The `sscanf` function in C++ is used to read formatted input from a string, allowing you to extract data from it based on a specified format.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <sstream>
int main() {
const char* str = "100 200.5 Hello";
int a;
float b;
char c[20];
sscanf(str, "%d %f %s", &a, &b, c);
std::cout << "Integer: " << a << ", Float: " << b << ", String: " << c << std::endl;
return 0;
}
What is sscanf?
The function sscanf is a powerful input function primarily used for reading formatted data from strings. In the context of C++, it provides a way to parse strings quickly, making it integral for applications where input comes in a specific format.
Key Differences Between sscanf in C and C++
While sscanf is traditionally a C function, its utility extends seamlessly into C++. The primary difference lies in style and integration within C++'s object-oriented paradigm. C++ offers enhanced type safety and other abstractions that can complement the use of sscanf.
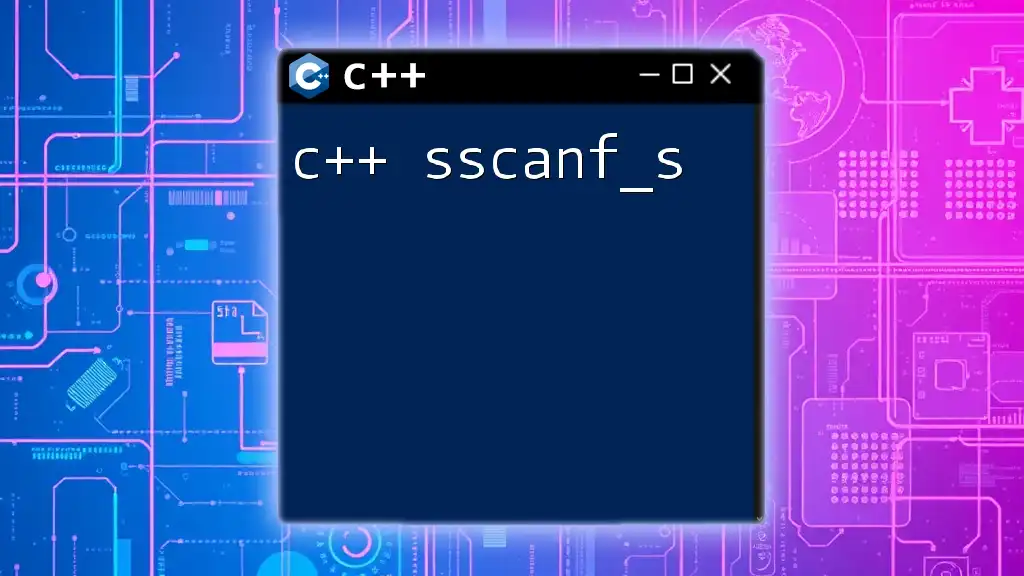
Syntax of sscanf
The syntax of sscanf can be broken down as follows:
int sscanf(const char *str, const char *format, ...);
Explanation of Parameters
- str: The input string that contains the data to be parsed.
- format: A string that specifies how to read the data. It consists of format specifiers that define the expected type of each element in the string.
- ...: A variable number of arguments that are pointers to the variables where the parsed values will be stored.
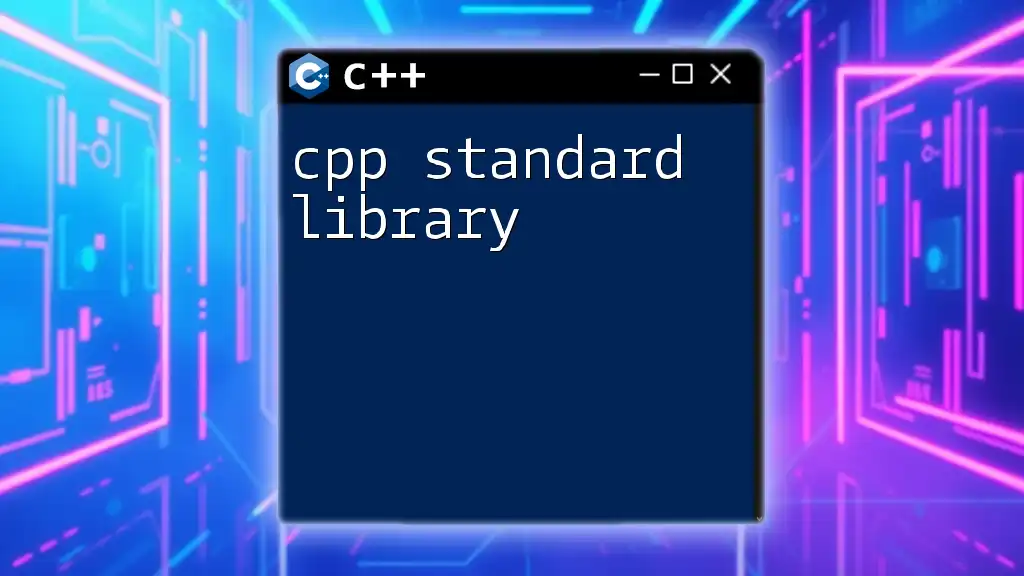
Common Format Specifiers
Understanding the format specifiers is crucial for using sscanf effectively. Here are some of the most commonly used ones:
- %d: Reads an integer.
- %f: Reads a floating-point number.
- %s: Reads a string until whitespace is encountered.
- %c: Reads a single character.
- %x: Reads a hexadecimal number.
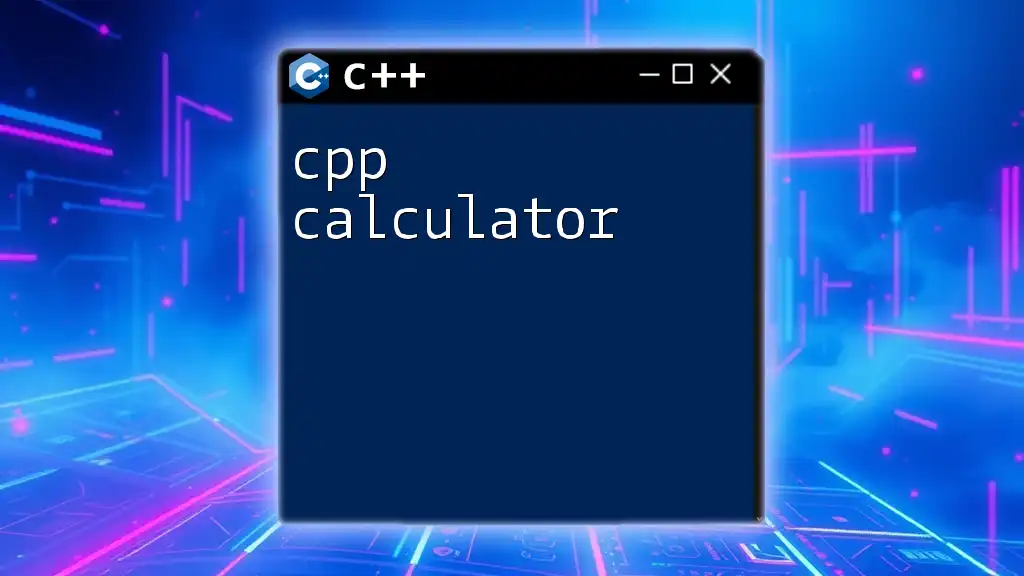
Understanding Return Value of sscanf
The return value of sscanf is particularly important:
- It returns the number of input items successfully matched and assigned. This value can be less than the number of format specifiers if the input data does not match the expected format.
- If the return value is EOF (end of file), it indicates an error or that no input items were matched. It’s vital to check this return value to ensure correct parsing.
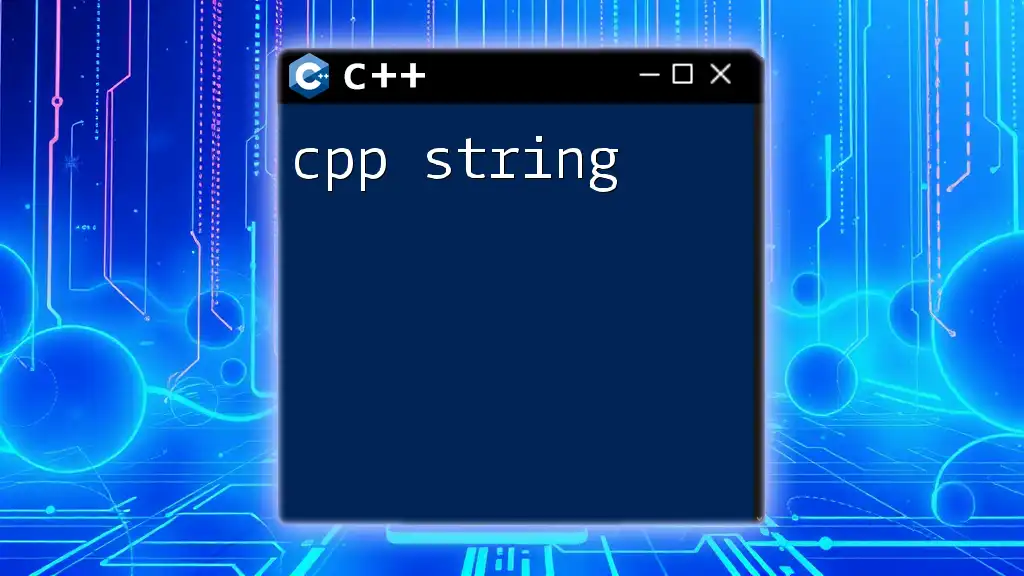
Examples of Using sscanf in C++
Simple Example: Parsing Integers
Here is a straightforward example that demonstrates how to parse an integer from a string:
#include <iostream>
#include <cstdio>
int main() {
const char *data = "42";
int number;
sscanf(data, "%d", &number);
std::cout << "Parsed number: " << number << std::endl;
return 0;
}
In this code, the string `"42"` is passed to sscanf, which reads it and assigns the parsed integer to the variable `number`. The output will be: `Parsed number: 42`.
Parsing Multiple Values
sscanf can also handle multiple values in a single string. Consider the following example:
#include <iostream>
#include <cstdio>
int main() {
const char *data = "John 25 180.5";
char name[50];
int age;
float height;
sscanf(data, "%s %d %f", name, &age, &height);
std::cout << "Name: " << name << ", Age: " << age << ", Height: " << height << std::endl;
return 0;
}
Here, the function reads three values from the string: a string (`name`), an integer (`age`), and a floating-point number (`height`). The output will be: `Name: John, Age: 25, Height: 180.5`.
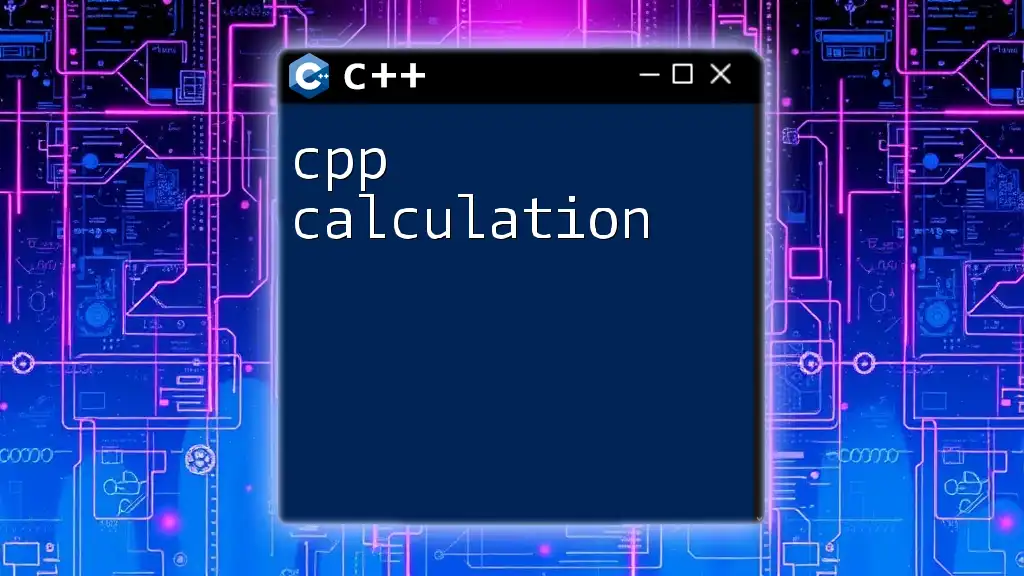
Advanced Usage of sscanf
Using Custom Format Specifiers
One of the strengths of sscanf is its ability to handle custom format strings. For example, if you have a string that contains a date in the format "YYYY-MM-DD", you can parse it like this:
#include <iostream>
#include <cstdio>
int main() {
const char *date = "2023-10-15";
int year, month, day;
sscanf(date, "%d-%d-%d", &year, &month, &day);
std::cout << "Year: " << year << ", Month: " << month << ", Day: " << day << std::endl;
return 0;
}
In this snippet, the format string matches the structure of the date, allowing for precise parsing.
Handling Edge Cases
Improperly formatted input can lead to issues. Here’s how to manage these scenarios effectively:
- Dealing with Incorrect Formats: If the input does not match the expected format, sscanf will stop parsing. Always check the return value to determine how many items were successfully matched.
- Implementing Error Handling: You can leverage the return value to manage parsing errors. If only one value is matched when multiple were expected, it signals that something went wrong.
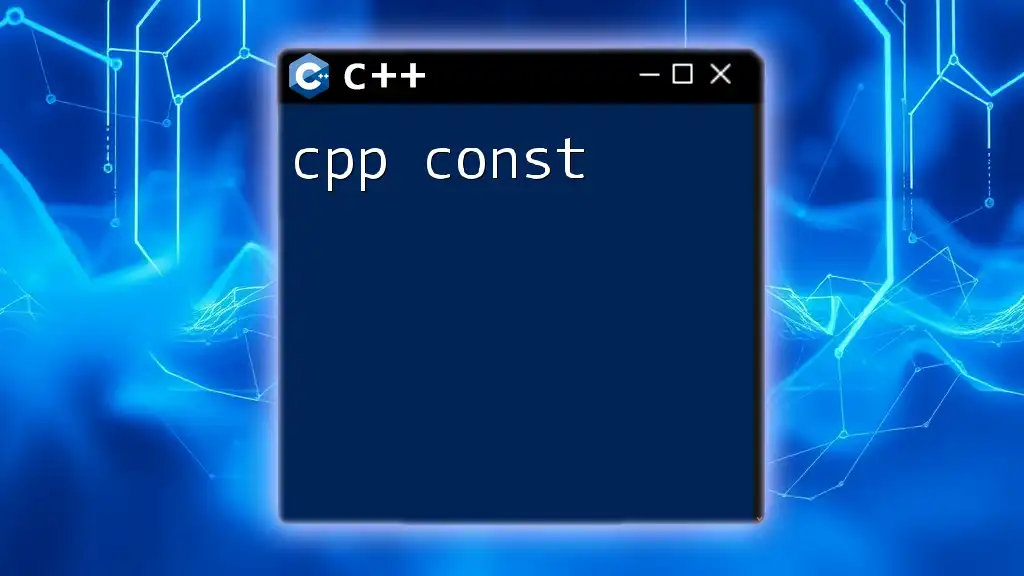
Common Pitfalls to Avoid with sscanf
When utilizing sscanf, there are several common pitfalls to be aware of:
- Buffer Overflow: Since %s does not limit input size, ensure to allocate sufficient buffer size to prevent overflow errors.
- Misinterpretation of Return Values: Always check the return value to avoid assumptions about successful parsing.
- Format Specifier Mismatches: Ensure that the data types in the format string correspond to the types of the variables being passed.
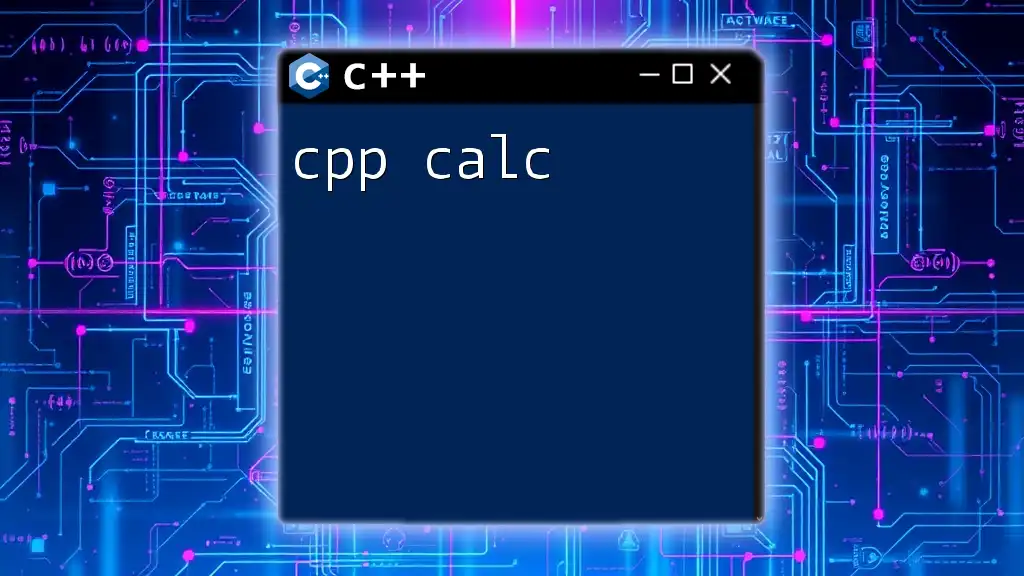
Best Practices when Using sscanf
Performance Considerations
If your application requires frequent parsing of formatted strings, consider profiling different methods. For large inputs or complex parsing, alternatives like regex or specialized libraries might offer improved performance.
Security Implications
Input validation is critical when using sscanf. Unsanitized data can lead to vulnerabilities. Always validate the source and format of the data you are parsing to mitigate potential risks.
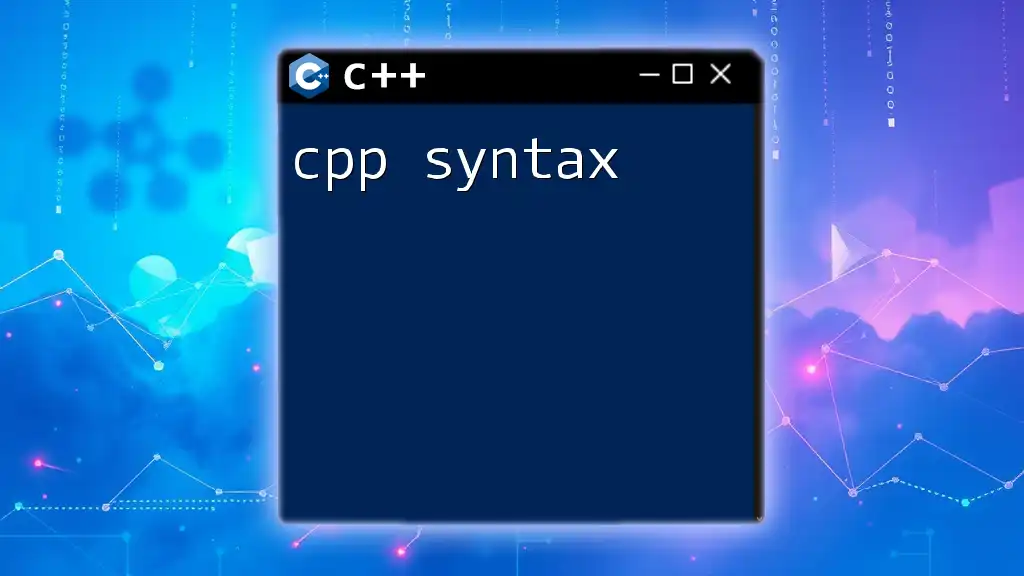
Conclusion
In summary, cpp sscanf is a vital tool for developers looking to parse formatted input from strings. Its flexibility, combined with careful handling of return values and input validation, allows for robust parsing scenarios. Practice with different formats and edge cases to become proficient in its usage, enhancing your C++ programming skills.
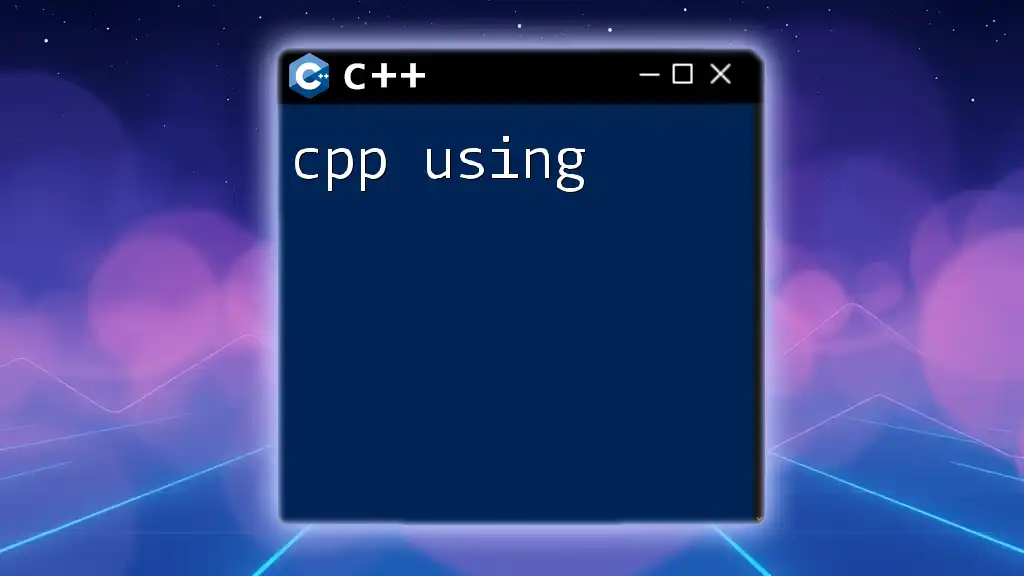
Further Reading
For those eager to deepen their understanding of C++ input handling, consider exploring additional resources, including books focused on C++ best practices and online documentation. Engaging with community forums can also provide practical insights and help resolve any specific queries regarding sscanf and C++ programming at large.