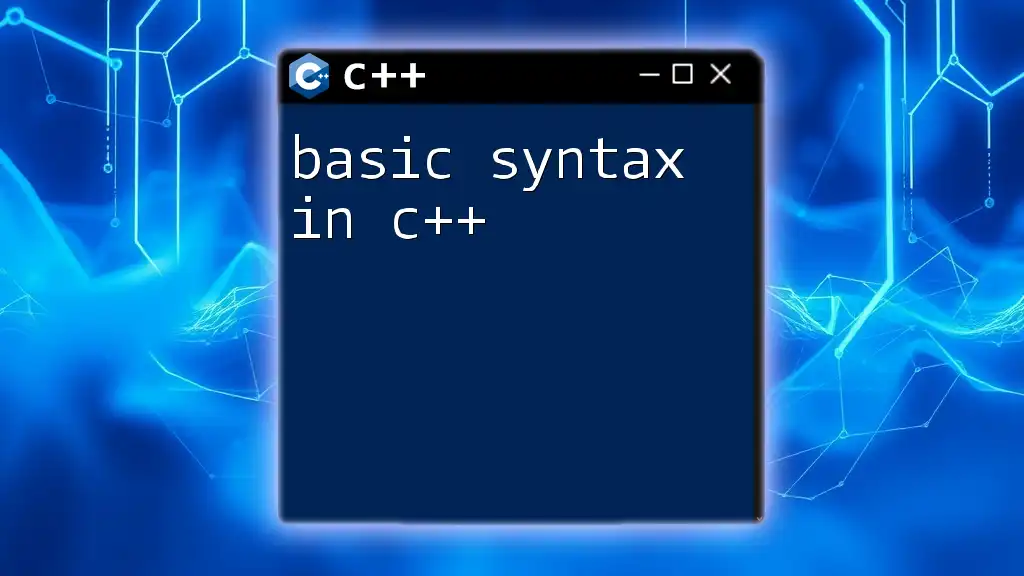
Conclusion
Mastering cpp syntax is not just about memorizing rules; it's about understanding the underlying principles that govern the language. By grasping concepts such as variable declaration, control structures, functions, object-oriented programming, and exception handling, you can elevate your programming skills to new heights.
Engaging with C++ syntax regularly will enhance your coding fluency and prepare you for more complex coding challenges. We invite you to explore further and consider joining our training program for a deeper understanding of C++ and how to use its commands effectively.
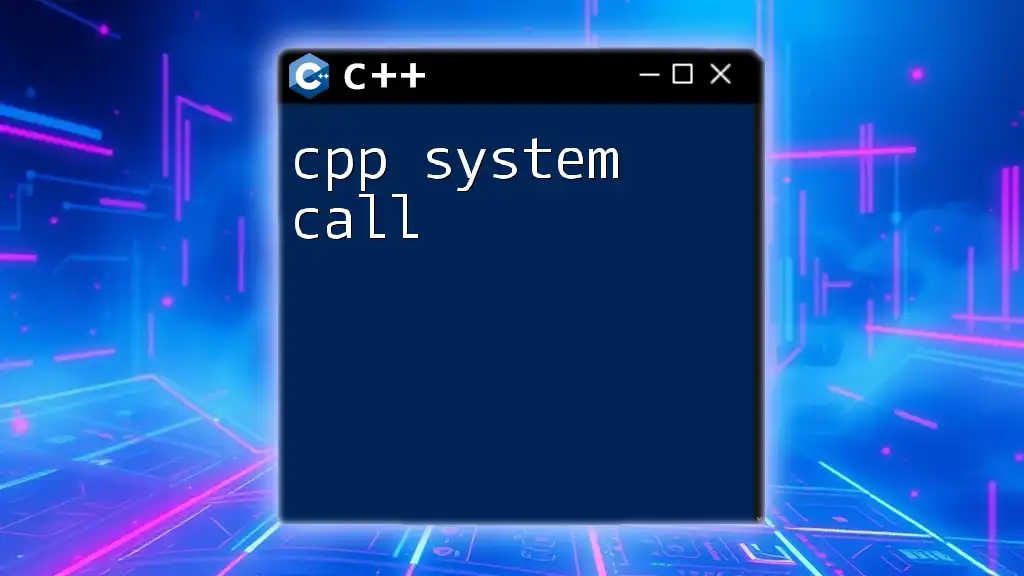
FAQs about C++ Syntax
What is the difference between C++ and C?
C++ is an extension of C that introduces object-oriented features, allowing for the creation of classes and objects, whereas C is procedural.
Is C++ syntax similar to Java?
While C++ and Java share similar syntax and programming concepts, they have different paradigms, memory management, and libraries.
How can I improve my understanding of C++ syntax?
Practice consistently by writing code, exploring projects, and using online resources, such as coding forums and tutorial websites. Additionally, engaging with a community can deepen your understanding and provide support.